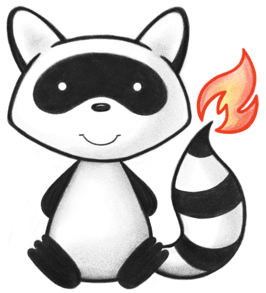
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>SampledDataDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * There is a need for a concise way to handle the data produced by devices that sample a physical state at a high frequency 088 * </p> 089 */ 090@DatatypeDef(name="SampledData") 091public class SampledDataDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public SampledDataDt() { 100 // nothing 101 } 102 103 104 @Child(name="origin", type=SimpleQuantityDt.class, order=0, min=1, max=1, summary=true, modifier=false) 105 @Description( 106 shortDefinition="", 107 formalDefinition="The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series" 108 ) 109 private SimpleQuantityDt myOrigin; 110 111 @Child(name="period", type=DecimalDt.class, order=1, min=1, max=1, summary=true, modifier=false) 112 @Description( 113 shortDefinition="", 114 formalDefinition="The length of time between sampling times, measured in milliseconds" 115 ) 116 private DecimalDt myPeriod; 117 118 @Child(name="factor", type=DecimalDt.class, order=2, min=0, max=1, summary=true, modifier=false) 119 @Description( 120 shortDefinition="", 121 formalDefinition="A correction factor that is applied to the sampled data points before they are added to the origin" 122 ) 123 private DecimalDt myFactor; 124 125 @Child(name="lowerLimit", type=DecimalDt.class, order=3, min=0, max=1, summary=true, modifier=false) 126 @Description( 127 shortDefinition="", 128 formalDefinition="The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit)" 129 ) 130 private DecimalDt myLowerLimit; 131 132 @Child(name="upperLimit", type=DecimalDt.class, order=4, min=0, max=1, summary=true, modifier=false) 133 @Description( 134 shortDefinition="", 135 formalDefinition="The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit)" 136 ) 137 private DecimalDt myUpperLimit; 138 139 @Child(name="dimensions", type=PositiveIntDt.class, order=5, min=1, max=1, summary=true, modifier=false) 140 @Description( 141 shortDefinition="", 142 formalDefinition="The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once" 143 ) 144 private PositiveIntDt myDimensions; 145 146 @Child(name="data", type=StringDt.class, order=6, min=1, max=1, summary=true, modifier=false) 147 @Description( 148 shortDefinition="", 149 formalDefinition="A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value" 150 ) 151 private StringDt myData; 152 153 154 @Override 155 public boolean isEmpty() { 156 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myOrigin, myPeriod, myFactor, myLowerLimit, myUpperLimit, myDimensions, myData); 157 } 158 159 @Override 160 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 161 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myOrigin, myPeriod, myFactor, myLowerLimit, myUpperLimit, myDimensions, myData); 162 } 163 164 /** 165 * Gets the value(s) for <b>origin</b> (). 166 * creating it if it does 167 * not exist. Will not return <code>null</code>. 168 * 169 * <p> 170 * <b>Definition:</b> 171 * The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series 172 * </p> 173 */ 174 public SimpleQuantityDt getOrigin() { 175 if (myOrigin == null) { 176 myOrigin = new SimpleQuantityDt(); 177 } 178 return myOrigin; 179 } 180 181 /** 182 * Sets the value(s) for <b>origin</b> () 183 * 184 * <p> 185 * <b>Definition:</b> 186 * The base quantity that a measured value of zero represents. In addition, this provides the units of the entire measurement series 187 * </p> 188 */ 189 public SampledDataDt setOrigin(SimpleQuantityDt theValue) { 190 myOrigin = theValue; 191 return this; 192 } 193 194 195 196 197 /** 198 * Gets the value(s) for <b>period</b> (). 199 * creating it if it does 200 * not exist. Will not return <code>null</code>. 201 * 202 * <p> 203 * <b>Definition:</b> 204 * The length of time between sampling times, measured in milliseconds 205 * </p> 206 */ 207 public DecimalDt getPeriodElement() { 208 if (myPeriod == null) { 209 myPeriod = new DecimalDt(); 210 } 211 return myPeriod; 212 } 213 214 215 /** 216 * Gets the value(s) for <b>period</b> (). 217 * creating it if it does 218 * not exist. This method may return <code>null</code>. 219 * 220 * <p> 221 * <b>Definition:</b> 222 * The length of time between sampling times, measured in milliseconds 223 * </p> 224 */ 225 public BigDecimal getPeriod() { 226 return getPeriodElement().getValue(); 227 } 228 229 /** 230 * Sets the value(s) for <b>period</b> () 231 * 232 * <p> 233 * <b>Definition:</b> 234 * The length of time between sampling times, measured in milliseconds 235 * </p> 236 */ 237 public SampledDataDt setPeriod(DecimalDt theValue) { 238 myPeriod = theValue; 239 return this; 240 } 241 242 243 244 /** 245 * Sets the value for <b>period</b> () 246 * 247 * <p> 248 * <b>Definition:</b> 249 * The length of time between sampling times, measured in milliseconds 250 * </p> 251 */ 252 public SampledDataDt setPeriod( long theValue) { 253 myPeriod = new DecimalDt(theValue); 254 return this; 255 } 256 257 /** 258 * Sets the value for <b>period</b> () 259 * 260 * <p> 261 * <b>Definition:</b> 262 * The length of time between sampling times, measured in milliseconds 263 * </p> 264 */ 265 public SampledDataDt setPeriod( double theValue) { 266 myPeriod = new DecimalDt(theValue); 267 return this; 268 } 269 270 /** 271 * Sets the value for <b>period</b> () 272 * 273 * <p> 274 * <b>Definition:</b> 275 * The length of time between sampling times, measured in milliseconds 276 * </p> 277 */ 278 public SampledDataDt setPeriod( java.math.BigDecimal theValue) { 279 myPeriod = new DecimalDt(theValue); 280 return this; 281 } 282 283 284 /** 285 * Gets the value(s) for <b>factor</b> (). 286 * creating it if it does 287 * not exist. Will not return <code>null</code>. 288 * 289 * <p> 290 * <b>Definition:</b> 291 * A correction factor that is applied to the sampled data points before they are added to the origin 292 * </p> 293 */ 294 public DecimalDt getFactorElement() { 295 if (myFactor == null) { 296 myFactor = new DecimalDt(); 297 } 298 return myFactor; 299 } 300 301 302 /** 303 * Gets the value(s) for <b>factor</b> (). 304 * creating it if it does 305 * not exist. This method may return <code>null</code>. 306 * 307 * <p> 308 * <b>Definition:</b> 309 * A correction factor that is applied to the sampled data points before they are added to the origin 310 * </p> 311 */ 312 public BigDecimal getFactor() { 313 return getFactorElement().getValue(); 314 } 315 316 /** 317 * Sets the value(s) for <b>factor</b> () 318 * 319 * <p> 320 * <b>Definition:</b> 321 * A correction factor that is applied to the sampled data points before they are added to the origin 322 * </p> 323 */ 324 public SampledDataDt setFactor(DecimalDt theValue) { 325 myFactor = theValue; 326 return this; 327 } 328 329 330 331 /** 332 * Sets the value for <b>factor</b> () 333 * 334 * <p> 335 * <b>Definition:</b> 336 * A correction factor that is applied to the sampled data points before they are added to the origin 337 * </p> 338 */ 339 public SampledDataDt setFactor( long theValue) { 340 myFactor = new DecimalDt(theValue); 341 return this; 342 } 343 344 /** 345 * Sets the value for <b>factor</b> () 346 * 347 * <p> 348 * <b>Definition:</b> 349 * A correction factor that is applied to the sampled data points before they are added to the origin 350 * </p> 351 */ 352 public SampledDataDt setFactor( double theValue) { 353 myFactor = new DecimalDt(theValue); 354 return this; 355 } 356 357 /** 358 * Sets the value for <b>factor</b> () 359 * 360 * <p> 361 * <b>Definition:</b> 362 * A correction factor that is applied to the sampled data points before they are added to the origin 363 * </p> 364 */ 365 public SampledDataDt setFactor( java.math.BigDecimal theValue) { 366 myFactor = new DecimalDt(theValue); 367 return this; 368 } 369 370 371 /** 372 * Gets the value(s) for <b>lowerLimit</b> (). 373 * creating it if it does 374 * not exist. Will not return <code>null</code>. 375 * 376 * <p> 377 * <b>Definition:</b> 378 * The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit) 379 * </p> 380 */ 381 public DecimalDt getLowerLimitElement() { 382 if (myLowerLimit == null) { 383 myLowerLimit = new DecimalDt(); 384 } 385 return myLowerLimit; 386 } 387 388 389 /** 390 * Gets the value(s) for <b>lowerLimit</b> (). 391 * creating it if it does 392 * not exist. This method may return <code>null</code>. 393 * 394 * <p> 395 * <b>Definition:</b> 396 * The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit) 397 * </p> 398 */ 399 public BigDecimal getLowerLimit() { 400 return getLowerLimitElement().getValue(); 401 } 402 403 /** 404 * Sets the value(s) for <b>lowerLimit</b> () 405 * 406 * <p> 407 * <b>Definition:</b> 408 * The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit) 409 * </p> 410 */ 411 public SampledDataDt setLowerLimit(DecimalDt theValue) { 412 myLowerLimit = theValue; 413 return this; 414 } 415 416 417 418 /** 419 * Sets the value for <b>lowerLimit</b> () 420 * 421 * <p> 422 * <b>Definition:</b> 423 * The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit) 424 * </p> 425 */ 426 public SampledDataDt setLowerLimit( long theValue) { 427 myLowerLimit = new DecimalDt(theValue); 428 return this; 429 } 430 431 /** 432 * Sets the value for <b>lowerLimit</b> () 433 * 434 * <p> 435 * <b>Definition:</b> 436 * The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit) 437 * </p> 438 */ 439 public SampledDataDt setLowerLimit( double theValue) { 440 myLowerLimit = new DecimalDt(theValue); 441 return this; 442 } 443 444 /** 445 * Sets the value for <b>lowerLimit</b> () 446 * 447 * <p> 448 * <b>Definition:</b> 449 * The lower limit of detection of the measured points. This is needed if any of the data points have the value \"L\" (lower than detection limit) 450 * </p> 451 */ 452 public SampledDataDt setLowerLimit( java.math.BigDecimal theValue) { 453 myLowerLimit = new DecimalDt(theValue); 454 return this; 455 } 456 457 458 /** 459 * Gets the value(s) for <b>upperLimit</b> (). 460 * creating it if it does 461 * not exist. Will not return <code>null</code>. 462 * 463 * <p> 464 * <b>Definition:</b> 465 * The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit) 466 * </p> 467 */ 468 public DecimalDt getUpperLimitElement() { 469 if (myUpperLimit == null) { 470 myUpperLimit = new DecimalDt(); 471 } 472 return myUpperLimit; 473 } 474 475 476 /** 477 * Gets the value(s) for <b>upperLimit</b> (). 478 * creating it if it does 479 * not exist. This method may return <code>null</code>. 480 * 481 * <p> 482 * <b>Definition:</b> 483 * The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit) 484 * </p> 485 */ 486 public BigDecimal getUpperLimit() { 487 return getUpperLimitElement().getValue(); 488 } 489 490 /** 491 * Sets the value(s) for <b>upperLimit</b> () 492 * 493 * <p> 494 * <b>Definition:</b> 495 * The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit) 496 * </p> 497 */ 498 public SampledDataDt setUpperLimit(DecimalDt theValue) { 499 myUpperLimit = theValue; 500 return this; 501 } 502 503 504 505 /** 506 * Sets the value for <b>upperLimit</b> () 507 * 508 * <p> 509 * <b>Definition:</b> 510 * The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit) 511 * </p> 512 */ 513 public SampledDataDt setUpperLimit( long theValue) { 514 myUpperLimit = new DecimalDt(theValue); 515 return this; 516 } 517 518 /** 519 * Sets the value for <b>upperLimit</b> () 520 * 521 * <p> 522 * <b>Definition:</b> 523 * The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit) 524 * </p> 525 */ 526 public SampledDataDt setUpperLimit( double theValue) { 527 myUpperLimit = new DecimalDt(theValue); 528 return this; 529 } 530 531 /** 532 * Sets the value for <b>upperLimit</b> () 533 * 534 * <p> 535 * <b>Definition:</b> 536 * The upper limit of detection of the measured points. This is needed if any of the data points have the value \"U\" (higher than detection limit) 537 * </p> 538 */ 539 public SampledDataDt setUpperLimit( java.math.BigDecimal theValue) { 540 myUpperLimit = new DecimalDt(theValue); 541 return this; 542 } 543 544 545 /** 546 * Gets the value(s) for <b>dimensions</b> (). 547 * creating it if it does 548 * not exist. Will not return <code>null</code>. 549 * 550 * <p> 551 * <b>Definition:</b> 552 * The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once 553 * </p> 554 */ 555 public PositiveIntDt getDimensionsElement() { 556 if (myDimensions == null) { 557 myDimensions = new PositiveIntDt(); 558 } 559 return myDimensions; 560 } 561 562 563 /** 564 * Gets the value(s) for <b>dimensions</b> (). 565 * creating it if it does 566 * not exist. This method may return <code>null</code>. 567 * 568 * <p> 569 * <b>Definition:</b> 570 * The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once 571 * </p> 572 */ 573 public Integer getDimensions() { 574 return getDimensionsElement().getValue(); 575 } 576 577 /** 578 * Sets the value(s) for <b>dimensions</b> () 579 * 580 * <p> 581 * <b>Definition:</b> 582 * The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once 583 * </p> 584 */ 585 public SampledDataDt setDimensions(PositiveIntDt theValue) { 586 myDimensions = theValue; 587 return this; 588 } 589 590 591 592 /** 593 * Sets the value for <b>dimensions</b> () 594 * 595 * <p> 596 * <b>Definition:</b> 597 * The number of sample points at each time point. If this value is greater than one, then the dimensions will be interlaced - all the sample points for a point in time will be recorded at once 598 * </p> 599 */ 600 public SampledDataDt setDimensions( int theInteger) { 601 myDimensions = new PositiveIntDt(theInteger); 602 return this; 603 } 604 605 606 /** 607 * Gets the value(s) for <b>data</b> (). 608 * creating it if it does 609 * not exist. Will not return <code>null</code>. 610 * 611 * <p> 612 * <b>Definition:</b> 613 * A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value 614 * </p> 615 */ 616 public StringDt getDataElement() { 617 if (myData == null) { 618 myData = new StringDt(); 619 } 620 return myData; 621 } 622 623 624 /** 625 * Gets the value(s) for <b>data</b> (). 626 * creating it if it does 627 * not exist. This method may return <code>null</code>. 628 * 629 * <p> 630 * <b>Definition:</b> 631 * A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value 632 * </p> 633 */ 634 public String getData() { 635 return getDataElement().getValue(); 636 } 637 638 /** 639 * Sets the value(s) for <b>data</b> () 640 * 641 * <p> 642 * <b>Definition:</b> 643 * A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value 644 * </p> 645 */ 646 public SampledDataDt setData(StringDt theValue) { 647 myData = theValue; 648 return this; 649 } 650 651 652 653 /** 654 * Sets the value for <b>data</b> () 655 * 656 * <p> 657 * <b>Definition:</b> 658 * A series of data points which are decimal values separated by a single space (character u20). The special values \"E\" (error), \"L\" (below detection limit) and \"U\" (above detection limit) can also be used in place of a decimal value 659 * </p> 660 */ 661 public SampledDataDt setData( String theString) { 662 myData = new StringDt(theString); 663 return this; 664 } 665 666 667 668 669}