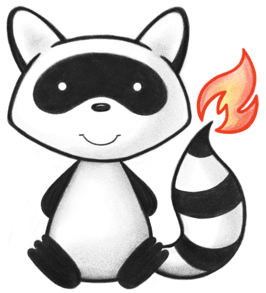
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum AdjudicationErrorCodesEnum { 009 010 /** 011 * Code Value: <b>A001</b> 012 */ 013 A001("A001", "http://hl7.org/fhir/adjudication-error"), 014 015 /** 016 * Code Value: <b>A002</b> 017 */ 018 A002("A002", "http://hl7.org/fhir/adjudication-error"), 019 020 ; 021 022 /** 023 * Identifier for this Value Set: 024 * 025 */ 026 public static final String VALUESET_IDENTIFIER = ""; 027 028 /** 029 * Name for this Value Set: 030 * Adjudication Error Codes 031 */ 032 public static final String VALUESET_NAME = "Adjudication Error Codes"; 033 034 private static Map<String, AdjudicationErrorCodesEnum> CODE_TO_ENUM = new HashMap<String, AdjudicationErrorCodesEnum>(); 035 private static Map<String, Map<String, AdjudicationErrorCodesEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, AdjudicationErrorCodesEnum>>(); 036 037 private final String myCode; 038 private final String mySystem; 039 040 static { 041 for (AdjudicationErrorCodesEnum next : AdjudicationErrorCodesEnum.values()) { 042 CODE_TO_ENUM.put(next.getCode(), next); 043 044 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 045 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, AdjudicationErrorCodesEnum>()); 046 } 047 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 048 } 049 } 050 051 /** 052 * Returns the code associated with this enumerated value 053 */ 054 public String getCode() { 055 return myCode; 056 } 057 058 /** 059 * Returns the code system associated with this enumerated value 060 */ 061 public String getSystem() { 062 return mySystem; 063 } 064 065 /** 066 * Returns the enumerated value associated with this code 067 */ 068 public static AdjudicationErrorCodesEnum forCode(String theCode) { 069 AdjudicationErrorCodesEnum retVal = CODE_TO_ENUM.get(theCode); 070 return retVal; 071 } 072 073 /** 074 * Converts codes to their respective enumerated values 075 */ 076 public static final IValueSetEnumBinder<AdjudicationErrorCodesEnum> VALUESET_BINDER = new IValueSetEnumBinder<AdjudicationErrorCodesEnum>() { 077 @Override 078 public String toCodeString(AdjudicationErrorCodesEnum theEnum) { 079 return theEnum.getCode(); 080 } 081 082 @Override 083 public String toSystemString(AdjudicationErrorCodesEnum theEnum) { 084 return theEnum.getSystem(); 085 } 086 087 @Override 088 public AdjudicationErrorCodesEnum fromCodeString(String theCodeString) { 089 return CODE_TO_ENUM.get(theCodeString); 090 } 091 092 @Override 093 public AdjudicationErrorCodesEnum fromCodeString(String theCodeString, String theSystemString) { 094 Map<String, AdjudicationErrorCodesEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 095 if (map == null) { 096 return null; 097 } 098 return map.get(theCodeString); 099 } 100 101 }; 102 103 /** 104 * Constructor 105 */ 106 AdjudicationErrorCodesEnum(String theCode, String theSystem) { 107 myCode = theCode; 108 mySystem = theSystem; 109 } 110 111 112}