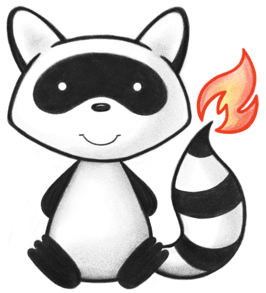
001/* 002 * #%L 003 * HAPI FHIR Structures - DSTU2 (FHIR v1.0.0) 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.model.dstu2.resource; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.model.api.BaseElement; 024import ca.uhn.fhir.model.api.IResource; 025import ca.uhn.fhir.model.api.ResourceMetadataKeyEnum; 026import ca.uhn.fhir.model.api.Tag; 027import ca.uhn.fhir.model.api.TagList; 028import ca.uhn.fhir.model.api.annotation.Child; 029import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 030import ca.uhn.fhir.model.base.composite.BaseCodingDt; 031import ca.uhn.fhir.model.base.resource.ResourceMetadataMap; 032import ca.uhn.fhir.model.dstu2.composite.CodingDt; 033import ca.uhn.fhir.model.dstu2.composite.ContainedDt; 034import ca.uhn.fhir.model.dstu2.composite.NarrativeDt; 035import ca.uhn.fhir.model.primitive.CodeDt; 036import ca.uhn.fhir.model.primitive.IdDt; 037import ca.uhn.fhir.model.primitive.InstantDt; 038import ca.uhn.fhir.rest.gclient.StringClientParam; 039import ca.uhn.fhir.util.ElementUtil; 040import org.apache.commons.lang3.Validate; 041import org.apache.commons.lang3.builder.ToStringBuilder; 042import org.apache.commons.lang3.builder.ToStringStyle; 043import org.hl7.fhir.instance.model.api.IBaseCoding; 044import org.hl7.fhir.instance.model.api.IBaseMetaType; 045import org.hl7.fhir.instance.model.api.IIdType; 046import org.hl7.fhir.instance.model.api.IPrimitiveType; 047 048import java.util.ArrayList; 049import java.util.Collections; 050import java.util.Date; 051import java.util.List; 052 053public abstract class BaseResource extends BaseElement implements IResource { 054 055 /** 056 * <b>Fluent Client</b> search parameter constant for <b>_id</b> 057 * <p> 058 * Description: <b>the _id of a resource</b><br> 059 * Type: <b>string</b><br> 060 * Path: <b>Resource._id</b><br> 061 * </p> 062 */ 063 public static final StringClientParam RES_ID = new StringClientParam(BaseResource.SP_RES_ID); 064 065 /** 066 * Search parameter constant for <b>_id</b> 067 */ 068 @SearchParamDefinition(name = "_id", path = "", description = "The ID of the resource", type = "string") 069 public static final String SP_RES_ID = "_id"; 070 071 @Child(name = "contained", order = 2, min = 0, max = 1) 072 private ContainedDt myContained; 073 074 private IdDt myId; 075 076 @Child(name = "language", order = 0, min = 0, max = 1) 077 private CodeDt myLanguage; 078 079 private ResourceMetadataMap myResourceMetadata; 080 081 @Child(name = "text", order = 1, min = 0, max = 1) 082 private NarrativeDt myText; 083 084 @Override 085 public ContainedDt getContained() { 086 if (myContained == null) { 087 myContained = new ContainedDt(); 088 } 089 return myContained; 090 } 091 092 @Override 093 public IdDt getId() { 094 if (myId == null) { 095 myId = new IdDt(); 096 } 097 return myId; 098 } 099 100 @Override 101 public IIdType getIdElement() { 102 return getId(); 103 } 104 105 @Override 106 public CodeDt getLanguage() { 107 if (myLanguage == null) { 108 myLanguage = new CodeDt(); 109 } 110 return myLanguage; 111 } 112 113 @Override 114 public IBaseMetaType getMeta() { 115 return new IBaseMetaType() { 116 117 private static final long serialVersionUID = 1L; 118 119 @Override 120 public IBaseMetaType addProfile(String theProfile) { 121 ArrayList<IdDt> newTagList = new ArrayList<>(); 122 List<IdDt> existingTagList = ResourceMetadataKeyEnum.PROFILES.get(BaseResource.this); 123 if (existingTagList != null) { 124 newTagList.addAll(existingTagList); 125 } 126 ResourceMetadataKeyEnum.PROFILES.put(BaseResource.this, newTagList); 127 128 IdDt tag = new IdDt(theProfile); 129 newTagList.add(tag); 130 return this; 131 } 132 133 @Override 134 public IBaseCoding addSecurity() { 135 List<BaseCodingDt> tagList = ResourceMetadataKeyEnum.SECURITY_LABELS.get(BaseResource.this); 136 if (tagList == null) { 137 tagList = new ArrayList<>(); 138 ResourceMetadataKeyEnum.SECURITY_LABELS.put(BaseResource.this, tagList); 139 } 140 CodingDt tag = new CodingDt(); 141 tagList.add(tag); 142 return tag; 143 } 144 145 @Override 146 public IBaseCoding addTag() { 147 TagList tagList = ResourceMetadataKeyEnum.TAG_LIST.get(BaseResource.this); 148 if (tagList == null) { 149 tagList = new TagList(); 150 ResourceMetadataKeyEnum.TAG_LIST.put(BaseResource.this, tagList); 151 } 152 Tag tag = new Tag(); 153 tagList.add(tag); 154 return tag; 155 } 156 157 @Override 158 public List<String> getFormatCommentsPost() { 159 return Collections.emptyList(); 160 } 161 162 @Override 163 public Object getUserData(String theName) { 164 throw new UnsupportedOperationException(Msg.code(582)); 165 } 166 167 @Override 168 public void setUserData(String theName, Object theValue) { 169 throw new UnsupportedOperationException(Msg.code(583)); 170 } 171 172 @Override 173 public List<String> getFormatCommentsPre() { 174 return Collections.emptyList(); 175 } 176 177 @Override 178 public Date getLastUpdated() { 179 InstantDt lu = ResourceMetadataKeyEnum.UPDATED.get(BaseResource.this); 180 if (lu != null) { 181 return lu.getValue(); 182 } 183 return null; 184 } 185 186 @Override 187 public List<? extends IPrimitiveType<String>> getProfile() { 188 ArrayList<IPrimitiveType<String>> retVal = new ArrayList<>(); 189 List<IdDt> profilesList = ResourceMetadataKeyEnum.PROFILES.get(BaseResource.this); 190 if (profilesList == null) { 191 return Collections.emptyList(); 192 } 193 for (IdDt next : profilesList) { 194 retVal.add(next); 195 } 196 return retVal; 197 } 198 199 @Override 200 public List<? extends IBaseCoding> getSecurity() { 201 ArrayList<CodingDt> retVal = new ArrayList<>(); 202 List<BaseCodingDt> labelsList = ResourceMetadataKeyEnum.SECURITY_LABELS.get(BaseResource.this); 203 if (labelsList == null) { 204 return Collections.emptyList(); 205 } 206 for (BaseCodingDt next : labelsList) { 207 CodingDt c = new CodingDt( 208 next.getSystemElement().getValue(), 209 next.getCodeElement().getValue()); 210 c.setDisplay(next.getDisplayElement().getValue()); 211 c.setUserSelected(next.getUserSelectedElement()); 212 c.setVersion(next.getVersionElement()); 213 retVal.add(c); 214 } 215 return retVal; 216 } 217 218 @Override 219 public IBaseCoding getSecurity(String theSystem, String theCode) { 220 for (IBaseCoding next : getSecurity()) { 221 if (theSystem.equals(next.getSystem()) && theCode.equals(next.getCode())) { 222 return next; 223 } 224 } 225 return null; 226 } 227 228 @Override 229 public List<? extends IBaseCoding> getTag() { 230 ArrayList<IBaseCoding> retVal = new ArrayList<>(); 231 TagList tagList = ResourceMetadataKeyEnum.TAG_LIST.get(BaseResource.this); 232 if (tagList == null) { 233 return Collections.emptyList(); 234 } 235 for (Tag next : tagList) { 236 retVal.add(next); 237 } 238 return retVal; 239 } 240 241 @Override 242 public IBaseCoding getTag(String theSystem, String theCode) { 243 for (IBaseCoding next : getTag()) { 244 if (next.getSystem().equals(theSystem) && next.getCode().equals(theCode)) { 245 return next; 246 } 247 } 248 return null; 249 } 250 251 @Override 252 public String getVersionId() { 253 return getId().getVersionIdPart(); 254 } 255 256 @Override 257 public boolean hasFormatComment() { 258 return false; 259 } 260 261 @Override 262 public boolean isEmpty() { 263 return getResourceMetadata().isEmpty(); 264 } 265 266 @Override 267 public IBaseMetaType setLastUpdated(Date theHeaderDateValue) { 268 if (theHeaderDateValue == null) { 269 getResourceMetadata().remove(ResourceMetadataKeyEnum.UPDATED); 270 } else { 271 ResourceMetadataKeyEnum.UPDATED.put(BaseResource.this, new InstantDt(theHeaderDateValue)); 272 } 273 return this; 274 } 275 276 @Override 277 public IBaseMetaType setVersionId(String theVersionId) { 278 setId(getId().withVersion(theVersionId)); 279 return this; 280 } 281 }; 282 } 283 284 @Override 285 public ResourceMetadataMap getResourceMetadata() { 286 if (myResourceMetadata == null) { 287 myResourceMetadata = new ResourceMetadataMap(); 288 } 289 return myResourceMetadata; 290 } 291 292 @Override 293 public NarrativeDt getText() { 294 if (myText == null) { 295 myText = new NarrativeDt(); 296 } 297 return myText; 298 } 299 300 /** 301 * Intended to be called by extending classes {@link #isEmpty()} implementations, returns <code>true</code> if all 302 * content in this superclass instance is empty per the semantics of {@link #isEmpty()}. 303 */ 304 @Override 305 protected boolean isBaseEmpty() { 306 return super.isBaseEmpty() && ElementUtil.isEmpty(myLanguage, myText, myId); 307 } 308 309 public void setContained(ContainedDt theContained) { 310 myContained = theContained; 311 } 312 313 @Override 314 public void setId(IdDt theId) { 315 myId = theId; 316 } 317 318 @Override 319 public BaseResource setId(IIdType theId) { 320 if (theId instanceof IdDt) { 321 myId = (IdDt) theId; 322 } else if (theId != null) { 323 myId = new IdDt(theId.getValue()); 324 } else { 325 myId = null; 326 } 327 return this; 328 } 329 330 @Override 331 public BaseResource setId(String theId) { 332 if (theId == null) { 333 myId = null; 334 } else { 335 myId = new IdDt(theId); 336 } 337 return this; 338 } 339 340 @Override 341 public void setLanguage(CodeDt theLanguage) { 342 myLanguage = theLanguage; 343 } 344 345 @Override 346 public void setResourceMetadata(ResourceMetadataMap theMap) { 347 Validate.notNull(theMap, "The Map must not be null"); 348 myResourceMetadata = theMap; 349 } 350 351 public void setText(NarrativeDt theText) { 352 myText = theText; 353 } 354 355 @Override 356 public String toString() { 357 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 358 b.append("id", getId().toUnqualified()); 359 return b.toString(); 360 } 361}