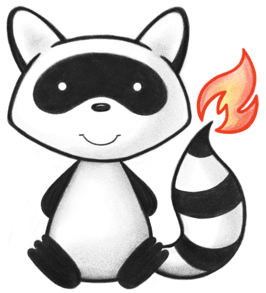
001package ca.uhn.fhir.subscription.api; 002 003/*- 004 * #%L 005 * HAPI FHIR Storage api 006 * %% 007 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 008 * %% 009 * Licensed under the Apache License, Version 2.0 (the "License"); 010 * you may not use this file except in compliance with the License. 011 * You may obtain a copy of the License at 012 * 013 * http://www.apache.org/licenses/LICENSE-2.0 014 * 015 * Unless required by applicable law or agreed to in writing, software 016 * distributed under the License is distributed on an "AS IS" BASIS, 017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 018 * See the License for the specific language governing permissions and 019 * limitations under the License. 020 * #L% 021 */ 022 023import ca.uhn.fhir.jpa.model.entity.IPersistedResourceModifiedMessage; 024import ca.uhn.fhir.jpa.model.entity.IPersistedResourceModifiedMessagePK; 025import ca.uhn.fhir.jpa.subscription.model.ResourceModifiedMessage; 026import org.springframework.data.domain.Page; 027import org.springframework.data.domain.Pageable; 028 029import java.util.Optional; 030 031/** 032 * An implementer of this interface will provide {@link ResourceModifiedMessage} persistence services. 033 * 034 * Client of this interface should persist ResourceModifiedMessage as part of the processing of an operation on 035 * a resource. Upon a successful submission to the subscription pipeline, the persisted message should be deleted. 036 * When submission fails, the message should be left un-altered for re-submission at a later time (see {@link IResourceModifiedConsumerWithRetries}). 037 */ 038public interface IResourceModifiedMessagePersistenceSvc { 039 040 /** 041 * Find all persistedResourceModifiedMessage sorted by ascending created dates (oldest to newest). 042 * @param thePageable Page request 043 * @return A sorted list of persistedResourceModifiedMessage needing submission. 044 */ 045 Page<IPersistedResourceModifiedMessage> findAllOrderedByCreatedTime(Pageable thePageable); 046 047 /** 048 * Delete a persistedResourceModifiedMessage by its primary key. 049 * 050 * @param thePersistedResourceModifiedMessagePK The primary key of the persistedResourceModifiedMessage to delete. 051 * @return Whether the persistedResourceModifiedMessage pointed to by <code>theResourceModifiedPK</code> was deleted. 052 */ 053 boolean deleteByPK(IPersistedResourceModifiedMessagePK thePersistedResourceModifiedMessagePK); 054 055 /** 056 * Persist a resourceModifiedMessage and return its resulting persisted representation. 057 * 058 * @param theMsg The resourceModifiedMessage to persist. 059 * @return The persisted representation of <code>theMsg</code>. 060 */ 061 IPersistedResourceModifiedMessage persist(ResourceModifiedMessage theMsg); 062 063 /** 064 * Restore a resourceModifiedMessage to its pre persistence representation. 065 * 066 * @param theResourceModifiedMessage The message needing restoration. 067 * @return The resourceModifiedMessage in its pre persistence form. 068 */ 069 ResourceModifiedMessage inflatePersistedResourceModifiedMessage(ResourceModifiedMessage theResourceModifiedMessage); 070 071 /** 072 * Restore a resourceModifiedMessage to its pre persistence representation or null if the resource does not exist. 073 * 074 * @param theResourceModifiedMessage 075 * @return An Optional containing The resourceModifiedMessage in its pre persistence form or null when the resource 076 * does not exist 077 */ 078 Optional<ResourceModifiedMessage> inflatePersistedResourceModifiedMessageOrNull( 079 ResourceModifiedMessage theResourceModifiedMessage); 080 081 /** 082 * Create a ResourceModifiedMessage without its pre persistence representation, i.e. without the resource body in 083 * payload 084 * 085 * @param thePersistedResourceModifiedMessage The message needing creation 086 * @return The resourceModifiedMessage without its pre persistence form 087 */ 088 ResourceModifiedMessage createResourceModifiedMessageFromEntityWithoutInflation( 089 IPersistedResourceModifiedMessage thePersistedResourceModifiedMessage); 090 091 /** 092 * 093 * @return the number of persisted resourceModifiedMessage. 094 */ 095 long getMessagePersistedCount(); 096}