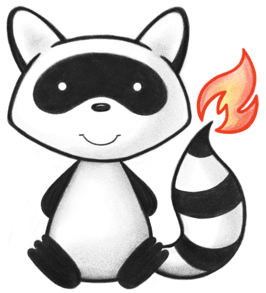
001/* 002 * #%L 003 * HAPI FHIR Structures - DSTU2 (FHIR v1.0.0) 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.dstu2; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.context.FhirVersionEnum; 025import ca.uhn.fhir.context.RuntimeResourceDefinition; 026import ca.uhn.fhir.fhirpath.IFhirPath; 027import ca.uhn.fhir.i18n.Msg; 028import ca.uhn.fhir.model.api.IFhirVersion; 029import ca.uhn.fhir.model.api.IResource; 030import ca.uhn.fhir.model.api.ResourceMetadataKeyEnum; 031import ca.uhn.fhir.model.base.composite.BaseCodingDt; 032import ca.uhn.fhir.model.base.composite.BaseContainedDt; 033import ca.uhn.fhir.model.base.composite.BaseResourceReferenceDt; 034import ca.uhn.fhir.model.dstu2.composite.CodingDt; 035import ca.uhn.fhir.model.dstu2.composite.ContainedDt; 036import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 037import ca.uhn.fhir.model.dstu2.resource.StructureDefinition; 038import ca.uhn.fhir.model.primitive.IdDt; 039import ca.uhn.fhir.rest.api.IVersionSpecificBundleFactory; 040import ca.uhn.fhir.rest.server.provider.dstu2.Dstu2BundleFactory; 041import ca.uhn.fhir.util.ReflectionUtil; 042import org.apache.commons.lang3.StringUtils; 043import org.hl7.fhir.instance.model.api.IBaseResource; 044import org.hl7.fhir.instance.model.api.IIdType; 045import org.hl7.fhir.instance.model.api.IPrimitiveType; 046 047import java.io.InputStream; 048import java.util.Date; 049 050public class FhirDstu2 implements IFhirVersion { 051 052 private String myId; 053 054 @Override 055 public IFhirPath createFhirPathExecutor(FhirContext theFhirContext) { 056 throw new UnsupportedOperationException(Msg.code(578) + "FluentPath is not supported in DSTU2 contexts"); 057 } 058 059 @Override 060 public IResource generateProfile(RuntimeResourceDefinition theRuntimeResourceDefinition, String theServerBase) { 061 StructureDefinition retVal = new StructureDefinition(); 062 063 RuntimeResourceDefinition def = theRuntimeResourceDefinition; 064 065 myId = def.getId(); 066 if (StringUtils.isBlank(myId)) { 067 myId = theRuntimeResourceDefinition.getName().toLowerCase(); 068 } 069 070 retVal.setId(new IdDt(myId)); 071 return retVal; 072 } 073 074 @Override 075 public Class<? extends BaseContainedDt> getContainedType() { 076 return ContainedDt.class; 077 } 078 079 @Override 080 public InputStream getFhirVersionPropertiesFile() { 081 InputStream str = FhirDstu2.class.getResourceAsStream("/ca/uhn/fhir/model/dstu2/fhirversion.properties"); 082 if (str == null) { 083 str = FhirDstu2.class.getResourceAsStream("ca/uhn/fhir/model/dstu2/fhirversion.properties"); 084 } 085 if (str == null) { 086 throw new ConfigurationException(Msg.code(579) + "Can not find model property file on classpath: " 087 + "/ca/uhn/fhir/model/dstu2/fhirversion.properties"); 088 } 089 return str; 090 } 091 092 @Override 093 public IPrimitiveType<Date> getLastUpdated(IBaseResource theResource) { 094 return ResourceMetadataKeyEnum.UPDATED.get((IResource) theResource); 095 } 096 097 @Override 098 public String getPathToSchemaDefinitions() { 099 return "/org/hl7/fhir/instance/model/schema"; 100 } 101 102 @Override 103 public Class<? extends BaseResourceReferenceDt> getResourceReferenceType() { 104 return ResourceReferenceDt.class; 105 } 106 107 @Override 108 public FhirVersionEnum getVersion() { 109 return FhirVersionEnum.DSTU2; 110 } 111 112 @Override 113 public IVersionSpecificBundleFactory newBundleFactory(FhirContext theContext) { 114 return new Dstu2BundleFactory(theContext); 115 } 116 117 @Override 118 public BaseCodingDt newCodingDt() { 119 return new CodingDt(); 120 } 121 122 @Override 123 public IIdType newIdType() { 124 return new IdDt(); 125 } 126 127 @Override 128 public Object getServerVersion() { 129 return ReflectionUtil.newInstanceOfFhirServerType("ca.uhn.fhir.model.dstu2.FhirServerDstu2"); 130 } 131}