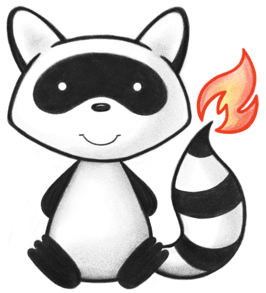
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>ElementDefinitionDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * Captures constraints on each element within the resource, profile, or extension 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * 088 * </p> 089 */ 090@DatatypeDef(name="ElementDefinition") 091public class ElementDefinitionDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public ElementDefinitionDt() { 100 // nothing 101 } 102 103 104 @Child(name="path", type=StringDt.class, order=0, min=1, max=1, summary=true, modifier=false) 105 @Description( 106 shortDefinition="", 107 formalDefinition="The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension" 108 ) 109 private StringDt myPath; 110 111 @Child(name="representation", type=CodeDt.class, order=1, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 112 @Description( 113 shortDefinition="", 114 formalDefinition="Codes that define how this element is represented in instances, when the deviation varies from the normal case" 115 ) 116 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/property-representation") 117 private java.util.List<BoundCodeDt<PropertyRepresentationEnum>> myRepresentation; 118 119 @Child(name="name", type=StringDt.class, order=2, min=0, max=1, summary=true, modifier=false) 120 @Description( 121 shortDefinition="", 122 formalDefinition="The name of this element definition (to refer to it from other element definitions using ElementDefinition.nameReference). This is a unique name referring to a specific set of constraints applied to this element. One use of this is to provide a name to different slices of the same element" 123 ) 124 private StringDt myName; 125 126 @Child(name="label", type=StringDt.class, order=3, min=0, max=1, summary=true, modifier=false) 127 @Description( 128 shortDefinition="", 129 formalDefinition="The text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form." 130 ) 131 private StringDt myLabel; 132 133 @Child(name="code", type=CodingDt.class, order=4, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 134 @Description( 135 shortDefinition="", 136 formalDefinition="A code that provides the meaning for the element according to a particular terminology." 137 ) 138 private java.util.List<CodingDt> myCode; 139 140 @Child(name="slicing", order=5, min=0, max=1, summary=true, modifier=false) 141 @Description( 142 shortDefinition="", 143 formalDefinition="Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set)" 144 ) 145 private Slicing mySlicing; 146 147 @Child(name="short", type=StringDt.class, order=6, min=0, max=1, summary=true, modifier=false) 148 @Description( 149 shortDefinition="", 150 formalDefinition="A concise description of what this element means (e.g. for use in autogenerated summaries)" 151 ) 152 private StringDt myShort; 153 154 @Child(name="definition", type=MarkdownDt.class, order=7, min=0, max=1, summary=true, modifier=false) 155 @Description( 156 shortDefinition="", 157 formalDefinition="Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource" 158 ) 159 private MarkdownDt myDefinition; 160 161 @Child(name="comments", type=MarkdownDt.class, order=8, min=0, max=1, summary=true, modifier=false) 162 @Description( 163 shortDefinition="", 164 formalDefinition="Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc." 165 ) 166 private MarkdownDt myComments; 167 168 @Child(name="requirements", type=MarkdownDt.class, order=9, min=0, max=1, summary=true, modifier=false) 169 @Description( 170 shortDefinition="", 171 formalDefinition="This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element." 172 ) 173 private MarkdownDt myRequirements; 174 175 @Child(name="alias", type=StringDt.class, order=10, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 176 @Description( 177 shortDefinition="", 178 formalDefinition="Identifies additional names by which this element might also be known" 179 ) 180 private java.util.List<StringDt> myAlias; 181 182 @Child(name="min", type=IntegerDt.class, order=11, min=0, max=1, summary=true, modifier=false) 183 @Description( 184 shortDefinition="", 185 formalDefinition="The minimum number of times this element SHALL appear in the instance" 186 ) 187 private IntegerDt myMin; 188 189 @Child(name="max", type=StringDt.class, order=12, min=0, max=1, summary=true, modifier=false) 190 @Description( 191 shortDefinition="", 192 formalDefinition="The maximum number of times this element is permitted to appear in the instance" 193 ) 194 private StringDt myMax; 195 196 @Child(name="base", order=13, min=0, max=1, summary=true, modifier=false) 197 @Description( 198 shortDefinition="", 199 formalDefinition="Information about the base definition of the element, provided to make it unncessary for tools to trace the deviation of the element through the derived and related profiles. This information is only provided where the element definition represents a constraint on another element definition, and must be present if there is a base element definition." 200 ) 201 private Base myBase; 202 203 @Child(name="type", order=14, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 204 @Description( 205 shortDefinition="", 206 formalDefinition="The data type or resource that the value of this element is permitted to be" 207 ) 208 private java.util.List<Type> myType; 209 210 @Child(name="nameReference", type=StringDt.class, order=15, min=0, max=1, summary=true, modifier=false) 211 @Description( 212 shortDefinition="", 213 formalDefinition="Identifies the name of a slice defined elsewhere in the profile whose constraints should be applied to the current element" 214 ) 215 private StringDt myNameReference; 216 217 @Child(name="defaultValue", type=IDatatype.class, order=16, min=0, max=1, summary=true, modifier=false) 218 @Description( 219 shortDefinition="", 220 formalDefinition="The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false')" 221 ) 222 private IDatatype myDefaultValue; 223 224 @Child(name="meaningWhenMissing", type=MarkdownDt.class, order=17, min=0, max=1, summary=true, modifier=false) 225 @Description( 226 shortDefinition="", 227 formalDefinition="The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'" 228 ) 229 private MarkdownDt myMeaningWhenMissing; 230 231 @Child(name="fixed", type=IDatatype.class, order=18, min=0, max=1, summary=true, modifier=false) 232 @Description( 233 shortDefinition="", 234 formalDefinition="Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing." 235 ) 236 private IDatatype myFixed; 237 238 @Child(name="pattern", type=IDatatype.class, order=19, min=0, max=1, summary=true, modifier=false) 239 @Description( 240 shortDefinition="", 241 formalDefinition="Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.)." 242 ) 243 private IDatatype myPattern; 244 245 @Child(name="example", type=IDatatype.class, order=20, min=0, max=1, summary=true, modifier=false) 246 @Description( 247 shortDefinition="", 248 formalDefinition="A sample value for this element demonstrating the type of information that would typically be captured." 249 ) 250 private IDatatype myExample; 251 252 @Child(name="minValue", type=IDatatype.class, order=21, min=0, max=1, summary=true, modifier=false) 253 @Description( 254 shortDefinition="", 255 formalDefinition="The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity" 256 ) 257 private IDatatype myMinValue; 258 259 @Child(name="maxValue", type=IDatatype.class, order=22, min=0, max=1, summary=true, modifier=false) 260 @Description( 261 shortDefinition="", 262 formalDefinition="The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity" 263 ) 264 private IDatatype myMaxValue; 265 266 @Child(name="maxLength", type=IntegerDt.class, order=23, min=0, max=1, summary=true, modifier=false) 267 @Description( 268 shortDefinition="", 269 formalDefinition="Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element" 270 ) 271 private IntegerDt myMaxLength; 272 273 @Child(name="condition", type=IdDt.class, order=24, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 274 @Description( 275 shortDefinition="", 276 formalDefinition="A reference to an invariant that may make additional statements about the cardinality or value in the instance" 277 ) 278 private java.util.List<IdDt> myCondition; 279 280 @Child(name="constraint", order=25, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 281 @Description( 282 shortDefinition="", 283 formalDefinition="Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance" 284 ) 285 private java.util.List<Constraint> myConstraint; 286 287 @Child(name="mustSupport", type=BooleanDt.class, order=26, min=0, max=1, summary=true, modifier=false) 288 @Description( 289 shortDefinition="", 290 formalDefinition="If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported" 291 ) 292 private BooleanDt myMustSupport; 293 294 @Child(name="isModifier", type=BooleanDt.class, order=27, min=0, max=1, summary=true, modifier=false) 295 @Description( 296 shortDefinition="", 297 formalDefinition="If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system." 298 ) 299 private BooleanDt myIsModifier; 300 301 @Child(name="isSummary", type=BooleanDt.class, order=28, min=0, max=1, summary=true, modifier=false) 302 @Description( 303 shortDefinition="", 304 formalDefinition="Whether the element should be included if a client requests a search with the parameter _summary=true" 305 ) 306 private BooleanDt myIsSummary; 307 308 @Child(name="binding", order=29, min=0, max=1, summary=true, modifier=false) 309 @Description( 310 shortDefinition="", 311 formalDefinition="Binds to a value set if this element is coded (code, Coding, CodeableConcept)" 312 ) 313 private Binding myBinding; 314 315 @Child(name="mapping", order=30, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 316 @Description( 317 shortDefinition="", 318 formalDefinition="Identifies a concept from an external specification that roughly corresponds to this element" 319 ) 320 private java.util.List<Mapping> myMapping; 321 322 323 @Override 324 public boolean isEmpty() { 325 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myPath, myRepresentation, myName, myLabel, myCode, mySlicing, myShort, myDefinition, myComments, myRequirements, myAlias, myMin, myMax, myBase, myType, myNameReference, myDefaultValue, myMeaningWhenMissing, myFixed, myPattern, myExample, myMinValue, myMaxValue, myMaxLength, myCondition, myConstraint, myMustSupport, myIsModifier, myIsSummary, myBinding, myMapping); 326 } 327 328 @Override 329 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 330 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myPath, myRepresentation, myName, myLabel, myCode, mySlicing, myShort, myDefinition, myComments, myRequirements, myAlias, myMin, myMax, myBase, myType, myNameReference, myDefaultValue, myMeaningWhenMissing, myFixed, myPattern, myExample, myMinValue, myMaxValue, myMaxLength, myCondition, myConstraint, myMustSupport, myIsModifier, myIsSummary, myBinding, myMapping); 331 } 332 333 /** 334 * Gets the value(s) for <b>path</b> (). 335 * creating it if it does 336 * not exist. Will not return <code>null</code>. 337 * 338 * <p> 339 * <b>Definition:</b> 340 * The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension 341 * </p> 342 */ 343 public StringDt getPathElement() { 344 if (myPath == null) { 345 myPath = new StringDt(); 346 } 347 return myPath; 348 } 349 350 351 /** 352 * Gets the value(s) for <b>path</b> (). 353 * creating it if it does 354 * not exist. This method may return <code>null</code>. 355 * 356 * <p> 357 * <b>Definition:</b> 358 * The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension 359 * </p> 360 */ 361 public String getPath() { 362 return getPathElement().getValue(); 363 } 364 365 /** 366 * Sets the value(s) for <b>path</b> () 367 * 368 * <p> 369 * <b>Definition:</b> 370 * The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension 371 * </p> 372 */ 373 public ElementDefinitionDt setPath(StringDt theValue) { 374 myPath = theValue; 375 return this; 376 } 377 378 379 380 /** 381 * Sets the value for <b>path</b> () 382 * 383 * <p> 384 * <b>Definition:</b> 385 * The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension 386 * </p> 387 */ 388 public ElementDefinitionDt setPath( String theString) { 389 myPath = new StringDt(theString); 390 return this; 391 } 392 393 394 /** 395 * Gets the value(s) for <b>representation</b> (). 396 * creating it if it does 397 * not exist. Will not return <code>null</code>. 398 * 399 * <p> 400 * <b>Definition:</b> 401 * Codes that define how this element is represented in instances, when the deviation varies from the normal case 402 * </p> 403 */ 404 public java.util.List<BoundCodeDt<PropertyRepresentationEnum>> getRepresentation() { 405 if (myRepresentation == null) { 406 myRepresentation = new java.util.ArrayList<BoundCodeDt<PropertyRepresentationEnum>>(); 407 } 408 return myRepresentation; 409 } 410 411 /** 412 * Sets the value(s) for <b>representation</b> () 413 * 414 * <p> 415 * <b>Definition:</b> 416 * Codes that define how this element is represented in instances, when the deviation varies from the normal case 417 * </p> 418 */ 419 public ElementDefinitionDt setRepresentation(java.util.List<BoundCodeDt<PropertyRepresentationEnum>> theValue) { 420 myRepresentation = theValue; 421 return this; 422 } 423 424 425 426 /** 427 * Add a value for <b>representation</b> () using an enumerated type. This 428 * is intended as a convenience method for situations where the FHIR defined ValueSets are mandatory 429 * or contain the desirable codes. If you wish to use codes other than those which are built-in, 430 * you may also use the {@link #addRepresentation()} method. 431 * 432 * <p> 433 * <b>Definition:</b> 434 * Codes that define how this element is represented in instances, when the deviation varies from the normal case 435 * </p> 436 */ 437 public BoundCodeDt<PropertyRepresentationEnum> addRepresentation(PropertyRepresentationEnum theValue) { 438 BoundCodeDt<PropertyRepresentationEnum> retVal = new BoundCodeDt<PropertyRepresentationEnum>(PropertyRepresentationEnum.VALUESET_BINDER, theValue); 439 getRepresentation().add(retVal); 440 return retVal; 441 } 442 443 /** 444 * Gets the first repetition for <b>representation</b> (), 445 * creating it if it does not already exist. 446 * 447 * <p> 448 * <b>Definition:</b> 449 * Codes that define how this element is represented in instances, when the deviation varies from the normal case 450 * </p> 451 */ 452 public BoundCodeDt<PropertyRepresentationEnum> getRepresentationFirstRep() { 453 if (getRepresentation().size() == 0) { 454 addRepresentation(); 455 } 456 return getRepresentation().get(0); 457 } 458 459 /** 460 * Add a value for <b>representation</b> () 461 * 462 * <p> 463 * <b>Definition:</b> 464 * Codes that define how this element is represented in instances, when the deviation varies from the normal case 465 * </p> 466 */ 467 public BoundCodeDt<PropertyRepresentationEnum> addRepresentation() { 468 BoundCodeDt<PropertyRepresentationEnum> retVal = new BoundCodeDt<PropertyRepresentationEnum>(PropertyRepresentationEnum.VALUESET_BINDER); 469 getRepresentation().add(retVal); 470 return retVal; 471 } 472 473 /** 474 * Sets the value(s), and clears any existing value(s) for <b>representation</b> () 475 * 476 * <p> 477 * <b>Definition:</b> 478 * Codes that define how this element is represented in instances, when the deviation varies from the normal case 479 * </p> 480 */ 481 public ElementDefinitionDt setRepresentation(PropertyRepresentationEnum theValue) { 482 getRepresentation().clear(); 483 addRepresentation(theValue); 484 return this; 485 } 486 487 488 /** 489 * Gets the value(s) for <b>name</b> (). 490 * creating it if it does 491 * not exist. Will not return <code>null</code>. 492 * 493 * <p> 494 * <b>Definition:</b> 495 * The name of this element definition (to refer to it from other element definitions using ElementDefinition.nameReference). This is a unique name referring to a specific set of constraints applied to this element. One use of this is to provide a name to different slices of the same element 496 * </p> 497 */ 498 public StringDt getNameElement() { 499 if (myName == null) { 500 myName = new StringDt(); 501 } 502 return myName; 503 } 504 505 506 /** 507 * Gets the value(s) for <b>name</b> (). 508 * creating it if it does 509 * not exist. This method may return <code>null</code>. 510 * 511 * <p> 512 * <b>Definition:</b> 513 * The name of this element definition (to refer to it from other element definitions using ElementDefinition.nameReference). This is a unique name referring to a specific set of constraints applied to this element. One use of this is to provide a name to different slices of the same element 514 * </p> 515 */ 516 public String getName() { 517 return getNameElement().getValue(); 518 } 519 520 /** 521 * Sets the value(s) for <b>name</b> () 522 * 523 * <p> 524 * <b>Definition:</b> 525 * The name of this element definition (to refer to it from other element definitions using ElementDefinition.nameReference). This is a unique name referring to a specific set of constraints applied to this element. One use of this is to provide a name to different slices of the same element 526 * </p> 527 */ 528 public ElementDefinitionDt setName(StringDt theValue) { 529 myName = theValue; 530 return this; 531 } 532 533 534 535 /** 536 * Sets the value for <b>name</b> () 537 * 538 * <p> 539 * <b>Definition:</b> 540 * The name of this element definition (to refer to it from other element definitions using ElementDefinition.nameReference). This is a unique name referring to a specific set of constraints applied to this element. One use of this is to provide a name to different slices of the same element 541 * </p> 542 */ 543 public ElementDefinitionDt setName( String theString) { 544 myName = new StringDt(theString); 545 return this; 546 } 547 548 549 /** 550 * Gets the value(s) for <b>label</b> (). 551 * creating it if it does 552 * not exist. Will not return <code>null</code>. 553 * 554 * <p> 555 * <b>Definition:</b> 556 * The text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 557 * </p> 558 */ 559 public StringDt getLabelElement() { 560 if (myLabel == null) { 561 myLabel = new StringDt(); 562 } 563 return myLabel; 564 } 565 566 567 /** 568 * Gets the value(s) for <b>label</b> (). 569 * creating it if it does 570 * not exist. This method may return <code>null</code>. 571 * 572 * <p> 573 * <b>Definition:</b> 574 * The text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 575 * </p> 576 */ 577 public String getLabel() { 578 return getLabelElement().getValue(); 579 } 580 581 /** 582 * Sets the value(s) for <b>label</b> () 583 * 584 * <p> 585 * <b>Definition:</b> 586 * The text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 587 * </p> 588 */ 589 public ElementDefinitionDt setLabel(StringDt theValue) { 590 myLabel = theValue; 591 return this; 592 } 593 594 595 596 /** 597 * Sets the value for <b>label</b> () 598 * 599 * <p> 600 * <b>Definition:</b> 601 * The text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 602 * </p> 603 */ 604 public ElementDefinitionDt setLabel( String theString) { 605 myLabel = new StringDt(theString); 606 return this; 607 } 608 609 610 /** 611 * Gets the value(s) for <b>code</b> (). 612 * creating it if it does 613 * not exist. Will not return <code>null</code>. 614 * 615 * <p> 616 * <b>Definition:</b> 617 * A code that provides the meaning for the element according to a particular terminology. 618 * </p> 619 */ 620 public java.util.List<CodingDt> getCode() { 621 if (myCode == null) { 622 myCode = new java.util.ArrayList<CodingDt>(); 623 } 624 return myCode; 625 } 626 627 /** 628 * Sets the value(s) for <b>code</b> () 629 * 630 * <p> 631 * <b>Definition:</b> 632 * A code that provides the meaning for the element according to a particular terminology. 633 * </p> 634 */ 635 public ElementDefinitionDt setCode(java.util.List<CodingDt> theValue) { 636 myCode = theValue; 637 return this; 638 } 639 640 641 642 /** 643 * Adds and returns a new value for <b>code</b> () 644 * 645 * <p> 646 * <b>Definition:</b> 647 * A code that provides the meaning for the element according to a particular terminology. 648 * </p> 649 */ 650 public CodingDt addCode() { 651 CodingDt newType = new CodingDt(); 652 getCode().add(newType); 653 return newType; 654 } 655 656 /** 657 * Adds a given new value for <b>code</b> () 658 * 659 * <p> 660 * <b>Definition:</b> 661 * A code that provides the meaning for the element according to a particular terminology. 662 * </p> 663 * @param theValue The code to add (must not be <code>null</code>) 664 */ 665 public ElementDefinitionDt addCode(CodingDt theValue) { 666 if (theValue == null) { 667 throw new NullPointerException("theValue must not be null"); 668 } 669 getCode().add(theValue); 670 return this; 671 } 672 673 /** 674 * Gets the first repetition for <b>code</b> (), 675 * creating it if it does not already exist. 676 * 677 * <p> 678 * <b>Definition:</b> 679 * A code that provides the meaning for the element according to a particular terminology. 680 * </p> 681 */ 682 public CodingDt getCodeFirstRep() { 683 if (getCode().isEmpty()) { 684 return addCode(); 685 } 686 return getCode().get(0); 687 } 688 689 /** 690 * Gets the value(s) for <b>slicing</b> (). 691 * creating it if it does 692 * not exist. Will not return <code>null</code>. 693 * 694 * <p> 695 * <b>Definition:</b> 696 * Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set) 697 * </p> 698 */ 699 public Slicing getSlicing() { 700 if (mySlicing == null) { 701 mySlicing = new Slicing(); 702 } 703 return mySlicing; 704 } 705 706 /** 707 * Sets the value(s) for <b>slicing</b> () 708 * 709 * <p> 710 * <b>Definition:</b> 711 * Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set) 712 * </p> 713 */ 714 public ElementDefinitionDt setSlicing(Slicing theValue) { 715 mySlicing = theValue; 716 return this; 717 } 718 719 720 721 722 /** 723 * Gets the value(s) for <b>short</b> (). 724 * creating it if it does 725 * not exist. Will not return <code>null</code>. 726 * 727 * <p> 728 * <b>Definition:</b> 729 * A concise description of what this element means (e.g. for use in autogenerated summaries) 730 * </p> 731 */ 732 public StringDt getShortElement() { 733 if (myShort == null) { 734 myShort = new StringDt(); 735 } 736 return myShort; 737 } 738 739 740 /** 741 * Gets the value(s) for <b>short</b> (). 742 * creating it if it does 743 * not exist. This method may return <code>null</code>. 744 * 745 * <p> 746 * <b>Definition:</b> 747 * A concise description of what this element means (e.g. for use in autogenerated summaries) 748 * </p> 749 */ 750 public String getShort() { 751 return getShortElement().getValue(); 752 } 753 754 /** 755 * Sets the value(s) for <b>short</b> () 756 * 757 * <p> 758 * <b>Definition:</b> 759 * A concise description of what this element means (e.g. for use in autogenerated summaries) 760 * </p> 761 */ 762 public ElementDefinitionDt setShort(StringDt theValue) { 763 myShort = theValue; 764 return this; 765 } 766 767 768 769 /** 770 * Sets the value for <b>short</b> () 771 * 772 * <p> 773 * <b>Definition:</b> 774 * A concise description of what this element means (e.g. for use in autogenerated summaries) 775 * </p> 776 */ 777 public ElementDefinitionDt setShort( String theString) { 778 myShort = new StringDt(theString); 779 return this; 780 } 781 782 783 /** 784 * Gets the value(s) for <b>definition</b> (). 785 * creating it if it does 786 * not exist. Will not return <code>null</code>. 787 * 788 * <p> 789 * <b>Definition:</b> 790 * Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource 791 * </p> 792 */ 793 public MarkdownDt getDefinitionElement() { 794 if (myDefinition == null) { 795 myDefinition = new MarkdownDt(); 796 } 797 return myDefinition; 798 } 799 800 801 /** 802 * Gets the value(s) for <b>definition</b> (). 803 * creating it if it does 804 * not exist. This method may return <code>null</code>. 805 * 806 * <p> 807 * <b>Definition:</b> 808 * Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource 809 * </p> 810 */ 811 public String getDefinition() { 812 return getDefinitionElement().getValue(); 813 } 814 815 /** 816 * Sets the value(s) for <b>definition</b> () 817 * 818 * <p> 819 * <b>Definition:</b> 820 * Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource 821 * </p> 822 */ 823 public ElementDefinitionDt setDefinition(MarkdownDt theValue) { 824 myDefinition = theValue; 825 return this; 826 } 827 828 829 830 831 /** 832 * Gets the value(s) for <b>comments</b> (). 833 * creating it if it does 834 * not exist. Will not return <code>null</code>. 835 * 836 * <p> 837 * <b>Definition:</b> 838 * Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. 839 * </p> 840 */ 841 public MarkdownDt getCommentsElement() { 842 if (myComments == null) { 843 myComments = new MarkdownDt(); 844 } 845 return myComments; 846 } 847 848 849 /** 850 * Gets the value(s) for <b>comments</b> (). 851 * creating it if it does 852 * not exist. This method may return <code>null</code>. 853 * 854 * <p> 855 * <b>Definition:</b> 856 * Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. 857 * </p> 858 */ 859 public String getComments() { 860 return getCommentsElement().getValue(); 861 } 862 863 /** 864 * Sets the value(s) for <b>comments</b> () 865 * 866 * <p> 867 * <b>Definition:</b> 868 * Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. 869 * </p> 870 */ 871 public ElementDefinitionDt setComments(MarkdownDt theValue) { 872 myComments = theValue; 873 return this; 874 } 875 876 877 878 879 /** 880 * Gets the value(s) for <b>requirements</b> (). 881 * creating it if it does 882 * not exist. Will not return <code>null</code>. 883 * 884 * <p> 885 * <b>Definition:</b> 886 * This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 887 * </p> 888 */ 889 public MarkdownDt getRequirementsElement() { 890 if (myRequirements == null) { 891 myRequirements = new MarkdownDt(); 892 } 893 return myRequirements; 894 } 895 896 897 /** 898 * Gets the value(s) for <b>requirements</b> (). 899 * creating it if it does 900 * not exist. This method may return <code>null</code>. 901 * 902 * <p> 903 * <b>Definition:</b> 904 * This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 905 * </p> 906 */ 907 public String getRequirements() { 908 return getRequirementsElement().getValue(); 909 } 910 911 /** 912 * Sets the value(s) for <b>requirements</b> () 913 * 914 * <p> 915 * <b>Definition:</b> 916 * This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 917 * </p> 918 */ 919 public ElementDefinitionDt setRequirements(MarkdownDt theValue) { 920 myRequirements = theValue; 921 return this; 922 } 923 924 925 926 927 /** 928 * Gets the value(s) for <b>alias</b> (). 929 * creating it if it does 930 * not exist. Will not return <code>null</code>. 931 * 932 * <p> 933 * <b>Definition:</b> 934 * Identifies additional names by which this element might also be known 935 * </p> 936 */ 937 public java.util.List<StringDt> getAlias() { 938 if (myAlias == null) { 939 myAlias = new java.util.ArrayList<StringDt>(); 940 } 941 return myAlias; 942 } 943 944 /** 945 * Sets the value(s) for <b>alias</b> () 946 * 947 * <p> 948 * <b>Definition:</b> 949 * Identifies additional names by which this element might also be known 950 * </p> 951 */ 952 public ElementDefinitionDt setAlias(java.util.List<StringDt> theValue) { 953 myAlias = theValue; 954 return this; 955 } 956 957 958 959 /** 960 * Adds and returns a new value for <b>alias</b> () 961 * 962 * <p> 963 * <b>Definition:</b> 964 * Identifies additional names by which this element might also be known 965 * </p> 966 */ 967 public StringDt addAlias() { 968 StringDt newType = new StringDt(); 969 getAlias().add(newType); 970 return newType; 971 } 972 973 /** 974 * Adds a given new value for <b>alias</b> () 975 * 976 * <p> 977 * <b>Definition:</b> 978 * Identifies additional names by which this element might also be known 979 * </p> 980 * @param theValue The alias to add (must not be <code>null</code>) 981 */ 982 public ElementDefinitionDt addAlias(StringDt theValue) { 983 if (theValue == null) { 984 throw new NullPointerException("theValue must not be null"); 985 } 986 getAlias().add(theValue); 987 return this; 988 } 989 990 /** 991 * Gets the first repetition for <b>alias</b> (), 992 * creating it if it does not already exist. 993 * 994 * <p> 995 * <b>Definition:</b> 996 * Identifies additional names by which this element might also be known 997 * </p> 998 */ 999 public StringDt getAliasFirstRep() { 1000 if (getAlias().isEmpty()) { 1001 return addAlias(); 1002 } 1003 return getAlias().get(0); 1004 } 1005 /** 1006 * Adds a new value for <b>alias</b> () 1007 * 1008 * <p> 1009 * <b>Definition:</b> 1010 * Identifies additional names by which this element might also be known 1011 * </p> 1012 * 1013 * @return Returns a reference to this object, to allow for simple chaining. 1014 */ 1015 public ElementDefinitionDt addAlias( String theString) { 1016 if (myAlias == null) { 1017 myAlias = new java.util.ArrayList<StringDt>(); 1018 } 1019 myAlias.add(new StringDt(theString)); 1020 return this; 1021 } 1022 1023 1024 /** 1025 * Gets the value(s) for <b>min</b> (). 1026 * creating it if it does 1027 * not exist. Will not return <code>null</code>. 1028 * 1029 * <p> 1030 * <b>Definition:</b> 1031 * The minimum number of times this element SHALL appear in the instance 1032 * </p> 1033 */ 1034 public IntegerDt getMinElement() { 1035 if (myMin == null) { 1036 myMin = new IntegerDt(); 1037 } 1038 return myMin; 1039 } 1040 1041 1042 /** 1043 * Gets the value(s) for <b>min</b> (). 1044 * creating it if it does 1045 * not exist. This method may return <code>null</code>. 1046 * 1047 * <p> 1048 * <b>Definition:</b> 1049 * The minimum number of times this element SHALL appear in the instance 1050 * </p> 1051 */ 1052 public Integer getMin() { 1053 return getMinElement().getValue(); 1054 } 1055 1056 /** 1057 * Sets the value(s) for <b>min</b> () 1058 * 1059 * <p> 1060 * <b>Definition:</b> 1061 * The minimum number of times this element SHALL appear in the instance 1062 * </p> 1063 */ 1064 public ElementDefinitionDt setMin(IntegerDt theValue) { 1065 myMin = theValue; 1066 return this; 1067 } 1068 1069 1070 1071 /** 1072 * Sets the value for <b>min</b> () 1073 * 1074 * <p> 1075 * <b>Definition:</b> 1076 * The minimum number of times this element SHALL appear in the instance 1077 * </p> 1078 */ 1079 public ElementDefinitionDt setMin( int theInteger) { 1080 myMin = new IntegerDt(theInteger); 1081 return this; 1082 } 1083 1084 1085 /** 1086 * Gets the value(s) for <b>max</b> (). 1087 * creating it if it does 1088 * not exist. Will not return <code>null</code>. 1089 * 1090 * <p> 1091 * <b>Definition:</b> 1092 * The maximum number of times this element is permitted to appear in the instance 1093 * </p> 1094 */ 1095 public StringDt getMaxElement() { 1096 if (myMax == null) { 1097 myMax = new StringDt(); 1098 } 1099 return myMax; 1100 } 1101 1102 1103 /** 1104 * Gets the value(s) for <b>max</b> (). 1105 * creating it if it does 1106 * not exist. This method may return <code>null</code>. 1107 * 1108 * <p> 1109 * <b>Definition:</b> 1110 * The maximum number of times this element is permitted to appear in the instance 1111 * </p> 1112 */ 1113 public String getMax() { 1114 return getMaxElement().getValue(); 1115 } 1116 1117 /** 1118 * Sets the value(s) for <b>max</b> () 1119 * 1120 * <p> 1121 * <b>Definition:</b> 1122 * The maximum number of times this element is permitted to appear in the instance 1123 * </p> 1124 */ 1125 public ElementDefinitionDt setMax(StringDt theValue) { 1126 myMax = theValue; 1127 return this; 1128 } 1129 1130 1131 1132 /** 1133 * Sets the value for <b>max</b> () 1134 * 1135 * <p> 1136 * <b>Definition:</b> 1137 * The maximum number of times this element is permitted to appear in the instance 1138 * </p> 1139 */ 1140 public ElementDefinitionDt setMax( String theString) { 1141 myMax = new StringDt(theString); 1142 return this; 1143 } 1144 1145 1146 /** 1147 * Gets the value(s) for <b>base</b> (). 1148 * creating it if it does 1149 * not exist. Will not return <code>null</code>. 1150 * 1151 * <p> 1152 * <b>Definition:</b> 1153 * Information about the base definition of the element, provided to make it unncessary for tools to trace the deviation of the element through the derived and related profiles. This information is only provided where the element definition represents a constraint on another element definition, and must be present if there is a base element definition. 1154 * </p> 1155 */ 1156 public Base getBase() { 1157 if (myBase == null) { 1158 myBase = new Base(); 1159 } 1160 return myBase; 1161 } 1162 1163 /** 1164 * Sets the value(s) for <b>base</b> () 1165 * 1166 * <p> 1167 * <b>Definition:</b> 1168 * Information about the base definition of the element, provided to make it unncessary for tools to trace the deviation of the element through the derived and related profiles. This information is only provided where the element definition represents a constraint on another element definition, and must be present if there is a base element definition. 1169 * </p> 1170 */ 1171 public ElementDefinitionDt setBase(Base theValue) { 1172 myBase = theValue; 1173 return this; 1174 } 1175 1176 1177 1178 1179 /** 1180 * Gets the value(s) for <b>type</b> (). 1181 * creating it if it does 1182 * not exist. Will not return <code>null</code>. 1183 * 1184 * <p> 1185 * <b>Definition:</b> 1186 * The data type or resource that the value of this element is permitted to be 1187 * </p> 1188 */ 1189 public java.util.List<Type> getType() { 1190 if (myType == null) { 1191 myType = new java.util.ArrayList<Type>(); 1192 } 1193 return myType; 1194 } 1195 1196 /** 1197 * Sets the value(s) for <b>type</b> () 1198 * 1199 * <p> 1200 * <b>Definition:</b> 1201 * The data type or resource that the value of this element is permitted to be 1202 * </p> 1203 */ 1204 public ElementDefinitionDt setType(java.util.List<Type> theValue) { 1205 myType = theValue; 1206 return this; 1207 } 1208 1209 1210 1211 /** 1212 * Adds and returns a new value for <b>type</b> () 1213 * 1214 * <p> 1215 * <b>Definition:</b> 1216 * The data type or resource that the value of this element is permitted to be 1217 * </p> 1218 */ 1219 public Type addType() { 1220 Type newType = new Type(); 1221 getType().add(newType); 1222 return newType; 1223 } 1224 1225 /** 1226 * Adds a given new value for <b>type</b> () 1227 * 1228 * <p> 1229 * <b>Definition:</b> 1230 * The data type or resource that the value of this element is permitted to be 1231 * </p> 1232 * @param theValue The type to add (must not be <code>null</code>) 1233 */ 1234 public ElementDefinitionDt addType(Type theValue) { 1235 if (theValue == null) { 1236 throw new NullPointerException("theValue must not be null"); 1237 } 1238 getType().add(theValue); 1239 return this; 1240 } 1241 1242 /** 1243 * Gets the first repetition for <b>type</b> (), 1244 * creating it if it does not already exist. 1245 * 1246 * <p> 1247 * <b>Definition:</b> 1248 * The data type or resource that the value of this element is permitted to be 1249 * </p> 1250 */ 1251 public Type getTypeFirstRep() { 1252 if (getType().isEmpty()) { 1253 return addType(); 1254 } 1255 return getType().get(0); 1256 } 1257 1258 /** 1259 * Gets the value(s) for <b>nameReference</b> (). 1260 * creating it if it does 1261 * not exist. Will not return <code>null</code>. 1262 * 1263 * <p> 1264 * <b>Definition:</b> 1265 * Identifies the name of a slice defined elsewhere in the profile whose constraints should be applied to the current element 1266 * </p> 1267 */ 1268 public StringDt getNameReferenceElement() { 1269 if (myNameReference == null) { 1270 myNameReference = new StringDt(); 1271 } 1272 return myNameReference; 1273 } 1274 1275 1276 /** 1277 * Gets the value(s) for <b>nameReference</b> (). 1278 * creating it if it does 1279 * not exist. This method may return <code>null</code>. 1280 * 1281 * <p> 1282 * <b>Definition:</b> 1283 * Identifies the name of a slice defined elsewhere in the profile whose constraints should be applied to the current element 1284 * </p> 1285 */ 1286 public String getNameReference() { 1287 return getNameReferenceElement().getValue(); 1288 } 1289 1290 /** 1291 * Sets the value(s) for <b>nameReference</b> () 1292 * 1293 * <p> 1294 * <b>Definition:</b> 1295 * Identifies the name of a slice defined elsewhere in the profile whose constraints should be applied to the current element 1296 * </p> 1297 */ 1298 public ElementDefinitionDt setNameReference(StringDt theValue) { 1299 myNameReference = theValue; 1300 return this; 1301 } 1302 1303 1304 1305 /** 1306 * Sets the value for <b>nameReference</b> () 1307 * 1308 * <p> 1309 * <b>Definition:</b> 1310 * Identifies the name of a slice defined elsewhere in the profile whose constraints should be applied to the current element 1311 * </p> 1312 */ 1313 public ElementDefinitionDt setNameReference( String theString) { 1314 myNameReference = new StringDt(theString); 1315 return this; 1316 } 1317 1318 1319 /** 1320 * Gets the value(s) for <b>defaultValue[x]</b> (). 1321 * creating it if it does 1322 * not exist. Will not return <code>null</code>. 1323 * 1324 * <p> 1325 * <b>Definition:</b> 1326 * The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false') 1327 * </p> 1328 */ 1329 public IDatatype getDefaultValue() { 1330 return myDefaultValue; 1331 } 1332 1333 /** 1334 * Sets the value(s) for <b>defaultValue[x]</b> () 1335 * 1336 * <p> 1337 * <b>Definition:</b> 1338 * The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false') 1339 * </p> 1340 */ 1341 public ElementDefinitionDt setDefaultValue(IDatatype theValue) { 1342 myDefaultValue = theValue; 1343 return this; 1344 } 1345 1346 1347 1348 1349 /** 1350 * Gets the value(s) for <b>meaningWhenMissing</b> (). 1351 * creating it if it does 1352 * not exist. Will not return <code>null</code>. 1353 * 1354 * <p> 1355 * <b>Definition:</b> 1356 * The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing' 1357 * </p> 1358 */ 1359 public MarkdownDt getMeaningWhenMissingElement() { 1360 if (myMeaningWhenMissing == null) { 1361 myMeaningWhenMissing = new MarkdownDt(); 1362 } 1363 return myMeaningWhenMissing; 1364 } 1365 1366 1367 /** 1368 * Gets the value(s) for <b>meaningWhenMissing</b> (). 1369 * creating it if it does 1370 * not exist. This method may return <code>null</code>. 1371 * 1372 * <p> 1373 * <b>Definition:</b> 1374 * The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing' 1375 * </p> 1376 */ 1377 public String getMeaningWhenMissing() { 1378 return getMeaningWhenMissingElement().getValue(); 1379 } 1380 1381 /** 1382 * Sets the value(s) for <b>meaningWhenMissing</b> () 1383 * 1384 * <p> 1385 * <b>Definition:</b> 1386 * The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing' 1387 * </p> 1388 */ 1389 public ElementDefinitionDt setMeaningWhenMissing(MarkdownDt theValue) { 1390 myMeaningWhenMissing = theValue; 1391 return this; 1392 } 1393 1394 1395 1396 1397 /** 1398 * Gets the value(s) for <b>fixed[x]</b> (). 1399 * creating it if it does 1400 * not exist. Will not return <code>null</code>. 1401 * 1402 * <p> 1403 * <b>Definition:</b> 1404 * Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing. 1405 * </p> 1406 */ 1407 public IDatatype getFixed() { 1408 return myFixed; 1409 } 1410 1411 /** 1412 * Sets the value(s) for <b>fixed[x]</b> () 1413 * 1414 * <p> 1415 * <b>Definition:</b> 1416 * Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing. 1417 * </p> 1418 */ 1419 public ElementDefinitionDt setFixed(IDatatype theValue) { 1420 myFixed = theValue; 1421 return this; 1422 } 1423 1424 1425 1426 1427 /** 1428 * Gets the value(s) for <b>pattern[x]</b> (). 1429 * creating it if it does 1430 * not exist. Will not return <code>null</code>. 1431 * 1432 * <p> 1433 * <b>Definition:</b> 1434 * Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.). 1435 * </p> 1436 */ 1437 public IDatatype getPattern() { 1438 return myPattern; 1439 } 1440 1441 /** 1442 * Sets the value(s) for <b>pattern[x]</b> () 1443 * 1444 * <p> 1445 * <b>Definition:</b> 1446 * Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.). 1447 * </p> 1448 */ 1449 public ElementDefinitionDt setPattern(IDatatype theValue) { 1450 myPattern = theValue; 1451 return this; 1452 } 1453 1454 1455 1456 1457 /** 1458 * Gets the value(s) for <b>example[x]</b> (). 1459 * creating it if it does 1460 * not exist. Will not return <code>null</code>. 1461 * 1462 * <p> 1463 * <b>Definition:</b> 1464 * A sample value for this element demonstrating the type of information that would typically be captured. 1465 * </p> 1466 */ 1467 public IDatatype getExample() { 1468 return myExample; 1469 } 1470 1471 /** 1472 * Sets the value(s) for <b>example[x]</b> () 1473 * 1474 * <p> 1475 * <b>Definition:</b> 1476 * A sample value for this element demonstrating the type of information that would typically be captured. 1477 * </p> 1478 */ 1479 public ElementDefinitionDt setExample(IDatatype theValue) { 1480 myExample = theValue; 1481 return this; 1482 } 1483 1484 1485 1486 1487 /** 1488 * Gets the value(s) for <b>minValue[x]</b> (). 1489 * creating it if it does 1490 * not exist. Will not return <code>null</code>. 1491 * 1492 * <p> 1493 * <b>Definition:</b> 1494 * The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity 1495 * </p> 1496 */ 1497 public IDatatype getMinValue() { 1498 return myMinValue; 1499 } 1500 1501 /** 1502 * Sets the value(s) for <b>minValue[x]</b> () 1503 * 1504 * <p> 1505 * <b>Definition:</b> 1506 * The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity 1507 * </p> 1508 */ 1509 public ElementDefinitionDt setMinValue(IDatatype theValue) { 1510 myMinValue = theValue; 1511 return this; 1512 } 1513 1514 1515 1516 1517 /** 1518 * Gets the value(s) for <b>maxValue[x]</b> (). 1519 * creating it if it does 1520 * not exist. Will not return <code>null</code>. 1521 * 1522 * <p> 1523 * <b>Definition:</b> 1524 * The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity 1525 * </p> 1526 */ 1527 public IDatatype getMaxValue() { 1528 return myMaxValue; 1529 } 1530 1531 /** 1532 * Sets the value(s) for <b>maxValue[x]</b> () 1533 * 1534 * <p> 1535 * <b>Definition:</b> 1536 * The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity 1537 * </p> 1538 */ 1539 public ElementDefinitionDt setMaxValue(IDatatype theValue) { 1540 myMaxValue = theValue; 1541 return this; 1542 } 1543 1544 1545 1546 1547 /** 1548 * Gets the value(s) for <b>maxLength</b> (). 1549 * creating it if it does 1550 * not exist. Will not return <code>null</code>. 1551 * 1552 * <p> 1553 * <b>Definition:</b> 1554 * Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element 1555 * </p> 1556 */ 1557 public IntegerDt getMaxLengthElement() { 1558 if (myMaxLength == null) { 1559 myMaxLength = new IntegerDt(); 1560 } 1561 return myMaxLength; 1562 } 1563 1564 1565 /** 1566 * Gets the value(s) for <b>maxLength</b> (). 1567 * creating it if it does 1568 * not exist. This method may return <code>null</code>. 1569 * 1570 * <p> 1571 * <b>Definition:</b> 1572 * Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element 1573 * </p> 1574 */ 1575 public Integer getMaxLength() { 1576 return getMaxLengthElement().getValue(); 1577 } 1578 1579 /** 1580 * Sets the value(s) for <b>maxLength</b> () 1581 * 1582 * <p> 1583 * <b>Definition:</b> 1584 * Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element 1585 * </p> 1586 */ 1587 public ElementDefinitionDt setMaxLength(IntegerDt theValue) { 1588 myMaxLength = theValue; 1589 return this; 1590 } 1591 1592 1593 1594 /** 1595 * Sets the value for <b>maxLength</b> () 1596 * 1597 * <p> 1598 * <b>Definition:</b> 1599 * Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element 1600 * </p> 1601 */ 1602 public ElementDefinitionDt setMaxLength( int theInteger) { 1603 myMaxLength = new IntegerDt(theInteger); 1604 return this; 1605 } 1606 1607 1608 /** 1609 * Gets the value(s) for <b>condition</b> (). 1610 * creating it if it does 1611 * not exist. Will not return <code>null</code>. 1612 * 1613 * <p> 1614 * <b>Definition:</b> 1615 * A reference to an invariant that may make additional statements about the cardinality or value in the instance 1616 * </p> 1617 */ 1618 public java.util.List<IdDt> getCondition() { 1619 if (myCondition == null) { 1620 myCondition = new java.util.ArrayList<IdDt>(); 1621 } 1622 return myCondition; 1623 } 1624 1625 /** 1626 * Sets the value(s) for <b>condition</b> () 1627 * 1628 * <p> 1629 * <b>Definition:</b> 1630 * A reference to an invariant that may make additional statements about the cardinality or value in the instance 1631 * </p> 1632 */ 1633 public ElementDefinitionDt setCondition(java.util.List<IdDt> theValue) { 1634 myCondition = theValue; 1635 return this; 1636 } 1637 1638 1639 1640 /** 1641 * Adds and returns a new value for <b>condition</b> () 1642 * 1643 * <p> 1644 * <b>Definition:</b> 1645 * A reference to an invariant that may make additional statements about the cardinality or value in the instance 1646 * </p> 1647 */ 1648 public IdDt addCondition() { 1649 IdDt newType = new IdDt(); 1650 getCondition().add(newType); 1651 return newType; 1652 } 1653 1654 /** 1655 * Adds a given new value for <b>condition</b> () 1656 * 1657 * <p> 1658 * <b>Definition:</b> 1659 * A reference to an invariant that may make additional statements about the cardinality or value in the instance 1660 * </p> 1661 * @param theValue The condition to add (must not be <code>null</code>) 1662 */ 1663 public ElementDefinitionDt addCondition(IdDt theValue) { 1664 if (theValue == null) { 1665 throw new NullPointerException("theValue must not be null"); 1666 } 1667 getCondition().add(theValue); 1668 return this; 1669 } 1670 1671 /** 1672 * Gets the first repetition for <b>condition</b> (), 1673 * creating it if it does not already exist. 1674 * 1675 * <p> 1676 * <b>Definition:</b> 1677 * A reference to an invariant that may make additional statements about the cardinality or value in the instance 1678 * </p> 1679 */ 1680 public IdDt getConditionFirstRep() { 1681 if (getCondition().isEmpty()) { 1682 return addCondition(); 1683 } 1684 return getCondition().get(0); 1685 } 1686 /** 1687 * Adds a new value for <b>condition</b> () 1688 * 1689 * <p> 1690 * <b>Definition:</b> 1691 * A reference to an invariant that may make additional statements about the cardinality or value in the instance 1692 * </p> 1693 * 1694 * @return Returns a reference to this object, to allow for simple chaining. 1695 */ 1696 public ElementDefinitionDt addCondition( String theId) { 1697 if (myCondition == null) { 1698 myCondition = new java.util.ArrayList<IdDt>(); 1699 } 1700 myCondition.add(new IdDt(theId)); 1701 return this; 1702 } 1703 1704 1705 /** 1706 * Gets the value(s) for <b>constraint</b> (). 1707 * creating it if it does 1708 * not exist. Will not return <code>null</code>. 1709 * 1710 * <p> 1711 * <b>Definition:</b> 1712 * Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance 1713 * </p> 1714 */ 1715 public java.util.List<Constraint> getConstraint() { 1716 if (myConstraint == null) { 1717 myConstraint = new java.util.ArrayList<Constraint>(); 1718 } 1719 return myConstraint; 1720 } 1721 1722 /** 1723 * Sets the value(s) for <b>constraint</b> () 1724 * 1725 * <p> 1726 * <b>Definition:</b> 1727 * Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance 1728 * </p> 1729 */ 1730 public ElementDefinitionDt setConstraint(java.util.List<Constraint> theValue) { 1731 myConstraint = theValue; 1732 return this; 1733 } 1734 1735 1736 1737 /** 1738 * Adds and returns a new value for <b>constraint</b> () 1739 * 1740 * <p> 1741 * <b>Definition:</b> 1742 * Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance 1743 * </p> 1744 */ 1745 public Constraint addConstraint() { 1746 Constraint newType = new Constraint(); 1747 getConstraint().add(newType); 1748 return newType; 1749 } 1750 1751 /** 1752 * Adds a given new value for <b>constraint</b> () 1753 * 1754 * <p> 1755 * <b>Definition:</b> 1756 * Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance 1757 * </p> 1758 * @param theValue The constraint to add (must not be <code>null</code>) 1759 */ 1760 public ElementDefinitionDt addConstraint(Constraint theValue) { 1761 if (theValue == null) { 1762 throw new NullPointerException("theValue must not be null"); 1763 } 1764 getConstraint().add(theValue); 1765 return this; 1766 } 1767 1768 /** 1769 * Gets the first repetition for <b>constraint</b> (), 1770 * creating it if it does not already exist. 1771 * 1772 * <p> 1773 * <b>Definition:</b> 1774 * Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance 1775 * </p> 1776 */ 1777 public Constraint getConstraintFirstRep() { 1778 if (getConstraint().isEmpty()) { 1779 return addConstraint(); 1780 } 1781 return getConstraint().get(0); 1782 } 1783 1784 /** 1785 * Gets the value(s) for <b>mustSupport</b> (). 1786 * creating it if it does 1787 * not exist. Will not return <code>null</code>. 1788 * 1789 * <p> 1790 * <b>Definition:</b> 1791 * If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported 1792 * </p> 1793 */ 1794 public BooleanDt getMustSupportElement() { 1795 if (myMustSupport == null) { 1796 myMustSupport = new BooleanDt(); 1797 } 1798 return myMustSupport; 1799 } 1800 1801 1802 /** 1803 * Gets the value(s) for <b>mustSupport</b> (). 1804 * creating it if it does 1805 * not exist. This method may return <code>null</code>. 1806 * 1807 * <p> 1808 * <b>Definition:</b> 1809 * If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported 1810 * </p> 1811 */ 1812 public Boolean getMustSupport() { 1813 return getMustSupportElement().getValue(); 1814 } 1815 1816 /** 1817 * Sets the value(s) for <b>mustSupport</b> () 1818 * 1819 * <p> 1820 * <b>Definition:</b> 1821 * If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported 1822 * </p> 1823 */ 1824 public ElementDefinitionDt setMustSupport(BooleanDt theValue) { 1825 myMustSupport = theValue; 1826 return this; 1827 } 1828 1829 1830 1831 /** 1832 * Sets the value for <b>mustSupport</b> () 1833 * 1834 * <p> 1835 * <b>Definition:</b> 1836 * If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported 1837 * </p> 1838 */ 1839 public ElementDefinitionDt setMustSupport( boolean theBoolean) { 1840 myMustSupport = new BooleanDt(theBoolean); 1841 return this; 1842 } 1843 1844 1845 /** 1846 * Gets the value(s) for <b>isModifier</b> (). 1847 * creating it if it does 1848 * not exist. Will not return <code>null</code>. 1849 * 1850 * <p> 1851 * <b>Definition:</b> 1852 * If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. 1853 * </p> 1854 */ 1855 public BooleanDt getIsModifierElement() { 1856 if (myIsModifier == null) { 1857 myIsModifier = new BooleanDt(); 1858 } 1859 return myIsModifier; 1860 } 1861 1862 1863 /** 1864 * Gets the value(s) for <b>isModifier</b> (). 1865 * creating it if it does 1866 * not exist. This method may return <code>null</code>. 1867 * 1868 * <p> 1869 * <b>Definition:</b> 1870 * If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. 1871 * </p> 1872 */ 1873 public Boolean getIsModifier() { 1874 return getIsModifierElement().getValue(); 1875 } 1876 1877 /** 1878 * Sets the value(s) for <b>isModifier</b> () 1879 * 1880 * <p> 1881 * <b>Definition:</b> 1882 * If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. 1883 * </p> 1884 */ 1885 public ElementDefinitionDt setIsModifier(BooleanDt theValue) { 1886 myIsModifier = theValue; 1887 return this; 1888 } 1889 1890 1891 1892 /** 1893 * Sets the value for <b>isModifier</b> () 1894 * 1895 * <p> 1896 * <b>Definition:</b> 1897 * If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. 1898 * </p> 1899 */ 1900 public ElementDefinitionDt setIsModifier( boolean theBoolean) { 1901 myIsModifier = new BooleanDt(theBoolean); 1902 return this; 1903 } 1904 1905 1906 /** 1907 * Gets the value(s) for <b>isSummary</b> (). 1908 * creating it if it does 1909 * not exist. Will not return <code>null</code>. 1910 * 1911 * <p> 1912 * <b>Definition:</b> 1913 * Whether the element should be included if a client requests a search with the parameter _summary=true 1914 * </p> 1915 */ 1916 public BooleanDt getIsSummaryElement() { 1917 if (myIsSummary == null) { 1918 myIsSummary = new BooleanDt(); 1919 } 1920 return myIsSummary; 1921 } 1922 1923 1924 /** 1925 * Gets the value(s) for <b>isSummary</b> (). 1926 * creating it if it does 1927 * not exist. This method may return <code>null</code>. 1928 * 1929 * <p> 1930 * <b>Definition:</b> 1931 * Whether the element should be included if a client requests a search with the parameter _summary=true 1932 * </p> 1933 */ 1934 public Boolean getIsSummary() { 1935 return getIsSummaryElement().getValue(); 1936 } 1937 1938 /** 1939 * Sets the value(s) for <b>isSummary</b> () 1940 * 1941 * <p> 1942 * <b>Definition:</b> 1943 * Whether the element should be included if a client requests a search with the parameter _summary=true 1944 * </p> 1945 */ 1946 public ElementDefinitionDt setIsSummary(BooleanDt theValue) { 1947 myIsSummary = theValue; 1948 return this; 1949 } 1950 1951 1952 1953 /** 1954 * Sets the value for <b>isSummary</b> () 1955 * 1956 * <p> 1957 * <b>Definition:</b> 1958 * Whether the element should be included if a client requests a search with the parameter _summary=true 1959 * </p> 1960 */ 1961 public ElementDefinitionDt setIsSummary( boolean theBoolean) { 1962 myIsSummary = new BooleanDt(theBoolean); 1963 return this; 1964 } 1965 1966 1967 /** 1968 * Gets the value(s) for <b>binding</b> (). 1969 * creating it if it does 1970 * not exist. Will not return <code>null</code>. 1971 * 1972 * <p> 1973 * <b>Definition:</b> 1974 * Binds to a value set if this element is coded (code, Coding, CodeableConcept) 1975 * </p> 1976 */ 1977 public Binding getBinding() { 1978 if (myBinding == null) { 1979 myBinding = new Binding(); 1980 } 1981 return myBinding; 1982 } 1983 1984 /** 1985 * Sets the value(s) for <b>binding</b> () 1986 * 1987 * <p> 1988 * <b>Definition:</b> 1989 * Binds to a value set if this element is coded (code, Coding, CodeableConcept) 1990 * </p> 1991 */ 1992 public ElementDefinitionDt setBinding(Binding theValue) { 1993 myBinding = theValue; 1994 return this; 1995 } 1996 1997 1998 1999 2000 /** 2001 * Gets the value(s) for <b>mapping</b> (). 2002 * creating it if it does 2003 * not exist. Will not return <code>null</code>. 2004 * 2005 * <p> 2006 * <b>Definition:</b> 2007 * Identifies a concept from an external specification that roughly corresponds to this element 2008 * </p> 2009 */ 2010 public java.util.List<Mapping> getMapping() { 2011 if (myMapping == null) { 2012 myMapping = new java.util.ArrayList<Mapping>(); 2013 } 2014 return myMapping; 2015 } 2016 2017 /** 2018 * Sets the value(s) for <b>mapping</b> () 2019 * 2020 * <p> 2021 * <b>Definition:</b> 2022 * Identifies a concept from an external specification that roughly corresponds to this element 2023 * </p> 2024 */ 2025 public ElementDefinitionDt setMapping(java.util.List<Mapping> theValue) { 2026 myMapping = theValue; 2027 return this; 2028 } 2029 2030 2031 2032 /** 2033 * Adds and returns a new value for <b>mapping</b> () 2034 * 2035 * <p> 2036 * <b>Definition:</b> 2037 * Identifies a concept from an external specification that roughly corresponds to this element 2038 * </p> 2039 */ 2040 public Mapping addMapping() { 2041 Mapping newType = new Mapping(); 2042 getMapping().add(newType); 2043 return newType; 2044 } 2045 2046 /** 2047 * Adds a given new value for <b>mapping</b> () 2048 * 2049 * <p> 2050 * <b>Definition:</b> 2051 * Identifies a concept from an external specification that roughly corresponds to this element 2052 * </p> 2053 * @param theValue The mapping to add (must not be <code>null</code>) 2054 */ 2055 public ElementDefinitionDt addMapping(Mapping theValue) { 2056 if (theValue == null) { 2057 throw new NullPointerException("theValue must not be null"); 2058 } 2059 getMapping().add(theValue); 2060 return this; 2061 } 2062 2063 /** 2064 * Gets the first repetition for <b>mapping</b> (), 2065 * creating it if it does not already exist. 2066 * 2067 * <p> 2068 * <b>Definition:</b> 2069 * Identifies a concept from an external specification that roughly corresponds to this element 2070 * </p> 2071 */ 2072 public Mapping getMappingFirstRep() { 2073 if (getMapping().isEmpty()) { 2074 return addMapping(); 2075 } 2076 return getMapping().get(0); 2077 } 2078 2079 /** 2080 * Block class for child element: <b>ElementDefinition.slicing</b> () 2081 * 2082 * <p> 2083 * <b>Definition:</b> 2084 * Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set) 2085 * </p> 2086 */ 2087 @Block() 2088 public static class Slicing 2089 extends BaseIdentifiableElement 2090 implements IResourceBlock { 2091 2092 @Child(name="discriminator", type=StringDt.class, order=0, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 2093 @Description( 2094 shortDefinition="", 2095 formalDefinition="Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices" 2096 ) 2097 private java.util.List<StringDt> myDiscriminator; 2098 2099 @Child(name="description", type=StringDt.class, order=1, min=0, max=1, summary=true, modifier=false) 2100 @Description( 2101 shortDefinition="", 2102 formalDefinition="A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated" 2103 ) 2104 private StringDt myDescription; 2105 2106 @Child(name="ordered", type=BooleanDt.class, order=2, min=0, max=1, summary=true, modifier=false) 2107 @Description( 2108 shortDefinition="", 2109 formalDefinition="If the matching elements have to occur in the same order as defined in the profile" 2110 ) 2111 private BooleanDt myOrdered; 2112 2113 @Child(name="rules", type=CodeDt.class, order=3, min=1, max=1, summary=true, modifier=false) 2114 @Description( 2115 shortDefinition="", 2116 formalDefinition="Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end" 2117 ) 2118 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-slicing-rules") 2119 private BoundCodeDt<SlicingRulesEnum> myRules; 2120 2121 2122 @Override 2123 public boolean isEmpty() { 2124 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myDiscriminator, myDescription, myOrdered, myRules); 2125 } 2126 2127 @Override 2128 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 2129 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myDiscriminator, myDescription, myOrdered, myRules); 2130 } 2131 2132 /** 2133 * Gets the value(s) for <b>discriminator</b> (). 2134 * creating it if it does 2135 * not exist. Will not return <code>null</code>. 2136 * 2137 * <p> 2138 * <b>Definition:</b> 2139 * Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices 2140 * </p> 2141 */ 2142 public java.util.List<StringDt> getDiscriminator() { 2143 if (myDiscriminator == null) { 2144 myDiscriminator = new java.util.ArrayList<StringDt>(); 2145 } 2146 return myDiscriminator; 2147 } 2148 2149 /** 2150 * Sets the value(s) for <b>discriminator</b> () 2151 * 2152 * <p> 2153 * <b>Definition:</b> 2154 * Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices 2155 * </p> 2156 */ 2157 public Slicing setDiscriminator(java.util.List<StringDt> theValue) { 2158 myDiscriminator = theValue; 2159 return this; 2160 } 2161 2162 2163 2164 /** 2165 * Adds and returns a new value for <b>discriminator</b> () 2166 * 2167 * <p> 2168 * <b>Definition:</b> 2169 * Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices 2170 * </p> 2171 */ 2172 public StringDt addDiscriminator() { 2173 StringDt newType = new StringDt(); 2174 getDiscriminator().add(newType); 2175 return newType; 2176 } 2177 2178 /** 2179 * Adds a given new value for <b>discriminator</b> () 2180 * 2181 * <p> 2182 * <b>Definition:</b> 2183 * Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices 2184 * </p> 2185 * @param theValue The discriminator to add (must not be <code>null</code>) 2186 */ 2187 public Slicing addDiscriminator(StringDt theValue) { 2188 if (theValue == null) { 2189 throw new NullPointerException("theValue must not be null"); 2190 } 2191 getDiscriminator().add(theValue); 2192 return this; 2193 } 2194 2195 /** 2196 * Gets the first repetition for <b>discriminator</b> (), 2197 * creating it if it does not already exist. 2198 * 2199 * <p> 2200 * <b>Definition:</b> 2201 * Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices 2202 * </p> 2203 */ 2204 public StringDt getDiscriminatorFirstRep() { 2205 if (getDiscriminator().isEmpty()) { 2206 return addDiscriminator(); 2207 } 2208 return getDiscriminator().get(0); 2209 } 2210 /** 2211 * Adds a new value for <b>discriminator</b> () 2212 * 2213 * <p> 2214 * <b>Definition:</b> 2215 * Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices 2216 * </p> 2217 * 2218 * @return Returns a reference to this object, to allow for simple chaining. 2219 */ 2220 public Slicing addDiscriminator( String theString) { 2221 if (myDiscriminator == null) { 2222 myDiscriminator = new java.util.ArrayList<StringDt>(); 2223 } 2224 myDiscriminator.add(new StringDt(theString)); 2225 return this; 2226 } 2227 2228 2229 /** 2230 * Gets the value(s) for <b>description</b> (). 2231 * creating it if it does 2232 * not exist. Will not return <code>null</code>. 2233 * 2234 * <p> 2235 * <b>Definition:</b> 2236 * A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated 2237 * </p> 2238 */ 2239 public StringDt getDescriptionElement() { 2240 if (myDescription == null) { 2241 myDescription = new StringDt(); 2242 } 2243 return myDescription; 2244 } 2245 2246 2247 /** 2248 * Gets the value(s) for <b>description</b> (). 2249 * creating it if it does 2250 * not exist. This method may return <code>null</code>. 2251 * 2252 * <p> 2253 * <b>Definition:</b> 2254 * A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated 2255 * </p> 2256 */ 2257 public String getDescription() { 2258 return getDescriptionElement().getValue(); 2259 } 2260 2261 /** 2262 * Sets the value(s) for <b>description</b> () 2263 * 2264 * <p> 2265 * <b>Definition:</b> 2266 * A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated 2267 * </p> 2268 */ 2269 public Slicing setDescription(StringDt theValue) { 2270 myDescription = theValue; 2271 return this; 2272 } 2273 2274 2275 2276 /** 2277 * Sets the value for <b>description</b> () 2278 * 2279 * <p> 2280 * <b>Definition:</b> 2281 * A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated 2282 * </p> 2283 */ 2284 public Slicing setDescription( String theString) { 2285 myDescription = new StringDt(theString); 2286 return this; 2287 } 2288 2289 2290 /** 2291 * Gets the value(s) for <b>ordered</b> (). 2292 * creating it if it does 2293 * not exist. Will not return <code>null</code>. 2294 * 2295 * <p> 2296 * <b>Definition:</b> 2297 * If the matching elements have to occur in the same order as defined in the profile 2298 * </p> 2299 */ 2300 public BooleanDt getOrderedElement() { 2301 if (myOrdered == null) { 2302 myOrdered = new BooleanDt(); 2303 } 2304 return myOrdered; 2305 } 2306 2307 2308 /** 2309 * Gets the value(s) for <b>ordered</b> (). 2310 * creating it if it does 2311 * not exist. This method may return <code>null</code>. 2312 * 2313 * <p> 2314 * <b>Definition:</b> 2315 * If the matching elements have to occur in the same order as defined in the profile 2316 * </p> 2317 */ 2318 public Boolean getOrdered() { 2319 return getOrderedElement().getValue(); 2320 } 2321 2322 /** 2323 * Sets the value(s) for <b>ordered</b> () 2324 * 2325 * <p> 2326 * <b>Definition:</b> 2327 * If the matching elements have to occur in the same order as defined in the profile 2328 * </p> 2329 */ 2330 public Slicing setOrdered(BooleanDt theValue) { 2331 myOrdered = theValue; 2332 return this; 2333 } 2334 2335 2336 2337 /** 2338 * Sets the value for <b>ordered</b> () 2339 * 2340 * <p> 2341 * <b>Definition:</b> 2342 * If the matching elements have to occur in the same order as defined in the profile 2343 * </p> 2344 */ 2345 public Slicing setOrdered( boolean theBoolean) { 2346 myOrdered = new BooleanDt(theBoolean); 2347 return this; 2348 } 2349 2350 2351 /** 2352 * Gets the value(s) for <b>rules</b> (). 2353 * creating it if it does 2354 * not exist. Will not return <code>null</code>. 2355 * 2356 * <p> 2357 * <b>Definition:</b> 2358 * Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end 2359 * </p> 2360 */ 2361 public BoundCodeDt<SlicingRulesEnum> getRulesElement() { 2362 if (myRules == null) { 2363 myRules = new BoundCodeDt<SlicingRulesEnum>(SlicingRulesEnum.VALUESET_BINDER); 2364 } 2365 return myRules; 2366 } 2367 2368 2369 /** 2370 * Gets the value(s) for <b>rules</b> (). 2371 * creating it if it does 2372 * not exist. This method may return <code>null</code>. 2373 * 2374 * <p> 2375 * <b>Definition:</b> 2376 * Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end 2377 * </p> 2378 */ 2379 public String getRules() { 2380 return getRulesElement().getValue(); 2381 } 2382 2383 /** 2384 * Sets the value(s) for <b>rules</b> () 2385 * 2386 * <p> 2387 * <b>Definition:</b> 2388 * Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end 2389 * </p> 2390 */ 2391 public Slicing setRules(BoundCodeDt<SlicingRulesEnum> theValue) { 2392 myRules = theValue; 2393 return this; 2394 } 2395 2396 2397 2398 /** 2399 * Sets the value(s) for <b>rules</b> () 2400 * 2401 * <p> 2402 * <b>Definition:</b> 2403 * Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end 2404 * </p> 2405 */ 2406 public Slicing setRules(SlicingRulesEnum theValue) { 2407 setRules(new BoundCodeDt<SlicingRulesEnum>(SlicingRulesEnum.VALUESET_BINDER, theValue)); 2408 2409/* 2410 getRulesElement().setValueAsEnum(theValue); 2411*/ 2412 return this; 2413 } 2414 2415 2416 2417 2418 } 2419 2420 2421 /** 2422 * Block class for child element: <b>ElementDefinition.base</b> () 2423 * 2424 * <p> 2425 * <b>Definition:</b> 2426 * Information about the base definition of the element, provided to make it unncessary for tools to trace the deviation of the element through the derived and related profiles. This information is only provided where the element definition represents a constraint on another element definition, and must be present if there is a base element definition. 2427 * </p> 2428 */ 2429 @Block() 2430 public static class Base 2431 extends BaseIdentifiableElement 2432 implements IResourceBlock { 2433 2434 @Child(name="path", type=StringDt.class, order=0, min=1, max=1, summary=true, modifier=false) 2435 @Description( 2436 shortDefinition="", 2437 formalDefinition="The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base" 2438 ) 2439 private StringDt myPath; 2440 2441 @Child(name="min", type=IntegerDt.class, order=1, min=1, max=1, summary=true, modifier=false) 2442 @Description( 2443 shortDefinition="", 2444 formalDefinition="Minimum cardinality of the base element identified by the path" 2445 ) 2446 private IntegerDt myMin; 2447 2448 @Child(name="max", type=StringDt.class, order=2, min=1, max=1, summary=true, modifier=false) 2449 @Description( 2450 shortDefinition="", 2451 formalDefinition="Maximum cardinality of the base element identified by the path" 2452 ) 2453 private StringDt myMax; 2454 2455 2456 @Override 2457 public boolean isEmpty() { 2458 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myPath, myMin, myMax); 2459 } 2460 2461 @Override 2462 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 2463 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myPath, myMin, myMax); 2464 } 2465 2466 /** 2467 * Gets the value(s) for <b>path</b> (). 2468 * creating it if it does 2469 * not exist. Will not return <code>null</code>. 2470 * 2471 * <p> 2472 * <b>Definition:</b> 2473 * The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base 2474 * </p> 2475 */ 2476 public StringDt getPathElement() { 2477 if (myPath == null) { 2478 myPath = new StringDt(); 2479 } 2480 return myPath; 2481 } 2482 2483 2484 /** 2485 * Gets the value(s) for <b>path</b> (). 2486 * creating it if it does 2487 * not exist. This method may return <code>null</code>. 2488 * 2489 * <p> 2490 * <b>Definition:</b> 2491 * The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base 2492 * </p> 2493 */ 2494 public String getPath() { 2495 return getPathElement().getValue(); 2496 } 2497 2498 /** 2499 * Sets the value(s) for <b>path</b> () 2500 * 2501 * <p> 2502 * <b>Definition:</b> 2503 * The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base 2504 * </p> 2505 */ 2506 public Base setPath(StringDt theValue) { 2507 myPath = theValue; 2508 return this; 2509 } 2510 2511 2512 2513 /** 2514 * Sets the value for <b>path</b> () 2515 * 2516 * <p> 2517 * <b>Definition:</b> 2518 * The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base 2519 * </p> 2520 */ 2521 public Base setPath( String theString) { 2522 myPath = new StringDt(theString); 2523 return this; 2524 } 2525 2526 2527 /** 2528 * Gets the value(s) for <b>min</b> (). 2529 * creating it if it does 2530 * not exist. Will not return <code>null</code>. 2531 * 2532 * <p> 2533 * <b>Definition:</b> 2534 * Minimum cardinality of the base element identified by the path 2535 * </p> 2536 */ 2537 public IntegerDt getMinElement() { 2538 if (myMin == null) { 2539 myMin = new IntegerDt(); 2540 } 2541 return myMin; 2542 } 2543 2544 2545 /** 2546 * Gets the value(s) for <b>min</b> (). 2547 * creating it if it does 2548 * not exist. This method may return <code>null</code>. 2549 * 2550 * <p> 2551 * <b>Definition:</b> 2552 * Minimum cardinality of the base element identified by the path 2553 * </p> 2554 */ 2555 public Integer getMin() { 2556 return getMinElement().getValue(); 2557 } 2558 2559 /** 2560 * Sets the value(s) for <b>min</b> () 2561 * 2562 * <p> 2563 * <b>Definition:</b> 2564 * Minimum cardinality of the base element identified by the path 2565 * </p> 2566 */ 2567 public Base setMin(IntegerDt theValue) { 2568 myMin = theValue; 2569 return this; 2570 } 2571 2572 2573 2574 /** 2575 * Sets the value for <b>min</b> () 2576 * 2577 * <p> 2578 * <b>Definition:</b> 2579 * Minimum cardinality of the base element identified by the path 2580 * </p> 2581 */ 2582 public Base setMin( int theInteger) { 2583 myMin = new IntegerDt(theInteger); 2584 return this; 2585 } 2586 2587 2588 /** 2589 * Gets the value(s) for <b>max</b> (). 2590 * creating it if it does 2591 * not exist. Will not return <code>null</code>. 2592 * 2593 * <p> 2594 * <b>Definition:</b> 2595 * Maximum cardinality of the base element identified by the path 2596 * </p> 2597 */ 2598 public StringDt getMaxElement() { 2599 if (myMax == null) { 2600 myMax = new StringDt(); 2601 } 2602 return myMax; 2603 } 2604 2605 2606 /** 2607 * Gets the value(s) for <b>max</b> (). 2608 * creating it if it does 2609 * not exist. This method may return <code>null</code>. 2610 * 2611 * <p> 2612 * <b>Definition:</b> 2613 * Maximum cardinality of the base element identified by the path 2614 * </p> 2615 */ 2616 public String getMax() { 2617 return getMaxElement().getValue(); 2618 } 2619 2620 /** 2621 * Sets the value(s) for <b>max</b> () 2622 * 2623 * <p> 2624 * <b>Definition:</b> 2625 * Maximum cardinality of the base element identified by the path 2626 * </p> 2627 */ 2628 public Base setMax(StringDt theValue) { 2629 myMax = theValue; 2630 return this; 2631 } 2632 2633 2634 2635 /** 2636 * Sets the value for <b>max</b> () 2637 * 2638 * <p> 2639 * <b>Definition:</b> 2640 * Maximum cardinality of the base element identified by the path 2641 * </p> 2642 */ 2643 public Base setMax( String theString) { 2644 myMax = new StringDt(theString); 2645 return this; 2646 } 2647 2648 2649 2650 2651 } 2652 2653 2654 /** 2655 * Block class for child element: <b>ElementDefinition.type</b> () 2656 * 2657 * <p> 2658 * <b>Definition:</b> 2659 * The data type or resource that the value of this element is permitted to be 2660 * </p> 2661 */ 2662 @Block() 2663 public static class Type 2664 extends BaseIdentifiableElement 2665 implements IResourceBlock { 2666 2667 @Child(name="code", type=CodeDt.class, order=0, min=1, max=1, summary=true, modifier=false) 2668 @Description( 2669 shortDefinition="", 2670 formalDefinition="Name of Data type or Resource that is a(or the) type used for this element" 2671 ) 2672 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/defined-types") 2673 private CodeDt myCode; 2674 2675 @Child(name="profile", type=UriDt.class, order=1, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 2676 @Description( 2677 shortDefinition="", 2678 formalDefinition="Identifies a profile structure or implementation Guide that SHALL hold for resources or datatypes referenced as the type of this element. Can be a local reference - to another structure in this profile, or a reference to a structure in another profile. When more than one profile is specified, the content must conform to all of them. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide" 2679 ) 2680 private java.util.List<UriDt> myProfile; 2681 2682 @Child(name="aggregation", type=CodeDt.class, order=2, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 2683 @Description( 2684 shortDefinition="", 2685 formalDefinition="If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle." 2686 ) 2687 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-aggregation-mode") 2688 private java.util.List<BoundCodeDt<AggregationModeEnum>> myAggregation; 2689 2690 2691 @Override 2692 public boolean isEmpty() { 2693 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myCode, myProfile, myAggregation); 2694 } 2695 2696 @Override 2697 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 2698 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myCode, myProfile, myAggregation); 2699 } 2700 2701 /** 2702 * Gets the value(s) for <b>code</b> (). 2703 * creating it if it does 2704 * not exist. Will not return <code>null</code>. 2705 * 2706 * <p> 2707 * <b>Definition:</b> 2708 * Name of Data type or Resource that is a(or the) type used for this element 2709 * </p> 2710 */ 2711 public CodeDt getCodeElement() { 2712 if (myCode == null) { 2713 myCode = new CodeDt(); 2714 } 2715 return myCode; 2716 } 2717 2718 2719 /** 2720 * Gets the value(s) for <b>code</b> (). 2721 * creating it if it does 2722 * not exist. This method may return <code>null</code>. 2723 * 2724 * <p> 2725 * <b>Definition:</b> 2726 * Name of Data type or Resource that is a(or the) type used for this element 2727 * </p> 2728 */ 2729 public String getCode() { 2730 return getCodeElement().getValue(); 2731 } 2732 2733 /** 2734 * Sets the value(s) for <b>code</b> () 2735 * 2736 * <p> 2737 * <b>Definition:</b> 2738 * Name of Data type or Resource that is a(or the) type used for this element 2739 * </p> 2740 */ 2741 public Type setCode(CodeDt theValue) { 2742 myCode = theValue; 2743 return this; 2744 } 2745 2746 2747 2748 /** 2749 * Sets the value for <b>code</b> () 2750 * 2751 * <p> 2752 * <b>Definition:</b> 2753 * Name of Data type or Resource that is a(or the) type used for this element 2754 * </p> 2755 */ 2756 public Type setCode( String theCode) { 2757 myCode = new CodeDt(theCode); 2758 return this; 2759 } 2760 2761 2762 /** 2763 * Gets the value(s) for <b>profile</b> (). 2764 * creating it if it does 2765 * not exist. Will not return <code>null</code>. 2766 * 2767 * <p> 2768 * <b>Definition:</b> 2769 * Identifies a profile structure or implementation Guide that SHALL hold for resources or datatypes referenced as the type of this element. Can be a local reference - to another structure in this profile, or a reference to a structure in another profile. When more than one profile is specified, the content must conform to all of them. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide 2770 * </p> 2771 */ 2772 public java.util.List<UriDt> getProfile() { 2773 if (myProfile == null) { 2774 myProfile = new java.util.ArrayList<UriDt>(); 2775 } 2776 return myProfile; 2777 } 2778 2779 /** 2780 * Sets the value(s) for <b>profile</b> () 2781 * 2782 * <p> 2783 * <b>Definition:</b> 2784 * Identifies a profile structure or implementation Guide that SHALL hold for resources or datatypes referenced as the type of this element. Can be a local reference - to another structure in this profile, or a reference to a structure in another profile. When more than one profile is specified, the content must conform to all of them. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide 2785 * </p> 2786 */ 2787 public Type setProfile(java.util.List<UriDt> theValue) { 2788 myProfile = theValue; 2789 return this; 2790 } 2791 2792 2793 2794 /** 2795 * Adds and returns a new value for <b>profile</b> () 2796 * 2797 * <p> 2798 * <b>Definition:</b> 2799 * Identifies a profile structure or implementation Guide that SHALL hold for resources or datatypes referenced as the type of this element. Can be a local reference - to another structure in this profile, or a reference to a structure in another profile. When more than one profile is specified, the content must conform to all of them. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide 2800 * </p> 2801 */ 2802 public UriDt addProfile() { 2803 UriDt newType = new UriDt(); 2804 getProfile().add(newType); 2805 return newType; 2806 } 2807 2808 /** 2809 * Adds a given new value for <b>profile</b> () 2810 * 2811 * <p> 2812 * <b>Definition:</b> 2813 * Identifies a profile structure or implementation Guide that SHALL hold for resources or datatypes referenced as the type of this element. Can be a local reference - to another structure in this profile, or a reference to a structure in another profile. When more than one profile is specified, the content must conform to all of them. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide 2814 * </p> 2815 * @param theValue The profile to add (must not be <code>null</code>) 2816 */ 2817 public Type addProfile(UriDt theValue) { 2818 if (theValue == null) { 2819 throw new NullPointerException("theValue must not be null"); 2820 } 2821 getProfile().add(theValue); 2822 return this; 2823 } 2824 2825 /** 2826 * Gets the first repetition for <b>profile</b> (), 2827 * creating it if it does not already exist. 2828 * 2829 * <p> 2830 * <b>Definition:</b> 2831 * Identifies a profile structure or implementation Guide that SHALL hold for resources or datatypes referenced as the type of this element. Can be a local reference - to another structure in this profile, or a reference to a structure in another profile. When more than one profile is specified, the content must conform to all of them. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide 2832 * </p> 2833 */ 2834 public UriDt getProfileFirstRep() { 2835 if (getProfile().isEmpty()) { 2836 return addProfile(); 2837 } 2838 return getProfile().get(0); 2839 } 2840 /** 2841 * Adds a new value for <b>profile</b> () 2842 * 2843 * <p> 2844 * <b>Definition:</b> 2845 * Identifies a profile structure or implementation Guide that SHALL hold for resources or datatypes referenced as the type of this element. Can be a local reference - to another structure in this profile, or a reference to a structure in another profile. When more than one profile is specified, the content must conform to all of them. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide 2846 * </p> 2847 * 2848 * @return Returns a reference to this object, to allow for simple chaining. 2849 */ 2850 public Type addProfile( String theUri) { 2851 if (myProfile == null) { 2852 myProfile = new java.util.ArrayList<UriDt>(); 2853 } 2854 myProfile.add(new UriDt(theUri)); 2855 return this; 2856 } 2857 2858 2859 /** 2860 * Gets the value(s) for <b>aggregation</b> (). 2861 * creating it if it does 2862 * not exist. Will not return <code>null</code>. 2863 * 2864 * <p> 2865 * <b>Definition:</b> 2866 * If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle. 2867 * </p> 2868 */ 2869 public java.util.List<BoundCodeDt<AggregationModeEnum>> getAggregation() { 2870 if (myAggregation == null) { 2871 myAggregation = new java.util.ArrayList<BoundCodeDt<AggregationModeEnum>>(); 2872 } 2873 return myAggregation; 2874 } 2875 2876 /** 2877 * Sets the value(s) for <b>aggregation</b> () 2878 * 2879 * <p> 2880 * <b>Definition:</b> 2881 * If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle. 2882 * </p> 2883 */ 2884 public Type setAggregation(java.util.List<BoundCodeDt<AggregationModeEnum>> theValue) { 2885 myAggregation = theValue; 2886 return this; 2887 } 2888 2889 2890 2891 /** 2892 * Add a value for <b>aggregation</b> () using an enumerated type. This 2893 * is intended as a convenience method for situations where the FHIR defined ValueSets are mandatory 2894 * or contain the desirable codes. If you wish to use codes other than those which are built-in, 2895 * you may also use the {@link #addAggregation()} method. 2896 * 2897 * <p> 2898 * <b>Definition:</b> 2899 * If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle. 2900 * </p> 2901 */ 2902 public BoundCodeDt<AggregationModeEnum> addAggregation(AggregationModeEnum theValue) { 2903 BoundCodeDt<AggregationModeEnum> retVal = new BoundCodeDt<AggregationModeEnum>(AggregationModeEnum.VALUESET_BINDER, theValue); 2904 getAggregation().add(retVal); 2905 return retVal; 2906 } 2907 2908 /** 2909 * Gets the first repetition for <b>aggregation</b> (), 2910 * creating it if it does not already exist. 2911 * 2912 * <p> 2913 * <b>Definition:</b> 2914 * If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle. 2915 * </p> 2916 */ 2917 public BoundCodeDt<AggregationModeEnum> getAggregationFirstRep() { 2918 if (getAggregation().size() == 0) { 2919 addAggregation(); 2920 } 2921 return getAggregation().get(0); 2922 } 2923 2924 /** 2925 * Add a value for <b>aggregation</b> () 2926 * 2927 * <p> 2928 * <b>Definition:</b> 2929 * If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle. 2930 * </p> 2931 */ 2932 public BoundCodeDt<AggregationModeEnum> addAggregation() { 2933 BoundCodeDt<AggregationModeEnum> retVal = new BoundCodeDt<AggregationModeEnum>(AggregationModeEnum.VALUESET_BINDER); 2934 getAggregation().add(retVal); 2935 return retVal; 2936 } 2937 2938 /** 2939 * Sets the value(s), and clears any existing value(s) for <b>aggregation</b> () 2940 * 2941 * <p> 2942 * <b>Definition:</b> 2943 * If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle. 2944 * </p> 2945 */ 2946 public Type setAggregation(AggregationModeEnum theValue) { 2947 getAggregation().clear(); 2948 addAggregation(theValue); 2949 return this; 2950 } 2951 2952 2953 2954 2955 } 2956 2957 2958 /** 2959 * Block class for child element: <b>ElementDefinition.constraint</b> () 2960 * 2961 * <p> 2962 * <b>Definition:</b> 2963 * Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance 2964 * </p> 2965 */ 2966 @Block() 2967 public static class Constraint 2968 extends BaseIdentifiableElement 2969 implements IResourceBlock { 2970 2971 @Child(name="key", type=IdDt.class, order=0, min=1, max=1, summary=true, modifier=false) 2972 @Description( 2973 shortDefinition="", 2974 formalDefinition="Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality" 2975 ) 2976 private IdDt myKey; 2977 2978 @Child(name="requirements", type=StringDt.class, order=1, min=0, max=1, summary=true, modifier=false) 2979 @Description( 2980 shortDefinition="", 2981 formalDefinition="Description of why this constraint is necessary or appropriate" 2982 ) 2983 private StringDt myRequirements; 2984 2985 @Child(name="severity", type=CodeDt.class, order=2, min=1, max=1, summary=true, modifier=false) 2986 @Description( 2987 shortDefinition="", 2988 formalDefinition="Identifies the impact constraint violation has on the conformance of the instance" 2989 ) 2990 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/constraint-severity") 2991 private BoundCodeDt<ConstraintSeverityEnum> mySeverity; 2992 2993 @Child(name="human", type=StringDt.class, order=3, min=1, max=1, summary=true, modifier=false) 2994 @Description( 2995 shortDefinition="", 2996 formalDefinition="Text that can be used to describe the constraint in messages identifying that the constraint has been violated" 2997 ) 2998 private StringDt myHuman; 2999 3000 @Child(name="xpath", type=StringDt.class, order=4, min=1, max=1, summary=true, modifier=false) 3001 @Description( 3002 shortDefinition="", 3003 formalDefinition="An XPath expression of constraint that can be executed to see if this constraint is met" 3004 ) 3005 private StringDt myXpath; 3006 3007 3008 @Override 3009 public boolean isEmpty() { 3010 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myKey, myRequirements, mySeverity, myHuman, myXpath); 3011 } 3012 3013 @Override 3014 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 3015 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myKey, myRequirements, mySeverity, myHuman, myXpath); 3016 } 3017 3018 /** 3019 * Gets the value(s) for <b>key</b> (). 3020 * creating it if it does 3021 * not exist. Will not return <code>null</code>. 3022 * 3023 * <p> 3024 * <b>Definition:</b> 3025 * Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality 3026 * </p> 3027 */ 3028 public IdDt getKeyElement() { 3029 if (myKey == null) { 3030 myKey = new IdDt(); 3031 } 3032 return myKey; 3033 } 3034 3035 3036 /** 3037 * Gets the value(s) for <b>key</b> (). 3038 * creating it if it does 3039 * not exist. This method may return <code>null</code>. 3040 * 3041 * <p> 3042 * <b>Definition:</b> 3043 * Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality 3044 * </p> 3045 */ 3046 public String getKey() { 3047 return getKeyElement().getValue(); 3048 } 3049 3050 /** 3051 * Sets the value(s) for <b>key</b> () 3052 * 3053 * <p> 3054 * <b>Definition:</b> 3055 * Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality 3056 * </p> 3057 */ 3058 public Constraint setKey(IdDt theValue) { 3059 myKey = theValue; 3060 return this; 3061 } 3062 3063 3064 3065 /** 3066 * Sets the value for <b>key</b> () 3067 * 3068 * <p> 3069 * <b>Definition:</b> 3070 * Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality 3071 * </p> 3072 */ 3073 public Constraint setKey( String theId) { 3074 myKey = new IdDt(theId); 3075 return this; 3076 } 3077 3078 3079 /** 3080 * Gets the value(s) for <b>requirements</b> (). 3081 * creating it if it does 3082 * not exist. Will not return <code>null</code>. 3083 * 3084 * <p> 3085 * <b>Definition:</b> 3086 * Description of why this constraint is necessary or appropriate 3087 * </p> 3088 */ 3089 public StringDt getRequirementsElement() { 3090 if (myRequirements == null) { 3091 myRequirements = new StringDt(); 3092 } 3093 return myRequirements; 3094 } 3095 3096 3097 /** 3098 * Gets the value(s) for <b>requirements</b> (). 3099 * creating it if it does 3100 * not exist. This method may return <code>null</code>. 3101 * 3102 * <p> 3103 * <b>Definition:</b> 3104 * Description of why this constraint is necessary or appropriate 3105 * </p> 3106 */ 3107 public String getRequirements() { 3108 return getRequirementsElement().getValue(); 3109 } 3110 3111 /** 3112 * Sets the value(s) for <b>requirements</b> () 3113 * 3114 * <p> 3115 * <b>Definition:</b> 3116 * Description of why this constraint is necessary or appropriate 3117 * </p> 3118 */ 3119 public Constraint setRequirements(StringDt theValue) { 3120 myRequirements = theValue; 3121 return this; 3122 } 3123 3124 3125 3126 /** 3127 * Sets the value for <b>requirements</b> () 3128 * 3129 * <p> 3130 * <b>Definition:</b> 3131 * Description of why this constraint is necessary or appropriate 3132 * </p> 3133 */ 3134 public Constraint setRequirements( String theString) { 3135 myRequirements = new StringDt(theString); 3136 return this; 3137 } 3138 3139 3140 /** 3141 * Gets the value(s) for <b>severity</b> (). 3142 * creating it if it does 3143 * not exist. Will not return <code>null</code>. 3144 * 3145 * <p> 3146 * <b>Definition:</b> 3147 * Identifies the impact constraint violation has on the conformance of the instance 3148 * </p> 3149 */ 3150 public BoundCodeDt<ConstraintSeverityEnum> getSeverityElement() { 3151 if (mySeverity == null) { 3152 mySeverity = new BoundCodeDt<ConstraintSeverityEnum>(ConstraintSeverityEnum.VALUESET_BINDER); 3153 } 3154 return mySeverity; 3155 } 3156 3157 3158 /** 3159 * Gets the value(s) for <b>severity</b> (). 3160 * creating it if it does 3161 * not exist. This method may return <code>null</code>. 3162 * 3163 * <p> 3164 * <b>Definition:</b> 3165 * Identifies the impact constraint violation has on the conformance of the instance 3166 * </p> 3167 */ 3168 public String getSeverity() { 3169 return getSeverityElement().getValue(); 3170 } 3171 3172 /** 3173 * Sets the value(s) for <b>severity</b> () 3174 * 3175 * <p> 3176 * <b>Definition:</b> 3177 * Identifies the impact constraint violation has on the conformance of the instance 3178 * </p> 3179 */ 3180 public Constraint setSeverity(BoundCodeDt<ConstraintSeverityEnum> theValue) { 3181 mySeverity = theValue; 3182 return this; 3183 } 3184 3185 3186 3187 /** 3188 * Sets the value(s) for <b>severity</b> () 3189 * 3190 * <p> 3191 * <b>Definition:</b> 3192 * Identifies the impact constraint violation has on the conformance of the instance 3193 * </p> 3194 */ 3195 public Constraint setSeverity(ConstraintSeverityEnum theValue) { 3196 setSeverity(new BoundCodeDt<ConstraintSeverityEnum>(ConstraintSeverityEnum.VALUESET_BINDER, theValue)); 3197 3198/* 3199 getSeverityElement().setValueAsEnum(theValue); 3200*/ 3201 return this; 3202 } 3203 3204 3205 /** 3206 * Gets the value(s) for <b>human</b> (). 3207 * creating it if it does 3208 * not exist. Will not return <code>null</code>. 3209 * 3210 * <p> 3211 * <b>Definition:</b> 3212 * Text that can be used to describe the constraint in messages identifying that the constraint has been violated 3213 * </p> 3214 */ 3215 public StringDt getHumanElement() { 3216 if (myHuman == null) { 3217 myHuman = new StringDt(); 3218 } 3219 return myHuman; 3220 } 3221 3222 3223 /** 3224 * Gets the value(s) for <b>human</b> (). 3225 * creating it if it does 3226 * not exist. This method may return <code>null</code>. 3227 * 3228 * <p> 3229 * <b>Definition:</b> 3230 * Text that can be used to describe the constraint in messages identifying that the constraint has been violated 3231 * </p> 3232 */ 3233 public String getHuman() { 3234 return getHumanElement().getValue(); 3235 } 3236 3237 /** 3238 * Sets the value(s) for <b>human</b> () 3239 * 3240 * <p> 3241 * <b>Definition:</b> 3242 * Text that can be used to describe the constraint in messages identifying that the constraint has been violated 3243 * </p> 3244 */ 3245 public Constraint setHuman(StringDt theValue) { 3246 myHuman = theValue; 3247 return this; 3248 } 3249 3250 3251 3252 /** 3253 * Sets the value for <b>human</b> () 3254 * 3255 * <p> 3256 * <b>Definition:</b> 3257 * Text that can be used to describe the constraint in messages identifying that the constraint has been violated 3258 * </p> 3259 */ 3260 public Constraint setHuman( String theString) { 3261 myHuman = new StringDt(theString); 3262 return this; 3263 } 3264 3265 3266 /** 3267 * Gets the value(s) for <b>xpath</b> (). 3268 * creating it if it does 3269 * not exist. Will not return <code>null</code>. 3270 * 3271 * <p> 3272 * <b>Definition:</b> 3273 * An XPath expression of constraint that can be executed to see if this constraint is met 3274 * </p> 3275 */ 3276 public StringDt getXpathElement() { 3277 if (myXpath == null) { 3278 myXpath = new StringDt(); 3279 } 3280 return myXpath; 3281 } 3282 3283 3284 /** 3285 * Gets the value(s) for <b>xpath</b> (). 3286 * creating it if it does 3287 * not exist. This method may return <code>null</code>. 3288 * 3289 * <p> 3290 * <b>Definition:</b> 3291 * An XPath expression of constraint that can be executed to see if this constraint is met 3292 * </p> 3293 */ 3294 public String getXpath() { 3295 return getXpathElement().getValue(); 3296 } 3297 3298 /** 3299 * Sets the value(s) for <b>xpath</b> () 3300 * 3301 * <p> 3302 * <b>Definition:</b> 3303 * An XPath expression of constraint that can be executed to see if this constraint is met 3304 * </p> 3305 */ 3306 public Constraint setXpath(StringDt theValue) { 3307 myXpath = theValue; 3308 return this; 3309 } 3310 3311 3312 3313 /** 3314 * Sets the value for <b>xpath</b> () 3315 * 3316 * <p> 3317 * <b>Definition:</b> 3318 * An XPath expression of constraint that can be executed to see if this constraint is met 3319 * </p> 3320 */ 3321 public Constraint setXpath( String theString) { 3322 myXpath = new StringDt(theString); 3323 return this; 3324 } 3325 3326 3327 3328 3329 } 3330 3331 3332 /** 3333 * Block class for child element: <b>ElementDefinition.binding</b> () 3334 * 3335 * <p> 3336 * <b>Definition:</b> 3337 * Binds to a value set if this element is coded (code, Coding, CodeableConcept) 3338 * </p> 3339 */ 3340 @Block() 3341 public static class Binding 3342 extends BaseIdentifiableElement 3343 implements IResourceBlock { 3344 3345 @Child(name="strength", type=CodeDt.class, order=0, min=1, max=1, summary=true, modifier=false) 3346 @Description( 3347 shortDefinition="", 3348 formalDefinition="Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances" 3349 ) 3350 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/binding-strength") 3351 private BoundCodeDt<BindingStrengthEnum> myStrength; 3352 3353 @Child(name="description", type=StringDt.class, order=1, min=0, max=1, summary=true, modifier=false) 3354 @Description( 3355 shortDefinition="", 3356 formalDefinition="Describes the intended use of this particular set of codes" 3357 ) 3358 private StringDt myDescription; 3359 3360 @Child(name="valueSet", order=2, min=0, max=1, summary=true, modifier=false, type={ 3361 UriDt.class, 3362 ValueSet.class 3363 }) 3364 @Description( 3365 shortDefinition="", 3366 formalDefinition="Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used" 3367 ) 3368 private IDatatype myValueSet; 3369 3370 3371 @Override 3372 public boolean isEmpty() { 3373 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myStrength, myDescription, myValueSet); 3374 } 3375 3376 @Override 3377 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 3378 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myStrength, myDescription, myValueSet); 3379 } 3380 3381 /** 3382 * Gets the value(s) for <b>strength</b> (). 3383 * creating it if it does 3384 * not exist. Will not return <code>null</code>. 3385 * 3386 * <p> 3387 * <b>Definition:</b> 3388 * Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances 3389 * </p> 3390 */ 3391 public BoundCodeDt<BindingStrengthEnum> getStrengthElement() { 3392 if (myStrength == null) { 3393 myStrength = new BoundCodeDt<BindingStrengthEnum>(BindingStrengthEnum.VALUESET_BINDER); 3394 } 3395 return myStrength; 3396 } 3397 3398 3399 /** 3400 * Gets the value(s) for <b>strength</b> (). 3401 * creating it if it does 3402 * not exist. This method may return <code>null</code>. 3403 * 3404 * <p> 3405 * <b>Definition:</b> 3406 * Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances 3407 * </p> 3408 */ 3409 public String getStrength() { 3410 return getStrengthElement().getValue(); 3411 } 3412 3413 /** 3414 * Sets the value(s) for <b>strength</b> () 3415 * 3416 * <p> 3417 * <b>Definition:</b> 3418 * Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances 3419 * </p> 3420 */ 3421 public Binding setStrength(BoundCodeDt<BindingStrengthEnum> theValue) { 3422 myStrength = theValue; 3423 return this; 3424 } 3425 3426 3427 3428 /** 3429 * Sets the value(s) for <b>strength</b> () 3430 * 3431 * <p> 3432 * <b>Definition:</b> 3433 * Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances 3434 * </p> 3435 */ 3436 public Binding setStrength(BindingStrengthEnum theValue) { 3437 setStrength(new BoundCodeDt<BindingStrengthEnum>(BindingStrengthEnum.VALUESET_BINDER, theValue)); 3438 3439/* 3440 getStrengthElement().setValueAsEnum(theValue); 3441*/ 3442 return this; 3443 } 3444 3445 3446 /** 3447 * Gets the value(s) for <b>description</b> (). 3448 * creating it if it does 3449 * not exist. Will not return <code>null</code>. 3450 * 3451 * <p> 3452 * <b>Definition:</b> 3453 * Describes the intended use of this particular set of codes 3454 * </p> 3455 */ 3456 public StringDt getDescriptionElement() { 3457 if (myDescription == null) { 3458 myDescription = new StringDt(); 3459 } 3460 return myDescription; 3461 } 3462 3463 3464 /** 3465 * Gets the value(s) for <b>description</b> (). 3466 * creating it if it does 3467 * not exist. This method may return <code>null</code>. 3468 * 3469 * <p> 3470 * <b>Definition:</b> 3471 * Describes the intended use of this particular set of codes 3472 * </p> 3473 */ 3474 public String getDescription() { 3475 return getDescriptionElement().getValue(); 3476 } 3477 3478 /** 3479 * Sets the value(s) for <b>description</b> () 3480 * 3481 * <p> 3482 * <b>Definition:</b> 3483 * Describes the intended use of this particular set of codes 3484 * </p> 3485 */ 3486 public Binding setDescription(StringDt theValue) { 3487 myDescription = theValue; 3488 return this; 3489 } 3490 3491 3492 3493 /** 3494 * Sets the value for <b>description</b> () 3495 * 3496 * <p> 3497 * <b>Definition:</b> 3498 * Describes the intended use of this particular set of codes 3499 * </p> 3500 */ 3501 public Binding setDescription( String theString) { 3502 myDescription = new StringDt(theString); 3503 return this; 3504 } 3505 3506 3507 /** 3508 * Gets the value(s) for <b>valueSet[x]</b> (). 3509 * creating it if it does 3510 * not exist. Will not return <code>null</code>. 3511 * 3512 * <p> 3513 * <b>Definition:</b> 3514 * Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used 3515 * </p> 3516 */ 3517 public IDatatype getValueSet() { 3518 return myValueSet; 3519 } 3520 3521 /** 3522 * Sets the value(s) for <b>valueSet[x]</b> () 3523 * 3524 * <p> 3525 * <b>Definition:</b> 3526 * Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used 3527 * </p> 3528 */ 3529 public Binding setValueSet(IDatatype theValue) { 3530 myValueSet = theValue; 3531 return this; 3532 } 3533 3534 3535 3536 3537 3538 3539 } 3540 3541 3542 /** 3543 * Block class for child element: <b>ElementDefinition.mapping</b> () 3544 * 3545 * <p> 3546 * <b>Definition:</b> 3547 * Identifies a concept from an external specification that roughly corresponds to this element 3548 * </p> 3549 */ 3550 @Block() 3551 public static class Mapping 3552 extends BaseIdentifiableElement 3553 implements IResourceBlock { 3554 3555 @Child(name="identity", type=IdDt.class, order=0, min=1, max=1, summary=true, modifier=false) 3556 @Description( 3557 shortDefinition="", 3558 formalDefinition="An internal reference to the definition of a mapping" 3559 ) 3560 private IdDt myIdentity; 3561 3562 @Child(name="language", type=CodeDt.class, order=1, min=0, max=1, summary=true, modifier=false) 3563 @Description( 3564 shortDefinition="", 3565 formalDefinition="Identifies the computable language in which mapping.map is expressed." 3566 ) 3567 private CodeDt myLanguage; 3568 3569 @Child(name="map", type=StringDt.class, order=2, min=1, max=1, summary=true, modifier=false) 3570 @Description( 3571 shortDefinition="", 3572 formalDefinition="Expresses what part of the target specification corresponds to this element" 3573 ) 3574 private StringDt myMap; 3575 3576 3577 @Override 3578 public boolean isEmpty() { 3579 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myIdentity, myLanguage, myMap); 3580 } 3581 3582 @Override 3583 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 3584 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myIdentity, myLanguage, myMap); 3585 } 3586 3587 /** 3588 * Gets the value(s) for <b>identity</b> (). 3589 * creating it if it does 3590 * not exist. Will not return <code>null</code>. 3591 * 3592 * <p> 3593 * <b>Definition:</b> 3594 * An internal reference to the definition of a mapping 3595 * </p> 3596 */ 3597 public IdDt getIdentityElement() { 3598 if (myIdentity == null) { 3599 myIdentity = new IdDt(); 3600 } 3601 return myIdentity; 3602 } 3603 3604 3605 /** 3606 * Gets the value(s) for <b>identity</b> (). 3607 * creating it if it does 3608 * not exist. This method may return <code>null</code>. 3609 * 3610 * <p> 3611 * <b>Definition:</b> 3612 * An internal reference to the definition of a mapping 3613 * </p> 3614 */ 3615 public String getIdentity() { 3616 return getIdentityElement().getValue(); 3617 } 3618 3619 /** 3620 * Sets the value(s) for <b>identity</b> () 3621 * 3622 * <p> 3623 * <b>Definition:</b> 3624 * An internal reference to the definition of a mapping 3625 * </p> 3626 */ 3627 public Mapping setIdentity(IdDt theValue) { 3628 myIdentity = theValue; 3629 return this; 3630 } 3631 3632 3633 3634 /** 3635 * Sets the value for <b>identity</b> () 3636 * 3637 * <p> 3638 * <b>Definition:</b> 3639 * An internal reference to the definition of a mapping 3640 * </p> 3641 */ 3642 public Mapping setIdentity( String theId) { 3643 myIdentity = new IdDt(theId); 3644 return this; 3645 } 3646 3647 3648 /** 3649 * Gets the value(s) for <b>language</b> (). 3650 * creating it if it does 3651 * not exist. Will not return <code>null</code>. 3652 * 3653 * <p> 3654 * <b>Definition:</b> 3655 * Identifies the computable language in which mapping.map is expressed. 3656 * </p> 3657 */ 3658 public CodeDt getLanguageElement() { 3659 if (myLanguage == null) { 3660 myLanguage = new CodeDt(); 3661 } 3662 return myLanguage; 3663 } 3664 3665 3666 /** 3667 * Gets the value(s) for <b>language</b> (). 3668 * creating it if it does 3669 * not exist. This method may return <code>null</code>. 3670 * 3671 * <p> 3672 * <b>Definition:</b> 3673 * Identifies the computable language in which mapping.map is expressed. 3674 * </p> 3675 */ 3676 public String getLanguage() { 3677 return getLanguageElement().getValue(); 3678 } 3679 3680 /** 3681 * Sets the value(s) for <b>language</b> () 3682 * 3683 * <p> 3684 * <b>Definition:</b> 3685 * Identifies the computable language in which mapping.map is expressed. 3686 * </p> 3687 */ 3688 public Mapping setLanguage(CodeDt theValue) { 3689 myLanguage = theValue; 3690 return this; 3691 } 3692 3693 3694 3695 /** 3696 * Sets the value for <b>language</b> () 3697 * 3698 * <p> 3699 * <b>Definition:</b> 3700 * Identifies the computable language in which mapping.map is expressed. 3701 * </p> 3702 */ 3703 public Mapping setLanguage( String theCode) { 3704 myLanguage = new CodeDt(theCode); 3705 return this; 3706 } 3707 3708 3709 /** 3710 * Gets the value(s) for <b>map</b> (). 3711 * creating it if it does 3712 * not exist. Will not return <code>null</code>. 3713 * 3714 * <p> 3715 * <b>Definition:</b> 3716 * Expresses what part of the target specification corresponds to this element 3717 * </p> 3718 */ 3719 public StringDt getMapElement() { 3720 if (myMap == null) { 3721 myMap = new StringDt(); 3722 } 3723 return myMap; 3724 } 3725 3726 3727 /** 3728 * Gets the value(s) for <b>map</b> (). 3729 * creating it if it does 3730 * not exist. This method may return <code>null</code>. 3731 * 3732 * <p> 3733 * <b>Definition:</b> 3734 * Expresses what part of the target specification corresponds to this element 3735 * </p> 3736 */ 3737 public String getMap() { 3738 return getMapElement().getValue(); 3739 } 3740 3741 /** 3742 * Sets the value(s) for <b>map</b> () 3743 * 3744 * <p> 3745 * <b>Definition:</b> 3746 * Expresses what part of the target specification corresponds to this element 3747 * </p> 3748 */ 3749 public Mapping setMap(StringDt theValue) { 3750 myMap = theValue; 3751 return this; 3752 } 3753 3754 3755 3756 /** 3757 * Sets the value for <b>map</b> () 3758 * 3759 * <p> 3760 * <b>Definition:</b> 3761 * Expresses what part of the target specification corresponds to this element 3762 * </p> 3763 */ 3764 public Mapping setMap( String theString) { 3765 myMap = new StringDt(theString); 3766 return this; 3767 } 3768 3769 3770 3771 3772 } 3773 3774 3775 3776 3777}