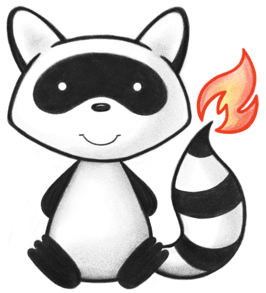
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.expunge; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.jpa.api.model.ExpungeOptions; 024import ca.uhn.fhir.jpa.api.model.ExpungeOutcome; 025import ca.uhn.fhir.rest.api.server.RequestDetails; 026import ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId; 027import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 028import org.slf4j.Logger; 029import org.slf4j.LoggerFactory; 030import org.springframework.beans.factory.annotation.Autowired; 031import org.springframework.context.ApplicationContext; 032import org.springframework.stereotype.Service; 033 034@Service 035public class ExpungeService { 036 private static final Logger ourLog = LoggerFactory.getLogger(ExpungeService.class); 037 038 @Autowired 039 private IExpungeEverythingService myExpungeEverythingService; 040 041 @Autowired 042 private IResourceExpungeService myExpungeDaoService; 043 044 @Autowired 045 private ApplicationContext myApplicationContext; 046 047 protected ExpungeOperation getExpungeOperation( 048 String theResourceName, 049 IResourcePersistentId theResourceId, 050 ExpungeOptions theExpungeOptions, 051 RequestDetails theRequestDetails) { 052 return myApplicationContext.getBean( 053 ExpungeOperation.class, theResourceName, theResourceId, theExpungeOptions, theRequestDetails); 054 } 055 056 public ExpungeOutcome expunge( 057 String theResourceName, 058 IResourcePersistentId theResourceId, 059 ExpungeOptions theExpungeOptions, 060 RequestDetails theRequest) { 061 ourLog.info( 062 "Expunge: ResourceName[{}] Id[{}] Version[{}] Options[{}]", 063 theResourceName, 064 theResourceId != null ? theResourceId.getId() : null, 065 theResourceId != null ? theResourceId.getVersion() : null, 066 theExpungeOptions); 067 ExpungeOperation expungeOperation = 068 getExpungeOperation(theResourceName, theResourceId, theExpungeOptions, theRequest); 069 070 if (theExpungeOptions.getLimit() < 1) { 071 throw new InvalidRequestException( 072 Msg.code(1087) + "Expunge limit may not be less than 1. Received expunge limit " 073 + theExpungeOptions.getLimit() + "."); 074 } 075 076 if (theResourceName == null 077 && (theResourceId == null || (theResourceId.getId() == null && theResourceId.getVersion() == null))) { 078 if (theExpungeOptions.isExpungeEverything()) { 079 myExpungeEverythingService.expungeEverything(theRequest); 080 return new ExpungeOutcome().setDeletedCount(myExpungeEverythingService.getExpungeDeletedEntityCount()); 081 } 082 } 083 084 return expungeOperation.call(); 085 } 086 087 public void deleteAllSearchParams(IResourcePersistentId theResourceId) { 088 myExpungeDaoService.deleteAllSearchParams(theResourceId); 089 } 090}