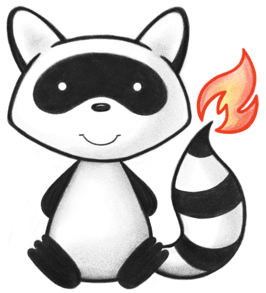
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>ContactPointDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc. 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * Need to track phone, fax, mobile, sms numbers, email addresses, twitter tags, etc. 088 * </p> 089 */ 090@DatatypeDef(name="ContactPoint") 091public class ContactPointDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public ContactPointDt() { 100 // nothing 101 } 102 103 104 @Child(name="system", type=CodeDt.class, order=0, min=0, max=1, summary=true, modifier=false) 105 @Description( 106 shortDefinition="", 107 formalDefinition="Telecommunications form for contact point - what communications system is required to make use of the contact" 108 ) 109 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contact-point-system") 110 private BoundCodeDt<ContactPointSystemEnum> mySystem; 111 112 @Child(name="value", type=StringDt.class, order=1, min=0, max=1, summary=true, modifier=false) 113 @Description( 114 shortDefinition="", 115 formalDefinition="The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address)." 116 ) 117 private StringDt myValue; 118 119 @Child(name="use", type=CodeDt.class, order=2, min=0, max=1, summary=true, modifier=true) 120 @Description( 121 shortDefinition="", 122 formalDefinition="Identifies the purpose for the contact point" 123 ) 124 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contact-point-use") 125 private BoundCodeDt<ContactPointUseEnum> myUse; 126 127 @Child(name="rank", type=PositiveIntDt.class, order=3, min=0, max=1, summary=true, modifier=false) 128 @Description( 129 shortDefinition="", 130 formalDefinition="Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values" 131 ) 132 private PositiveIntDt myRank; 133 134 @Child(name="period", type=PeriodDt.class, order=4, min=0, max=1, summary=true, modifier=false) 135 @Description( 136 shortDefinition="", 137 formalDefinition="Time period when the contact point was/is in use" 138 ) 139 private PeriodDt myPeriod; 140 141 142 @Override 143 public boolean isEmpty() { 144 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( mySystem, myValue, myUse, myRank, myPeriod); 145 } 146 147 @Override 148 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 149 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, mySystem, myValue, myUse, myRank, myPeriod); 150 } 151 152 /** 153 * Gets the value(s) for <b>system</b> (). 154 * creating it if it does 155 * not exist. Will not return <code>null</code>. 156 * 157 * <p> 158 * <b>Definition:</b> 159 * Telecommunications form for contact point - what communications system is required to make use of the contact 160 * </p> 161 */ 162 public BoundCodeDt<ContactPointSystemEnum> getSystemElement() { 163 if (mySystem == null) { 164 mySystem = new BoundCodeDt<ContactPointSystemEnum>(ContactPointSystemEnum.VALUESET_BINDER); 165 } 166 return mySystem; 167 } 168 169 170 /** 171 * Gets the value(s) for <b>system</b> (). 172 * creating it if it does 173 * not exist. This method may return <code>null</code>. 174 * 175 * <p> 176 * <b>Definition:</b> 177 * Telecommunications form for contact point - what communications system is required to make use of the contact 178 * </p> 179 */ 180 public String getSystem() { 181 return getSystemElement().getValue(); 182 } 183 184 /** 185 * Sets the value(s) for <b>system</b> () 186 * 187 * <p> 188 * <b>Definition:</b> 189 * Telecommunications form for contact point - what communications system is required to make use of the contact 190 * </p> 191 */ 192 public ContactPointDt setSystem(BoundCodeDt<ContactPointSystemEnum> theValue) { 193 mySystem = theValue; 194 return this; 195 } 196 197 198 199 /** 200 * Sets the value(s) for <b>system</b> () 201 * 202 * <p> 203 * <b>Definition:</b> 204 * Telecommunications form for contact point - what communications system is required to make use of the contact 205 * </p> 206 */ 207 public ContactPointDt setSystem(ContactPointSystemEnum theValue) { 208 setSystem(new BoundCodeDt<ContactPointSystemEnum>(ContactPointSystemEnum.VALUESET_BINDER, theValue)); 209 210/* 211 getSystemElement().setValueAsEnum(theValue); 212*/ 213 return this; 214 } 215 216 217 /** 218 * Gets the value(s) for <b>value</b> (). 219 * creating it if it does 220 * not exist. Will not return <code>null</code>. 221 * 222 * <p> 223 * <b>Definition:</b> 224 * The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address). 225 * </p> 226 */ 227 public StringDt getValueElement() { 228 if (myValue == null) { 229 myValue = new StringDt(); 230 } 231 return myValue; 232 } 233 234 235 /** 236 * Gets the value(s) for <b>value</b> (). 237 * creating it if it does 238 * not exist. This method may return <code>null</code>. 239 * 240 * <p> 241 * <b>Definition:</b> 242 * The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address). 243 * </p> 244 */ 245 public String getValue() { 246 return getValueElement().getValue(); 247 } 248 249 /** 250 * Sets the value(s) for <b>value</b> () 251 * 252 * <p> 253 * <b>Definition:</b> 254 * The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address). 255 * </p> 256 */ 257 public ContactPointDt setValue(StringDt theValue) { 258 myValue = theValue; 259 return this; 260 } 261 262 263 264 /** 265 * Sets the value for <b>value</b> () 266 * 267 * <p> 268 * <b>Definition:</b> 269 * The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address). 270 * </p> 271 */ 272 public ContactPointDt setValue( String theString) { 273 myValue = new StringDt(theString); 274 return this; 275 } 276 277 278 /** 279 * Gets the value(s) for <b>use</b> (). 280 * creating it if it does 281 * not exist. Will not return <code>null</code>. 282 * 283 * <p> 284 * <b>Definition:</b> 285 * Identifies the purpose for the contact point 286 * </p> 287 */ 288 public BoundCodeDt<ContactPointUseEnum> getUseElement() { 289 if (myUse == null) { 290 myUse = new BoundCodeDt<ContactPointUseEnum>(ContactPointUseEnum.VALUESET_BINDER); 291 } 292 return myUse; 293 } 294 295 296 /** 297 * Gets the value(s) for <b>use</b> (). 298 * creating it if it does 299 * not exist. This method may return <code>null</code>. 300 * 301 * <p> 302 * <b>Definition:</b> 303 * Identifies the purpose for the contact point 304 * </p> 305 */ 306 public String getUse() { 307 return getUseElement().getValue(); 308 } 309 310 /** 311 * Sets the value(s) for <b>use</b> () 312 * 313 * <p> 314 * <b>Definition:</b> 315 * Identifies the purpose for the contact point 316 * </p> 317 */ 318 public ContactPointDt setUse(BoundCodeDt<ContactPointUseEnum> theValue) { 319 myUse = theValue; 320 return this; 321 } 322 323 324 325 /** 326 * Sets the value(s) for <b>use</b> () 327 * 328 * <p> 329 * <b>Definition:</b> 330 * Identifies the purpose for the contact point 331 * </p> 332 */ 333 public ContactPointDt setUse(ContactPointUseEnum theValue) { 334 setUse(new BoundCodeDt<ContactPointUseEnum>(ContactPointUseEnum.VALUESET_BINDER, theValue)); 335 336/* 337 getUseElement().setValueAsEnum(theValue); 338*/ 339 return this; 340 } 341 342 343 /** 344 * Gets the value(s) for <b>rank</b> (). 345 * creating it if it does 346 * not exist. Will not return <code>null</code>. 347 * 348 * <p> 349 * <b>Definition:</b> 350 * Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values 351 * </p> 352 */ 353 public PositiveIntDt getRankElement() { 354 if (myRank == null) { 355 myRank = new PositiveIntDt(); 356 } 357 return myRank; 358 } 359 360 361 /** 362 * Gets the value(s) for <b>rank</b> (). 363 * creating it if it does 364 * not exist. This method may return <code>null</code>. 365 * 366 * <p> 367 * <b>Definition:</b> 368 * Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values 369 * </p> 370 */ 371 public Integer getRank() { 372 return getRankElement().getValue(); 373 } 374 375 /** 376 * Sets the value(s) for <b>rank</b> () 377 * 378 * <p> 379 * <b>Definition:</b> 380 * Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values 381 * </p> 382 */ 383 public ContactPointDt setRank(PositiveIntDt theValue) { 384 myRank = theValue; 385 return this; 386 } 387 388 389 390 /** 391 * Sets the value for <b>rank</b> () 392 * 393 * <p> 394 * <b>Definition:</b> 395 * Specifies a preferred order in which to use a set of contacts. Contacts are ranked with lower values coming before higher values 396 * </p> 397 */ 398 public ContactPointDt setRank( int theInteger) { 399 myRank = new PositiveIntDt(theInteger); 400 return this; 401 } 402 403 404 /** 405 * Gets the value(s) for <b>period</b> (). 406 * creating it if it does 407 * not exist. Will not return <code>null</code>. 408 * 409 * <p> 410 * <b>Definition:</b> 411 * Time period when the contact point was/is in use 412 * </p> 413 */ 414 public PeriodDt getPeriod() { 415 if (myPeriod == null) { 416 myPeriod = new PeriodDt(); 417 } 418 return myPeriod; 419 } 420 421 /** 422 * Sets the value(s) for <b>period</b> () 423 * 424 * <p> 425 * <b>Definition:</b> 426 * Time period when the contact point was/is in use 427 * </p> 428 */ 429 public ContactPointDt setPeriod(PeriodDt theValue) { 430 myPeriod = theValue; 431 return this; 432 } 433 434 435 436 437 438 439}