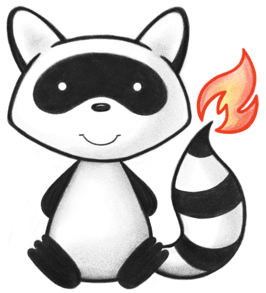
001/* 002 * #%L 003 * HAPI FHIR Structures - DSTU2 (FHIR v1.0.0) 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.dstu2.composite; 021 022import ca.uhn.fhir.model.api.IElement; 023import ca.uhn.fhir.model.api.annotation.Child; 024import ca.uhn.fhir.model.api.annotation.DatatypeDef; 025import ca.uhn.fhir.model.base.composite.BaseNarrativeDt; 026import ca.uhn.fhir.model.dstu2.valueset.NarrativeStatusEnum; 027import ca.uhn.fhir.model.primitive.BoundCodeDt; 028import ca.uhn.fhir.model.primitive.CodeDt; 029import ca.uhn.fhir.model.primitive.XhtmlDt; 030 031import java.util.List; 032 033/** 034 * HAPI/FHIR <b>Narrative</b> Datatype 035 * (A human-readable formatted text, including images) 036 * 037 * <p> 038 * <b>Definition:</b> 039 * A human-readable formatted text, including images 040 * </p> 041 * 042 * <p> 043 * <b>Requirements:</b> 044 * 045 * </p> 046 */ 047@DatatypeDef(name = "Narrative") 048public class NarrativeDt extends BaseNarrativeDt { 049 050 @Child(name = "status", type = CodeDt.class, order = 0, min = 1, max = 1) 051 private BoundCodeDt<NarrativeStatusEnum> myStatus; 052 053 @Child(name = "div", type = XhtmlDt.class, order = 1, min = 1, max = 1) 054 private XhtmlDt myDiv; 055 056 public NarrativeDt() { 057 // nothing 058 } 059 060 public NarrativeDt(XhtmlDt theDiv, NarrativeStatusEnum theStatus) { 061 setDiv(theDiv); 062 setStatus(theStatus); 063 } 064 065 @Override 066 public boolean isEmpty() { 067 return ca.uhn.fhir.util.ElementUtil.isEmpty(myStatus, myDiv); 068 } 069 070 @Override 071 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 072 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myStatus, myDiv); 073 } 074 075 /** 076 * Gets the value(s) for <b>status</b> (generated | extensions | additional). 077 * creating it if it does 078 * not exist. Will not return <code>null</code>. 079 * 080 * <p> 081 * <b>Definition:</b> 082 * The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data 083 * </p> 084 */ 085 public BoundCodeDt<NarrativeStatusEnum> getStatusElement() { 086 return getStatus(); 087 } 088 089 /** 090 * Gets the value(s) for <b>status</b> (generated | extensions | additional). 091 * creating it if it does 092 * not exist. Will not return <code>null</code>. 093 * 094 * <p> 095 * <b>Definition:</b> 096 * The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data 097 * </p> 098 */ 099 @Override 100 public BoundCodeDt<NarrativeStatusEnum> getStatus() { 101 if (myStatus == null) { 102 myStatus = new BoundCodeDt<NarrativeStatusEnum>(NarrativeStatusEnum.VALUESET_BINDER); 103 } 104 return myStatus; 105 } 106 107 /** 108 * Sets the value(s) for <b>status</b> (generated | extensions | additional) 109 * 110 * <p> 111 * <b>Definition:</b> 112 * The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data 113 * </p> 114 */ 115 public void setStatus(BoundCodeDt<NarrativeStatusEnum> theValue) { 116 myStatus = theValue; 117 } 118 119 /** 120 * Sets the value(s) for <b>status</b> (generated | extensions | additional) 121 * 122 * <p> 123 * <b>Definition:</b> 124 * The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data 125 * </p> 126 */ 127 public void setStatus(NarrativeStatusEnum theValue) { 128 getStatus().setValueAsEnum(theValue); 129 } 130 131 /** 132 * Gets the value(s) for <b>div</b> (Limited xhtml content). 133 * creating it if it does 134 * not exist. Will not return <code>null</code>. 135 * 136 * <p> 137 * <b>Definition:</b> 138 * The actual narrative content, a stripped down version of XHTML 139 * </p> 140 */ 141 public XhtmlDt getDivElement() { 142 return getDiv(); 143 } 144 145 /** 146 * Gets the value(s) for <b>div</b> (Limited xhtml content). 147 * creating it if it does 148 * not exist. Will not return <code>null</code>. 149 * 150 * <p> 151 * <b>Definition:</b> 152 * The actual narrative content, a stripped down version of XHTML 153 * </p> 154 */ 155 @Override 156 public XhtmlDt getDiv() { 157 if (myDiv == null) { 158 myDiv = new XhtmlDt(); 159 } 160 return myDiv; 161 } 162 163 /** 164 * Sets the value(s) for <b>div</b> (Limited xhtml content) 165 * 166 * <p> 167 * <b>Definition:</b> 168 * The actual narrative content, a stripped down version of XHTML 169 * </p> 170 */ 171 public void setDiv(XhtmlDt theValue) { 172 myDiv = theValue; 173 } 174 175 /** 176 * Sets the value using a textual DIV (or simple text block which will be 177 * converted to XHTML) 178 */ 179 public void setDiv(String theTextDiv) { 180 myDiv = new XhtmlDt(theTextDiv); 181 } 182}