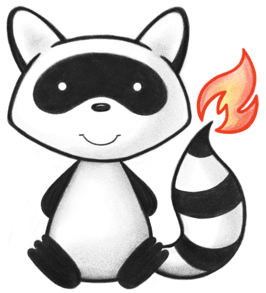
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>CodingDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * A reference to a code defined by a terminology system 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * References to codes are very common in healthcare models 088 * </p> 089 */ 090@DatatypeDef(name="Coding") 091public class CodingDt 092 extends BaseCodingDt 093 implements ICompositeDatatype, org.hl7.fhir.instance.model.api.IBaseCoding 094{ 095 096 /** 097 * Constructor 098 */ 099 public CodingDt() { 100 // nothing 101 } 102 103 /** 104 * Creates a new Coding with the given system and code 105 */ 106 public CodingDt(String theSystem, String theCode) { 107 setSystem(theSystem); 108 setCode(theCode); 109 } 110 111 /** 112 * Copy constructor: Creates a new Coding with the system and code copied out of the given coding 113 */ 114 public CodingDt(BaseCodingDt theCoding) { 115 this(theCoding.getSystemElement().getValueAsString(), theCoding.getCodeElement().getValue()); 116 } 117 118 119 @Child(name="system", type=UriDt.class, order=0, min=0, max=1, summary=true, modifier=false) 120 @Description( 121 shortDefinition="", 122 formalDefinition="The identification of the code system that defines the meaning of the symbol in the code." 123 ) 124 private UriDt mySystem; 125 126 @Child(name="version", type=StringDt.class, order=1, min=0, max=1, summary=true, modifier=false) 127 @Description( 128 shortDefinition="", 129 formalDefinition="The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged" 130 ) 131 private StringDt myVersion; 132 133 @Child(name="code", type=CodeDt.class, order=2, min=0, max=1, summary=true, modifier=false) 134 @Description( 135 shortDefinition="", 136 formalDefinition="A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination)" 137 ) 138 private CodeDt myCode; 139 140 @Child(name="display", type=StringDt.class, order=3, min=0, max=1, summary=true, modifier=false) 141 @Description( 142 shortDefinition="", 143 formalDefinition="A representation of the meaning of the code in the system, following the rules of the system" 144 ) 145 private StringDt myDisplay; 146 147 @Child(name="userSelected", type=BooleanDt.class, order=4, min=0, max=1, summary=true, modifier=false) 148 @Description( 149 shortDefinition="", 150 formalDefinition="Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays)" 151 ) 152 private BooleanDt myUserSelected; 153 154 155 @Override 156 public boolean isEmpty() { 157 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( mySystem, myVersion, myCode, myDisplay, myUserSelected); 158 } 159 160 @Override 161 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 162 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, mySystem, myVersion, myCode, myDisplay, myUserSelected); 163 } 164 165 /** 166 * Gets the value(s) for <b>system</b> (). 167 * creating it if it does 168 * not exist. Will not return <code>null</code>. 169 * 170 * <p> 171 * <b>Definition:</b> 172 * The identification of the code system that defines the meaning of the symbol in the code. 173 * </p> 174 */ 175 public UriDt getSystemElement() { 176 if (mySystem == null) { 177 mySystem = new UriDt(); 178 } 179 return mySystem; 180 } 181 182 183 /** 184 * Gets the value(s) for <b>system</b> (). 185 * creating it if it does 186 * not exist. This method may return <code>null</code>. 187 * 188 * <p> 189 * <b>Definition:</b> 190 * The identification of the code system that defines the meaning of the symbol in the code. 191 * </p> 192 */ 193 public String getSystem() { 194 return getSystemElement().getValue(); 195 } 196 197 /** 198 * Sets the value(s) for <b>system</b> () 199 * 200 * <p> 201 * <b>Definition:</b> 202 * The identification of the code system that defines the meaning of the symbol in the code. 203 * </p> 204 */ 205 public CodingDt setSystem(UriDt theValue) { 206 mySystem = theValue; 207 return this; 208 } 209 210 211 212 /** 213 * Sets the value for <b>system</b> () 214 * 215 * <p> 216 * <b>Definition:</b> 217 * The identification of the code system that defines the meaning of the symbol in the code. 218 * </p> 219 */ 220 public CodingDt setSystem( String theUri) { 221 mySystem = new UriDt(theUri); 222 return this; 223 } 224 225 226 /** 227 * Gets the value(s) for <b>version</b> (). 228 * creating it if it does 229 * not exist. Will not return <code>null</code>. 230 * 231 * <p> 232 * <b>Definition:</b> 233 * The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged 234 * </p> 235 */ 236 public StringDt getVersionElement() { 237 if (myVersion == null) { 238 myVersion = new StringDt(); 239 } 240 return myVersion; 241 } 242 243 244 /** 245 * Gets the value(s) for <b>version</b> (). 246 * creating it if it does 247 * not exist. This method may return <code>null</code>. 248 * 249 * <p> 250 * <b>Definition:</b> 251 * The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged 252 * </p> 253 */ 254 public String getVersion() { 255 return getVersionElement().getValue(); 256 } 257 258 /** 259 * Sets the value(s) for <b>version</b> () 260 * 261 * <p> 262 * <b>Definition:</b> 263 * The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged 264 * </p> 265 */ 266 public CodingDt setVersion(StringDt theValue) { 267 myVersion = theValue; 268 return this; 269 } 270 271 272 273 /** 274 * Sets the value for <b>version</b> () 275 * 276 * <p> 277 * <b>Definition:</b> 278 * The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged 279 * </p> 280 */ 281 public CodingDt setVersion( String theString) { 282 myVersion = new StringDt(theString); 283 return this; 284 } 285 286 287 /** 288 * Gets the value(s) for <b>code</b> (). 289 * creating it if it does 290 * not exist. Will not return <code>null</code>. 291 * 292 * <p> 293 * <b>Definition:</b> 294 * A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination) 295 * </p> 296 */ 297 public CodeDt getCodeElement() { 298 if (myCode == null) { 299 myCode = new CodeDt(); 300 } 301 return myCode; 302 } 303 304 305 /** 306 * Gets the value(s) for <b>code</b> (). 307 * creating it if it does 308 * not exist. This method may return <code>null</code>. 309 * 310 * <p> 311 * <b>Definition:</b> 312 * A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination) 313 * </p> 314 */ 315 public String getCode() { 316 return getCodeElement().getValue(); 317 } 318 319 /** 320 * Sets the value(s) for <b>code</b> () 321 * 322 * <p> 323 * <b>Definition:</b> 324 * A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination) 325 * </p> 326 */ 327 public CodingDt setCode(CodeDt theValue) { 328 myCode = theValue; 329 return this; 330 } 331 332 333 334 /** 335 * Sets the value for <b>code</b> () 336 * 337 * <p> 338 * <b>Definition:</b> 339 * A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination) 340 * </p> 341 */ 342 public CodingDt setCode( String theCode) { 343 myCode = new CodeDt(theCode); 344 return this; 345 } 346 347 348 /** 349 * Gets the value(s) for <b>display</b> (). 350 * creating it if it does 351 * not exist. Will not return <code>null</code>. 352 * 353 * <p> 354 * <b>Definition:</b> 355 * A representation of the meaning of the code in the system, following the rules of the system 356 * </p> 357 */ 358 public StringDt getDisplayElement() { 359 if (myDisplay == null) { 360 myDisplay = new StringDt(); 361 } 362 return myDisplay; 363 } 364 365 366 /** 367 * Gets the value(s) for <b>display</b> (). 368 * creating it if it does 369 * not exist. This method may return <code>null</code>. 370 * 371 * <p> 372 * <b>Definition:</b> 373 * A representation of the meaning of the code in the system, following the rules of the system 374 * </p> 375 */ 376 public String getDisplay() { 377 return getDisplayElement().getValue(); 378 } 379 380 /** 381 * Sets the value(s) for <b>display</b> () 382 * 383 * <p> 384 * <b>Definition:</b> 385 * A representation of the meaning of the code in the system, following the rules of the system 386 * </p> 387 */ 388 public CodingDt setDisplay(StringDt theValue) { 389 myDisplay = theValue; 390 return this; 391 } 392 393 394 395 /** 396 * Sets the value for <b>display</b> () 397 * 398 * <p> 399 * <b>Definition:</b> 400 * A representation of the meaning of the code in the system, following the rules of the system 401 * </p> 402 */ 403 public CodingDt setDisplay( String theString) { 404 myDisplay = new StringDt(theString); 405 return this; 406 } 407 408 409 /** 410 * Gets the value(s) for <b>userSelected</b> (). 411 * creating it if it does 412 * not exist. Will not return <code>null</code>. 413 * 414 * <p> 415 * <b>Definition:</b> 416 * Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays) 417 * </p> 418 */ 419 public BooleanDt getUserSelectedElement() { 420 if (myUserSelected == null) { 421 myUserSelected = new BooleanDt(); 422 } 423 return myUserSelected; 424 } 425 426 427 /** 428 * Gets the value(s) for <b>userSelected</b> (). 429 * creating it if it does 430 * not exist. This method may return <code>null</code>. 431 * 432 * <p> 433 * <b>Definition:</b> 434 * Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays) 435 * </p> 436 */ 437 public boolean getUserSelected() { 438 return getUserSelectedElement().getValue(); 439 } 440 441 /** 442 * Sets the value(s) for <b>userSelected</b> () 443 * 444 * <p> 445 * <b>Definition:</b> 446 * Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays) 447 * </p> 448 */ 449 public CodingDt setUserSelected(BooleanDt theValue) { 450 myUserSelected = theValue; 451 return this; 452 } 453 454 455 456 /** 457 * Sets the value for <b>userSelected</b> () 458 * 459 * <p> 460 * <b>Definition:</b> 461 * Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays) 462 * </p> 463 */ 464 public CodingDt setUserSelected( boolean theBoolean) { 465 myUserSelected = new BooleanDt(theBoolean); 466 return this; 467 } 468 469 470 471 472}