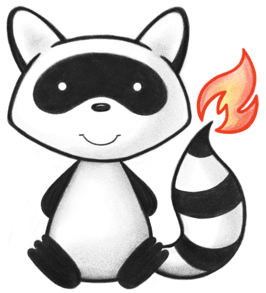
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>IdentifierDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * A technical identifier - identifies some entity uniquely and unambiguously 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * Need to be able to identify things with confidence and be sure that the identification is not subject to misinterpretation 088 * </p> 089 */ 090@DatatypeDef(name="Identifier") 091public class IdentifierDt 092 extends BaseIdentifierDt 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public IdentifierDt() { 100 // nothing 101 } 102 103 /** 104 * Creates a new identifier with the given system and value 105 */ 106 @SimpleSetter 107 public IdentifierDt(@SimpleSetter.Parameter(name="theSystem") String theSystem, @SimpleSetter.Parameter(name="theValue") String theValue) { 108 setSystem(theSystem); 109 setValue(theValue); 110 } 111 112 @Override 113 public String toString() { 114 return "IdentifierDt[" + getValue() + "]"; 115 } 116 117 @Child(name="use", type=CodeDt.class, order=0, min=0, max=1, summary=true, modifier=true) 118 @Description( 119 shortDefinition="", 120 formalDefinition="The purpose of this identifier" 121 ) 122 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/identifier-use") 123 private BoundCodeDt<IdentifierUseEnum> myUse; 124 125 @Child(name="type", type=CodeableConceptDt.class, order=1, min=0, max=1, summary=true, modifier=false) 126 @Description( 127 shortDefinition="", 128 formalDefinition="A coded type for the identifier that can be used to determine which identifier to use for a specific purpose" 129 ) 130 private BoundCodeableConceptDt<IdentifierTypeCodesEnum> myType; 131 132 @Child(name="system", type=UriDt.class, order=2, min=0, max=1, summary=true, modifier=false) 133 @Description( 134 shortDefinition="", 135 formalDefinition="Establishes the namespace in which set of possible id values is unique." 136 ) 137 private UriDt mySystem; 138 139 @Child(name="value", type=StringDt.class, order=3, min=0, max=1, summary=true, modifier=false) 140 @Description( 141 shortDefinition="", 142 formalDefinition="The portion of the identifier typically displayed to the user and which is unique within the context of the system." 143 ) 144 private StringDt myValue; 145 146 @Child(name="period", type=PeriodDt.class, order=4, min=0, max=1, summary=true, modifier=false) 147 @Description( 148 shortDefinition="", 149 formalDefinition="Time period during which identifier is/was valid for use" 150 ) 151 private PeriodDt myPeriod; 152 153 @Child(name="assigner", order=5, min=0, max=1, summary=true, modifier=false, type={ 154 ca.uhn.fhir.model.dstu2.resource.Organization.class 155 }) 156 @Description( 157 shortDefinition="", 158 formalDefinition="Organization that issued/manages the identifier" 159 ) 160 private ResourceReferenceDt myAssigner; 161 162 163 @Override 164 public boolean isEmpty() { 165 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myUse, myType, mySystem, myValue, myPeriod, myAssigner); 166 } 167 168 @Override 169 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 170 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myUse, myType, mySystem, myValue, myPeriod, myAssigner); 171 } 172 173 /** 174 * Gets the value(s) for <b>use</b> (). 175 * creating it if it does 176 * not exist. Will not return <code>null</code>. 177 * 178 * <p> 179 * <b>Definition:</b> 180 * The purpose of this identifier 181 * </p> 182 */ 183 public BoundCodeDt<IdentifierUseEnum> getUseElement() { 184 if (myUse == null) { 185 myUse = new BoundCodeDt<IdentifierUseEnum>(IdentifierUseEnum.VALUESET_BINDER); 186 } 187 return myUse; 188 } 189 190 191 /** 192 * Gets the value(s) for <b>use</b> (). 193 * creating it if it does 194 * not exist. This method may return <code>null</code>. 195 * 196 * <p> 197 * <b>Definition:</b> 198 * The purpose of this identifier 199 * </p> 200 */ 201 public String getUse() { 202 return getUseElement().getValue(); 203 } 204 205 /** 206 * Sets the value(s) for <b>use</b> () 207 * 208 * <p> 209 * <b>Definition:</b> 210 * The purpose of this identifier 211 * </p> 212 */ 213 public IdentifierDt setUse(BoundCodeDt<IdentifierUseEnum> theValue) { 214 myUse = theValue; 215 return this; 216 } 217 218 219 220 /** 221 * Sets the value(s) for <b>use</b> () 222 * 223 * <p> 224 * <b>Definition:</b> 225 * The purpose of this identifier 226 * </p> 227 */ 228 public IdentifierDt setUse(IdentifierUseEnum theValue) { 229 setUse(new BoundCodeDt<IdentifierUseEnum>(IdentifierUseEnum.VALUESET_BINDER, theValue)); 230 231/* 232 getUseElement().setValueAsEnum(theValue); 233*/ 234 return this; 235 } 236 237 238 /** 239 * Gets the value(s) for <b>type</b> (). 240 * creating it if it does 241 * not exist. Will not return <code>null</code>. 242 * 243 * <p> 244 * <b>Definition:</b> 245 * A coded type for the identifier that can be used to determine which identifier to use for a specific purpose 246 * </p> 247 */ 248 public BoundCodeableConceptDt<IdentifierTypeCodesEnum> getType() { 249 if (myType == null) { 250 myType = new BoundCodeableConceptDt<IdentifierTypeCodesEnum>(IdentifierTypeCodesEnum.VALUESET_BINDER); 251 } 252 return myType; 253 } 254 255 /** 256 * Sets the value(s) for <b>type</b> () 257 * 258 * <p> 259 * <b>Definition:</b> 260 * A coded type for the identifier that can be used to determine which identifier to use for a specific purpose 261 * </p> 262 */ 263 public IdentifierDt setType(BoundCodeableConceptDt<IdentifierTypeCodesEnum> theValue) { 264 myType = theValue; 265 return this; 266 } 267 268 269 270 /** 271 * Sets the value(s) for <b>type</b> () 272 * 273 * <p> 274 * <b>Definition:</b> 275 * A coded type for the identifier that can be used to determine which identifier to use for a specific purpose 276 * </p> 277 */ 278 public IdentifierDt setType(IdentifierTypeCodesEnum theValue) { 279 setType(new BoundCodeableConceptDt<IdentifierTypeCodesEnum>(IdentifierTypeCodesEnum.VALUESET_BINDER, theValue)); 280 281/* 282 getType().setValueAsEnum(theValue); 283*/ 284 return this; 285 } 286 287 288 /** 289 * Gets the value(s) for <b>system</b> (). 290 * creating it if it does 291 * not exist. Will not return <code>null</code>. 292 * 293 * <p> 294 * <b>Definition:</b> 295 * Establishes the namespace in which set of possible id values is unique. 296 * </p> 297 */ 298 public UriDt getSystemElement() { 299 if (mySystem == null) { 300 mySystem = new UriDt(); 301 } 302 return mySystem; 303 } 304 305 306 /** 307 * Gets the value(s) for <b>system</b> (). 308 * creating it if it does 309 * not exist. This method may return <code>null</code>. 310 * 311 * <p> 312 * <b>Definition:</b> 313 * Establishes the namespace in which set of possible id values is unique. 314 * </p> 315 */ 316 public String getSystem() { 317 return getSystemElement().getValue(); 318 } 319 320 /** 321 * Sets the value(s) for <b>system</b> () 322 * 323 * <p> 324 * <b>Definition:</b> 325 * Establishes the namespace in which set of possible id values is unique. 326 * </p> 327 */ 328 public IdentifierDt setSystem(UriDt theValue) { 329 mySystem = theValue; 330 return this; 331 } 332 333 334 335 /** 336 * Sets the value for <b>system</b> () 337 * 338 * <p> 339 * <b>Definition:</b> 340 * Establishes the namespace in which set of possible id values is unique. 341 * </p> 342 */ 343 public IdentifierDt setSystem( String theUri) { 344 mySystem = new UriDt(theUri); 345 return this; 346 } 347 348 349 /** 350 * Gets the value(s) for <b>value</b> (). 351 * creating it if it does 352 * not exist. Will not return <code>null</code>. 353 * 354 * <p> 355 * <b>Definition:</b> 356 * The portion of the identifier typically displayed to the user and which is unique within the context of the system. 357 * </p> 358 */ 359 public StringDt getValueElement() { 360 if (myValue == null) { 361 myValue = new StringDt(); 362 } 363 return myValue; 364 } 365 366 367 /** 368 * Gets the value(s) for <b>value</b> (). 369 * creating it if it does 370 * not exist. This method may return <code>null</code>. 371 * 372 * <p> 373 * <b>Definition:</b> 374 * The portion of the identifier typically displayed to the user and which is unique within the context of the system. 375 * </p> 376 */ 377 public String getValue() { 378 return getValueElement().getValue(); 379 } 380 381 /** 382 * Sets the value(s) for <b>value</b> () 383 * 384 * <p> 385 * <b>Definition:</b> 386 * The portion of the identifier typically displayed to the user and which is unique within the context of the system. 387 * </p> 388 */ 389 public IdentifierDt setValue(StringDt theValue) { 390 myValue = theValue; 391 return this; 392 } 393 394 395 396 /** 397 * Sets the value for <b>value</b> () 398 * 399 * <p> 400 * <b>Definition:</b> 401 * The portion of the identifier typically displayed to the user and which is unique within the context of the system. 402 * </p> 403 */ 404 public IdentifierDt setValue( String theString) { 405 myValue = new StringDt(theString); 406 return this; 407 } 408 409 410 /** 411 * Gets the value(s) for <b>period</b> (). 412 * creating it if it does 413 * not exist. Will not return <code>null</code>. 414 * 415 * <p> 416 * <b>Definition:</b> 417 * Time period during which identifier is/was valid for use 418 * </p> 419 */ 420 public PeriodDt getPeriod() { 421 if (myPeriod == null) { 422 myPeriod = new PeriodDt(); 423 } 424 return myPeriod; 425 } 426 427 /** 428 * Sets the value(s) for <b>period</b> () 429 * 430 * <p> 431 * <b>Definition:</b> 432 * Time period during which identifier is/was valid for use 433 * </p> 434 */ 435 public IdentifierDt setPeriod(PeriodDt theValue) { 436 myPeriod = theValue; 437 return this; 438 } 439 440 441 442 443 /** 444 * Gets the value(s) for <b>assigner</b> (). 445 * creating it if it does 446 * not exist. Will not return <code>null</code>. 447 * 448 * <p> 449 * <b>Definition:</b> 450 * Organization that issued/manages the identifier 451 * </p> 452 */ 453 public ResourceReferenceDt getAssigner() { 454 if (myAssigner == null) { 455 myAssigner = new ResourceReferenceDt(); 456 } 457 return myAssigner; 458 } 459 460 /** 461 * Sets the value(s) for <b>assigner</b> () 462 * 463 * <p> 464 * <b>Definition:</b> 465 * Organization that issued/manages the identifier 466 * </p> 467 */ 468 public IdentifierDt setAssigner(ResourceReferenceDt theValue) { 469 myAssigner = theValue; 470 return this; 471 } 472 473 474 475 476 477 478}