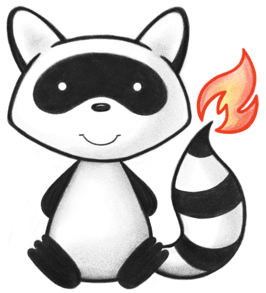
001/* 002 * #%L 003 * HAPI FHIR Structures - DSTU2 (FHIR v1.0.0) 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.dstu2.composite; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.model.api.IResource; 024import ca.uhn.fhir.model.api.annotation.Child; 025import ca.uhn.fhir.model.api.annotation.DatatypeDef; 026import ca.uhn.fhir.model.base.composite.BaseContainedDt; 027 028import java.util.ArrayList; 029import java.util.List; 030 031@DatatypeDef(name = "contained") 032public class ContainedDt extends BaseContainedDt { 033 034 @Child(name = "resource", type = IResource.class, order = 0, min = 0, max = Child.MAX_UNLIMITED) 035 private List<IResource> myContainedResources; 036 037 @Override 038 public List<IResource> getContainedResources() { 039 if (myContainedResources == null) { 040 myContainedResources = new ArrayList<IResource>(); 041 } 042 return myContainedResources; 043 } 044 045 public void setContainedResources(List<IResource> theContainedResources) { 046 myContainedResources = theContainedResources; 047 } 048 049 @Override 050 public boolean isEmpty() { 051 return myContainedResources == null || myContainedResources.size() == 0; 052 } 053 054 @Override 055 public Object getUserData(String theName) { 056 throw new UnsupportedOperationException(Msg.code(580)); 057 } 058 059 @Override 060 public void setUserData(String theName, Object theValue) { 061 throw new UnsupportedOperationException(Msg.code(581)); 062 } 063}