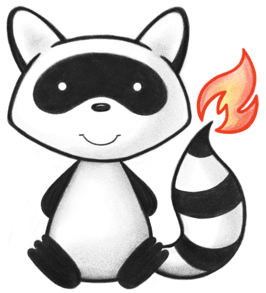
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.delete; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.jpa.api.model.DeleteConflict; 025import ca.uhn.fhir.jpa.api.model.DeleteConflictList; 026import ca.uhn.fhir.jpa.dao.BaseStorageDao; 027import ca.uhn.fhir.rest.server.exceptions.ResourceVersionConflictException; 028import ca.uhn.fhir.util.OperationOutcomeUtil; 029import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 030 031public final class DeleteConflictUtil { 032 private DeleteConflictUtil() {} 033 034 public static void validateDeleteConflictsEmptyOrThrowException( 035 FhirContext theFhirContext, DeleteConflictList theDeleteConflicts) { 036 IBaseOperationOutcome oo = null; 037 String firstMsg = null; 038 039 for (DeleteConflict next : theDeleteConflicts) { 040 041 if (theDeleteConflicts.isResourceIdToIgnoreConflict(next.getTargetId())) { 042 continue; 043 } 044 045 String msg = "Unable to delete " 046 + next.getTargetId().toUnqualifiedVersionless().getValue() 047 + " because at least one resource has a reference to this resource. First reference found was resource " 048 + next.getSourceId().toUnqualifiedVersionless().getValue() 049 + " in path " 050 + next.getSourcePath(); 051 052 if (firstMsg == null) { 053 firstMsg = msg; 054 oo = OperationOutcomeUtil.newInstance(theFhirContext); 055 } 056 OperationOutcomeUtil.addIssue( 057 theFhirContext, oo, BaseStorageDao.OO_SEVERITY_ERROR, msg, null, "processing"); 058 } 059 060 if (firstMsg == null) { 061 return; 062 } 063 064 throw new ResourceVersionConflictException(Msg.code(515) + firstMsg, oo); 065 } 066}