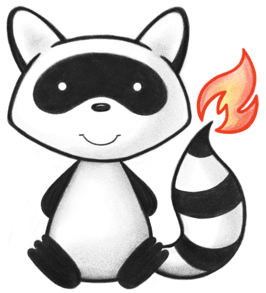
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>AttachmentDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * For referring to data content defined in other formats. 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * Many models need to include data defined in other specifications that is complex and opaque to the healthcare model. This includes documents, media recordings, structured data, etc. 088 * </p> 089 */ 090@DatatypeDef(name="Attachment") 091public class AttachmentDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public AttachmentDt() { 100 // nothing 101 } 102 103 104 @Child(name="contentType", type=CodeDt.class, order=0, min=0, max=1, summary=true, modifier=false) 105 @Description( 106 shortDefinition="", 107 formalDefinition="Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate" 108 ) 109 private CodeDt myContentType; 110 111 @Child(name="language", type=CodeDt.class, order=1, min=0, max=1, summary=true, modifier=false) 112 @Description( 113 shortDefinition="", 114 formalDefinition="The human language of the content. The value can be any valid value according to BCP 47" 115 ) 116 private CodeDt myLanguage; 117 118 @Child(name="data", type=Base64BinaryDt.class, order=2, min=0, max=1, summary=true, modifier=false) 119 @Description( 120 shortDefinition="", 121 formalDefinition="The actual data of the attachment - a sequence of bytes. In XML, represented using base64" 122 ) 123 private Base64BinaryDt myData; 124 125 @Child(name="url", type=UriDt.class, order=3, min=0, max=1, summary=true, modifier=false) 126 @Description( 127 shortDefinition="", 128 formalDefinition="An alternative location where the data can be accessed" 129 ) 130 private UriDt myUrl; 131 132 @Child(name="size", type=UnsignedIntDt.class, order=4, min=0, max=1, summary=true, modifier=false) 133 @Description( 134 shortDefinition="", 135 formalDefinition="The number of bytes of data that make up this attachment." 136 ) 137 private UnsignedIntDt mySize; 138 139 @Child(name="hash", type=Base64BinaryDt.class, order=5, min=0, max=1, summary=true, modifier=false) 140 @Description( 141 shortDefinition="", 142 formalDefinition="The calculated hash of the data using SHA-1. Represented using base64" 143 ) 144 private Base64BinaryDt myHash; 145 146 @Child(name="title", type=StringDt.class, order=6, min=0, max=1, summary=true, modifier=false) 147 @Description( 148 shortDefinition="", 149 formalDefinition="A label or set of text to display in place of the data" 150 ) 151 private StringDt myTitle; 152 153 @Child(name="creation", type=DateTimeDt.class, order=7, min=0, max=1, summary=true, modifier=false) 154 @Description( 155 shortDefinition="", 156 formalDefinition="The date that the attachment was first created" 157 ) 158 private DateTimeDt myCreation; 159 160 161 @Override 162 public boolean isEmpty() { 163 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myContentType, myLanguage, myData, myUrl, mySize, myHash, myTitle, myCreation); 164 } 165 166 @Override 167 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 168 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myContentType, myLanguage, myData, myUrl, mySize, myHash, myTitle, myCreation); 169 } 170 171 /** 172 * Gets the value(s) for <b>contentType</b> (). 173 * creating it if it does 174 * not exist. Will not return <code>null</code>. 175 * 176 * <p> 177 * <b>Definition:</b> 178 * Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate 179 * </p> 180 */ 181 public CodeDt getContentTypeElement() { 182 if (myContentType == null) { 183 myContentType = new CodeDt(); 184 } 185 return myContentType; 186 } 187 188 189 /** 190 * Gets the value(s) for <b>contentType</b> (). 191 * creating it if it does 192 * not exist. This method may return <code>null</code>. 193 * 194 * <p> 195 * <b>Definition:</b> 196 * Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate 197 * </p> 198 */ 199 public String getContentType() { 200 return getContentTypeElement().getValue(); 201 } 202 203 /** 204 * Sets the value(s) for <b>contentType</b> () 205 * 206 * <p> 207 * <b>Definition:</b> 208 * Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate 209 * </p> 210 */ 211 public AttachmentDt setContentType(CodeDt theValue) { 212 myContentType = theValue; 213 return this; 214 } 215 216 217 218 /** 219 * Sets the value for <b>contentType</b> () 220 * 221 * <p> 222 * <b>Definition:</b> 223 * Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate 224 * </p> 225 */ 226 public AttachmentDt setContentType( String theCode) { 227 myContentType = new CodeDt(theCode); 228 return this; 229 } 230 231 232 /** 233 * Gets the value(s) for <b>language</b> (). 234 * creating it if it does 235 * not exist. Will not return <code>null</code>. 236 * 237 * <p> 238 * <b>Definition:</b> 239 * The human language of the content. The value can be any valid value according to BCP 47 240 * </p> 241 */ 242 public CodeDt getLanguageElement() { 243 if (myLanguage == null) { 244 myLanguage = new CodeDt(); 245 } 246 return myLanguage; 247 } 248 249 250 /** 251 * Gets the value(s) for <b>language</b> (). 252 * creating it if it does 253 * not exist. This method may return <code>null</code>. 254 * 255 * <p> 256 * <b>Definition:</b> 257 * The human language of the content. The value can be any valid value according to BCP 47 258 * </p> 259 */ 260 public String getLanguage() { 261 return getLanguageElement().getValue(); 262 } 263 264 /** 265 * Sets the value(s) for <b>language</b> () 266 * 267 * <p> 268 * <b>Definition:</b> 269 * The human language of the content. The value can be any valid value according to BCP 47 270 * </p> 271 */ 272 public AttachmentDt setLanguage(CodeDt theValue) { 273 myLanguage = theValue; 274 return this; 275 } 276 277 278 279 /** 280 * Sets the value for <b>language</b> () 281 * 282 * <p> 283 * <b>Definition:</b> 284 * The human language of the content. The value can be any valid value according to BCP 47 285 * </p> 286 */ 287 public AttachmentDt setLanguage( String theCode) { 288 myLanguage = new CodeDt(theCode); 289 return this; 290 } 291 292 293 /** 294 * Gets the value(s) for <b>data</b> (). 295 * creating it if it does 296 * not exist. Will not return <code>null</code>. 297 * 298 * <p> 299 * <b>Definition:</b> 300 * The actual data of the attachment - a sequence of bytes. In XML, represented using base64 301 * </p> 302 */ 303 public Base64BinaryDt getDataElement() { 304 if (myData == null) { 305 myData = new Base64BinaryDt(); 306 } 307 return myData; 308 } 309 310 311 /** 312 * Gets the value(s) for <b>data</b> (). 313 * creating it if it does 314 * not exist. This method may return <code>null</code>. 315 * 316 * <p> 317 * <b>Definition:</b> 318 * The actual data of the attachment - a sequence of bytes. In XML, represented using base64 319 * </p> 320 */ 321 public byte[] getData() { 322 return getDataElement().getValue(); 323 } 324 325 /** 326 * Sets the value(s) for <b>data</b> () 327 * 328 * <p> 329 * <b>Definition:</b> 330 * The actual data of the attachment - a sequence of bytes. In XML, represented using base64 331 * </p> 332 */ 333 public AttachmentDt setData(Base64BinaryDt theValue) { 334 myData = theValue; 335 return this; 336 } 337 338 339 340 /** 341 * Sets the value for <b>data</b> () 342 * 343 * <p> 344 * <b>Definition:</b> 345 * The actual data of the attachment - a sequence of bytes. In XML, represented using base64 346 * </p> 347 */ 348 public AttachmentDt setData( byte[] theBytes) { 349 myData = new Base64BinaryDt(theBytes); 350 return this; 351 } 352 353 354 /** 355 * Gets the value(s) for <b>url</b> (). 356 * creating it if it does 357 * not exist. Will not return <code>null</code>. 358 * 359 * <p> 360 * <b>Definition:</b> 361 * An alternative location where the data can be accessed 362 * </p> 363 */ 364 public UriDt getUrlElement() { 365 if (myUrl == null) { 366 myUrl = new UriDt(); 367 } 368 return myUrl; 369 } 370 371 372 /** 373 * Gets the value(s) for <b>url</b> (). 374 * creating it if it does 375 * not exist. This method may return <code>null</code>. 376 * 377 * <p> 378 * <b>Definition:</b> 379 * An alternative location where the data can be accessed 380 * </p> 381 */ 382 public String getUrl() { 383 return getUrlElement().getValue(); 384 } 385 386 /** 387 * Sets the value(s) for <b>url</b> () 388 * 389 * <p> 390 * <b>Definition:</b> 391 * An alternative location where the data can be accessed 392 * </p> 393 */ 394 public AttachmentDt setUrl(UriDt theValue) { 395 myUrl = theValue; 396 return this; 397 } 398 399 400 401 /** 402 * Sets the value for <b>url</b> () 403 * 404 * <p> 405 * <b>Definition:</b> 406 * An alternative location where the data can be accessed 407 * </p> 408 */ 409 public AttachmentDt setUrl( String theUri) { 410 myUrl = new UriDt(theUri); 411 return this; 412 } 413 414 415 /** 416 * Gets the value(s) for <b>size</b> (). 417 * creating it if it does 418 * not exist. Will not return <code>null</code>. 419 * 420 * <p> 421 * <b>Definition:</b> 422 * The number of bytes of data that make up this attachment. 423 * </p> 424 */ 425 public UnsignedIntDt getSizeElement() { 426 if (mySize == null) { 427 mySize = new UnsignedIntDt(); 428 } 429 return mySize; 430 } 431 432 433 /** 434 * Gets the value(s) for <b>size</b> (). 435 * creating it if it does 436 * not exist. This method may return <code>null</code>. 437 * 438 * <p> 439 * <b>Definition:</b> 440 * The number of bytes of data that make up this attachment. 441 * </p> 442 */ 443 public Integer getSize() { 444 return getSizeElement().getValue(); 445 } 446 447 /** 448 * Sets the value(s) for <b>size</b> () 449 * 450 * <p> 451 * <b>Definition:</b> 452 * The number of bytes of data that make up this attachment. 453 * </p> 454 */ 455 public AttachmentDt setSize(UnsignedIntDt theValue) { 456 mySize = theValue; 457 return this; 458 } 459 460 461 462 /** 463 * Sets the value for <b>size</b> () 464 * 465 * <p> 466 * <b>Definition:</b> 467 * The number of bytes of data that make up this attachment. 468 * </p> 469 */ 470 public AttachmentDt setSize( int theInteger) { 471 mySize = new UnsignedIntDt(theInteger); 472 return this; 473 } 474 475 476 /** 477 * Gets the value(s) for <b>hash</b> (). 478 * creating it if it does 479 * not exist. Will not return <code>null</code>. 480 * 481 * <p> 482 * <b>Definition:</b> 483 * The calculated hash of the data using SHA-1. Represented using base64 484 * </p> 485 */ 486 public Base64BinaryDt getHashElement() { 487 if (myHash == null) { 488 myHash = new Base64BinaryDt(); 489 } 490 return myHash; 491 } 492 493 494 /** 495 * Gets the value(s) for <b>hash</b> (). 496 * creating it if it does 497 * not exist. This method may return <code>null</code>. 498 * 499 * <p> 500 * <b>Definition:</b> 501 * The calculated hash of the data using SHA-1. Represented using base64 502 * </p> 503 */ 504 public byte[] getHash() { 505 return getHashElement().getValue(); 506 } 507 508 /** 509 * Sets the value(s) for <b>hash</b> () 510 * 511 * <p> 512 * <b>Definition:</b> 513 * The calculated hash of the data using SHA-1. Represented using base64 514 * </p> 515 */ 516 public AttachmentDt setHash(Base64BinaryDt theValue) { 517 myHash = theValue; 518 return this; 519 } 520 521 522 523 /** 524 * Sets the value for <b>hash</b> () 525 * 526 * <p> 527 * <b>Definition:</b> 528 * The calculated hash of the data using SHA-1. Represented using base64 529 * </p> 530 */ 531 public AttachmentDt setHash( byte[] theBytes) { 532 myHash = new Base64BinaryDt(theBytes); 533 return this; 534 } 535 536 537 /** 538 * Gets the value(s) for <b>title</b> (). 539 * creating it if it does 540 * not exist. Will not return <code>null</code>. 541 * 542 * <p> 543 * <b>Definition:</b> 544 * A label or set of text to display in place of the data 545 * </p> 546 */ 547 public StringDt getTitleElement() { 548 if (myTitle == null) { 549 myTitle = new StringDt(); 550 } 551 return myTitle; 552 } 553 554 555 /** 556 * Gets the value(s) for <b>title</b> (). 557 * creating it if it does 558 * not exist. This method may return <code>null</code>. 559 * 560 * <p> 561 * <b>Definition:</b> 562 * A label or set of text to display in place of the data 563 * </p> 564 */ 565 public String getTitle() { 566 return getTitleElement().getValue(); 567 } 568 569 /** 570 * Sets the value(s) for <b>title</b> () 571 * 572 * <p> 573 * <b>Definition:</b> 574 * A label or set of text to display in place of the data 575 * </p> 576 */ 577 public AttachmentDt setTitle(StringDt theValue) { 578 myTitle = theValue; 579 return this; 580 } 581 582 583 584 /** 585 * Sets the value for <b>title</b> () 586 * 587 * <p> 588 * <b>Definition:</b> 589 * A label or set of text to display in place of the data 590 * </p> 591 */ 592 public AttachmentDt setTitle( String theString) { 593 myTitle = new StringDt(theString); 594 return this; 595 } 596 597 598 /** 599 * Gets the value(s) for <b>creation</b> (). 600 * creating it if it does 601 * not exist. Will not return <code>null</code>. 602 * 603 * <p> 604 * <b>Definition:</b> 605 * The date that the attachment was first created 606 * </p> 607 */ 608 public DateTimeDt getCreationElement() { 609 if (myCreation == null) { 610 myCreation = new DateTimeDt(); 611 } 612 return myCreation; 613 } 614 615 616 /** 617 * Gets the value(s) for <b>creation</b> (). 618 * creating it if it does 619 * not exist. This method may return <code>null</code>. 620 * 621 * <p> 622 * <b>Definition:</b> 623 * The date that the attachment was first created 624 * </p> 625 */ 626 public Date getCreation() { 627 return getCreationElement().getValue(); 628 } 629 630 /** 631 * Sets the value(s) for <b>creation</b> () 632 * 633 * <p> 634 * <b>Definition:</b> 635 * The date that the attachment was first created 636 * </p> 637 */ 638 public AttachmentDt setCreation(DateTimeDt theValue) { 639 myCreation = theValue; 640 return this; 641 } 642 643 644 645 /** 646 * Sets the value for <b>creation</b> () 647 * 648 * <p> 649 * <b>Definition:</b> 650 * The date that the attachment was first created 651 * </p> 652 */ 653 public AttachmentDt setCreationWithSecondsPrecision( Date theDate) { 654 myCreation = new DateTimeDt(theDate); 655 return this; 656 } 657 658 /** 659 * Sets the value for <b>creation</b> () 660 * 661 * <p> 662 * <b>Definition:</b> 663 * The date that the attachment was first created 664 * </p> 665 */ 666 public AttachmentDt setCreation( Date theDate, TemporalPrecisionEnum thePrecision) { 667 myCreation = new DateTimeDt(theDate, thePrecision); 668 return this; 669 } 670 671 672 673 674}