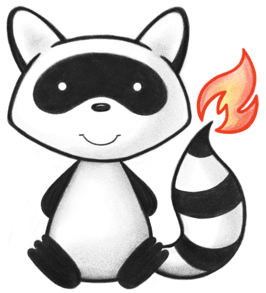
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.storage.interceptor.balp; 021 022import ca.uhn.fhir.rest.api.server.RequestDetails; 023import ca.uhn.fhir.rest.server.servlet.ServletRequestDetails; 024import jakarta.annotation.Nonnull; 025import org.hl7.fhir.instance.model.api.IBaseResource; 026import org.hl7.fhir.instance.model.api.IIdType; 027import org.hl7.fhir.r4.model.AuditEvent; 028import org.hl7.fhir.r4.model.Reference; 029 030/** 031 * This interface is intended to be implemented in order to supply implementation 032 * strategy details to the {@link BalpAuditCaptureInterceptor}. 033 * 034 * @since 6.6.0 035 */ 036public interface IBalpAuditContextServices { 037 038 /** 039 * Create and return a Reference to the client that was used to 040 * perform the action in question. 041 * 042 * @param theRequestDetails The request details object 043 */ 044 @Nonnull 045 Reference getAgentClientWho(RequestDetails theRequestDetails); 046 047 /** 048 * Create and return a Reference to the user that was used to 049 * perform the action in question. 050 * 051 * @param theRequestDetails The request details object 052 */ 053 @Nonnull 054 Reference getAgentUserWho(RequestDetails theRequestDetails); 055 056 /** 057 * Provide the requesting network address to include in the AuditEvent. 058 * 059 * @see #getNetworkAddressType(RequestDetails) If this method is returning an adress type that is not 060 * an IP address, you must also oerride this method and return the correct code. 061 */ 062 default String getNetworkAddress(RequestDetails theRequestDetails) { 063 String remoteAddr = null; 064 if (theRequestDetails instanceof ServletRequestDetails) { 065 remoteAddr = ((ServletRequestDetails) theRequestDetails) 066 .getServletRequest() 067 .getRemoteAddr(); 068 } 069 return remoteAddr; 070 } 071 072 /** 073 * Provides a code representing the appropriate return type for {@link #getNetworkAddress(RequestDetails)}. The 074 * default implementation returns {@link BalpConstants#AUDIT_EVENT_AGENT_NETWORK_TYPE_IP_ADDRESS}. 075 * 076 * @param theRequestDetails The request details object 077 * @see #getNetworkAddress(RequestDetails) 078 * @see BalpConstants#AUDIT_EVENT_AGENT_NETWORK_TYPE_MACHINE_NAME Potential return type for this method 079 * @see BalpConstants#AUDIT_EVENT_AGENT_NETWORK_TYPE_IP_ADDRESS Potential return type for this method 080 * @see BalpConstants#AUDIT_EVENT_AGENT_NETWORK_TYPE_URI Potential return type for this method 081 */ 082 default AuditEvent.AuditEventAgentNetworkType getNetworkAddressType(RequestDetails theRequestDetails) { 083 return BalpConstants.AUDIT_EVENT_AGENT_NETWORK_TYPE_IP_ADDRESS; 084 } 085 086 /** 087 * Turns an entity resource ID from an {@link IIdType} to a String. 088 * The default implementation injects the server's base URL into 089 * the ID in order to create fully qualified URLs for resource 090 * references within BALP events. 091 */ 092 @Nonnull 093 default String massageResourceIdForStorage( 094 @Nonnull RequestDetails theRequestDetails, 095 @Nonnull IBaseResource theResource, 096 @Nonnull IIdType theResourceId) { 097 String serverBaseUrl = theRequestDetails.getFhirServerBase(); 098 String resourceName = theResourceId.getResourceType(); 099 return theResourceId.withServerBase(serverBaseUrl, resourceName).getValue(); 100 } 101}