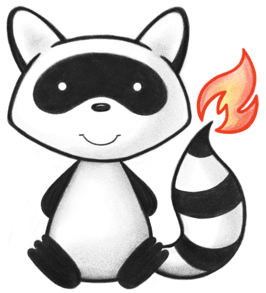
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.broker.api; 021 022import ca.uhn.fhir.rest.server.messaging.IMessage; 023 024/** 025 * IBrokerClient implementations communicate with Message Brokers to exchange messages. HAPI-FHIR uses a Message Broker for asynchronous 026 * processing tasks such as Batch Jobs, Subscription Processing, and MDM. HAPI-FHIR uses the term "Channel" to represent a Queue (JMS) 027 * or Topic (Kafka etc.) Services that send messages create a {@link IChannelProducer}. Services that 028 * receive messages from a channel create a {@link IChannelConsumer}. Each {@link IChannelConsumer} is created with a single 029 * {@link IMessageListener} that defines how messages are handled. A {@link IChannelConsumer} creates threads that are notified by the broker 030 * when new messages arrive so {@link IChannelConsumer} instances need to be properly closed when shutting down. 031 */ 032public interface IBrokerClient { 033 /** 034 * Create a consumer that receives messages from the channel. 035 * 036 * <p> 037 * Implementations can choose to return the same object for multiple invocations of this method 038 * when invoked with the same {@literal theChannelName} if they need to, or they can create a new instance. 039 * </p> 040 * 041 * @param theChannelName The actual underlying channel name 042 * @param theMessageType The object type that will be placed on this chanel. Objects will usually be a Jackson-annotated class. 043 * @param theMessageListener The message handler that will be called for each arriving message. If more than one message listeners is required for a single consumer, {@link ca.uhn.fhir.broker.impl.MultiplexingListener} can be used for the listener. 044 * @param theChannelConsumerSettings Defines the consumer configuration (e.g. number of listening threads) 045 */ 046 <T> IChannelConsumer<T> getOrCreateConsumer( 047 String theChannelName, 048 Class<? extends IMessage<T>> theMessageType, 049 IMessageListener<T> theMessageListener, 050 ChannelConsumerSettings theChannelConsumerSettings); 051 052 /** 053 * Create a producer that is used to send messages to the channel. 054 * 055 * <p> 056 * Implementations can choose to return the same object for multiple invocations of this method 057 * when invoked with the same {@literal theChannelName} if they need to, or they can create a new instance. 058 * </p> 059 * 060 * @param theChannelName The actual underlying channel name 061 * @param theMessageType The object type that will be placed on this channel. Objects will be Jackson-annotated structures. 062 * @param theChannelProducerSettings Contains the configuration for senders. 063 */ 064 <T> IChannelProducer<T> getOrCreateProducer( 065 String theChannelName, 066 Class<? extends IMessage<T>> theMessageType, 067 ChannelProducerSettings theChannelProducerSettings); 068 069 /** 070 * @return the IChannelNamer used by this broker client. 071 */ 072 IChannelNamer getChannelNamer(); 073}