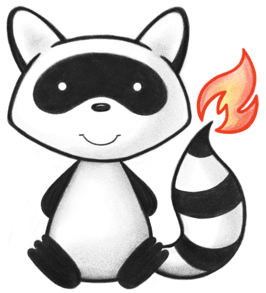
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.model; 021 022import com.fasterxml.jackson.annotation.JsonProperty; 023import org.apache.commons.lang3.builder.EqualsBuilder; 024import org.apache.commons.lang3.builder.HashCodeBuilder; 025import org.hl7.fhir.r5.model.Subscription; 026 027import java.util.ArrayList; 028import java.util.HashMap; 029import java.util.List; 030import java.util.Map; 031 032public class CanonicalTopicSubscription { 033 @JsonProperty("topic") 034 private String myTopic; 035 036 @JsonProperty("filters") 037 private List<CanonicalTopicSubscriptionFilter> myFilters; 038 039 @JsonProperty("parameters") 040 private Map<String, String> myParameters; 041 042 @JsonProperty("heartbeatPeriod") 043 private Integer myHeartbeatPeriod; 044 045 @JsonProperty("timeout") 046 private Integer myTimeout; 047 048 @JsonProperty("content") 049 private Subscription.SubscriptionPayloadContent myContent; 050 051 @JsonProperty("maxCount") 052 private Integer myMaxCount; 053 054 public String getTopic() { 055 return myTopic; 056 } 057 058 public void setTopic(String theTopic) { 059 myTopic = theTopic; 060 } 061 062 public List<CanonicalTopicSubscriptionFilter> getFilters() { 063 if (myFilters == null) { 064 myFilters = new ArrayList<>(); 065 } 066 return myFilters; 067 } 068 069 public void addFilter(CanonicalTopicSubscriptionFilter theFilter) { 070 getFilters().add(theFilter); 071 } 072 073 public void setFilters(List<CanonicalTopicSubscriptionFilter> theFilters) { 074 myFilters = theFilters; 075 } 076 077 public Map<String, String> getParameters() { 078 if (myParameters == null) { 079 myParameters = new HashMap<>(); 080 } 081 return myParameters; 082 } 083 084 public void setParameters(Map<String, String> theParameters) { 085 myParameters = theParameters; 086 } 087 088 public Integer getHeartbeatPeriod() { 089 return myHeartbeatPeriod; 090 } 091 092 public void setHeartbeatPeriod(Integer theHeartbeatPeriod) { 093 myHeartbeatPeriod = theHeartbeatPeriod; 094 } 095 096 public Integer getTimeout() { 097 return myTimeout; 098 } 099 100 public void setTimeout(Integer theTimeout) { 101 myTimeout = theTimeout; 102 } 103 104 public Integer getMaxCount() { 105 return myMaxCount; 106 } 107 108 public void setMaxCount(Integer theMaxCount) { 109 myMaxCount = theMaxCount; 110 } 111 112 public Subscription.SubscriptionPayloadContent getContent() { 113 return myContent; 114 } 115 116 public void setContent(Subscription.SubscriptionPayloadContent theContent) { 117 myContent = theContent; 118 } 119 120 @Override 121 public boolean equals(Object theO) { 122 if (this == theO) return true; 123 124 if (theO == null || getClass() != theO.getClass()) return false; 125 126 CanonicalTopicSubscription that = (CanonicalTopicSubscription) theO; 127 128 return new EqualsBuilder().append(myTopic, that.myTopic).isEquals(); 129 } 130 131 @Override 132 public int hashCode() { 133 return new HashCodeBuilder(17, 37).append(myTopic).toHashCode(); 134 } 135 136 public boolean hasFilters() { 137 return myFilters != null && !myFilters.isEmpty(); 138 } 139}