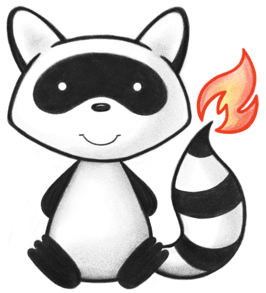
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.search; 021 022import ca.uhn.fhir.rest.api.server.RequestDetails; 023import ca.uhn.fhir.util.StopWatch; 024import jakarta.annotation.Nullable; 025 026/** 027 * This class contains a runtime in-memory description of a search operation, 028 * including details on processing time and other things 029 */ 030public class SearchRuntimeDetails { 031 private final String mySearchUuid; 032 private final RequestDetails myRequestDetails; 033 private StopWatch myQueryStopwatch; 034 private int myFoundMatchesCount; 035 private boolean myLoadSynchronous; 036 private String myQueryString; 037 private SearchStatusEnum mySearchStatus; 038 private int myFoundIndexMatchesCount; 039 040 public SearchRuntimeDetails(RequestDetails theRequestDetails, String theSearchUuid) { 041 myRequestDetails = theRequestDetails; 042 mySearchUuid = theSearchUuid; 043 } 044 045 @Nullable 046 public RequestDetails getRequestDetails() { 047 return myRequestDetails; 048 } 049 050 public String getSearchUuid() { 051 return mySearchUuid; 052 } 053 054 public StopWatch getQueryStopwatch() { 055 return myQueryStopwatch; 056 } 057 058 public void setQueryStopwatch(StopWatch theQueryStopwatch) { 059 myQueryStopwatch = theQueryStopwatch; 060 } 061 062 public int getFoundMatchesCount() { 063 return myFoundMatchesCount; 064 } 065 066 public void setFoundMatchesCount(int theFoundMatchesCount) { 067 myFoundMatchesCount = theFoundMatchesCount; 068 } 069 070 public int getFoundIndexMatchesCount() { 071 return myFoundIndexMatchesCount; 072 } 073 074 public void setFoundIndexMatchesCount(int theFoundIndexMatchesCount) { 075 myFoundIndexMatchesCount = theFoundIndexMatchesCount; 076 } 077 078 public boolean getLoadSynchronous() { 079 return myLoadSynchronous; 080 } 081 082 public void setLoadSynchronous(boolean theLoadSynchronous) { 083 myLoadSynchronous = theLoadSynchronous; 084 } 085 086 public String getQueryString() { 087 return myQueryString; 088 } 089 090 public void setQueryString(String theQueryString) { 091 myQueryString = theQueryString; 092 } 093 094 public SearchStatusEnum getSearchStatus() { 095 return mySearchStatus; 096 } 097 098 public void setSearchStatus(SearchStatusEnum theSearchStatus) { 099 mySearchStatus = theSearchStatus; 100 } 101}