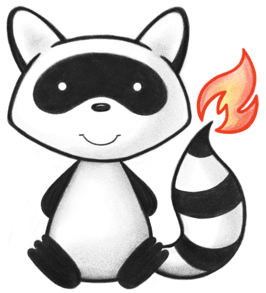
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.model; 021 022import ca.uhn.fhir.rest.server.messaging.ICanNullPayload; 023import ca.uhn.fhir.rest.server.messaging.json.BaseJsonMessage; 024import com.fasterxml.jackson.annotation.JsonProperty; 025import com.fasterxml.jackson.core.JsonProcessingException; 026import com.fasterxml.jackson.databind.ObjectMapper; 027import org.apache.commons.lang3.builder.ToStringBuilder; 028 029public class ResourceDeliveryJsonMessage extends BaseJsonMessage<ResourceDeliveryMessage> implements ICanNullPayload { 030 private static final ObjectMapper ourObjectMapper = 031 new ObjectMapper().registerModule(new com.fasterxml.jackson.datatype.jsr310.JavaTimeModule()); 032 033 @JsonProperty("payload") 034 private ResourceDeliveryMessage myPayload; 035 036 /** 037 * Constructor 038 */ 039 public ResourceDeliveryJsonMessage() { 040 super(); 041 } 042 043 /** 044 * Constructor 045 */ 046 public ResourceDeliveryJsonMessage(ResourceDeliveryMessage thePayload) { 047 myPayload = thePayload; 048 } 049 050 @Override 051 public ResourceDeliveryMessage getPayload() { 052 return myPayload; 053 } 054 055 public void setPayload(ResourceDeliveryMessage thePayload) { 056 myPayload = thePayload; 057 } 058 059 @Override 060 public String toString() { 061 return new ToStringBuilder(this).append("myPayload", myPayload).toString(); 062 } 063 064 public static ResourceDeliveryJsonMessage fromJson(String theJson) throws JsonProcessingException { 065 return ourObjectMapper.readValue(theJson, ResourceDeliveryJsonMessage.class); 066 } 067 068 public String asJson() throws JsonProcessingException { 069 return ourObjectMapper.writeValueAsString(this); 070 } 071 072 @Override 073 public void setPayloadToNull() { 074 if (myPayload != null) { 075 myPayload.setPayloadToNull(); 076 } 077 } 078}