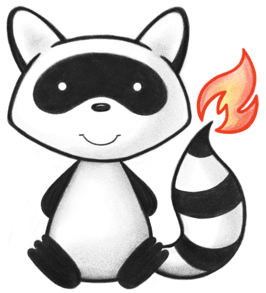
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.channel.api; 021 022import ca.uhn.fhir.jpa.subscription.channel.subscription.IChannelNamer; 023 024/** 025 * This interface is the factory for Queue Channels, which are the low level abstraction over a 026 * queue (e.g. memory queue, JMS queue, Kafka stream, etc.) for any purpose. 027 */ 028public interface IChannelFactory { 029 030 /** 031 * Create a channel that is used to receive messages from the queue. 032 * 033 * <p> 034 * Implementations can choose to return the same object for multiple invocations of this method (and {@link #getOrCreateReceiver(String, Class, ChannelConsumerSettings)} 035 * when invoked with the same {@literal theChannelName} if they need to, or they can create a new instance. 036 * </p> 037 * 038 * @param theChannelName The actual underlying queue name 039 * @param theMessageType The object type that will be placed on this queue. Objects will be Jackson-annotated structures. 040 * @param theChannelSettings Contains the configuration for subscribers. 041 */ 042 IChannelReceiver getOrCreateReceiver( 043 String theChannelName, Class<?> theMessageType, ChannelConsumerSettings theChannelSettings); 044 045 /** 046 * Create a channel that is used to send messages to the queue. 047 * 048 * <p> 049 * Implementations can choose to return the same object for multiple invocations of this method (and {@link #getOrCreateReceiver(String, Class, ChannelConsumerSettings)} 050 * when invoked with the same {@literal theChannelName} if they need to, or they can create a new instance. 051 * </p> 052 * 053 * @param theChannelName The actual underlying queue name 054 * @param theMessageType The object type that will be placed on this queue. Objects will be Jackson-annotated structures. 055 * @param theChannelSettings Contains the configuration for senders. 056 */ 057 IChannelProducer getOrCreateProducer( 058 String theChannelName, Class<?> theMessageType, ChannelProducerSettings theChannelSettings); 059 060 /** 061 * @return the IChannelNamer used by this factory 062 */ 063 IChannelNamer getChannelNamer(); 064}