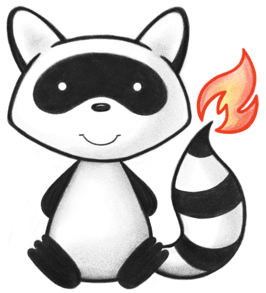
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>TimingDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * Specifies an event that may occur multiple times. Timing schedules are used to record when things are expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * Need to able to track proposed timing schedules. There are several different ways to do this: one or more specified times, a simple rules like three times a day, or before/after meals 088 * </p> 089 */ 090@DatatypeDef(name="Timing") 091public class TimingDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public TimingDt() { 100 // nothing 101 } 102 103 104 @Child(name="event", type=DateTimeDt.class, order=0, min=0, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 105 @Description( 106 shortDefinition="", 107 formalDefinition="Identifies specific times when the event occurs" 108 ) 109 private java.util.List<DateTimeDt> myEvent; 110 111 @Child(name="repeat", order=1, min=0, max=1, summary=true, modifier=false) 112 @Description( 113 shortDefinition="", 114 formalDefinition="A set of rules that describe when the event should occur" 115 ) 116 private Repeat myRepeat; 117 118 @Child(name="code", type=CodeableConceptDt.class, order=2, min=0, max=1, summary=true, modifier=false) 119 @Description( 120 shortDefinition="", 121 formalDefinition="A code for the timing pattern. Some codes such as BID are ubiquitous, but many institutions define their own additional codes" 122 ) 123 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/timing-abbreviation") 124 private BoundCodeableConceptDt<TimingAbbreviationEnum> myCode; 125 126 127 @Override 128 public boolean isEmpty() { 129 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myEvent, myRepeat, myCode); 130 } 131 132 @Override 133 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 134 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myEvent, myRepeat, myCode); 135 } 136 137 /** 138 * Gets the value(s) for <b>event</b> (). 139 * creating it if it does 140 * not exist. Will not return <code>null</code>. 141 * 142 * <p> 143 * <b>Definition:</b> 144 * Identifies specific times when the event occurs 145 * </p> 146 */ 147 public java.util.List<DateTimeDt> getEvent() { 148 if (myEvent == null) { 149 myEvent = new java.util.ArrayList<DateTimeDt>(); 150 } 151 return myEvent; 152 } 153 154 /** 155 * Sets the value(s) for <b>event</b> () 156 * 157 * <p> 158 * <b>Definition:</b> 159 * Identifies specific times when the event occurs 160 * </p> 161 */ 162 public TimingDt setEvent(java.util.List<DateTimeDt> theValue) { 163 myEvent = theValue; 164 return this; 165 } 166 167 168 169 /** 170 * Adds and returns a new value for <b>event</b> () 171 * 172 * <p> 173 * <b>Definition:</b> 174 * Identifies specific times when the event occurs 175 * </p> 176 */ 177 public DateTimeDt addEvent() { 178 DateTimeDt newType = new DateTimeDt(); 179 getEvent().add(newType); 180 return newType; 181 } 182 183 /** 184 * Adds a given new value for <b>event</b> () 185 * 186 * <p> 187 * <b>Definition:</b> 188 * Identifies specific times when the event occurs 189 * </p> 190 * @param theValue The event to add (must not be <code>null</code>) 191 */ 192 public TimingDt addEvent(DateTimeDt theValue) { 193 if (theValue == null) { 194 throw new NullPointerException("theValue must not be null"); 195 } 196 getEvent().add(theValue); 197 return this; 198 } 199 200 /** 201 * Gets the first repetition for <b>event</b> (), 202 * creating it if it does not already exist. 203 * 204 * <p> 205 * <b>Definition:</b> 206 * Identifies specific times when the event occurs 207 * </p> 208 */ 209 public DateTimeDt getEventFirstRep() { 210 if (getEvent().isEmpty()) { 211 return addEvent(); 212 } 213 return getEvent().get(0); 214 } 215 /** 216 * Adds a new value for <b>event</b> () 217 * 218 * <p> 219 * <b>Definition:</b> 220 * Identifies specific times when the event occurs 221 * </p> 222 * 223 * @return Returns a reference to this object, to allow for simple chaining. 224 */ 225 public TimingDt addEvent( Date theDate) { 226 if (myEvent == null) { 227 myEvent = new java.util.ArrayList<DateTimeDt>(); 228 } 229 myEvent.add(new DateTimeDt(theDate)); 230 return this; 231 } 232 233 /** 234 * Adds a new value for <b>event</b> () 235 * 236 * <p> 237 * <b>Definition:</b> 238 * Identifies specific times when the event occurs 239 * </p> 240 * 241 * @return Returns a reference to this object, to allow for simple chaining. 242 */ 243 public TimingDt addEvent( Date theDate, TemporalPrecisionEnum thePrecision) { 244 if (myEvent == null) { 245 myEvent = new java.util.ArrayList<DateTimeDt>(); 246 } 247 myEvent.add(new DateTimeDt(theDate, thePrecision)); 248 return this; 249 } 250 251 252 /** 253 * Gets the value(s) for <b>repeat</b> (). 254 * creating it if it does 255 * not exist. Will not return <code>null</code>. 256 * 257 * <p> 258 * <b>Definition:</b> 259 * A set of rules that describe when the event should occur 260 * </p> 261 */ 262 public Repeat getRepeat() { 263 if (myRepeat == null) { 264 myRepeat = new Repeat(); 265 } 266 return myRepeat; 267 } 268 269 /** 270 * Sets the value(s) for <b>repeat</b> () 271 * 272 * <p> 273 * <b>Definition:</b> 274 * A set of rules that describe when the event should occur 275 * </p> 276 */ 277 public TimingDt setRepeat(Repeat theValue) { 278 myRepeat = theValue; 279 return this; 280 } 281 282 283 284 285 /** 286 * Gets the value(s) for <b>code</b> (). 287 * creating it if it does 288 * not exist. Will not return <code>null</code>. 289 * 290 * <p> 291 * <b>Definition:</b> 292 * A code for the timing pattern. Some codes such as BID are ubiquitous, but many institutions define their own additional codes 293 * </p> 294 */ 295 public BoundCodeableConceptDt<TimingAbbreviationEnum> getCode() { 296 if (myCode == null) { 297 myCode = new BoundCodeableConceptDt<TimingAbbreviationEnum>(TimingAbbreviationEnum.VALUESET_BINDER); 298 } 299 return myCode; 300 } 301 302 /** 303 * Sets the value(s) for <b>code</b> () 304 * 305 * <p> 306 * <b>Definition:</b> 307 * A code for the timing pattern. Some codes such as BID are ubiquitous, but many institutions define their own additional codes 308 * </p> 309 */ 310 public TimingDt setCode(BoundCodeableConceptDt<TimingAbbreviationEnum> theValue) { 311 myCode = theValue; 312 return this; 313 } 314 315 316 317 /** 318 * Sets the value(s) for <b>code</b> () 319 * 320 * <p> 321 * <b>Definition:</b> 322 * A code for the timing pattern. Some codes such as BID are ubiquitous, but many institutions define their own additional codes 323 * </p> 324 */ 325 public TimingDt setCode(TimingAbbreviationEnum theValue) { 326 setCode(new BoundCodeableConceptDt<TimingAbbreviationEnum>(TimingAbbreviationEnum.VALUESET_BINDER, theValue)); 327 328/* 329 getCode().setValueAsEnum(theValue); 330*/ 331 return this; 332 } 333 334 335 /** 336 * Block class for child element: <b>Timing.repeat</b> () 337 * 338 * <p> 339 * <b>Definition:</b> 340 * A set of rules that describe when the event should occur 341 * </p> 342 */ 343 @Block() 344 public static class Repeat 345 extends BaseIdentifiableElement 346 implements IResourceBlock { 347 348 @Child(name="bounds", order=0, min=0, max=1, summary=true, modifier=false, type={ 349 DurationDt.class, 350 RangeDt.class, 351 PeriodDt.class 352 }) 353 @Description( 354 shortDefinition="", 355 formalDefinition="Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule" 356 ) 357 private IDatatype myBounds; 358 359 @Child(name="count", type=IntegerDt.class, order=1, min=0, max=1, summary=true, modifier=false) 360 @Description( 361 shortDefinition="", 362 formalDefinition="A total count of the desired number of repetitions" 363 ) 364 private IntegerDt myCount; 365 366 @Child(name="duration", type=DecimalDt.class, order=2, min=0, max=1, summary=true, modifier=false) 367 @Description( 368 shortDefinition="", 369 formalDefinition="How long this thing happens for when it happens" 370 ) 371 private DecimalDt myDuration; 372 373 @Child(name="durationMax", type=DecimalDt.class, order=3, min=0, max=1, summary=true, modifier=false) 374 @Description( 375 shortDefinition="", 376 formalDefinition="The upper limit of how long this thing happens for when it happens" 377 ) 378 private DecimalDt myDurationMax; 379 380 @Child(name="durationUnits", type=CodeDt.class, order=4, min=0, max=1, summary=true, modifier=false) 381 @Description( 382 shortDefinition="", 383 formalDefinition="The units of time for the duration, in UCUM units" 384 ) 385 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/units-of-time") 386 private BoundCodeDt<UnitsOfTimeEnum> myDurationUnits; 387 388 @Child(name="frequency", type=IntegerDt.class, order=5, min=0, max=1, summary=true, modifier=false) 389 @Description( 390 shortDefinition="", 391 formalDefinition="The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided)" 392 ) 393 private IntegerDt myFrequency; 394 395 @Child(name="frequencyMax", type=IntegerDt.class, order=6, min=0, max=1, summary=true, modifier=false) 396 @Description( 397 shortDefinition="", 398 formalDefinition="" 399 ) 400 private IntegerDt myFrequencyMax; 401 402 @Child(name="period", type=DecimalDt.class, order=7, min=0, max=1, summary=true, modifier=false) 403 @Description( 404 shortDefinition="", 405 formalDefinition="Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period" 406 ) 407 private DecimalDt myPeriod; 408 409 @Child(name="periodMax", type=DecimalDt.class, order=8, min=0, max=1, summary=true, modifier=false) 410 @Description( 411 shortDefinition="", 412 formalDefinition="If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days" 413 ) 414 private DecimalDt myPeriodMax; 415 416 @Child(name="periodUnits", type=CodeDt.class, order=9, min=0, max=1, summary=true, modifier=false) 417 @Description( 418 shortDefinition="", 419 formalDefinition="The units of time for the period in UCUM units" 420 ) 421 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/units-of-time") 422 private BoundCodeDt<UnitsOfTimeEnum> myPeriodUnits; 423 424 @Child(name="when", type=CodeDt.class, order=10, min=0, max=1, summary=true, modifier=false) 425 @Description( 426 shortDefinition="", 427 formalDefinition="A real world event that the occurrence of the event should be tied to." 428 ) 429 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/event-timing") 430 private BoundCodeDt<EventTimingEnum> myWhen; 431 432 433 @Override 434 public boolean isEmpty() { 435 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myBounds, myCount, myDuration, myDurationMax, myDurationUnits, myFrequency, myFrequencyMax, myPeriod, myPeriodMax, myPeriodUnits, myWhen); 436 } 437 438 @Override 439 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 440 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myBounds, myCount, myDuration, myDurationMax, myDurationUnits, myFrequency, myFrequencyMax, myPeriod, myPeriodMax, myPeriodUnits, myWhen); 441 } 442 443 /** 444 * Gets the value(s) for <b>bounds[x]</b> (). 445 * creating it if it does 446 * not exist. Will not return <code>null</code>. 447 * 448 * <p> 449 * <b>Definition:</b> 450 * Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule 451 * </p> 452 */ 453 public IDatatype getBounds() { 454 return myBounds; 455 } 456 457 /** 458 * Sets the value(s) for <b>bounds[x]</b> () 459 * 460 * <p> 461 * <b>Definition:</b> 462 * Either a duration for the length of the timing schedule, a range of possible length, or outer bounds for start and/or end limits of the timing schedule 463 * </p> 464 */ 465 public Repeat setBounds(IDatatype theValue) { 466 myBounds = theValue; 467 return this; 468 } 469 470 471 472 473 /** 474 * Gets the value(s) for <b>count</b> (). 475 * creating it if it does 476 * not exist. Will not return <code>null</code>. 477 * 478 * <p> 479 * <b>Definition:</b> 480 * A total count of the desired number of repetitions 481 * </p> 482 */ 483 public IntegerDt getCountElement() { 484 if (myCount == null) { 485 myCount = new IntegerDt(); 486 } 487 return myCount; 488 } 489 490 491 /** 492 * Gets the value(s) for <b>count</b> (). 493 * creating it if it does 494 * not exist. This method may return <code>null</code>. 495 * 496 * <p> 497 * <b>Definition:</b> 498 * A total count of the desired number of repetitions 499 * </p> 500 */ 501 public Integer getCount() { 502 return getCountElement().getValue(); 503 } 504 505 /** 506 * Sets the value(s) for <b>count</b> () 507 * 508 * <p> 509 * <b>Definition:</b> 510 * A total count of the desired number of repetitions 511 * </p> 512 */ 513 public Repeat setCount(IntegerDt theValue) { 514 myCount = theValue; 515 return this; 516 } 517 518 519 520 /** 521 * Sets the value for <b>count</b> () 522 * 523 * <p> 524 * <b>Definition:</b> 525 * A total count of the desired number of repetitions 526 * </p> 527 */ 528 public Repeat setCount( int theInteger) { 529 myCount = new IntegerDt(theInteger); 530 return this; 531 } 532 533 534 /** 535 * Gets the value(s) for <b>duration</b> (). 536 * creating it if it does 537 * not exist. Will not return <code>null</code>. 538 * 539 * <p> 540 * <b>Definition:</b> 541 * How long this thing happens for when it happens 542 * </p> 543 */ 544 public DecimalDt getDurationElement() { 545 if (myDuration == null) { 546 myDuration = new DecimalDt(); 547 } 548 return myDuration; 549 } 550 551 552 /** 553 * Gets the value(s) for <b>duration</b> (). 554 * creating it if it does 555 * not exist. This method may return <code>null</code>. 556 * 557 * <p> 558 * <b>Definition:</b> 559 * How long this thing happens for when it happens 560 * </p> 561 */ 562 public BigDecimal getDuration() { 563 return getDurationElement().getValue(); 564 } 565 566 /** 567 * Sets the value(s) for <b>duration</b> () 568 * 569 * <p> 570 * <b>Definition:</b> 571 * How long this thing happens for when it happens 572 * </p> 573 */ 574 public Repeat setDuration(DecimalDt theValue) { 575 myDuration = theValue; 576 return this; 577 } 578 579 580 581 /** 582 * Sets the value for <b>duration</b> () 583 * 584 * <p> 585 * <b>Definition:</b> 586 * How long this thing happens for when it happens 587 * </p> 588 */ 589 public Repeat setDuration( java.math.BigDecimal theValue) { 590 myDuration = new DecimalDt(theValue); 591 return this; 592 } 593 594 /** 595 * Sets the value for <b>duration</b> () 596 * 597 * <p> 598 * <b>Definition:</b> 599 * How long this thing happens for when it happens 600 * </p> 601 */ 602 public Repeat setDuration( long theValue) { 603 myDuration = new DecimalDt(theValue); 604 return this; 605 } 606 607 /** 608 * Sets the value for <b>duration</b> () 609 * 610 * <p> 611 * <b>Definition:</b> 612 * How long this thing happens for when it happens 613 * </p> 614 */ 615 public Repeat setDuration( double theValue) { 616 myDuration = new DecimalDt(theValue); 617 return this; 618 } 619 620 621 /** 622 * Gets the value(s) for <b>durationMax</b> (). 623 * creating it if it does 624 * not exist. Will not return <code>null</code>. 625 * 626 * <p> 627 * <b>Definition:</b> 628 * The upper limit of how long this thing happens for when it happens 629 * </p> 630 */ 631 public DecimalDt getDurationMaxElement() { 632 if (myDurationMax == null) { 633 myDurationMax = new DecimalDt(); 634 } 635 return myDurationMax; 636 } 637 638 639 /** 640 * Gets the value(s) for <b>durationMax</b> (). 641 * creating it if it does 642 * not exist. This method may return <code>null</code>. 643 * 644 * <p> 645 * <b>Definition:</b> 646 * The upper limit of how long this thing happens for when it happens 647 * </p> 648 */ 649 public BigDecimal getDurationMax() { 650 return getDurationMaxElement().getValue(); 651 } 652 653 /** 654 * Sets the value(s) for <b>durationMax</b> () 655 * 656 * <p> 657 * <b>Definition:</b> 658 * The upper limit of how long this thing happens for when it happens 659 * </p> 660 */ 661 public Repeat setDurationMax(DecimalDt theValue) { 662 myDurationMax = theValue; 663 return this; 664 } 665 666 667 668 /** 669 * Sets the value for <b>durationMax</b> () 670 * 671 * <p> 672 * <b>Definition:</b> 673 * The upper limit of how long this thing happens for when it happens 674 * </p> 675 */ 676 public Repeat setDurationMax( java.math.BigDecimal theValue) { 677 myDurationMax = new DecimalDt(theValue); 678 return this; 679 } 680 681 /** 682 * Sets the value for <b>durationMax</b> () 683 * 684 * <p> 685 * <b>Definition:</b> 686 * The upper limit of how long this thing happens for when it happens 687 * </p> 688 */ 689 public Repeat setDurationMax( long theValue) { 690 myDurationMax = new DecimalDt(theValue); 691 return this; 692 } 693 694 /** 695 * Sets the value for <b>durationMax</b> () 696 * 697 * <p> 698 * <b>Definition:</b> 699 * The upper limit of how long this thing happens for when it happens 700 * </p> 701 */ 702 public Repeat setDurationMax( double theValue) { 703 myDurationMax = new DecimalDt(theValue); 704 return this; 705 } 706 707 708 /** 709 * Gets the value(s) for <b>durationUnits</b> (). 710 * creating it if it does 711 * not exist. Will not return <code>null</code>. 712 * 713 * <p> 714 * <b>Definition:</b> 715 * The units of time for the duration, in UCUM units 716 * </p> 717 */ 718 public BoundCodeDt<UnitsOfTimeEnum> getDurationUnitsElement() { 719 if (myDurationUnits == null) { 720 myDurationUnits = new BoundCodeDt<UnitsOfTimeEnum>(UnitsOfTimeEnum.VALUESET_BINDER); 721 } 722 return myDurationUnits; 723 } 724 725 726 /** 727 * Gets the value(s) for <b>durationUnits</b> (). 728 * creating it if it does 729 * not exist. This method may return <code>null</code>. 730 * 731 * <p> 732 * <b>Definition:</b> 733 * The units of time for the duration, in UCUM units 734 * </p> 735 */ 736 public String getDurationUnits() { 737 return getDurationUnitsElement().getValue(); 738 } 739 740 /** 741 * Sets the value(s) for <b>durationUnits</b> () 742 * 743 * <p> 744 * <b>Definition:</b> 745 * The units of time for the duration, in UCUM units 746 * </p> 747 */ 748 public Repeat setDurationUnits(BoundCodeDt<UnitsOfTimeEnum> theValue) { 749 myDurationUnits = theValue; 750 return this; 751 } 752 753 754 755 /** 756 * Sets the value(s) for <b>durationUnits</b> () 757 * 758 * <p> 759 * <b>Definition:</b> 760 * The units of time for the duration, in UCUM units 761 * </p> 762 */ 763 public Repeat setDurationUnits(UnitsOfTimeEnum theValue) { 764 setDurationUnits(new BoundCodeDt<UnitsOfTimeEnum>(UnitsOfTimeEnum.VALUESET_BINDER, theValue)); 765 766/* 767 getDurationUnitsElement().setValueAsEnum(theValue); 768*/ 769 return this; 770 } 771 772 773 /** 774 * Gets the value(s) for <b>frequency</b> (). 775 * creating it if it does 776 * not exist. Will not return <code>null</code>. 777 * 778 * <p> 779 * <b>Definition:</b> 780 * The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided) 781 * </p> 782 */ 783 public IntegerDt getFrequencyElement() { 784 if (myFrequency == null) { 785 myFrequency = new IntegerDt(); 786 } 787 return myFrequency; 788 } 789 790 791 /** 792 * Gets the value(s) for <b>frequency</b> (). 793 * creating it if it does 794 * not exist. This method may return <code>null</code>. 795 * 796 * <p> 797 * <b>Definition:</b> 798 * The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided) 799 * </p> 800 */ 801 public Integer getFrequency() { 802 return getFrequencyElement().getValue(); 803 } 804 805 /** 806 * Sets the value(s) for <b>frequency</b> () 807 * 808 * <p> 809 * <b>Definition:</b> 810 * The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided) 811 * </p> 812 */ 813 public Repeat setFrequency(IntegerDt theValue) { 814 myFrequency = theValue; 815 return this; 816 } 817 818 819 820 /** 821 * Sets the value for <b>frequency</b> () 822 * 823 * <p> 824 * <b>Definition:</b> 825 * The number of times to repeat the action within the specified period / period range (i.e. both period and periodMax provided) 826 * </p> 827 */ 828 public Repeat setFrequency( int theInteger) { 829 myFrequency = new IntegerDt(theInteger); 830 return this; 831 } 832 833 834 /** 835 * Gets the value(s) for <b>frequencyMax</b> (). 836 * creating it if it does 837 * not exist. Will not return <code>null</code>. 838 * 839 * <p> 840 * <b>Definition:</b> 841 * 842 * </p> 843 */ 844 public IntegerDt getFrequencyMaxElement() { 845 if (myFrequencyMax == null) { 846 myFrequencyMax = new IntegerDt(); 847 } 848 return myFrequencyMax; 849 } 850 851 852 /** 853 * Gets the value(s) for <b>frequencyMax</b> (). 854 * creating it if it does 855 * not exist. This method may return <code>null</code>. 856 * 857 * <p> 858 * <b>Definition:</b> 859 * 860 * </p> 861 */ 862 public Integer getFrequencyMax() { 863 return getFrequencyMaxElement().getValue(); 864 } 865 866 /** 867 * Sets the value(s) for <b>frequencyMax</b> () 868 * 869 * <p> 870 * <b>Definition:</b> 871 * 872 * </p> 873 */ 874 public Repeat setFrequencyMax(IntegerDt theValue) { 875 myFrequencyMax = theValue; 876 return this; 877 } 878 879 880 881 /** 882 * Sets the value for <b>frequencyMax</b> () 883 * 884 * <p> 885 * <b>Definition:</b> 886 * 887 * </p> 888 */ 889 public Repeat setFrequencyMax( int theInteger) { 890 myFrequencyMax = new IntegerDt(theInteger); 891 return this; 892 } 893 894 895 /** 896 * Gets the value(s) for <b>period</b> (). 897 * creating it if it does 898 * not exist. Will not return <code>null</code>. 899 * 900 * <p> 901 * <b>Definition:</b> 902 * Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period 903 * </p> 904 */ 905 public DecimalDt getPeriodElement() { 906 if (myPeriod == null) { 907 myPeriod = new DecimalDt(); 908 } 909 return myPeriod; 910 } 911 912 913 /** 914 * Gets the value(s) for <b>period</b> (). 915 * creating it if it does 916 * not exist. This method may return <code>null</code>. 917 * 918 * <p> 919 * <b>Definition:</b> 920 * Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period 921 * </p> 922 */ 923 public BigDecimal getPeriod() { 924 return getPeriodElement().getValue(); 925 } 926 927 /** 928 * Sets the value(s) for <b>period</b> () 929 * 930 * <p> 931 * <b>Definition:</b> 932 * Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period 933 * </p> 934 */ 935 public Repeat setPeriod(DecimalDt theValue) { 936 myPeriod = theValue; 937 return this; 938 } 939 940 941 942 /** 943 * Sets the value for <b>period</b> () 944 * 945 * <p> 946 * <b>Definition:</b> 947 * Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period 948 * </p> 949 */ 950 public Repeat setPeriod( java.math.BigDecimal theValue) { 951 myPeriod = new DecimalDt(theValue); 952 return this; 953 } 954 955 /** 956 * Sets the value for <b>period</b> () 957 * 958 * <p> 959 * <b>Definition:</b> 960 * Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period 961 * </p> 962 */ 963 public Repeat setPeriod( long theValue) { 964 myPeriod = new DecimalDt(theValue); 965 return this; 966 } 967 968 /** 969 * Sets the value for <b>period</b> () 970 * 971 * <p> 972 * <b>Definition:</b> 973 * Indicates the duration of time over which repetitions are to occur; e.g. to express \"3 times per day\", 3 would be the frequency and \"1 day\" would be the period 974 * </p> 975 */ 976 public Repeat setPeriod( double theValue) { 977 myPeriod = new DecimalDt(theValue); 978 return this; 979 } 980 981 982 /** 983 * Gets the value(s) for <b>periodMax</b> (). 984 * creating it if it does 985 * not exist. Will not return <code>null</code>. 986 * 987 * <p> 988 * <b>Definition:</b> 989 * If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days 990 * </p> 991 */ 992 public DecimalDt getPeriodMaxElement() { 993 if (myPeriodMax == null) { 994 myPeriodMax = new DecimalDt(); 995 } 996 return myPeriodMax; 997 } 998 999 1000 /** 1001 * Gets the value(s) for <b>periodMax</b> (). 1002 * creating it if it does 1003 * not exist. This method may return <code>null</code>. 1004 * 1005 * <p> 1006 * <b>Definition:</b> 1007 * If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days 1008 * </p> 1009 */ 1010 public BigDecimal getPeriodMax() { 1011 return getPeriodMaxElement().getValue(); 1012 } 1013 1014 /** 1015 * Sets the value(s) for <b>periodMax</b> () 1016 * 1017 * <p> 1018 * <b>Definition:</b> 1019 * If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days 1020 * </p> 1021 */ 1022 public Repeat setPeriodMax(DecimalDt theValue) { 1023 myPeriodMax = theValue; 1024 return this; 1025 } 1026 1027 1028 1029 /** 1030 * Sets the value for <b>periodMax</b> () 1031 * 1032 * <p> 1033 * <b>Definition:</b> 1034 * If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days 1035 * </p> 1036 */ 1037 public Repeat setPeriodMax( java.math.BigDecimal theValue) { 1038 myPeriodMax = new DecimalDt(theValue); 1039 return this; 1040 } 1041 1042 /** 1043 * Sets the value for <b>periodMax</b> () 1044 * 1045 * <p> 1046 * <b>Definition:</b> 1047 * If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days 1048 * </p> 1049 */ 1050 public Repeat setPeriodMax( long theValue) { 1051 myPeriodMax = new DecimalDt(theValue); 1052 return this; 1053 } 1054 1055 /** 1056 * Sets the value for <b>periodMax</b> () 1057 * 1058 * <p> 1059 * <b>Definition:</b> 1060 * If present, indicates that the period is a range from [period] to [periodMax], allowing expressing concepts such as \"do this once every 3-5 days 1061 * </p> 1062 */ 1063 public Repeat setPeriodMax( double theValue) { 1064 myPeriodMax = new DecimalDt(theValue); 1065 return this; 1066 } 1067 1068 1069 /** 1070 * Gets the value(s) for <b>periodUnits</b> (). 1071 * creating it if it does 1072 * not exist. Will not return <code>null</code>. 1073 * 1074 * <p> 1075 * <b>Definition:</b> 1076 * The units of time for the period in UCUM units 1077 * </p> 1078 */ 1079 public BoundCodeDt<UnitsOfTimeEnum> getPeriodUnitsElement() { 1080 if (myPeriodUnits == null) { 1081 myPeriodUnits = new BoundCodeDt<UnitsOfTimeEnum>(UnitsOfTimeEnum.VALUESET_BINDER); 1082 } 1083 return myPeriodUnits; 1084 } 1085 1086 1087 /** 1088 * Gets the value(s) for <b>periodUnits</b> (). 1089 * creating it if it does 1090 * not exist. This method may return <code>null</code>. 1091 * 1092 * <p> 1093 * <b>Definition:</b> 1094 * The units of time for the period in UCUM units 1095 * </p> 1096 */ 1097 public String getPeriodUnits() { 1098 return getPeriodUnitsElement().getValue(); 1099 } 1100 1101 /** 1102 * Sets the value(s) for <b>periodUnits</b> () 1103 * 1104 * <p> 1105 * <b>Definition:</b> 1106 * The units of time for the period in UCUM units 1107 * </p> 1108 */ 1109 public Repeat setPeriodUnits(BoundCodeDt<UnitsOfTimeEnum> theValue) { 1110 myPeriodUnits = theValue; 1111 return this; 1112 } 1113 1114 1115 1116 /** 1117 * Sets the value(s) for <b>periodUnits</b> () 1118 * 1119 * <p> 1120 * <b>Definition:</b> 1121 * The units of time for the period in UCUM units 1122 * </p> 1123 */ 1124 public Repeat setPeriodUnits(UnitsOfTimeEnum theValue) { 1125 setPeriodUnits(new BoundCodeDt<UnitsOfTimeEnum>(UnitsOfTimeEnum.VALUESET_BINDER, theValue)); 1126 1127/* 1128 getPeriodUnitsElement().setValueAsEnum(theValue); 1129*/ 1130 return this; 1131 } 1132 1133 1134 /** 1135 * Gets the value(s) for <b>when</b> (). 1136 * creating it if it does 1137 * not exist. Will not return <code>null</code>. 1138 * 1139 * <p> 1140 * <b>Definition:</b> 1141 * A real world event that the occurrence of the event should be tied to. 1142 * </p> 1143 */ 1144 public BoundCodeDt<EventTimingEnum> getWhenElement() { 1145 if (myWhen == null) { 1146 myWhen = new BoundCodeDt<EventTimingEnum>(EventTimingEnum.VALUESET_BINDER); 1147 } 1148 return myWhen; 1149 } 1150 1151 1152 /** 1153 * Gets the value(s) for <b>when</b> (). 1154 * creating it if it does 1155 * not exist. This method may return <code>null</code>. 1156 * 1157 * <p> 1158 * <b>Definition:</b> 1159 * A real world event that the occurrence of the event should be tied to. 1160 * </p> 1161 */ 1162 public String getWhen() { 1163 return getWhenElement().getValue(); 1164 } 1165 1166 /** 1167 * Sets the value(s) for <b>when</b> () 1168 * 1169 * <p> 1170 * <b>Definition:</b> 1171 * A real world event that the occurrence of the event should be tied to. 1172 * </p> 1173 */ 1174 public Repeat setWhen(BoundCodeDt<EventTimingEnum> theValue) { 1175 myWhen = theValue; 1176 return this; 1177 } 1178 1179 1180 1181 /** 1182 * Sets the value(s) for <b>when</b> () 1183 * 1184 * <p> 1185 * <b>Definition:</b> 1186 * A real world event that the occurrence of the event should be tied to. 1187 * </p> 1188 */ 1189 public Repeat setWhen(EventTimingEnum theValue) { 1190 setWhen(new BoundCodeDt<EventTimingEnum>(EventTimingEnum.VALUESET_BINDER, theValue)); 1191 1192/* 1193 getWhenElement().setValueAsEnum(theValue); 1194*/ 1195 return this; 1196 } 1197 1198 1199 1200 1201 } 1202 1203 1204 1205 1206}