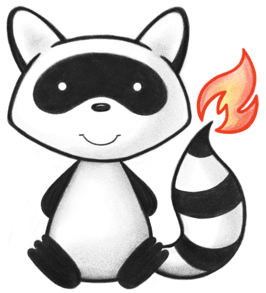
001/* 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.api; 021 022import ca.uhn.fhir.jpa.term.UploadStatistics; 023import ca.uhn.fhir.rest.api.server.RequestDetails; 024 025import java.io.ByteArrayInputStream; 026import java.io.InputStream; 027import java.util.List; 028 029/** 030 * This service handles bulk loading concepts into the terminology service concept tables 031 * using any of several predefined input formats 032 */ 033public interface ITermLoaderSvc { 034 035 String IMGTHLA_URI = "http://www.ebi.ac.uk/ipd/imgt/hla"; 036 String LOINC_URI = "http://loinc.org"; 037 String SCT_URI = "http://snomed.info/sct"; 038 String ICD10_URI = "http://hl7.org/fhir/sid/icd-10"; 039 String ICD10CM_URI = "http://hl7.org/fhir/sid/icd-10-cm"; 040 String IEEE_11073_10101_URI = "urn:iso:std:iso:11073:10101"; 041 042 UploadStatistics loadImgthla(List<FileDescriptor> theFiles, RequestDetails theRequestDetails); 043 044 UploadStatistics loadLoinc(List<FileDescriptor> theFiles, RequestDetails theRequestDetails); 045 046 UploadStatistics loadSnomedCt(List<FileDescriptor> theFiles, RequestDetails theRequestDetails); 047 048 default UploadStatistics loadIcd10(List<FileDescriptor> theFiles, RequestDetails theRequestDetails) { 049 return null; 050 } 051 052 UploadStatistics loadIcd10cm(List<FileDescriptor> theFiles, RequestDetails theRequestDetails); 053 054 UploadStatistics loadCustom(String theSystem, List<FileDescriptor> theFiles, RequestDetails theRequestDetails); 055 056 UploadStatistics loadDeltaAdd(String theSystem, List<FileDescriptor> theFiles, RequestDetails theRequestDetails); 057 058 UploadStatistics loadDeltaRemove(String theSystem, List<FileDescriptor> theFiles, RequestDetails theRequestDetails); 059 060 interface FileDescriptor { 061 062 String getFilename(); 063 064 InputStream getInputStream(); 065 } 066 067 class ByteArrayFileDescriptor implements FileDescriptor { 068 069 private final String myNextUrl; 070 private final byte[] myNextData; 071 072 public ByteArrayFileDescriptor(String theNextUrl, byte[] theNextData) { 073 myNextUrl = theNextUrl; 074 myNextData = theNextData; 075 } 076 077 @Override 078 public String getFilename() { 079 return myNextUrl; 080 } 081 082 @Override 083 public InputStream getInputStream() { 084 return new ByteArrayInputStream(myNextData); 085 } 086 } 087}