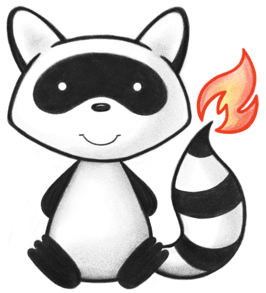
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>HumanNameDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * A human's name with the ability to identify parts and usage 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * Need to be able to record names, along with notes about their use 088 * </p> 089 */ 090@DatatypeDef(name="HumanName") 091public class HumanNameDt 092 extends BaseHumanNameDt 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public HumanNameDt() { 100 // nothing 101 } 102 103 104 @Child(name="use", type=CodeDt.class, order=0, min=0, max=1, summary=true, modifier=true) 105 @Description( 106 shortDefinition="", 107 formalDefinition="Identifies the purpose for this name" 108 ) 109 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/name-use") 110 private BoundCodeDt<NameUseEnum> myUse; 111 112 @Child(name="text", type=StringDt.class, order=1, min=0, max=1, summary=true, modifier=false) 113 @Description( 114 shortDefinition="", 115 formalDefinition="A full text representation of the name" 116 ) 117 private StringDt myText; 118 119 @Child(name="family", type=StringDt.class, order=2, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 120 @Description( 121 shortDefinition="", 122 formalDefinition="The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father." 123 ) 124 private java.util.List<StringDt> myFamily; 125 126 @Child(name="given", type=StringDt.class, order=3, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 127 @Description( 128 shortDefinition="", 129 formalDefinition="Given name" 130 ) 131 private java.util.List<StringDt> myGiven; 132 133 @Child(name="prefix", type=StringDt.class, order=4, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 134 @Description( 135 shortDefinition="", 136 formalDefinition="Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name" 137 ) 138 private java.util.List<StringDt> myPrefix; 139 140 @Child(name="suffix", type=StringDt.class, order=5, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 141 @Description( 142 shortDefinition="", 143 formalDefinition="Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name" 144 ) 145 private java.util.List<StringDt> mySuffix; 146 147 @Child(name="period", type=PeriodDt.class, order=6, min=0, max=1, summary=true, modifier=false) 148 @Description( 149 shortDefinition="", 150 formalDefinition="Indicates the period of time when this name was valid for the named person." 151 ) 152 private PeriodDt myPeriod; 153 154 155 @Override 156 public boolean isEmpty() { 157 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myUse, myText, myFamily, myGiven, myPrefix, mySuffix, myPeriod); 158 } 159 160 @Override 161 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 162 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myUse, myText, myFamily, myGiven, myPrefix, mySuffix, myPeriod); 163 } 164 165 /** 166 * Gets the value(s) for <b>use</b> (). 167 * creating it if it does 168 * not exist. Will not return <code>null</code>. 169 * 170 * <p> 171 * <b>Definition:</b> 172 * Identifies the purpose for this name 173 * </p> 174 */ 175 public BoundCodeDt<NameUseEnum> getUseElement() { 176 if (myUse == null) { 177 myUse = new BoundCodeDt<NameUseEnum>(NameUseEnum.VALUESET_BINDER); 178 } 179 return myUse; 180 } 181 182 183 /** 184 * Gets the value(s) for <b>use</b> (). 185 * creating it if it does 186 * not exist. This method may return <code>null</code>. 187 * 188 * <p> 189 * <b>Definition:</b> 190 * Identifies the purpose for this name 191 * </p> 192 */ 193 public String getUse() { 194 return getUseElement().getValue(); 195 } 196 197 /** 198 * Sets the value(s) for <b>use</b> () 199 * 200 * <p> 201 * <b>Definition:</b> 202 * Identifies the purpose for this name 203 * </p> 204 */ 205 public HumanNameDt setUse(BoundCodeDt<NameUseEnum> theValue) { 206 myUse = theValue; 207 return this; 208 } 209 210 211 212 /** 213 * Sets the value(s) for <b>use</b> () 214 * 215 * <p> 216 * <b>Definition:</b> 217 * Identifies the purpose for this name 218 * </p> 219 */ 220 public HumanNameDt setUse(NameUseEnum theValue) { 221 setUse(new BoundCodeDt<NameUseEnum>(NameUseEnum.VALUESET_BINDER, theValue)); 222 223/* 224 getUseElement().setValueAsEnum(theValue); 225*/ 226 return this; 227 } 228 229 230 /** 231 * Gets the value(s) for <b>text</b> (). 232 * creating it if it does 233 * not exist. Will not return <code>null</code>. 234 * 235 * <p> 236 * <b>Definition:</b> 237 * A full text representation of the name 238 * </p> 239 */ 240 public StringDt getTextElement() { 241 if (myText == null) { 242 myText = new StringDt(); 243 } 244 return myText; 245 } 246 247 248 /** 249 * Gets the value(s) for <b>text</b> (). 250 * creating it if it does 251 * not exist. This method may return <code>null</code>. 252 * 253 * <p> 254 * <b>Definition:</b> 255 * A full text representation of the name 256 * </p> 257 */ 258 public String getText() { 259 return getTextElement().getValue(); 260 } 261 262 /** 263 * Sets the value(s) for <b>text</b> () 264 * 265 * <p> 266 * <b>Definition:</b> 267 * A full text representation of the name 268 * </p> 269 */ 270 public HumanNameDt setText(StringDt theValue) { 271 myText = theValue; 272 return this; 273 } 274 275 276 277 /** 278 * Sets the value for <b>text</b> () 279 * 280 * <p> 281 * <b>Definition:</b> 282 * A full text representation of the name 283 * </p> 284 */ 285 public HumanNameDt setText( String theString) { 286 myText = new StringDt(theString); 287 return this; 288 } 289 290 291 /** 292 * Gets the value(s) for <b>family</b> (). 293 * creating it if it does 294 * not exist. Will not return <code>null</code>. 295 * 296 * <p> 297 * <b>Definition:</b> 298 * The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 299 * </p> 300 */ 301 public java.util.List<StringDt> getFamily() { 302 if (myFamily == null) { 303 myFamily = new java.util.ArrayList<StringDt>(); 304 } 305 return myFamily; 306 } 307 308 /** 309 * Sets the value(s) for <b>family</b> () 310 * 311 * <p> 312 * <b>Definition:</b> 313 * The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 314 * </p> 315 */ 316 public HumanNameDt setFamily(java.util.List<StringDt> theValue) { 317 myFamily = theValue; 318 return this; 319 } 320 321 322 323 /** 324 * Adds and returns a new value for <b>family</b> () 325 * 326 * <p> 327 * <b>Definition:</b> 328 * The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 329 * </p> 330 */ 331 public StringDt addFamily() { 332 StringDt newType = new StringDt(); 333 getFamily().add(newType); 334 return newType; 335 } 336 337 /** 338 * Adds a given new value for <b>family</b> () 339 * 340 * <p> 341 * <b>Definition:</b> 342 * The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 343 * </p> 344 * @param theValue The family to add (must not be <code>null</code>) 345 */ 346 public HumanNameDt addFamily(StringDt theValue) { 347 if (theValue == null) { 348 throw new NullPointerException("theValue must not be null"); 349 } 350 getFamily().add(theValue); 351 return this; 352 } 353 354 /** 355 * Gets the first repetition for <b>family</b> (), 356 * creating it if it does not already exist. 357 * 358 * <p> 359 * <b>Definition:</b> 360 * The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 361 * </p> 362 */ 363 public StringDt getFamilyFirstRep() { 364 if (getFamily().isEmpty()) { 365 return addFamily(); 366 } 367 return getFamily().get(0); 368 } 369 /** 370 * Adds a new value for <b>family</b> () 371 * 372 * <p> 373 * <b>Definition:</b> 374 * The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 375 * </p> 376 * 377 * @return Returns a reference to this object, to allow for simple chaining. 378 */ 379 public HumanNameDt addFamily( String theString) { 380 if (myFamily == null) { 381 myFamily = new java.util.ArrayList<StringDt>(); 382 } 383 myFamily.add(new StringDt(theString)); 384 return this; 385 } 386 387 388 /** 389 * Gets the value(s) for <b>given</b> (). 390 * creating it if it does 391 * not exist. Will not return <code>null</code>. 392 * 393 * <p> 394 * <b>Definition:</b> 395 * Given name 396 * </p> 397 */ 398 public java.util.List<StringDt> getGiven() { 399 if (myGiven == null) { 400 myGiven = new java.util.ArrayList<StringDt>(); 401 } 402 return myGiven; 403 } 404 405 /** 406 * Sets the value(s) for <b>given</b> () 407 * 408 * <p> 409 * <b>Definition:</b> 410 * Given name 411 * </p> 412 */ 413 public HumanNameDt setGiven(java.util.List<StringDt> theValue) { 414 myGiven = theValue; 415 return this; 416 } 417 418 419 420 /** 421 * Adds and returns a new value for <b>given</b> () 422 * 423 * <p> 424 * <b>Definition:</b> 425 * Given name 426 * </p> 427 */ 428 public StringDt addGiven() { 429 StringDt newType = new StringDt(); 430 getGiven().add(newType); 431 return newType; 432 } 433 434 /** 435 * Adds a given new value for <b>given</b> () 436 * 437 * <p> 438 * <b>Definition:</b> 439 * Given name 440 * </p> 441 * @param theValue The given to add (must not be <code>null</code>) 442 */ 443 public HumanNameDt addGiven(StringDt theValue) { 444 if (theValue == null) { 445 throw new NullPointerException("theValue must not be null"); 446 } 447 getGiven().add(theValue); 448 return this; 449 } 450 451 /** 452 * Gets the first repetition for <b>given</b> (), 453 * creating it if it does not already exist. 454 * 455 * <p> 456 * <b>Definition:</b> 457 * Given name 458 * </p> 459 */ 460 public StringDt getGivenFirstRep() { 461 if (getGiven().isEmpty()) { 462 return addGiven(); 463 } 464 return getGiven().get(0); 465 } 466 /** 467 * Adds a new value for <b>given</b> () 468 * 469 * <p> 470 * <b>Definition:</b> 471 * Given name 472 * </p> 473 * 474 * @return Returns a reference to this object, to allow for simple chaining. 475 */ 476 public HumanNameDt addGiven( String theString) { 477 if (myGiven == null) { 478 myGiven = new java.util.ArrayList<StringDt>(); 479 } 480 myGiven.add(new StringDt(theString)); 481 return this; 482 } 483 484 485 /** 486 * Gets the value(s) for <b>prefix</b> (). 487 * creating it if it does 488 * not exist. Will not return <code>null</code>. 489 * 490 * <p> 491 * <b>Definition:</b> 492 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name 493 * </p> 494 */ 495 public java.util.List<StringDt> getPrefix() { 496 if (myPrefix == null) { 497 myPrefix = new java.util.ArrayList<StringDt>(); 498 } 499 return myPrefix; 500 } 501 502 /** 503 * Sets the value(s) for <b>prefix</b> () 504 * 505 * <p> 506 * <b>Definition:</b> 507 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name 508 * </p> 509 */ 510 public HumanNameDt setPrefix(java.util.List<StringDt> theValue) { 511 myPrefix = theValue; 512 return this; 513 } 514 515 516 517 /** 518 * Adds and returns a new value for <b>prefix</b> () 519 * 520 * <p> 521 * <b>Definition:</b> 522 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name 523 * </p> 524 */ 525 public StringDt addPrefix() { 526 StringDt newType = new StringDt(); 527 getPrefix().add(newType); 528 return newType; 529 } 530 531 /** 532 * Adds a given new value for <b>prefix</b> () 533 * 534 * <p> 535 * <b>Definition:</b> 536 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name 537 * </p> 538 * @param theValue The prefix to add (must not be <code>null</code>) 539 */ 540 public HumanNameDt addPrefix(StringDt theValue) { 541 if (theValue == null) { 542 throw new NullPointerException("theValue must not be null"); 543 } 544 getPrefix().add(theValue); 545 return this; 546 } 547 548 /** 549 * Gets the first repetition for <b>prefix</b> (), 550 * creating it if it does not already exist. 551 * 552 * <p> 553 * <b>Definition:</b> 554 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name 555 * </p> 556 */ 557 public StringDt getPrefixFirstRep() { 558 if (getPrefix().isEmpty()) { 559 return addPrefix(); 560 } 561 return getPrefix().get(0); 562 } 563 /** 564 * Adds a new value for <b>prefix</b> () 565 * 566 * <p> 567 * <b>Definition:</b> 568 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name 569 * </p> 570 * 571 * @return Returns a reference to this object, to allow for simple chaining. 572 */ 573 public HumanNameDt addPrefix( String theString) { 574 if (myPrefix == null) { 575 myPrefix = new java.util.ArrayList<StringDt>(); 576 } 577 myPrefix.add(new StringDt(theString)); 578 return this; 579 } 580 581 582 /** 583 * Gets the value(s) for <b>suffix</b> (). 584 * creating it if it does 585 * not exist. Will not return <code>null</code>. 586 * 587 * <p> 588 * <b>Definition:</b> 589 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name 590 * </p> 591 */ 592 public java.util.List<StringDt> getSuffix() { 593 if (mySuffix == null) { 594 mySuffix = new java.util.ArrayList<StringDt>(); 595 } 596 return mySuffix; 597 } 598 599 /** 600 * Sets the value(s) for <b>suffix</b> () 601 * 602 * <p> 603 * <b>Definition:</b> 604 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name 605 * </p> 606 */ 607 public HumanNameDt setSuffix(java.util.List<StringDt> theValue) { 608 mySuffix = theValue; 609 return this; 610 } 611 612 613 614 /** 615 * Adds and returns a new value for <b>suffix</b> () 616 * 617 * <p> 618 * <b>Definition:</b> 619 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name 620 * </p> 621 */ 622 public StringDt addSuffix() { 623 StringDt newType = new StringDt(); 624 getSuffix().add(newType); 625 return newType; 626 } 627 628 /** 629 * Adds a given new value for <b>suffix</b> () 630 * 631 * <p> 632 * <b>Definition:</b> 633 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name 634 * </p> 635 * @param theValue The suffix to add (must not be <code>null</code>) 636 */ 637 public HumanNameDt addSuffix(StringDt theValue) { 638 if (theValue == null) { 639 throw new NullPointerException("theValue must not be null"); 640 } 641 getSuffix().add(theValue); 642 return this; 643 } 644 645 /** 646 * Gets the first repetition for <b>suffix</b> (), 647 * creating it if it does not already exist. 648 * 649 * <p> 650 * <b>Definition:</b> 651 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name 652 * </p> 653 */ 654 public StringDt getSuffixFirstRep() { 655 if (getSuffix().isEmpty()) { 656 return addSuffix(); 657 } 658 return getSuffix().get(0); 659 } 660 /** 661 * Adds a new value for <b>suffix</b> () 662 * 663 * <p> 664 * <b>Definition:</b> 665 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name 666 * </p> 667 * 668 * @return Returns a reference to this object, to allow for simple chaining. 669 */ 670 public HumanNameDt addSuffix( String theString) { 671 if (mySuffix == null) { 672 mySuffix = new java.util.ArrayList<StringDt>(); 673 } 674 mySuffix.add(new StringDt(theString)); 675 return this; 676 } 677 678 679 /** 680 * Gets the value(s) for <b>period</b> (). 681 * creating it if it does 682 * not exist. Will not return <code>null</code>. 683 * 684 * <p> 685 * <b>Definition:</b> 686 * Indicates the period of time when this name was valid for the named person. 687 * </p> 688 */ 689 public PeriodDt getPeriod() { 690 if (myPeriod == null) { 691 myPeriod = new PeriodDt(); 692 } 693 return myPeriod; 694 } 695 696 /** 697 * Sets the value(s) for <b>period</b> () 698 * 699 * <p> 700 * <b>Definition:</b> 701 * Indicates the period of time when this name was valid for the named person. 702 * </p> 703 */ 704 public HumanNameDt setPeriod(PeriodDt theValue) { 705 myPeriod = theValue; 706 return this; 707 } 708 709 710 711 712 713 714}