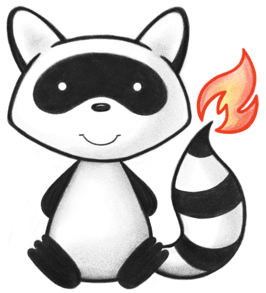
001/* 002 * #%L 003 * HAPI FHIR Structures - DSTU2 (FHIR v1.0.0) 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.dstu2.composite; 021 022import ca.uhn.fhir.model.api.annotation.DatatypeDef; 023import ca.uhn.fhir.model.api.annotation.SimpleSetter; 024import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 025 026@DatatypeDef(name = "SimpleQuantity", profileOf = QuantityDt.class) 027public class SimpleQuantityDt extends QuantityDt { 028 029 private static final long serialVersionUID = 1L; 030 031 /** 032 * Constructor 033 */ 034 public SimpleQuantityDt() { 035 // nothing 036 } 037 038 /** 039 * Constructor 040 */ 041 @SimpleSetter 042 public SimpleQuantityDt(@SimpleSetter.Parameter(name = "theValue") double theValue) { 043 setValue(theValue); 044 } 045 046 /** 047 * Constructor 048 */ 049 @SimpleSetter 050 public SimpleQuantityDt(@SimpleSetter.Parameter(name = "theValue") long theValue) { 051 setValue(theValue); 052 } 053 054 /** 055 * Constructor 056 */ 057 @SimpleSetter 058 public SimpleQuantityDt( 059 @SimpleSetter.Parameter(name = "theComparator") QuantityComparatorEnum theComparator, 060 @SimpleSetter.Parameter(name = "theValue") double theValue, 061 @SimpleSetter.Parameter(name = "theUnits") String theUnits) { 062 setValue(theValue); 063 setComparator(theComparator); 064 setUnit(theUnits); 065 } 066 067 /** 068 * Constructor 069 */ 070 @SimpleSetter 071 public SimpleQuantityDt( 072 @SimpleSetter.Parameter(name = "theComparator") QuantityComparatorEnum theComparator, 073 @SimpleSetter.Parameter(name = "theValue") long theValue, 074 @SimpleSetter.Parameter(name = "theUnits") String theUnits) { 075 setValue(theValue); 076 setComparator(theComparator); 077 setUnit(theUnits); 078 } 079 080 /** 081 * Constructor 082 */ 083 @SimpleSetter 084 public SimpleQuantityDt( 085 @SimpleSetter.Parameter(name = "theValue") double theValue, 086 @SimpleSetter.Parameter(name = "theSystem") String theSystem, 087 @SimpleSetter.Parameter(name = "theUnits") String theUnits) { 088 setValue(theValue); 089 setSystem(theSystem); 090 setUnit(theUnits); 091 } 092 093 /** 094 * Constructor 095 */ 096 @SimpleSetter 097 public SimpleQuantityDt( 098 @SimpleSetter.Parameter(name = "theValue") long theValue, 099 @SimpleSetter.Parameter(name = "theSystem") String theSystem, 100 @SimpleSetter.Parameter(name = "theUnits") String theUnits) { 101 setValue(theValue); 102 setSystem(theSystem); 103 setUnit(theUnits); 104 } 105}