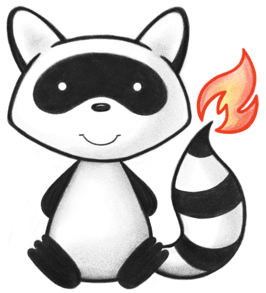
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.storage.interceptor.balp; 021 022import org.hl7.fhir.r4.model.AuditEvent; 023import org.hl7.fhir.r4.model.Coding; 024 025import java.util.function.Supplier; 026 027public enum BalpProfileEnum { 028 BASIC_CREATE( 029 "https://profiles.ihe.net/ITI/BALP/StructureDefinition/IHE.BasicAudit.Create", 030 AuditEvent.AuditEventAction.C, 031 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110153", "Source Role ID"), 032 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110152", "Destination Role ID")), 033 PATIENT_CREATE( 034 "https://profiles.ihe.net/ITI/BALP/StructureDefinition/IHE.BasicAudit.PatientCreate", 035 AuditEvent.AuditEventAction.C, 036 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110153", "Source Role ID"), 037 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110152", "Destination Role ID")), 038 039 BASIC_UPDATE( 040 "https://profiles.ihe.net/ITI/BALP/StructureDefinition/IHE.BasicAudit.Update", 041 AuditEvent.AuditEventAction.U, 042 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110153", "Source Role ID"), 043 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110152", "Destination Role ID")), 044 PATIENT_UPDATE( 045 "https://profiles.ihe.net/ITI/BALP/StructureDefinition/IHE.BasicAudit.PatientUpdate", 046 AuditEvent.AuditEventAction.U, 047 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110153", "Source Role ID"), 048 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110152", "Destination Role ID")), 049 050 BASIC_DELETE( 051 "https://profiles.ihe.net/ITI/BALP/StructureDefinition/IHE.BasicAudit.Delete", 052 AuditEvent.AuditEventAction.D, 053 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110150", "Application"), 054 () -> new Coding( 055 "http://terminology.hl7.org/CodeSystem/provenance-participant-type", "custodian", "Custodian")), 056 PATIENT_DELETE( 057 "https://profiles.ihe.net/ITI/BALP/StructureDefinition/IHE.BasicAudit.PatientDelete", 058 AuditEvent.AuditEventAction.D, 059 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110150", "Application"), 060 () -> new Coding( 061 "http://terminology.hl7.org/CodeSystem/provenance-participant-type", "custodian", "Custodian")), 062 063 BASIC_READ( 064 "https://profiles.ihe.net/ITI/BALP/StructureDefinition/IHE.BasicAudit.Read", 065 AuditEvent.AuditEventAction.R, 066 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110153", "Source Role ID"), 067 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110152", "Destination Role ID")), 068 PATIENT_READ( 069 "https://profiles.ihe.net/ITI/BALP/StructureDefinition/IHE.BasicAudit.PatientRead", 070 AuditEvent.AuditEventAction.R, 071 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110153", "Source Role ID"), 072 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110152", "Destination Role ID")), 073 074 BASIC_QUERY( 075 "https://profiles.ihe.net/ITI/BALP/StructureDefinition/IHE.BasicAudit.Query", 076 AuditEvent.AuditEventAction.E, 077 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110153", "Source Role ID"), 078 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110152", "Destination Role ID")), 079 PATIENT_QUERY( 080 "https://profiles.ihe.net/ITI/BALP/StructureDefinition/IHE.BasicAudit.PatientQuery", 081 AuditEvent.AuditEventAction.E, 082 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110153", "Source Role ID"), 083 () -> new Coding("http://dicom.nema.org/resources/ontology/DCM", "110152", "Destination Role ID")), 084 ; 085 private final String myProfileUrl; 086 private final AuditEvent.AuditEventAction myAction; 087 private final Supplier<Coding> myAgentClientTypeCoding; 088 private final Supplier<Coding> myAgentServerTypeCoding; 089 090 BalpProfileEnum( 091 String theProfileUrl, 092 AuditEvent.AuditEventAction theAction, 093 Supplier<Coding> theAgentClientTypeCoding, 094 Supplier<Coding> theAgentServerTypeCoding) { 095 myProfileUrl = theProfileUrl; 096 myAction = theAction; 097 myAgentClientTypeCoding = theAgentClientTypeCoding; 098 myAgentServerTypeCoding = theAgentServerTypeCoding; 099 } 100 101 public Coding getAgentClientTypeCoding() { 102 return myAgentClientTypeCoding.get(); 103 } 104 105 public Coding getAgentServerTypeCoding() { 106 return myAgentServerTypeCoding.get(); 107 } 108 109 public String getProfileUrl() { 110 return myProfileUrl; 111 } 112 113 public AuditEvent.AuditEventAction getAction() { 114 return myAction; 115 } 116}