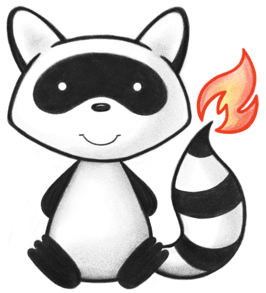
001/* 002 * #%L 003 * HAPI FHIR Structures - DSTU2 (FHIR v1.0.0) 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020 021package ca.uhn.fhir.model.dstu2.composite; 022 023import ca.uhn.fhir.model.api.ICompositeDatatype; 024import ca.uhn.fhir.model.api.IElement; 025import ca.uhn.fhir.model.api.IResource; 026import ca.uhn.fhir.model.api.annotation.Child; 027import ca.uhn.fhir.model.api.annotation.DatatypeDef; 028import ca.uhn.fhir.model.api.annotation.Description; 029import ca.uhn.fhir.model.api.annotation.SimpleSetter; 030import ca.uhn.fhir.model.base.composite.BaseResourceReferenceDt; 031import ca.uhn.fhir.model.primitive.IdDt; 032import ca.uhn.fhir.model.primitive.StringDt; 033import org.hl7.fhir.instance.model.api.IIdType; 034 035import java.util.List; 036 037/** 038 * HAPI/FHIR <b>ResourceReferenceDt</b> Datatype 039 * (A reference from one resource to another) 040 * 041 * <p> 042 * <b>Definition:</b> 043 * A reference from one resource to another 044 * </p> 045 * 046 * <p> 047 * <b>Requirements:</b> 048 * 049 * </p> 050 */ 051@DatatypeDef(name = "reference") 052public class ResourceReferenceDt extends BaseResourceReferenceDt implements ICompositeDatatype { 053 054 /** 055 * Constructor 056 */ 057 public ResourceReferenceDt() { 058 // nothing 059 } 060 061 /** 062 * Constructor which creates a resource reference containing the actual resource in question. 063 * <p> 064 * <b> When using this in a server:</b> Generally if this is serialized, it will be serialized as a contained 065 * resource, so this should not be used if the intent is not to actually supply the referenced resource. This is not 066 * a hard-and-fast rule however, as the server can be configured to not serialized this resource, or to load an ID 067 * and contain even if this constructor is not used. 068 * </p> 069 * 070 * @param theResource 071 * The resource instance 072 */ 073 @SimpleSetter() 074 public ResourceReferenceDt(IResource theResource) { 075 super(theResource); 076 } 077 078 /** 079 * Constructor which accepts a reference directly (this can be an ID, a partial/relative URL or a complete/absolute 080 * URL) 081 * 082 * @param theId 083 * The reference itself 084 */ 085 public ResourceReferenceDt(String theId) { 086 setReference(new IdDt(theId)); 087 } 088 089 /** 090 * Constructor which accepts a reference directly (this can be an ID, a partial/relative URL or a complete/absolute 091 * URL) 092 * 093 * @param theResourceId 094 * The reference itself 095 */ 096 public ResourceReferenceDt(IdDt theResourceId) { 097 setReference(theResourceId); 098 } 099 100 /** 101 * Constructor which accepts a reference directly (this can be an ID, a partial/relative URL or a complete/absolute 102 * URL) 103 * 104 * @param theResourceId 105 * The reference itself 106 */ 107 public ResourceReferenceDt(IIdType theResourceId) { 108 setReference(theResourceId); 109 } 110 111 @Child(name = "reference", type = IdDt.class, order = 0, min = 0, max = 1) 112 @Description( 113 shortDefinition = "Relative, internal or absolute URL reference", 114 formalDefinition = 115 "A reference to a location at which the other resource is found. The reference may a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources") 116 private IdDt myReference; 117 118 @Child(name = "display", type = StringDt.class, order = 1, min = 0, max = 1) 119 @Description( 120 shortDefinition = "Text alternative for the resource", 121 formalDefinition = 122 "Plain text narrative that identifies the resource in addition to the resource reference") 123 private StringDt myDisplay; 124 125 @Override 126 public boolean isEmpty() { 127 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(myReference, myDisplay); 128 } 129 130 @Override 131 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 132 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myReference, myDisplay); 133 } 134 135 /** 136 * Gets the value(s) for <b>reference</b> (Relative, internal or absolute URL reference). 137 * creating it if it does 138 * not exist. Will not return <code>null</code>. 139 * 140 * <p> 141 * <b>Definition:</b> 142 * A reference to a location at which the other resource is found. The reference may a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources 143 * </p> 144 */ 145 @Override 146 public IdDt getReference() { 147 if (myReference == null) { 148 myReference = new IdDt(); 149 } 150 return myReference; 151 } 152 153 @Override 154 public IdDt getReferenceElement() { 155 return getReference(); 156 } 157 158 /** 159 * Sets the value(s) for <b>reference</b> (Relative, internal or absolute URL reference) 160 * 161 * <p> 162 * <b>Definition:</b> 163 * A reference to a location at which the other resource is found. The reference may a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources 164 * </p> 165 */ 166 @Override 167 public ResourceReferenceDt setReference(IdDt theValue) { 168 myReference = theValue; 169 return this; 170 } 171 172 /** 173 * Sets the value for <b>reference</b> (Relative, internal or absolute URL reference) 174 * 175 * <p> 176 * <b>Definition:</b> 177 * A reference to a location at which the other resource is found. The reference may a relative reference, in which case it is relative to the service base URL, or an absolute URL that resolves to the location where the resource is found. The reference may be version specific or not. If the reference is not to a FHIR RESTful server, then it should be assumed to be version specific. Internal fragment references (start with '#') refer to contained resources 178 * </p> 179 */ 180 @Override 181 public ResourceReferenceDt setReference(String theId) { 182 myReference = new IdDt(theId); 183 return this; 184 } 185 186 /** 187 * Gets the value(s) for <b>display</b> (Text alternative for the resource). 188 * creating it if it does 189 * not exist. Will not return <code>null</code>. 190 * 191 * <p> 192 * <b>Definition:</b> 193 * Plain text narrative that identifies the resource in addition to the resource reference 194 * </p> 195 */ 196 public StringDt getDisplay() { 197 if (myDisplay == null) { 198 myDisplay = new StringDt(); 199 } 200 return myDisplay; 201 } 202 203 /** 204 * Sets the value(s) for <b>display</b> (Text alternative for the resource) 205 * 206 * <p> 207 * <b>Definition:</b> 208 * Plain text narrative that identifies the resource in addition to the resource reference 209 * </p> 210 */ 211 public ResourceReferenceDt setDisplay(StringDt theValue) { 212 myDisplay = theValue; 213 return this; 214 } 215 216 /** 217 * Sets the value for <b>display</b> (Text alternative for the resource) 218 * 219 * <p> 220 * <b>Definition:</b> 221 * Plain text narrative that identifies the resource in addition to the resource reference 222 * </p> 223 */ 224 @Override 225 public ResourceReferenceDt setDisplay(String theString) { 226 myDisplay = new StringDt(theString); 227 return this; 228 } 229 230 @Override 231 public StringDt getDisplayElement() { 232 return getDisplay(); 233 } 234}