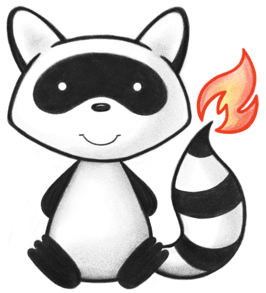
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import org.hl7.fhir.instance.model.api.IBaseCoding; 023import org.hl7.fhir.instance.model.api.IBaseMetaType; 024import org.hl7.fhir.instance.model.api.IPrimitiveType; 025 026import java.util.Comparator; 027import java.util.List; 028 029/** 030 * Contains methods to sort resource meta fields that are sets (i.e., tags, security labels and profiles) in alphabetical order. 031 * It sorts the Coding type sets (tags and security labels) based on the (system, code) pair. 032 * The system field has higher priority on sorting than the code field so the Coding set will be sorted first by system 033 * and then by code for each system. 034 */ 035public class MetaTagSorterAlphabetical implements IMetaTagSorter { 036 037 private final Comparator<String> nullFirstStringComparator = Comparator.nullsFirst(Comparator.naturalOrder()); 038 039 private final Comparator<IBaseCoding> myCodingAlphabeticalComparator = Comparator.comparing( 040 IBaseCoding::getSystem, nullFirstStringComparator) 041 .thenComparing(IBaseCoding::getCode, nullFirstStringComparator); 042 043 private final Comparator<IPrimitiveType<String>> myPrimitiveStringAlphabeticalComparator = 044 Comparator.comparing(IPrimitiveType::getValue, nullFirstStringComparator); 045 046 public void sortCodings(List<? extends IBaseCoding> theCodings) { 047 theCodings.sort(myCodingAlphabeticalComparator); 048 } 049 050 public void sortPrimitiveStrings(List<? extends IPrimitiveType<String>> theList) { 051 theList.sort(myPrimitiveStringAlphabeticalComparator); 052 } 053 054 public void sort(IBaseMetaType theMeta) { 055 sortCodings(theMeta.getTag()); 056 sortCodings(theMeta.getSecurity()); 057 sortPrimitiveStrings(theMeta.getProfile()); 058 } 059}