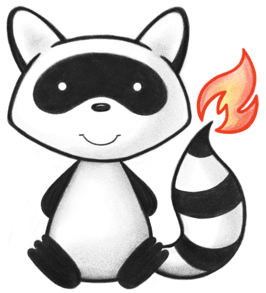
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>PeriodDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * A time period defined by a start and end date and optionally time. 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * 088 * </p> 089 */ 090@DatatypeDef(name="Period") 091public class PeriodDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public PeriodDt() { 100 // nothing 101 } 102 103 104 @Child(name="start", type=DateTimeDt.class, order=0, min=0, max=1, summary=true, modifier=false) 105 @Description( 106 shortDefinition="", 107 formalDefinition="The start of the period. The boundary is inclusive." 108 ) 109 private DateTimeDt myStart; 110 111 @Child(name="end", type=DateTimeDt.class, order=1, min=0, max=1, summary=true, modifier=false) 112 @Description( 113 shortDefinition="", 114 formalDefinition="The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time" 115 ) 116 private DateTimeDt myEnd; 117 118 119 @Override 120 public boolean isEmpty() { 121 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myStart, myEnd); 122 } 123 124 @Override 125 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 126 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myStart, myEnd); 127 } 128 129 /** 130 * Gets the value(s) for <b>start</b> (). 131 * creating it if it does 132 * not exist. Will not return <code>null</code>. 133 * 134 * <p> 135 * <b>Definition:</b> 136 * The start of the period. The boundary is inclusive. 137 * </p> 138 */ 139 public DateTimeDt getStartElement() { 140 if (myStart == null) { 141 myStart = new DateTimeDt(); 142 } 143 return myStart; 144 } 145 146 147 /** 148 * Gets the value(s) for <b>start</b> (). 149 * creating it if it does 150 * not exist. This method may return <code>null</code>. 151 * 152 * <p> 153 * <b>Definition:</b> 154 * The start of the period. The boundary is inclusive. 155 * </p> 156 */ 157 public Date getStart() { 158 return getStartElement().getValue(); 159 } 160 161 /** 162 * Sets the value(s) for <b>start</b> () 163 * 164 * <p> 165 * <b>Definition:</b> 166 * The start of the period. The boundary is inclusive. 167 * </p> 168 */ 169 public PeriodDt setStart(DateTimeDt theValue) { 170 myStart = theValue; 171 return this; 172 } 173 174 175 176 /** 177 * Sets the value for <b>start</b> () 178 * 179 * <p> 180 * <b>Definition:</b> 181 * The start of the period. The boundary is inclusive. 182 * </p> 183 */ 184 public PeriodDt setStartWithSecondsPrecision( Date theDate) { 185 myStart = new DateTimeDt(theDate); 186 return this; 187 } 188 189 /** 190 * Sets the value for <b>start</b> () 191 * 192 * <p> 193 * <b>Definition:</b> 194 * The start of the period. The boundary is inclusive. 195 * </p> 196 */ 197 public PeriodDt setStart( Date theDate, TemporalPrecisionEnum thePrecision) { 198 myStart = new DateTimeDt(theDate, thePrecision); 199 return this; 200 } 201 202 203 /** 204 * Gets the value(s) for <b>end</b> (). 205 * creating it if it does 206 * not exist. Will not return <code>null</code>. 207 * 208 * <p> 209 * <b>Definition:</b> 210 * The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time 211 * </p> 212 */ 213 public DateTimeDt getEndElement() { 214 if (myEnd == null) { 215 myEnd = new DateTimeDt(); 216 } 217 return myEnd; 218 } 219 220 221 /** 222 * Gets the value(s) for <b>end</b> (). 223 * creating it if it does 224 * not exist. This method may return <code>null</code>. 225 * 226 * <p> 227 * <b>Definition:</b> 228 * The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time 229 * </p> 230 */ 231 public Date getEnd() { 232 return getEndElement().getValue(); 233 } 234 235 /** 236 * Sets the value(s) for <b>end</b> () 237 * 238 * <p> 239 * <b>Definition:</b> 240 * The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time 241 * </p> 242 */ 243 public PeriodDt setEnd(DateTimeDt theValue) { 244 myEnd = theValue; 245 return this; 246 } 247 248 249 250 /** 251 * Sets the value for <b>end</b> () 252 * 253 * <p> 254 * <b>Definition:</b> 255 * The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time 256 * </p> 257 */ 258 public PeriodDt setEndWithSecondsPrecision( Date theDate) { 259 myEnd = new DateTimeDt(theDate); 260 return this; 261 } 262 263 /** 264 * Sets the value for <b>end</b> () 265 * 266 * <p> 267 * <b>Definition:</b> 268 * The end of the period. If the end of the period is missing, it means that the period is ongoing. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time 269 * </p> 270 */ 271 public PeriodDt setEnd( Date theDate, TemporalPrecisionEnum thePrecision) { 272 myEnd = new DateTimeDt(theDate, thePrecision); 273 return this; 274 } 275 276 277 278 279}