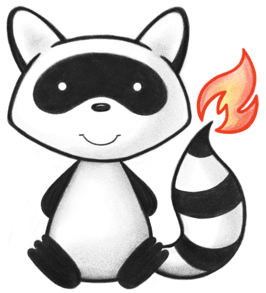
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.model.api.IElement; 024import ca.uhn.fhir.model.base.composite.BaseIdentifierDt; 025import ca.uhn.fhir.model.primitive.StringDt; 026import ca.uhn.fhir.model.primitive.UriDt; 027import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 028import org.apache.commons.lang3.builder.EqualsBuilder; 029import org.apache.commons.lang3.builder.HashCodeBuilder; 030import org.hl7.fhir.instance.model.api.IBase; 031 032import java.util.List; 033 034/** 035 * Version independent identifier 036 */ 037public class CanonicalIdentifier extends BaseIdentifierDt { 038 UriDt mySystem; 039 StringDt myValue; 040 041 @Override 042 public UriDt getSystemElement() { 043 return mySystem; 044 } 045 046 @Override 047 public StringDt getValueElement() { 048 return myValue; 049 } 050 051 @Override 052 public CanonicalIdentifier setSystem(String theUri) { 053 mySystem = new UriDt((theUri)); 054 return this; 055 } 056 057 @Override 058 public CanonicalIdentifier setValue(String theString) { 059 myValue = new StringDt(theString); 060 return this; 061 } 062 063 @Override 064 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 065 throw new UnsupportedOperationException(Msg.code(1488)); 066 } 067 068 @Override 069 public boolean isEmpty() { 070 if (mySystem != null && !mySystem.isEmpty()) { 071 return false; 072 } 073 if (myValue != null && !myValue.isEmpty()) { 074 return false; 075 } 076 return true; 077 } 078 079 @Override 080 public boolean equals(Object theO) { 081 if (this == theO) return true; 082 083 if (theO == null || getClass() != theO.getClass()) return false; 084 085 CanonicalIdentifier that = (CanonicalIdentifier) theO; 086 087 return new EqualsBuilder() 088 .append(mySystem, that.mySystem) 089 .append(myValue, that.myValue) 090 .isEquals(); 091 } 092 093 @Override 094 public int hashCode() { 095 return new HashCodeBuilder(17, 37).append(mySystem).append(myValue).toHashCode(); 096 } 097 098 public static CanonicalIdentifier fromIdentifier(IBase theIdentifier) { 099 CanonicalIdentifier retval = new CanonicalIdentifier(); 100 101 // TODO add other fields like "use" etc 102 if (theIdentifier instanceof org.hl7.fhir.dstu3.model.Identifier) { 103 org.hl7.fhir.dstu3.model.Identifier ident = (org.hl7.fhir.dstu3.model.Identifier) theIdentifier; 104 retval.setSystem(ident.getSystem()).setValue(ident.getValue()); 105 } else if (theIdentifier instanceof org.hl7.fhir.r4.model.Identifier) { 106 org.hl7.fhir.r4.model.Identifier ident = (org.hl7.fhir.r4.model.Identifier) theIdentifier; 107 retval.setSystem(ident.getSystem()).setValue(ident.getValue()); 108 } else if (theIdentifier instanceof org.hl7.fhir.r5.model.Identifier) { 109 org.hl7.fhir.r5.model.Identifier ident = (org.hl7.fhir.r5.model.Identifier) theIdentifier; 110 retval.setSystem(ident.getSystem()).setValue(ident.getValue()); 111 } else { 112 throw new InternalErrorException(Msg.code(1486) + "Expected 'Identifier' type but was '" 113 + theIdentifier.getClass().getName() + "'"); 114 } 115 return retval; 116 } 117}