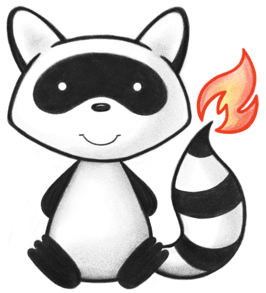
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.search; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.model.api.Include; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId; 026import ca.uhn.fhir.rest.param.DateRangeParam; 027import jakarta.persistence.EntityManager; 028 029import java.util.ArrayList; 030import java.util.Collection; 031import java.util.List; 032 033public class SearchBuilderLoadIncludesParameters<T extends IResourcePersistentId> { 034 035 private FhirContext myFhirContext; 036 037 private EntityManager myEntityManager; 038 039 /** 040 * A collection of already obtained PIDs 041 */ 042 private Collection<T> myMatches; 043 044 /** 045 * A collection of fhirpaths to include in the search. 046 * Used to search for resources recursively. 047 */ 048 private Collection<Include> myIncludeFilters; 049 050 private boolean myReverseMode; 051 052 private DateRangeParam myLastUpdated; 053 054 private String mySearchIdOrDescription; 055 056 private RequestDetails myRequestDetails; 057 058 private Integer myMaxCount; 059 060 /** 061 * List of resource types of interest. 062 * If specified, only these resource types are returned. 063 * 064 * This may have performance issues as TARGET_RESOURCE_TYPE is not 065 * an indexed field. 066 * 067 * Use sparingly 068 */ 069 private List<String> myDesiredResourceTypes; 070 071 public FhirContext getFhirContext() { 072 return myFhirContext; 073 } 074 075 public void setFhirContext(FhirContext theFhirContext) { 076 myFhirContext = theFhirContext; 077 } 078 079 public EntityManager getEntityManager() { 080 return myEntityManager; 081 } 082 083 public void setEntityManager(EntityManager theEntityManager) { 084 myEntityManager = theEntityManager; 085 } 086 087 public Collection<T> getMatches() { 088 if (myMatches == null) { 089 myMatches = new ArrayList<>(); 090 } 091 return myMatches; 092 } 093 094 public void setMatches(Collection<T> theMatches) { 095 myMatches = theMatches; 096 } 097 098 public Collection<Include> getIncludeFilters() { 099 return myIncludeFilters; 100 } 101 102 public void setIncludeFilters(Collection<Include> theIncludeFilters) { 103 myIncludeFilters = theIncludeFilters; 104 } 105 106 public boolean isReverseMode() { 107 return myReverseMode; 108 } 109 110 public void setReverseMode(boolean theReverseMode) { 111 myReverseMode = theReverseMode; 112 } 113 114 public DateRangeParam getLastUpdated() { 115 return myLastUpdated; 116 } 117 118 public void setLastUpdated(DateRangeParam theLastUpdated) { 119 myLastUpdated = theLastUpdated; 120 } 121 122 public String getSearchIdOrDescription() { 123 return mySearchIdOrDescription; 124 } 125 126 public void setSearchIdOrDescription(String theSearchIdOrDescription) { 127 mySearchIdOrDescription = theSearchIdOrDescription; 128 } 129 130 public RequestDetails getRequestDetails() { 131 return myRequestDetails; 132 } 133 134 public void setRequestDetails(RequestDetails theRequestDetails) { 135 myRequestDetails = theRequestDetails; 136 } 137 138 public Integer getMaxCount() { 139 return myMaxCount; 140 } 141 142 public void setMaxCount(Integer theMaxCount) { 143 myMaxCount = theMaxCount; 144 } 145 146 public List<String> getDesiredResourceTypes() { 147 return myDesiredResourceTypes; 148 } 149 150 public void setDesiredResourceTypes(List<String> theDesiredResourceTypes) { 151 myDesiredResourceTypes = theDesiredResourceTypes; 152 } 153}