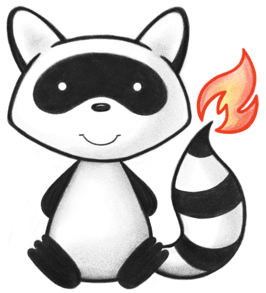
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.subscription.model; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.interceptor.model.RequestPartitionId; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import ca.uhn.fhir.rest.server.messaging.BaseResourceModifiedMessage; 026import com.fasterxml.jackson.annotation.JsonProperty; 027import org.apache.commons.lang3.builder.ToStringBuilder; 028import org.hl7.fhir.instance.model.api.IBaseResource; 029import org.hl7.fhir.instance.model.api.IIdType; 030 031import java.util.Objects; 032 033/** 034 * Most of this class has been moved to ResourceModifiedMessage in the hapi-fhir-server project, for a reusable channel ResourceModifiedMessage 035 * that doesn't require knowledge of subscriptions. 036 */ 037// TODO KHS rename classes like this to ResourceModifiedPayload so they're not confused with the actual message wrapper 038// class 039public class ResourceModifiedMessage extends BaseResourceModifiedMessage { 040 041 /** 042 * This will only be set if the resource is being triggered for a specific 043 * subscription 044 */ 045 @JsonProperty(value = "subscriptionId") 046 private String mySubscriptionId; 047 048 /** 049 * Constructor 050 */ 051 public ResourceModifiedMessage() { 052 super(); 053 } 054 055 public ResourceModifiedMessage(IIdType theIdType, OperationTypeEnum theOperationType) { 056 super(theIdType, theOperationType); 057 setPartitionId(RequestPartitionId.defaultPartition()); 058 } 059 060 public ResourceModifiedMessage( 061 FhirContext theFhirContext, IBaseResource theResource, OperationTypeEnum theOperationType) { 062 super(theFhirContext, theResource, theOperationType); 063 setPartitionId(RequestPartitionId.defaultPartition()); 064 } 065 066 public ResourceModifiedMessage( 067 FhirContext theFhirContext, 068 IBaseResource theResource, 069 OperationTypeEnum theOperationType, 070 RequestPartitionId theRequestPartitionId) { 071 super(theFhirContext, theResource, theOperationType); 072 setPartitionId(theRequestPartitionId); 073 } 074 075 public ResourceModifiedMessage( 076 FhirContext theFhirContext, 077 IBaseResource theNewResource, 078 OperationTypeEnum theOperationType, 079 RequestDetails theRequest) { 080 super(theFhirContext, theNewResource, theOperationType, theRequest); 081 setPartitionId(RequestPartitionId.defaultPartition()); 082 } 083 084 public ResourceModifiedMessage( 085 FhirContext theFhirContext, 086 IBaseResource theNewResource, 087 OperationTypeEnum theOperationType, 088 RequestDetails theRequest, 089 RequestPartitionId theRequestPartitionId) { 090 super(theFhirContext, theNewResource, theOperationType, theRequest, theRequestPartitionId); 091 } 092 093 public String getSubscriptionId() { 094 return mySubscriptionId; 095 } 096 097 public void setSubscriptionId(String theSubscriptionId) { 098 mySubscriptionId = theSubscriptionId; 099 } 100 101 public void setPayloadToNull() { 102 myPayload = null; 103 } 104 105 @Override 106 public String toString() { 107 return new ToStringBuilder(this) 108 .append("operationType", myOperationType) 109 .append("subscriptionId", mySubscriptionId) 110 .append("payloadId", myPayloadId) 111 .append("partitionId", myPartitionId) 112 .toString(); 113 } 114 115 @Override 116 public boolean equals(Object theO) { 117 if (this == theO) return true; 118 if (theO == null || getClass() != theO.getClass()) return false; 119 if (!super.equals(theO)) return false; 120 ResourceModifiedMessage that = (ResourceModifiedMessage) theO; 121 return Objects.equals(getSubscriptionId(), that.getSubscriptionId()); 122 } 123 124 @Override 125 public int hashCode() { 126 return Objects.hash(super.hashCode(), getSubscriptionId()); 127 } 128}