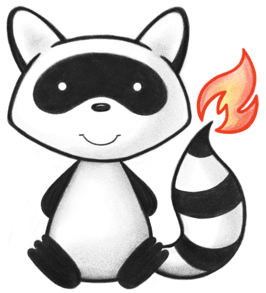
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.provider; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.jpa.model.util.JpaConstants; 024import ca.uhn.fhir.jpa.subscription.triggering.ISubscriptionTriggeringSvc; 025import ca.uhn.fhir.model.dstu2.valueset.ResourceTypeEnum; 026import ca.uhn.fhir.rest.annotation.IdParam; 027import ca.uhn.fhir.rest.annotation.Operation; 028import ca.uhn.fhir.rest.annotation.OperationParam; 029import ca.uhn.fhir.rest.server.IResourceProvider; 030import ca.uhn.fhir.rest.server.provider.ProviderConstants; 031import org.hl7.fhir.instance.model.api.IBaseParameters; 032import org.hl7.fhir.instance.model.api.IBaseResource; 033import org.hl7.fhir.instance.model.api.IIdType; 034import org.hl7.fhir.instance.model.api.IPrimitiveType; 035import org.springframework.beans.factory.annotation.Autowired; 036 037import java.util.List; 038 039public class SubscriptionTriggeringProvider implements IResourceProvider { 040 @Autowired 041 private FhirContext myFhirContext; 042 043 @Autowired 044 private ISubscriptionTriggeringSvc mySubscriptionTriggeringSvc; 045 046 @Operation(name = JpaConstants.OPERATION_TRIGGER_SUBSCRIPTION) 047 public IBaseParameters triggerSubscription( 048 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 049 @OperationParam( 050 name = ProviderConstants.SUBSCRIPTION_TRIGGERING_PARAM_RESOURCE_ID, 051 min = 0, 052 max = OperationParam.MAX_UNLIMITED, 053 typeName = "uri") 054 List<IPrimitiveType<String>> theResourceIds, 055 @OperationParam( 056 name = ProviderConstants.SUBSCRIPTION_TRIGGERING_PARAM_SEARCH_URL, 057 min = 0, 058 max = OperationParam.MAX_UNLIMITED, 059 typeName = "string") 060 List<IPrimitiveType<String>> theSearchUrls) { 061 return mySubscriptionTriggeringSvc.triggerSubscription(theResourceIds, theSearchUrls, null, theRequestDetails); 062 } 063 064 @Operation(name = JpaConstants.OPERATION_TRIGGER_SUBSCRIPTION) 065 public IBaseParameters triggerSubscription( 066 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 067 @IdParam IIdType theSubscriptionId, 068 @OperationParam( 069 name = ProviderConstants.SUBSCRIPTION_TRIGGERING_PARAM_RESOURCE_ID, 070 min = 0, 071 max = OperationParam.MAX_UNLIMITED, 072 typeName = "uri") 073 List<IPrimitiveType<String>> theResourceIds, 074 @OperationParam( 075 name = ProviderConstants.SUBSCRIPTION_TRIGGERING_PARAM_SEARCH_URL, 076 min = 0, 077 max = OperationParam.MAX_UNLIMITED, 078 typeName = "string") 079 List<IPrimitiveType<String>> theSearchUrls) { 080 return mySubscriptionTriggeringSvc.triggerSubscription( 081 theResourceIds, theSearchUrls, theSubscriptionId, theRequestDetails); 082 } 083 084 @Override 085 public Class<? extends IBaseResource> getResourceType() { 086 return myFhirContext 087 .getResourceDefinition(ResourceTypeEnum.SUBSCRIPTION.getCode()) 088 .getImplementingClass(); 089 } 090}