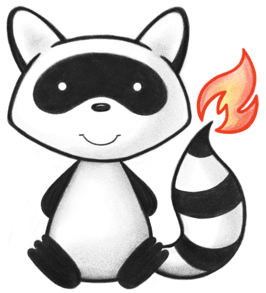
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.update; 021 022import ca.uhn.fhir.jpa.model.cross.IBasePersistedResource; 023import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import ca.uhn.fhir.rest.api.server.storage.TransactionDetails; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027import org.hl7.fhir.instance.model.api.IIdType; 028 029public class UpdateParameters<T extends IBaseResource> { 030 031 private RequestDetails myRequestDetails; 032 private IIdType myResourceIdToUpdate; 033 private String myMatchUrl; 034 private boolean myShouldPerformIndexing; 035 private boolean myShouldForceUpdateVersion; 036 private T myExistingResource; 037 private IBasePersistedResource myExistingEntity; 038 private RestOperationTypeEnum myOperationType; 039 private TransactionDetails myTransactionDetails; 040 041 /** 042 * In the update methods, we have a local variable to keep track of the old resource before performing the update 043 * This old resource may be useful for processing/performing checks (eg. enforcing Patient compartment changes with {@link ca.uhn.fhir.jpa.interceptor.PatientCompartmentEnforcingInterceptor} 044 * However, populating this can be expensive, and is skipped in Mass Ingestion mode 045 * 046 * If this is set to true, the old resource will be forcefully populated. 047 */ 048 private boolean myShouldForcePopulateOldResourceForProcessing; 049 050 public RequestDetails getRequest() { 051 return myRequestDetails; 052 } 053 054 public UpdateParameters<T> setRequestDetails(RequestDetails theRequest) { 055 this.myRequestDetails = theRequest; 056 return this; 057 } 058 059 public IIdType getResourceIdToUpdate() { 060 return myResourceIdToUpdate; 061 } 062 063 public UpdateParameters<T> setResourceIdToUpdate(IIdType theResourceId) { 064 this.myResourceIdToUpdate = theResourceId; 065 return this; 066 } 067 068 public String getMatchUrl() { 069 return myMatchUrl; 070 } 071 072 public UpdateParameters<T> setMatchUrl(String theMatchUrl) { 073 this.myMatchUrl = theMatchUrl; 074 return this; 075 } 076 077 public boolean shouldPerformIndexing() { 078 return myShouldPerformIndexing; 079 } 080 081 public UpdateParameters<T> setShouldPerformIndexing(boolean thePerformIndexing) { 082 this.myShouldPerformIndexing = thePerformIndexing; 083 return this; 084 } 085 086 public boolean shouldForceUpdateVersion() { 087 return myShouldForceUpdateVersion; 088 } 089 090 public UpdateParameters<T> setShouldForceUpdateVersion(boolean theForceUpdateVersion) { 091 this.myShouldForceUpdateVersion = theForceUpdateVersion; 092 return this; 093 } 094 095 public T getResource() { 096 return myExistingResource; 097 } 098 099 public UpdateParameters<T> setResource(T theResource) { 100 this.myExistingResource = theResource; 101 return this; 102 } 103 104 public IBasePersistedResource getEntity() { 105 return myExistingEntity; 106 } 107 108 public UpdateParameters<T> setEntity(IBasePersistedResource theEntity) { 109 this.myExistingEntity = theEntity; 110 return this; 111 } 112 113 public RestOperationTypeEnum getOperationType() { 114 return myOperationType; 115 } 116 117 public UpdateParameters<T> setOperationType(RestOperationTypeEnum myOperationType) { 118 this.myOperationType = myOperationType; 119 return this; 120 } 121 122 public TransactionDetails getTransactionDetails() { 123 return myTransactionDetails; 124 } 125 126 public UpdateParameters<T> setTransactionDetails(TransactionDetails myTransactionDetails) { 127 this.myTransactionDetails = myTransactionDetails; 128 return this; 129 } 130 131 public boolean shouldForcePopulateOldResourceForProcessing() { 132 return myShouldForcePopulateOldResourceForProcessing; 133 } 134 135 public UpdateParameters<T> setShouldForcePopulateOldResourceForProcessing( 136 boolean myShouldForcePopulateOldResourceForProcessing) { 137 this.myShouldForcePopulateOldResourceForProcessing = myShouldForcePopulateOldResourceForProcessing; 138 return this; 139 } 140}