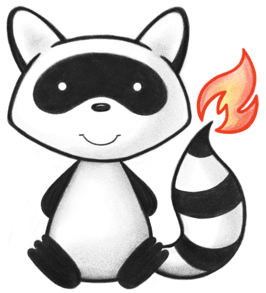
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.svc; 021 022/** 023 * Resolution mode parameter for methods on {@link IIdHelperService} 024 */ 025public class ResolveIdentityMode { 026 027 private final boolean myIncludeDeleted; 028 private final boolean myUseCache; 029 private final boolean myFailOnDeleted; 030 031 /** 032 * Non-instantiable. Use the factory methods on this class. 033 */ 034 private ResolveIdentityMode(boolean theIncludeDeleted, boolean theFailOnDeleted, boolean theUseCache) { 035 myIncludeDeleted = theIncludeDeleted; 036 myUseCache = theUseCache; 037 myFailOnDeleted = theFailOnDeleted; 038 } 039 040 public boolean isUseCache(boolean theDeleteEnabled) { 041 if (myUseCache) { 042 return true; 043 } else { 044 return !theDeleteEnabled; 045 } 046 } 047 048 public boolean isIncludeDeleted() { 049 return myIncludeDeleted; 050 } 051 052 public boolean isFailOnDeleted() { 053 return myFailOnDeleted; 054 } 055 056 /** 057 * Deleted resource identities can be included in the results 058 */ 059 public static Builder includeDeleted() { 060 return new Builder(true, false); 061 } 062 063 /** 064 * Deleted resource identities should be excluded from the results 065 */ 066 public static Builder excludeDeleted() { 067 return new Builder(false, false); 068 } 069 070 /** 071 * Throw a {@link ca.uhn.fhir.rest.server.exceptions.ResourceGoneException} if 072 * any of the supplied IDs corresponds to a deleted resource 073 */ 074 public static Builder failOnDeleted() { 075 return new Builder(false, true); 076 } 077 078 public static class Builder { 079 080 private final boolean myIncludeDeleted; 081 private final boolean myFailOnDeleted; 082 083 private Builder(boolean theIncludeDeleted, boolean theFailOnDeleted) { 084 myIncludeDeleted = theIncludeDeleted; 085 myFailOnDeleted = theFailOnDeleted; 086 } 087 088 /** 089 * Cached results are acceptable. This mode is obviously more efficient since it'll always 090 * try the cache first, but it should not be used in cases where it matters whether the 091 * deleted status has changed for a resource. 092 */ 093 public ResolveIdentityMode cacheOk() { 094 return new ResolveIdentityMode(myIncludeDeleted, myFailOnDeleted, true); 095 } 096 097 /** 098 * In this mode, the cache won't be used unless deletes are disabled on this server 099 * (meaning that the deleted status of a resource is not able to change) 100 */ 101 public ResolveIdentityMode noCacheUnlessDeletesDisabled() { 102 return new ResolveIdentityMode(myIncludeDeleted, myFailOnDeleted, false); 103 } 104 } 105}