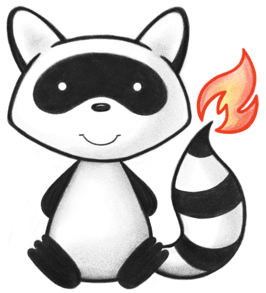
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>CodeableConceptDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * This is a common pattern in healthcare - a concept that may be defined by one or more codes from formal definitions including LOINC and SNOMED CT, and/or defined by the provision of text that captures a human sense of the concept 088 * </p> 089 */ 090@DatatypeDef(name="CodeableConcept") 091public class CodeableConceptDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public CodeableConceptDt() { 100 // nothing 101 } 102 103 /** 104 * Constructor which creates a CodeableConceptDt with one coding repetition, containing 105 * the given system and code 106 */ 107 public CodeableConceptDt(String theSystem, String theCode) { 108 addCoding().setSystem(theSystem).setCode(theCode); 109 } 110 111 @Child(name="coding", type=CodingDt.class, order=0, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 112 @Description( 113 shortDefinition="", 114 formalDefinition="A reference to a code defined by a terminology system" 115 ) 116 private java.util.List<CodingDt> myCoding; 117 118 @Child(name="text", type=StringDt.class, order=1, min=0, max=1, summary=true, modifier=false) 119 @Description( 120 shortDefinition="", 121 formalDefinition="A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user" 122 ) 123 private StringDt myText; 124 125 126 @Override 127 public boolean isEmpty() { 128 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myCoding, myText); 129 } 130 131 @Override 132 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 133 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myCoding, myText); 134 } 135 136 /** 137 * Gets the value(s) for <b>coding</b> (). 138 * creating it if it does 139 * not exist. Will not return <code>null</code>. 140 * 141 * <p> 142 * <b>Definition:</b> 143 * A reference to a code defined by a terminology system 144 * </p> 145 */ 146 public java.util.List<CodingDt> getCoding() { 147 if (myCoding == null) { 148 myCoding = new java.util.ArrayList<CodingDt>(); 149 } 150 return myCoding; 151 } 152 153 /** 154 * Sets the value(s) for <b>coding</b> () 155 * 156 * <p> 157 * <b>Definition:</b> 158 * A reference to a code defined by a terminology system 159 * </p> 160 */ 161 public CodeableConceptDt setCoding(java.util.List<CodingDt> theValue) { 162 myCoding = theValue; 163 return this; 164 } 165 166 167 168 /** 169 * Adds and returns a new value for <b>coding</b> () 170 * 171 * <p> 172 * <b>Definition:</b> 173 * A reference to a code defined by a terminology system 174 * </p> 175 */ 176 public CodingDt addCoding() { 177 CodingDt newType = new CodingDt(); 178 getCoding().add(newType); 179 return newType; 180 } 181 182 /** 183 * Adds a given new value for <b>coding</b> () 184 * 185 * <p> 186 * <b>Definition:</b> 187 * A reference to a code defined by a terminology system 188 * </p> 189 * @param theValue The coding to add (must not be <code>null</code>) 190 */ 191 public CodeableConceptDt addCoding(CodingDt theValue) { 192 if (theValue == null) { 193 throw new NullPointerException("theValue must not be null"); 194 } 195 getCoding().add(theValue); 196 return this; 197 } 198 199 /** 200 * Gets the first repetition for <b>coding</b> (), 201 * creating it if it does not already exist. 202 * 203 * <p> 204 * <b>Definition:</b> 205 * A reference to a code defined by a terminology system 206 * </p> 207 */ 208 public CodingDt getCodingFirstRep() { 209 if (getCoding().isEmpty()) { 210 return addCoding(); 211 } 212 return getCoding().get(0); 213 } 214 215 /** 216 * Gets the value(s) for <b>text</b> (). 217 * creating it if it does 218 * not exist. Will not return <code>null</code>. 219 * 220 * <p> 221 * <b>Definition:</b> 222 * A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user 223 * </p> 224 */ 225 public StringDt getTextElement() { 226 if (myText == null) { 227 myText = new StringDt(); 228 } 229 return myText; 230 } 231 232 233 /** 234 * Gets the value(s) for <b>text</b> (). 235 * creating it if it does 236 * not exist. This method may return <code>null</code>. 237 * 238 * <p> 239 * <b>Definition:</b> 240 * A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user 241 * </p> 242 */ 243 public String getText() { 244 return getTextElement().getValue(); 245 } 246 247 /** 248 * Sets the value(s) for <b>text</b> () 249 * 250 * <p> 251 * <b>Definition:</b> 252 * A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user 253 * </p> 254 */ 255 public CodeableConceptDt setText(StringDt theValue) { 256 myText = theValue; 257 return this; 258 } 259 260 261 262 /** 263 * Sets the value for <b>text</b> () 264 * 265 * <p> 266 * <b>Definition:</b> 267 * A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user 268 * </p> 269 */ 270 public CodeableConceptDt setText( String theString) { 271 myText = new StringDt(theString); 272 return this; 273 } 274 275 276 277 278}