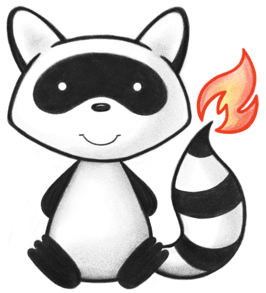
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>AnnotationDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * A text note which also contains information about who made the statement and when 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * 088 * </p> 089 */ 090@DatatypeDef(name="Annotation") 091public class AnnotationDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public AnnotationDt() { 100 // nothing 101 } 102 103 104 @Child(name="author", order=0, min=0, max=1, summary=true, modifier=false, type={ 105 Practitioner.class, 106 Patient.class, 107 RelatedPerson.class, 108 StringDt.class 109 }) 110 @Description( 111 shortDefinition="", 112 formalDefinition="The individual responsible for making the annotation." 113 ) 114 private IDatatype myAuthor; 115 116 @Child(name="time", type=DateTimeDt.class, order=1, min=0, max=1, summary=true, modifier=false) 117 @Description( 118 shortDefinition="", 119 formalDefinition="Indicates when this particular annotation was made" 120 ) 121 private DateTimeDt myTime; 122 123 @Child(name="text", type=StringDt.class, order=2, min=1, max=1, summary=true, modifier=false) 124 @Description( 125 shortDefinition="", 126 formalDefinition="The text of the annotation" 127 ) 128 private StringDt myText; 129 130 131 @Override 132 public boolean isEmpty() { 133 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myAuthor, myTime, myText); 134 } 135 136 @Override 137 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 138 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myAuthor, myTime, myText); 139 } 140 141 /** 142 * Gets the value(s) for <b>author[x]</b> (). 143 * creating it if it does 144 * not exist. Will not return <code>null</code>. 145 * 146 * <p> 147 * <b>Definition:</b> 148 * The individual responsible for making the annotation. 149 * </p> 150 */ 151 public IDatatype getAuthor() { 152 return myAuthor; 153 } 154 155 /** 156 * Sets the value(s) for <b>author[x]</b> () 157 * 158 * <p> 159 * <b>Definition:</b> 160 * The individual responsible for making the annotation. 161 * </p> 162 */ 163 public AnnotationDt setAuthor(IDatatype theValue) { 164 myAuthor = theValue; 165 return this; 166 } 167 168 169 170 171 /** 172 * Gets the value(s) for <b>time</b> (). 173 * creating it if it does 174 * not exist. Will not return <code>null</code>. 175 * 176 * <p> 177 * <b>Definition:</b> 178 * Indicates when this particular annotation was made 179 * </p> 180 */ 181 public DateTimeDt getTimeElement() { 182 if (myTime == null) { 183 myTime = new DateTimeDt(); 184 } 185 return myTime; 186 } 187 188 189 /** 190 * Gets the value(s) for <b>time</b> (). 191 * creating it if it does 192 * not exist. This method may return <code>null</code>. 193 * 194 * <p> 195 * <b>Definition:</b> 196 * Indicates when this particular annotation was made 197 * </p> 198 */ 199 public Date getTime() { 200 return getTimeElement().getValue(); 201 } 202 203 /** 204 * Sets the value(s) for <b>time</b> () 205 * 206 * <p> 207 * <b>Definition:</b> 208 * Indicates when this particular annotation was made 209 * </p> 210 */ 211 public AnnotationDt setTime(DateTimeDt theValue) { 212 myTime = theValue; 213 return this; 214 } 215 216 217 218 /** 219 * Sets the value for <b>time</b> () 220 * 221 * <p> 222 * <b>Definition:</b> 223 * Indicates when this particular annotation was made 224 * </p> 225 */ 226 public AnnotationDt setTimeWithSecondsPrecision( Date theDate) { 227 myTime = new DateTimeDt(theDate); 228 return this; 229 } 230 231 /** 232 * Sets the value for <b>time</b> () 233 * 234 * <p> 235 * <b>Definition:</b> 236 * Indicates when this particular annotation was made 237 * </p> 238 */ 239 public AnnotationDt setTime( Date theDate, TemporalPrecisionEnum thePrecision) { 240 myTime = new DateTimeDt(theDate, thePrecision); 241 return this; 242 } 243 244 245 /** 246 * Gets the value(s) for <b>text</b> (). 247 * creating it if it does 248 * not exist. Will not return <code>null</code>. 249 * 250 * <p> 251 * <b>Definition:</b> 252 * The text of the annotation 253 * </p> 254 */ 255 public StringDt getTextElement() { 256 if (myText == null) { 257 myText = new StringDt(); 258 } 259 return myText; 260 } 261 262 263 /** 264 * Gets the value(s) for <b>text</b> (). 265 * creating it if it does 266 * not exist. This method may return <code>null</code>. 267 * 268 * <p> 269 * <b>Definition:</b> 270 * The text of the annotation 271 * </p> 272 */ 273 public String getText() { 274 return getTextElement().getValue(); 275 } 276 277 /** 278 * Sets the value(s) for <b>text</b> () 279 * 280 * <p> 281 * <b>Definition:</b> 282 * The text of the annotation 283 * </p> 284 */ 285 public AnnotationDt setText(StringDt theValue) { 286 myText = theValue; 287 return this; 288 } 289 290 291 292 /** 293 * Sets the value for <b>text</b> () 294 * 295 * <p> 296 * <b>Definition:</b> 297 * The text of the annotation 298 * </p> 299 */ 300 public AnnotationDt setText( String theString) { 301 myText = new StringDt(theString); 302 return this; 303 } 304 305 306 307 308}