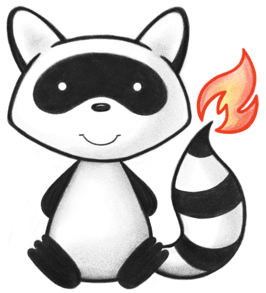
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>AddressDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * There is a variety of postal address formats defined around the world. This format defines a superset that is the basis for all addresses around the world 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * Need to be able to record postal addresses, along with notes about their use 088 * </p> 089 */ 090@DatatypeDef(name="Address") 091public class AddressDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public AddressDt() { 100 // nothing 101 } 102 103 104 @Child(name="use", type=CodeDt.class, order=0, min=0, max=1, summary=true, modifier=true) 105 @Description( 106 shortDefinition="", 107 formalDefinition="The purpose of this address" 108 ) 109 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/address-use") 110 private BoundCodeDt<AddressUseEnum> myUse; 111 112 @Child(name="type", type=CodeDt.class, order=1, min=0, max=1, summary=true, modifier=false) 113 @Description( 114 shortDefinition="", 115 formalDefinition="Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both" 116 ) 117 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/address-type") 118 private BoundCodeDt<AddressTypeEnum> myType; 119 120 @Child(name="text", type=StringDt.class, order=2, min=0, max=1, summary=true, modifier=false) 121 @Description( 122 shortDefinition="", 123 formalDefinition="A full text representation of the address" 124 ) 125 private StringDt myText; 126 127 @Child(name="line", type=StringDt.class, order=3, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 128 @Description( 129 shortDefinition="", 130 formalDefinition="This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information" 131 ) 132 private java.util.List<StringDt> myLine; 133 134 @Child(name="city", type=StringDt.class, order=4, min=0, max=1, summary=true, modifier=false) 135 @Description( 136 shortDefinition="", 137 formalDefinition="The name of the city, town, village or other community or delivery center" 138 ) 139 private StringDt myCity; 140 141 @Child(name="district", type=StringDt.class, order=5, min=0, max=1, summary=true, modifier=false) 142 @Description( 143 shortDefinition="", 144 formalDefinition="The name of the administrative area (county)" 145 ) 146 private StringDt myDistrict; 147 148 @Child(name="state", type=StringDt.class, order=6, min=0, max=1, summary=true, modifier=false) 149 @Description( 150 shortDefinition="", 151 formalDefinition="Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes)" 152 ) 153 private StringDt myState; 154 155 @Child(name="postalCode", type=StringDt.class, order=7, min=0, max=1, summary=true, modifier=false) 156 @Description( 157 shortDefinition="", 158 formalDefinition="A postal code designating a region defined by the postal service" 159 ) 160 private StringDt myPostalCode; 161 162 @Child(name="country", type=StringDt.class, order=8, min=0, max=1, summary=true, modifier=false) 163 @Description( 164 shortDefinition="", 165 formalDefinition="Country - a nation as commonly understood or generally accepted" 166 ) 167 private StringDt myCountry; 168 169 @Child(name="period", type=PeriodDt.class, order=9, min=0, max=1, summary=true, modifier=false) 170 @Description( 171 shortDefinition="", 172 formalDefinition="Time period when address was/is in use" 173 ) 174 private PeriodDt myPeriod; 175 176 177 @Override 178 public boolean isEmpty() { 179 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myUse, myType, myText, myLine, myCity, myDistrict, myState, myPostalCode, myCountry, myPeriod); 180 } 181 182 @Override 183 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 184 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myUse, myType, myText, myLine, myCity, myDistrict, myState, myPostalCode, myCountry, myPeriod); 185 } 186 187 /** 188 * Gets the value(s) for <b>use</b> (). 189 * creating it if it does 190 * not exist. Will not return <code>null</code>. 191 * 192 * <p> 193 * <b>Definition:</b> 194 * The purpose of this address 195 * </p> 196 */ 197 public BoundCodeDt<AddressUseEnum> getUseElement() { 198 if (myUse == null) { 199 myUse = new BoundCodeDt<AddressUseEnum>(AddressUseEnum.VALUESET_BINDER); 200 } 201 return myUse; 202 } 203 204 205 /** 206 * Gets the value(s) for <b>use</b> (). 207 * creating it if it does 208 * not exist. This method may return <code>null</code>. 209 * 210 * <p> 211 * <b>Definition:</b> 212 * The purpose of this address 213 * </p> 214 */ 215 public String getUse() { 216 return getUseElement().getValue(); 217 } 218 219 /** 220 * Sets the value(s) for <b>use</b> () 221 * 222 * <p> 223 * <b>Definition:</b> 224 * The purpose of this address 225 * </p> 226 */ 227 public AddressDt setUse(BoundCodeDt<AddressUseEnum> theValue) { 228 myUse = theValue; 229 return this; 230 } 231 232 233 234 /** 235 * Sets the value(s) for <b>use</b> () 236 * 237 * <p> 238 * <b>Definition:</b> 239 * The purpose of this address 240 * </p> 241 */ 242 public AddressDt setUse(AddressUseEnum theValue) { 243 setUse(new BoundCodeDt<AddressUseEnum>(AddressUseEnum.VALUESET_BINDER, theValue)); 244 245/* 246 getUseElement().setValueAsEnum(theValue); 247*/ 248 return this; 249 } 250 251 252 /** 253 * Gets the value(s) for <b>type</b> (). 254 * creating it if it does 255 * not exist. Will not return <code>null</code>. 256 * 257 * <p> 258 * <b>Definition:</b> 259 * Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both 260 * </p> 261 */ 262 public BoundCodeDt<AddressTypeEnum> getTypeElement() { 263 if (myType == null) { 264 myType = new BoundCodeDt<AddressTypeEnum>(AddressTypeEnum.VALUESET_BINDER); 265 } 266 return myType; 267 } 268 269 270 /** 271 * Gets the value(s) for <b>type</b> (). 272 * creating it if it does 273 * not exist. This method may return <code>null</code>. 274 * 275 * <p> 276 * <b>Definition:</b> 277 * Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both 278 * </p> 279 */ 280 public String getType() { 281 return getTypeElement().getValue(); 282 } 283 284 /** 285 * Sets the value(s) for <b>type</b> () 286 * 287 * <p> 288 * <b>Definition:</b> 289 * Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both 290 * </p> 291 */ 292 public AddressDt setType(BoundCodeDt<AddressTypeEnum> theValue) { 293 myType = theValue; 294 return this; 295 } 296 297 298 299 /** 300 * Sets the value(s) for <b>type</b> () 301 * 302 * <p> 303 * <b>Definition:</b> 304 * Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both 305 * </p> 306 */ 307 public AddressDt setType(AddressTypeEnum theValue) { 308 setType(new BoundCodeDt<AddressTypeEnum>(AddressTypeEnum.VALUESET_BINDER, theValue)); 309 310/* 311 getTypeElement().setValueAsEnum(theValue); 312*/ 313 return this; 314 } 315 316 317 /** 318 * Gets the value(s) for <b>text</b> (). 319 * creating it if it does 320 * not exist. Will not return <code>null</code>. 321 * 322 * <p> 323 * <b>Definition:</b> 324 * A full text representation of the address 325 * </p> 326 */ 327 public StringDt getTextElement() { 328 if (myText == null) { 329 myText = new StringDt(); 330 } 331 return myText; 332 } 333 334 335 /** 336 * Gets the value(s) for <b>text</b> (). 337 * creating it if it does 338 * not exist. This method may return <code>null</code>. 339 * 340 * <p> 341 * <b>Definition:</b> 342 * A full text representation of the address 343 * </p> 344 */ 345 public String getText() { 346 return getTextElement().getValue(); 347 } 348 349 /** 350 * Sets the value(s) for <b>text</b> () 351 * 352 * <p> 353 * <b>Definition:</b> 354 * A full text representation of the address 355 * </p> 356 */ 357 public AddressDt setText(StringDt theValue) { 358 myText = theValue; 359 return this; 360 } 361 362 363 364 /** 365 * Sets the value for <b>text</b> () 366 * 367 * <p> 368 * <b>Definition:</b> 369 * A full text representation of the address 370 * </p> 371 */ 372 public AddressDt setText( String theString) { 373 myText = new StringDt(theString); 374 return this; 375 } 376 377 378 /** 379 * Gets the value(s) for <b>line</b> (). 380 * creating it if it does 381 * not exist. Will not return <code>null</code>. 382 * 383 * <p> 384 * <b>Definition:</b> 385 * This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information 386 * </p> 387 */ 388 public java.util.List<StringDt> getLine() { 389 if (myLine == null) { 390 myLine = new java.util.ArrayList<StringDt>(); 391 } 392 return myLine; 393 } 394 395 /** 396 * Sets the value(s) for <b>line</b> () 397 * 398 * <p> 399 * <b>Definition:</b> 400 * This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information 401 * </p> 402 */ 403 public AddressDt setLine(java.util.List<StringDt> theValue) { 404 myLine = theValue; 405 return this; 406 } 407 408 409 410 /** 411 * Adds and returns a new value for <b>line</b> () 412 * 413 * <p> 414 * <b>Definition:</b> 415 * This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information 416 * </p> 417 */ 418 public StringDt addLine() { 419 StringDt newType = new StringDt(); 420 getLine().add(newType); 421 return newType; 422 } 423 424 /** 425 * Adds a given new value for <b>line</b> () 426 * 427 * <p> 428 * <b>Definition:</b> 429 * This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information 430 * </p> 431 * @param theValue The line to add (must not be <code>null</code>) 432 */ 433 public AddressDt addLine(StringDt theValue) { 434 if (theValue == null) { 435 throw new NullPointerException("theValue must not be null"); 436 } 437 getLine().add(theValue); 438 return this; 439 } 440 441 /** 442 * Gets the first repetition for <b>line</b> (), 443 * creating it if it does not already exist. 444 * 445 * <p> 446 * <b>Definition:</b> 447 * This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information 448 * </p> 449 */ 450 public StringDt getLineFirstRep() { 451 if (getLine().isEmpty()) { 452 return addLine(); 453 } 454 return getLine().get(0); 455 } 456 /** 457 * Adds a new value for <b>line</b> () 458 * 459 * <p> 460 * <b>Definition:</b> 461 * This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information 462 * </p> 463 * 464 * @return Returns a reference to this object, to allow for simple chaining. 465 */ 466 public AddressDt addLine( String theString) { 467 if (myLine == null) { 468 myLine = new java.util.ArrayList<StringDt>(); 469 } 470 myLine.add(new StringDt(theString)); 471 return this; 472 } 473 474 475 /** 476 * Gets the value(s) for <b>city</b> (). 477 * creating it if it does 478 * not exist. Will not return <code>null</code>. 479 * 480 * <p> 481 * <b>Definition:</b> 482 * The name of the city, town, village or other community or delivery center 483 * </p> 484 */ 485 public StringDt getCityElement() { 486 if (myCity == null) { 487 myCity = new StringDt(); 488 } 489 return myCity; 490 } 491 492 493 /** 494 * Gets the value(s) for <b>city</b> (). 495 * creating it if it does 496 * not exist. This method may return <code>null</code>. 497 * 498 * <p> 499 * <b>Definition:</b> 500 * The name of the city, town, village or other community or delivery center 501 * </p> 502 */ 503 public String getCity() { 504 return getCityElement().getValue(); 505 } 506 507 /** 508 * Sets the value(s) for <b>city</b> () 509 * 510 * <p> 511 * <b>Definition:</b> 512 * The name of the city, town, village or other community or delivery center 513 * </p> 514 */ 515 public AddressDt setCity(StringDt theValue) { 516 myCity = theValue; 517 return this; 518 } 519 520 521 522 /** 523 * Sets the value for <b>city</b> () 524 * 525 * <p> 526 * <b>Definition:</b> 527 * The name of the city, town, village or other community or delivery center 528 * </p> 529 */ 530 public AddressDt setCity( String theString) { 531 myCity = new StringDt(theString); 532 return this; 533 } 534 535 536 /** 537 * Gets the value(s) for <b>district</b> (). 538 * creating it if it does 539 * not exist. Will not return <code>null</code>. 540 * 541 * <p> 542 * <b>Definition:</b> 543 * The name of the administrative area (county) 544 * </p> 545 */ 546 public StringDt getDistrictElement() { 547 if (myDistrict == null) { 548 myDistrict = new StringDt(); 549 } 550 return myDistrict; 551 } 552 553 554 /** 555 * Gets the value(s) for <b>district</b> (). 556 * creating it if it does 557 * not exist. This method may return <code>null</code>. 558 * 559 * <p> 560 * <b>Definition:</b> 561 * The name of the administrative area (county) 562 * </p> 563 */ 564 public String getDistrict() { 565 return getDistrictElement().getValue(); 566 } 567 568 /** 569 * Sets the value(s) for <b>district</b> () 570 * 571 * <p> 572 * <b>Definition:</b> 573 * The name of the administrative area (county) 574 * </p> 575 */ 576 public AddressDt setDistrict(StringDt theValue) { 577 myDistrict = theValue; 578 return this; 579 } 580 581 582 583 /** 584 * Sets the value for <b>district</b> () 585 * 586 * <p> 587 * <b>Definition:</b> 588 * The name of the administrative area (county) 589 * </p> 590 */ 591 public AddressDt setDistrict( String theString) { 592 myDistrict = new StringDt(theString); 593 return this; 594 } 595 596 597 /** 598 * Gets the value(s) for <b>state</b> (). 599 * creating it if it does 600 * not exist. Will not return <code>null</code>. 601 * 602 * <p> 603 * <b>Definition:</b> 604 * Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes) 605 * </p> 606 */ 607 public StringDt getStateElement() { 608 if (myState == null) { 609 myState = new StringDt(); 610 } 611 return myState; 612 } 613 614 615 /** 616 * Gets the value(s) for <b>state</b> (). 617 * creating it if it does 618 * not exist. This method may return <code>null</code>. 619 * 620 * <p> 621 * <b>Definition:</b> 622 * Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes) 623 * </p> 624 */ 625 public String getState() { 626 return getStateElement().getValue(); 627 } 628 629 /** 630 * Sets the value(s) for <b>state</b> () 631 * 632 * <p> 633 * <b>Definition:</b> 634 * Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes) 635 * </p> 636 */ 637 public AddressDt setState(StringDt theValue) { 638 myState = theValue; 639 return this; 640 } 641 642 643 644 /** 645 * Sets the value for <b>state</b> () 646 * 647 * <p> 648 * <b>Definition:</b> 649 * Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes) 650 * </p> 651 */ 652 public AddressDt setState( String theString) { 653 myState = new StringDt(theString); 654 return this; 655 } 656 657 658 /** 659 * Gets the value(s) for <b>postalCode</b> (). 660 * creating it if it does 661 * not exist. Will not return <code>null</code>. 662 * 663 * <p> 664 * <b>Definition:</b> 665 * A postal code designating a region defined by the postal service 666 * </p> 667 */ 668 public StringDt getPostalCodeElement() { 669 if (myPostalCode == null) { 670 myPostalCode = new StringDt(); 671 } 672 return myPostalCode; 673 } 674 675 676 /** 677 * Gets the value(s) for <b>postalCode</b> (). 678 * creating it if it does 679 * not exist. This method may return <code>null</code>. 680 * 681 * <p> 682 * <b>Definition:</b> 683 * A postal code designating a region defined by the postal service 684 * </p> 685 */ 686 public String getPostalCode() { 687 return getPostalCodeElement().getValue(); 688 } 689 690 /** 691 * Sets the value(s) for <b>postalCode</b> () 692 * 693 * <p> 694 * <b>Definition:</b> 695 * A postal code designating a region defined by the postal service 696 * </p> 697 */ 698 public AddressDt setPostalCode(StringDt theValue) { 699 myPostalCode = theValue; 700 return this; 701 } 702 703 704 705 /** 706 * Sets the value for <b>postalCode</b> () 707 * 708 * <p> 709 * <b>Definition:</b> 710 * A postal code designating a region defined by the postal service 711 * </p> 712 */ 713 public AddressDt setPostalCode( String theString) { 714 myPostalCode = new StringDt(theString); 715 return this; 716 } 717 718 719 /** 720 * Gets the value(s) for <b>country</b> (). 721 * creating it if it does 722 * not exist. Will not return <code>null</code>. 723 * 724 * <p> 725 * <b>Definition:</b> 726 * Country - a nation as commonly understood or generally accepted 727 * </p> 728 */ 729 public StringDt getCountryElement() { 730 if (myCountry == null) { 731 myCountry = new StringDt(); 732 } 733 return myCountry; 734 } 735 736 737 /** 738 * Gets the value(s) for <b>country</b> (). 739 * creating it if it does 740 * not exist. This method may return <code>null</code>. 741 * 742 * <p> 743 * <b>Definition:</b> 744 * Country - a nation as commonly understood or generally accepted 745 * </p> 746 */ 747 public String getCountry() { 748 return getCountryElement().getValue(); 749 } 750 751 /** 752 * Sets the value(s) for <b>country</b> () 753 * 754 * <p> 755 * <b>Definition:</b> 756 * Country - a nation as commonly understood or generally accepted 757 * </p> 758 */ 759 public AddressDt setCountry(StringDt theValue) { 760 myCountry = theValue; 761 return this; 762 } 763 764 765 766 /** 767 * Sets the value for <b>country</b> () 768 * 769 * <p> 770 * <b>Definition:</b> 771 * Country - a nation as commonly understood or generally accepted 772 * </p> 773 */ 774 public AddressDt setCountry( String theString) { 775 myCountry = new StringDt(theString); 776 return this; 777 } 778 779 780 /** 781 * Gets the value(s) for <b>period</b> (). 782 * creating it if it does 783 * not exist. Will not return <code>null</code>. 784 * 785 * <p> 786 * <b>Definition:</b> 787 * Time period when address was/is in use 788 * </p> 789 */ 790 public PeriodDt getPeriod() { 791 if (myPeriod == null) { 792 myPeriod = new PeriodDt(); 793 } 794 return myPeriod; 795 } 796 797 /** 798 * Sets the value(s) for <b>period</b> () 799 * 800 * <p> 801 * <b>Definition:</b> 802 * Time period when address was/is in use 803 * </p> 804 */ 805 public AddressDt setPeriod(PeriodDt theValue) { 806 myPeriod = theValue; 807 return this; 808 } 809 810 811 812 813 814 815}