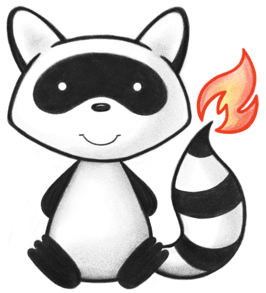
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>RangeDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * A set of ordered Quantities defined by a low and high limit. 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * Need to be able to specify ranges of values 088 * </p> 089 */ 090@DatatypeDef(name="Range") 091public class RangeDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public RangeDt() { 100 // nothing 101 } 102 103 104 @Child(name="low", type=SimpleQuantityDt.class, order=0, min=0, max=1, summary=true, modifier=false) 105 @Description( 106 shortDefinition="", 107 formalDefinition="The low limit. The boundary is inclusive." 108 ) 109 private SimpleQuantityDt myLow; 110 111 @Child(name="high", type=SimpleQuantityDt.class, order=1, min=0, max=1, summary=true, modifier=false) 112 @Description( 113 shortDefinition="", 114 formalDefinition="The high limit. The boundary is inclusive." 115 ) 116 private SimpleQuantityDt myHigh; 117 118 119 @Override 120 public boolean isEmpty() { 121 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myLow, myHigh); 122 } 123 124 @Override 125 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 126 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myLow, myHigh); 127 } 128 129 /** 130 * Gets the value(s) for <b>low</b> (). 131 * creating it if it does 132 * not exist. Will not return <code>null</code>. 133 * 134 * <p> 135 * <b>Definition:</b> 136 * The low limit. The boundary is inclusive. 137 * </p> 138 */ 139 public SimpleQuantityDt getLow() { 140 if (myLow == null) { 141 myLow = new SimpleQuantityDt(); 142 } 143 return myLow; 144 } 145 146 /** 147 * Sets the value(s) for <b>low</b> () 148 * 149 * <p> 150 * <b>Definition:</b> 151 * The low limit. The boundary is inclusive. 152 * </p> 153 */ 154 public RangeDt setLow(SimpleQuantityDt theValue) { 155 myLow = theValue; 156 return this; 157 } 158 159 160 161 162 /** 163 * Gets the value(s) for <b>high</b> (). 164 * creating it if it does 165 * not exist. Will not return <code>null</code>. 166 * 167 * <p> 168 * <b>Definition:</b> 169 * The high limit. The boundary is inclusive. 170 * </p> 171 */ 172 public SimpleQuantityDt getHigh() { 173 if (myHigh == null) { 174 myHigh = new SimpleQuantityDt(); 175 } 176 return myHigh; 177 } 178 179 /** 180 * Sets the value(s) for <b>high</b> () 181 * 182 * <p> 183 * <b>Definition:</b> 184 * The high limit. The boundary is inclusive. 185 * </p> 186 */ 187 public RangeDt setHigh(SimpleQuantityDt theValue) { 188 myHigh = theValue; 189 return this; 190 } 191 192 193 194 195 196 197}