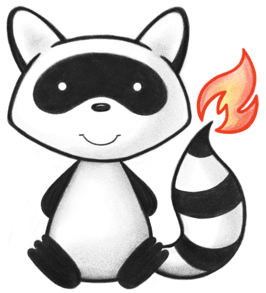
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.interceptor.validation; 021 022import ca.uhn.fhir.rest.api.server.RequestDetails; 023import jakarta.annotation.Nonnull; 024import org.apache.commons.lang3.Validate; 025import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027 028/** 029 * This is an internal API for HAPI FHIR. It is subject to change without warning. 030 */ 031public interface IRepositoryValidatingRule { 032 033 @Nonnull 034 String getResourceType(); 035 036 @Nonnull 037 RuleEvaluation evaluate(RequestDetails theRequestDetails, @Nonnull IBaseResource theResource); 038 039 class RuleEvaluation { 040 041 private final IBaseOperationOutcome myOperationOutcome; 042 private IRepositoryValidatingRule myRule; 043 private boolean myPasses; 044 private String myFailureDescription; 045 046 private RuleEvaluation( 047 IRepositoryValidatingRule theRule, 048 boolean thePasses, 049 String theFailureDescription, 050 IBaseOperationOutcome theOperationOutcome) { 051 myRule = theRule; 052 myPasses = thePasses; 053 myFailureDescription = theFailureDescription; 054 myOperationOutcome = theOperationOutcome; 055 } 056 057 static RuleEvaluation forSuccess(IRepositoryValidatingRule theRule) { 058 Validate.notNull(theRule); 059 return new RuleEvaluation(theRule, true, null, null); 060 } 061 062 static RuleEvaluation forFailure(IRepositoryValidatingRule theRule, String theFailureDescription) { 063 Validate.notNull(theRule); 064 Validate.notNull(theFailureDescription); 065 return new RuleEvaluation(theRule, false, theFailureDescription, null); 066 } 067 068 static RuleEvaluation forFailure(IRepositoryValidatingRule theRule, IBaseOperationOutcome theOperationOutcome) { 069 Validate.notNull(theRule); 070 Validate.notNull(theOperationOutcome); 071 return new RuleEvaluation(theRule, false, null, theOperationOutcome); 072 } 073 074 public IBaseOperationOutcome getOperationOutcome() { 075 return myOperationOutcome; 076 } 077 078 public IRepositoryValidatingRule getRule() { 079 return myRule; 080 } 081 082 public boolean isPasses() { 083 return myPasses; 084 } 085 086 public String getFailureDescription() { 087 return myFailureDescription; 088 } 089 } 090}