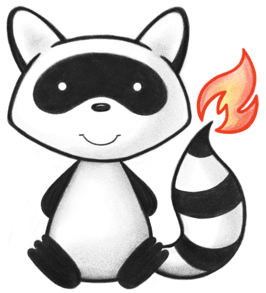
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.model; 021 022import ca.uhn.fhir.util.Logs; 023import ca.uhn.fhir.util.UrlUtil; 024import com.fasterxml.jackson.annotation.JsonProperty; 025import org.hl7.fhir.r5.model.Enumerations; 026import org.hl7.fhir.r5.model.Subscription; 027import org.slf4j.Logger; 028 029import java.util.ArrayList; 030import java.util.List; 031import java.util.Map; 032 033public class CanonicalTopicSubscriptionFilter { 034 private static final Logger ourLog = Logs.getSubscriptionTopicLog(); 035 036 @JsonProperty("resourceType") 037 String myResourceType; 038 039 @JsonProperty("filterParameter") 040 String myFilterParameter; 041 042 @JsonProperty("comparator") 043 Enumerations.SearchComparator myComparator; 044 045 @JsonProperty("modifier") 046 Enumerations.SearchModifierCode myModifier; 047 048 @JsonProperty("value") 049 String myValue; 050 051 public String getResourceType() { 052 return myResourceType; 053 } 054 055 public void setResourceType(String theResourceType) { 056 myResourceType = theResourceType; 057 } 058 059 public String getFilterParameter() { 060 return myFilterParameter; 061 } 062 063 public void setFilterParameter(String theFilterParameter) { 064 myFilterParameter = theFilterParameter; 065 } 066 067 public Enumerations.SearchComparator getComparator() { 068 return myComparator; 069 } 070 071 public void setComparator(Enumerations.SearchComparator theComparator) { 072 myComparator = theComparator; 073 } 074 075 public Enumerations.SearchModifierCode getModifier() { 076 return myModifier; 077 } 078 079 public void setModifier(Enumerations.SearchModifierCode theModifier) { 080 myModifier = theModifier; 081 } 082 083 public String getValue() { 084 return myValue; 085 } 086 087 public void setValue(String theValue) { 088 myValue = theValue; 089 } 090 091 public static List<CanonicalTopicSubscriptionFilter> fromQueryUrl(String theQueryUrl) { 092 UrlUtil.UrlParts urlParts = UrlUtil.parseUrl(theQueryUrl); 093 String resourceName = urlParts.getResourceType(); 094 095 Map<String, String[]> params = UrlUtil.parseQueryString(urlParts.getParams()); 096 List<CanonicalTopicSubscriptionFilter> retval = new ArrayList<>(); 097 params.forEach((key, valueList) -> { 098 for (String value : valueList) { 099 CanonicalTopicSubscriptionFilter filter = new CanonicalTopicSubscriptionFilter(); 100 filter.setResourceType(resourceName); 101 filter.setFilterParameter(key); 102 // WIP STR5 set modifier and comparator properly. This may be tricky without access to 103 // searchparameters, 104 // But this method cannot assume searchparameters exist on the server. 105 filter.setComparator(Enumerations.SearchComparator.EQ); 106 filter.setValue(value); 107 retval.add(filter); 108 } 109 }); 110 return retval; 111 } 112 113 public Subscription.SubscriptionFilterByComponent toSubscriptionFilterByComponent() { 114 Subscription.SubscriptionFilterByComponent retval = new Subscription.SubscriptionFilterByComponent(); 115 retval.setResourceType(myResourceType); 116 retval.setFilterParameter(myFilterParameter); 117 retval.setComparator(myComparator); 118 retval.setModifier(myModifier); 119 retval.setValue(myValue); 120 return retval; 121 } 122 123 public String asCriteriaString() { 124 String comparator = "="; 125 if (myComparator != null) { 126 switch (myComparator) { 127 case EQ: 128 comparator = "="; 129 break; 130 case NE: 131 comparator = ":not="; 132 break; 133 default: 134 ourLog.warn("Unsupported comparator: {}", myComparator); 135 } 136 } 137 return myResourceType + "?" + myFilterParameter + comparator + myValue; 138 } 139}