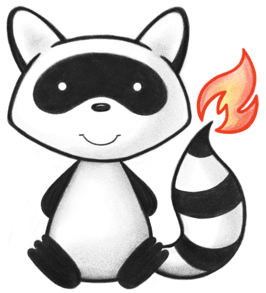
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.model; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.interceptor.model.RequestPartitionId; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import ca.uhn.fhir.rest.server.messaging.BaseResourceModifiedMessage; 026import com.fasterxml.jackson.annotation.JsonProperty; 027import org.apache.commons.lang3.builder.ToStringBuilder; 028import org.hl7.fhir.instance.model.api.IBaseResource; 029import org.hl7.fhir.instance.model.api.IIdType; 030 031import java.util.Objects; 032 033/** 034 * Most of this class has been moved to ResourceModifiedMessage in the hapi-fhir-server project, for a reusable channel ResourceModifiedMessage 035 * that doesn't require knowledge of subscriptions. 036 */ 037// TODO KHS rename classes like this to ResourceModifiedPayload so they're not confused with the actual message wrapper 038// class 039public class ResourceModifiedMessage extends BaseResourceModifiedMessage { 040 041 /** 042 * This will only be set if the resource is being triggered for a specific 043 * subscription 044 */ 045 @JsonProperty(value = "subscriptionId") 046 private String mySubscriptionId; 047 048 /** 049 * Constructor 050 */ 051 public ResourceModifiedMessage() { 052 super(); 053 } 054 055 public ResourceModifiedMessage( 056 IIdType theIdType, OperationTypeEnum theOperationType, RequestPartitionId theRequestPartitionId) { 057 super(theIdType, theOperationType); 058 setPartitionId(theRequestPartitionId); 059 } 060 061 /** 062 * @deprecated use {@link ResourceModifiedMessage#ResourceModifiedMessage(FhirContext, IBaseResource, OperationTypeEnum, RequestDetails, RequestPartitionId)} instead 063 */ 064 @Deprecated 065 public ResourceModifiedMessage( 066 FhirContext theFhirContext, IBaseResource theResource, OperationTypeEnum theOperationType) { 067 super(theFhirContext, theResource, theOperationType); 068 setPartitionId(RequestPartitionId.defaultPartition()); 069 } 070 071 public ResourceModifiedMessage( 072 FhirContext theFhirContext, 073 IBaseResource theResource, 074 OperationTypeEnum theOperationType, 075 RequestPartitionId theRequestPartitionId) { 076 super(theFhirContext, theResource, theOperationType); 077 setPartitionId(theRequestPartitionId); 078 } 079 080 public ResourceModifiedMessage( 081 FhirContext theFhirContext, 082 IBaseResource theNewResource, 083 OperationTypeEnum theOperationType, 084 RequestDetails theRequest, 085 RequestPartitionId theRequestPartitionId) { 086 super(theFhirContext, theNewResource, theOperationType, theRequest, theRequestPartitionId); 087 } 088 089 public String getSubscriptionId() { 090 return mySubscriptionId; 091 } 092 093 public void setSubscriptionId(String theSubscriptionId) { 094 mySubscriptionId = theSubscriptionId; 095 } 096 097 public void setPayloadToNull() { 098 myPayload = null; 099 } 100 101 @Override 102 public String toString() { 103 return new ToStringBuilder(this) 104 .append("operationType", myOperationType) 105 .append("subscriptionId", mySubscriptionId) 106 .append("payloadId", myPayloadId) 107 .append("partitionId", myPartitionId) 108 .toString(); 109 } 110 111 @Override 112 public boolean equals(Object theO) { 113 if (this == theO) return true; 114 if (theO == null || getClass() != theO.getClass()) return false; 115 if (!super.equals(theO)) return false; 116 ResourceModifiedMessage that = (ResourceModifiedMessage) theO; 117 return Objects.equals(getSubscriptionId(), that.getSubscriptionId()); 118 } 119 120 @Override 121 public int hashCode() { 122 return Objects.hash(super.hashCode(), getSubscriptionId()); 123 } 124}