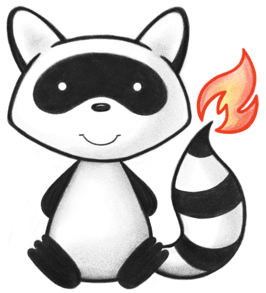
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.api; 021 022import ca.uhn.fhir.jpa.model.entity.IdAndPartitionId; 023import ca.uhn.fhir.jpa.term.models.CodeSystemConceptsDeleteResult; 024 025import java.util.Iterator; 026 027public interface ITermCodeSystemDeleteJobSvc { 028 029 /** 030 * Gets an iterator for all code system version PIDs 031 * 032 * @param thePid 033 * @return 034 */ 035 Iterator<IdAndPartitionId> getAllCodeSystemVersionForCodeSystemPid(long thePid); 036 037 /** 038 * Deletes all metadata associated with a code system version 039 * Specific metadata deleted: 040 * * concept links 041 * * concept properties 042 * * concept designations 043 * * concepts 044 * @param theVersionPid - the version id of the code system to delete 045 * @return - a wrapper for the delete results of each of the deletes (if desired) 046 */ 047 CodeSystemConceptsDeleteResult deleteCodeSystemConceptsByCodeSystemVersionPid(long theVersionPid); 048 049 /** 050 * Deletes a Code System Version 051 * NB: it is expected that any concepts related to the Code System Version are 052 * deleted first. 053 * @param theVersionPid - the code system version pid for the version to delete 054 */ 055 void deleteCodeSystemVersion(long theVersionPid); 056 057 /** 058 * Deletes a code system. 059 * NB: it is expected that all code system versions are deleted first. 060 * @param thePid - the code system pid 061 */ 062 void deleteCodeSystem(long thePid); 063 064 /** 065 * Notifies that the job has completed (or errored out). 066 * @param theJobId - the job id 067 */ 068 void notifyJobComplete(String theJobId); 069}