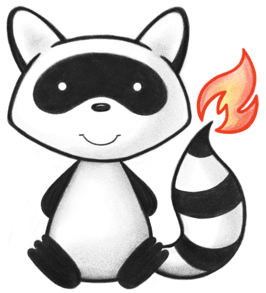
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>MetaDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * 088 * </p> 089 */ 090@DatatypeDef(name="Meta") 091public class MetaDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype, org.hl7.fhir.instance.model.api.IBaseMetaType 094{ 095 096 /** 097 * Constructor 098 */ 099 public MetaDt() { 100 // nothing 101 } 102 103 @Override 104 public MetaDt setLastUpdated(Date theHeaderDateValue) { 105 return setLastUpdated(theHeaderDateValue, TemporalPrecisionEnum.SECOND); 106 } 107 108 /** 109 * Returns the first tag (if any) that has the given system and code, or returns 110 * <code>null</code> if none 111 */ 112 public CodingDt getTag(String theSystem, String theCode) { 113 for (CodingDt next : getTag()) { 114 if (ca.uhn.fhir.util.ObjectUtil.equals(next.getSystem(), theSystem) && ca.uhn.fhir.util.ObjectUtil.equals(next.getCode(), theCode)) { 115 return next; 116 } 117 } 118 return null; 119 } 120 121 /** 122 * Returns the first security label (if any) that has the given system and code, or returns 123 * <code>null</code> if none 124 */ 125 public CodingDt getSecurity(String theSystem, String theCode) { 126 for (CodingDt next : getTag()) { 127 if (ca.uhn.fhir.util.ObjectUtil.equals(next.getSystem(), theSystem) && ca.uhn.fhir.util.ObjectUtil.equals(next.getCode(), theCode)) { 128 return next; 129 } 130 } 131 return null; 132 } 133 134 135 @Child(name="versionId", type=IdDt.class, order=0, min=0, max=1, summary=true, modifier=false) 136 @Description( 137 shortDefinition="", 138 formalDefinition="The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted" 139 ) 140 private IdDt myVersionId; 141 142 @Child(name="lastUpdated", type=InstantDt.class, order=1, min=0, max=1, summary=true, modifier=false) 143 @Description( 144 shortDefinition="", 145 formalDefinition="When the resource last changed - e.g. when the version changed" 146 ) 147 private InstantDt myLastUpdated; 148 149 @Child(name="profile", type=UriDt.class, order=2, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 150 @Description( 151 shortDefinition="", 152 formalDefinition="A list of profiles [[[StructureDefinition]]]s that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]]" 153 ) 154 private java.util.List<UriDt> myProfile; 155 156 @Child(name="security", type=CodingDt.class, order=3, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 157 @Description( 158 shortDefinition="", 159 formalDefinition="Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure" 160 ) 161 private java.util.List<CodingDt> mySecurity; 162 163 @Child(name="tag", type=CodingDt.class, order=4, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 164 @Description( 165 shortDefinition="", 166 formalDefinition="Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource" 167 ) 168 private java.util.List<CodingDt> myTag; 169 170 171 @Override 172 public boolean isEmpty() { 173 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myVersionId, myLastUpdated, myProfile, mySecurity, myTag); 174 } 175 176 @Override 177 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 178 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myVersionId, myLastUpdated, myProfile, mySecurity, myTag); 179 } 180 181 /** 182 * Gets the value(s) for <b>versionId</b> (). 183 * creating it if it does 184 * not exist. Will not return <code>null</code>. 185 * 186 * <p> 187 * <b>Definition:</b> 188 * The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted 189 * </p> 190 */ 191 public IdDt getVersionIdElement() { 192 if (myVersionId == null) { 193 myVersionId = new IdDt(); 194 } 195 return myVersionId; 196 } 197 198 199 /** 200 * Gets the value(s) for <b>versionId</b> (). 201 * creating it if it does 202 * not exist. This method may return <code>null</code>. 203 * 204 * <p> 205 * <b>Definition:</b> 206 * The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted 207 * </p> 208 */ 209 public String getVersionId() { 210 return getVersionIdElement().getValue(); 211 } 212 213 /** 214 * Sets the value(s) for <b>versionId</b> () 215 * 216 * <p> 217 * <b>Definition:</b> 218 * The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted 219 * </p> 220 */ 221 public MetaDt setVersionId(IdDt theValue) { 222 myVersionId = theValue; 223 return this; 224 } 225 226 227 228 /** 229 * Sets the value for <b>versionId</b> () 230 * 231 * <p> 232 * <b>Definition:</b> 233 * The version specific identifier, as it appears in the version portion of the URL. This values changes when the resource is created, updated, or deleted 234 * </p> 235 */ 236 public MetaDt setVersionId( String theId) { 237 myVersionId = new IdDt(theId); 238 return this; 239 } 240 241 242 /** 243 * Gets the value(s) for <b>lastUpdated</b> (). 244 * creating it if it does 245 * not exist. Will not return <code>null</code>. 246 * 247 * <p> 248 * <b>Definition:</b> 249 * When the resource last changed - e.g. when the version changed 250 * </p> 251 */ 252 public InstantDt getLastUpdatedElement() { 253 if (myLastUpdated == null) { 254 myLastUpdated = new InstantDt(); 255 } 256 return myLastUpdated; 257 } 258 259 260 /** 261 * Gets the value(s) for <b>lastUpdated</b> (). 262 * creating it if it does 263 * not exist. This method may return <code>null</code>. 264 * 265 * <p> 266 * <b>Definition:</b> 267 * When the resource last changed - e.g. when the version changed 268 * </p> 269 */ 270 public Date getLastUpdated() { 271 return getLastUpdatedElement().getValue(); 272 } 273 274 /** 275 * Sets the value(s) for <b>lastUpdated</b> () 276 * 277 * <p> 278 * <b>Definition:</b> 279 * When the resource last changed - e.g. when the version changed 280 * </p> 281 */ 282 public MetaDt setLastUpdated(InstantDt theValue) { 283 myLastUpdated = theValue; 284 return this; 285 } 286 287 288 289 /** 290 * Sets the value for <b>lastUpdated</b> () 291 * 292 * <p> 293 * <b>Definition:</b> 294 * When the resource last changed - e.g. when the version changed 295 * </p> 296 */ 297 public MetaDt setLastUpdatedWithMillisPrecision( Date theDate) { 298 myLastUpdated = new InstantDt(theDate); 299 return this; 300 } 301 302 /** 303 * Sets the value for <b>lastUpdated</b> () 304 * 305 * <p> 306 * <b>Definition:</b> 307 * When the resource last changed - e.g. when the version changed 308 * </p> 309 */ 310 public MetaDt setLastUpdated( Date theDate, TemporalPrecisionEnum thePrecision) { 311 myLastUpdated = new InstantDt(theDate, thePrecision); 312 return this; 313 } 314 315 316 /** 317 * Gets the value(s) for <b>profile</b> (). 318 * creating it if it does 319 * not exist. Will not return <code>null</code>. 320 * 321 * <p> 322 * <b>Definition:</b> 323 * A list of profiles [[[StructureDefinition]]]s that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]] 324 * </p> 325 */ 326 public java.util.List<UriDt> getProfile() { 327 if (myProfile == null) { 328 myProfile = new java.util.ArrayList<UriDt>(); 329 } 330 return myProfile; 331 } 332 333 /** 334 * Sets the value(s) for <b>profile</b> () 335 * 336 * <p> 337 * <b>Definition:</b> 338 * A list of profiles [[[StructureDefinition]]]s that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]] 339 * </p> 340 */ 341 public MetaDt setProfile(java.util.List<UriDt> theValue) { 342 myProfile = theValue; 343 return this; 344 } 345 346 347 348 /** 349 * Adds and returns a new value for <b>profile</b> () 350 * 351 * <p> 352 * <b>Definition:</b> 353 * A list of profiles [[[StructureDefinition]]]s that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]] 354 * </p> 355 */ 356 public UriDt addProfile() { 357 UriDt newType = new UriDt(); 358 getProfile().add(newType); 359 return newType; 360 } 361 362 /** 363 * Adds a given new value for <b>profile</b> () 364 * 365 * <p> 366 * <b>Definition:</b> 367 * A list of profiles [[[StructureDefinition]]]s that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]] 368 * </p> 369 * @param theValue The profile to add (must not be <code>null</code>) 370 */ 371 public MetaDt addProfile(UriDt theValue) { 372 if (theValue == null) { 373 throw new NullPointerException("theValue must not be null"); 374 } 375 getProfile().add(theValue); 376 return this; 377 } 378 379 /** 380 * Gets the first repetition for <b>profile</b> (), 381 * creating it if it does not already exist. 382 * 383 * <p> 384 * <b>Definition:</b> 385 * A list of profiles [[[StructureDefinition]]]s that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]] 386 * </p> 387 */ 388 public UriDt getProfileFirstRep() { 389 if (getProfile().isEmpty()) { 390 return addProfile(); 391 } 392 return getProfile().get(0); 393 } 394 /** 395 * Adds a new value for <b>profile</b> () 396 * 397 * <p> 398 * <b>Definition:</b> 399 * A list of profiles [[[StructureDefinition]]]s that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]] 400 * </p> 401 * 402 * @return Returns a reference to this object, to allow for simple chaining. 403 */ 404 public MetaDt addProfile( String theUri) { 405 if (myProfile == null) { 406 myProfile = new java.util.ArrayList<UriDt>(); 407 } 408 myProfile.add(new UriDt(theUri)); 409 return this; 410 } 411 412 413 /** 414 * Gets the value(s) for <b>security</b> (). 415 * creating it if it does 416 * not exist. Will not return <code>null</code>. 417 * 418 * <p> 419 * <b>Definition:</b> 420 * Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure 421 * </p> 422 */ 423 public java.util.List<CodingDt> getSecurity() { 424 if (mySecurity == null) { 425 mySecurity = new java.util.ArrayList<CodingDt>(); 426 } 427 return mySecurity; 428 } 429 430 /** 431 * Sets the value(s) for <b>security</b> () 432 * 433 * <p> 434 * <b>Definition:</b> 435 * Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure 436 * </p> 437 */ 438 public MetaDt setSecurity(java.util.List<CodingDt> theValue) { 439 mySecurity = theValue; 440 return this; 441 } 442 443 444 445 /** 446 * Adds and returns a new value for <b>security</b> () 447 * 448 * <p> 449 * <b>Definition:</b> 450 * Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure 451 * </p> 452 */ 453 public CodingDt addSecurity() { 454 CodingDt newType = new CodingDt(); 455 getSecurity().add(newType); 456 return newType; 457 } 458 459 /** 460 * Adds a given new value for <b>security</b> () 461 * 462 * <p> 463 * <b>Definition:</b> 464 * Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure 465 * </p> 466 * @param theValue The security to add (must not be <code>null</code>) 467 */ 468 public MetaDt addSecurity(CodingDt theValue) { 469 if (theValue == null) { 470 throw new NullPointerException("theValue must not be null"); 471 } 472 getSecurity().add(theValue); 473 return this; 474 } 475 476 /** 477 * Gets the first repetition for <b>security</b> (), 478 * creating it if it does not already exist. 479 * 480 * <p> 481 * <b>Definition:</b> 482 * Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure 483 * </p> 484 */ 485 public CodingDt getSecurityFirstRep() { 486 if (getSecurity().isEmpty()) { 487 return addSecurity(); 488 } 489 return getSecurity().get(0); 490 } 491 492 /** 493 * Gets the value(s) for <b>tag</b> (). 494 * creating it if it does 495 * not exist. Will not return <code>null</code>. 496 * 497 * <p> 498 * <b>Definition:</b> 499 * Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource 500 * </p> 501 */ 502 public java.util.List<CodingDt> getTag() { 503 if (myTag == null) { 504 myTag = new java.util.ArrayList<CodingDt>(); 505 } 506 return myTag; 507 } 508 509 /** 510 * Sets the value(s) for <b>tag</b> () 511 * 512 * <p> 513 * <b>Definition:</b> 514 * Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource 515 * </p> 516 */ 517 public MetaDt setTag(java.util.List<CodingDt> theValue) { 518 myTag = theValue; 519 return this; 520 } 521 522 523 524 /** 525 * Adds and returns a new value for <b>tag</b> () 526 * 527 * <p> 528 * <b>Definition:</b> 529 * Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource 530 * </p> 531 */ 532 public CodingDt addTag() { 533 CodingDt newType = new CodingDt(); 534 getTag().add(newType); 535 return newType; 536 } 537 538 /** 539 * Adds a given new value for <b>tag</b> () 540 * 541 * <p> 542 * <b>Definition:</b> 543 * Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource 544 * </p> 545 * @param theValue The tag to add (must not be <code>null</code>) 546 */ 547 public MetaDt addTag(CodingDt theValue) { 548 if (theValue == null) { 549 throw new NullPointerException("theValue must not be null"); 550 } 551 getTag().add(theValue); 552 return this; 553 } 554 555 /** 556 * Gets the first repetition for <b>tag</b> (), 557 * creating it if it does not already exist. 558 * 559 * <p> 560 * <b>Definition:</b> 561 * Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource 562 * </p> 563 */ 564 public CodingDt getTagFirstRep() { 565 if (getTag().isEmpty()) { 566 return addTag(); 567 } 568 return getTag().get(0); 569 } 570 571 572 573}