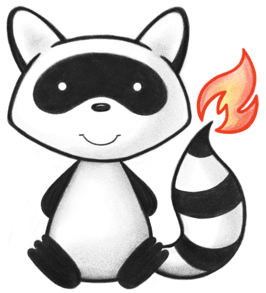
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.model; 021 022import ca.uhn.fhir.i18n.Msg; 023import jakarta.annotation.Nonnull; 024import jakarta.annotation.Nullable; 025import org.hl7.fhir.dstu2.model.Subscription; 026import org.hl7.fhir.exceptions.FHIRException; 027 028import static org.apache.commons.lang3.StringUtils.isBlank; 029 030public enum CanonicalSubscriptionChannelType { 031 /** 032 * The channel is executed by making a post to the URI. If a payload is included, the URL is interpreted as the service base, and an update (PUT) is made. 033 */ 034 RESTHOOK, 035 /** 036 * The channel is executed by sending a packet across a web socket connection maintained by the client. The URL identifies the websocket, and the client binds to this URL. 037 */ 038 WEBSOCKET, 039 /** 040 * The channel is executed by sending an email to the email addressed in the URI (which must be a mailto:). 041 */ 042 EMAIL, 043 /** 044 * The channel is executed by sending an SMS message to the phone number identified in the URL (tel:). 045 */ 046 SMS, 047 /** 048 * The channel is executed by sending a message (e.g. a Bundle with a MessageHeader resource etc.) to the application identified in the URI. 049 */ 050 MESSAGE, 051 /** 052 * added to help the parsers with the generic types 053 */ 054 NULL; 055 056 public static CanonicalSubscriptionChannelType fromCode(@Nullable String theSystem, @Nonnull String codeString) 057 throws FHIRException { 058 if (isBlank(codeString)) { 059 return null; 060 } else if ("rest-hook".equals(codeString)) { 061 if (theSystem == null 062 || theSystem.equals("http://terminology.hl7.org/CodeSystem/subscription-channel-type")) { 063 return RESTHOOK; 064 } 065 } else if ("websocket".equals(codeString)) { 066 if (theSystem == null 067 || theSystem.equals("http://terminology.hl7.org/CodeSystem/subscription-channel-type")) { 068 return WEBSOCKET; 069 } 070 } else if ("email".equals(codeString)) { 071 if (theSystem == null 072 || theSystem.equals("http://terminology.hl7.org/CodeSystem/subscription-channel-type")) { 073 return EMAIL; 074 } 075 } else if ("sms".equals(codeString)) { 076 if (theSystem == null 077 || theSystem.equals("http://terminology.hl7.org/CodeSystem/subscription-channel-type")) { 078 return SMS; 079 } 080 } else if ("message".equals(codeString)) { 081 if (theSystem == null 082 || theSystem.equals("http://terminology.hl7.org/CodeSystem/subscription-channel-type")) { 083 return MESSAGE; 084 } 085 } 086 087 throw new FHIRException(Msg.code(569) + "Unknown SubscriptionChannelType code '" + codeString + "'"); 088 } 089 090 public String toCode() { 091 switch (this) { 092 case RESTHOOK: 093 return "rest-hook"; 094 case WEBSOCKET: 095 return "websocket"; 096 case EMAIL: 097 return "email"; 098 case SMS: 099 return "sms"; 100 case MESSAGE: 101 return "message"; 102 case NULL: 103 default: 104 return "?"; 105 } 106 } 107 108 public String getSystem() { 109 switch (this) { 110 case RESTHOOK: 111 case WEBSOCKET: 112 case EMAIL: 113 case SMS: 114 case MESSAGE: 115 return "http://terminology.hl7.org/CodeSystem/subscription-channel-type"; 116 case NULL: 117 default: 118 return "?"; 119 } 120 } 121 122 public String getDefinition() { 123 switch (this) { 124 case RESTHOOK: 125 return "The channel is executed by making a post to the URI. If a payload is included, the URL is interpreted as the service base, and an update (PUT) is made."; 126 case WEBSOCKET: 127 return "The channel is executed by sending a packet across a web socket connection maintained by the client. The URL identifies the websocket, and the client binds to this URL."; 128 case EMAIL: 129 return "The channel is executed by sending an email to the email addressed in the URI (which must be a mailto:)."; 130 case SMS: 131 return "The channel is executed by sending an SMS message to the phone number identified in the URL (tel:)."; 132 case MESSAGE: 133 return "The channel is executed by sending a message (e.g. a Bundle with a MessageHeader resource etc.) to the application identified in the URI."; 134 case NULL: 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDisplay() { 141 switch (this) { 142 case RESTHOOK: 143 return "Rest Hook"; 144 case WEBSOCKET: 145 return "Websocket"; 146 case EMAIL: 147 return "Email"; 148 case SMS: 149 return "SMS"; 150 case MESSAGE: 151 return "Message"; 152 case NULL: 153 default: 154 return "?"; 155 } 156 } 157 158 public Subscription.SubscriptionChannelType toCanonical() { 159 return Subscription.SubscriptionChannelType.fromCode(toCode()); 160 } 161}