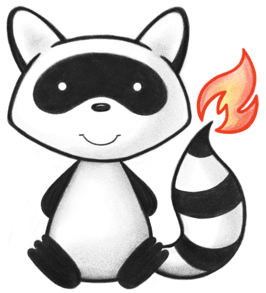
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.replacereferences; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.interceptor.model.RequestPartitionId; 024import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 025import jakarta.annotation.Nonnull; 026import org.hl7.fhir.instance.model.api.IIdType; 027 028import static ca.uhn.fhir.rest.server.provider.ProviderConstants.OPERATION_REPLACE_REFERENCES_PARAM_SOURCE_REFERENCE_ID; 029import static ca.uhn.fhir.rest.server.provider.ProviderConstants.OPERATION_REPLACE_REFERENCES_PARAM_TARGET_REFERENCE_ID; 030import static org.apache.commons.lang3.StringUtils.isBlank; 031 032public class ReplaceReferencesRequest { 033 /** 034 * Unqualified source id 035 */ 036 @Nonnull 037 public final IIdType sourceId; 038 039 /** 040 * Unqualified target id 041 */ 042 @Nonnull 043 public final IIdType targetId; 044 045 public final int resourceLimit; 046 047 public final RequestPartitionId partitionId; 048 049 public ReplaceReferencesRequest( 050 @Nonnull IIdType theSourceId, 051 @Nonnull IIdType theTargetId, 052 int theResourceLimit, 053 RequestPartitionId thePartitionId) { 054 sourceId = theSourceId.toUnqualifiedVersionless(); 055 targetId = theTargetId.toUnqualifiedVersionless(); 056 resourceLimit = theResourceLimit; 057 partitionId = thePartitionId; 058 } 059 060 public void validateOrThrowInvalidParameterException() { 061 if (isBlank(sourceId.getResourceType())) { 062 throw new InvalidRequestException( 063 Msg.code(2585) + "'" + OPERATION_REPLACE_REFERENCES_PARAM_SOURCE_REFERENCE_ID 064 + "' must be a resource type qualified id"); 065 } 066 067 if (isBlank(targetId.getResourceType())) { 068 throw new InvalidRequestException( 069 Msg.code(2586) + "'" + OPERATION_REPLACE_REFERENCES_PARAM_TARGET_REFERENCE_ID 070 + "' must be a resource type qualified id"); 071 } 072 073 if (!targetId.getResourceType().equals(sourceId.getResourceType())) { 074 throw new InvalidRequestException( 075 Msg.code(2587) + "Source and target id parameters must be for the same resource type"); 076 } 077 } 078}