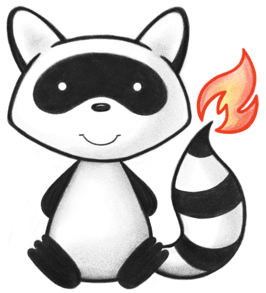
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum AdmitSourceEnum { 009 010 /** 011 * Display: <b>Transferred from other hospital</b><br> 012 * Code Value: <b>hosp-trans</b> 013 */ 014 TRANSFERRED_FROM_OTHER_HOSPITAL("hosp-trans", "http://hl7.org/fhir/admit-source"), 015 016 /** 017 * Display: <b>From accident/emergency department</b><br> 018 * Code Value: <b>emd</b> 019 */ 020 FROM_ACCIDENT_EMERGENCY_DEPARTMENT("emd", "http://hl7.org/fhir/admit-source"), 021 022 /** 023 * Display: <b>From outpatient department</b><br> 024 * Code Value: <b>outp</b> 025 */ 026 FROM_OUTPATIENT_DEPARTMENT("outp", "http://hl7.org/fhir/admit-source"), 027 028 /** 029 * Display: <b>Born in hospital</b><br> 030 * Code Value: <b>born</b> 031 */ 032 BORN_IN_HOSPITAL("born", "http://hl7.org/fhir/admit-source"), 033 034 /** 035 * Display: <b>General Practitioner referral</b><br> 036 * Code Value: <b>gp</b> 037 */ 038 GENERAL_PRACTITIONER_REFERRAL("gp", "http://hl7.org/fhir/admit-source"), 039 040 /** 041 * Display: <b>Medical Practitioner/physician referral</b><br> 042 * Code Value: <b>mp</b> 043 */ 044 MEDICAL_PRACTITIONER_PHYSICIAN_REFERRAL("mp", "http://hl7.org/fhir/admit-source"), 045 046 /** 047 * Display: <b>From nursing home</b><br> 048 * Code Value: <b>nursing</b> 049 */ 050 FROM_NURSING_HOME("nursing", "http://hl7.org/fhir/admit-source"), 051 052 /** 053 * Display: <b>From psychiatric hospital</b><br> 054 * Code Value: <b>psych</b> 055 */ 056 FROM_PSYCHIATRIC_HOSPITAL("psych", "http://hl7.org/fhir/admit-source"), 057 058 /** 059 * Display: <b>From rehabilitation facility</b><br> 060 * Code Value: <b>rehab</b> 061 */ 062 FROM_REHABILITATION_FACILITY("rehab", "http://hl7.org/fhir/admit-source"), 063 064 /** 065 * Display: <b>Other</b><br> 066 * Code Value: <b>other</b> 067 */ 068 OTHER("other", "http://hl7.org/fhir/admit-source"), 069 070 ; 071 072 /** 073 * Identifier for this Value Set: 074 * 075 */ 076 public static final String VALUESET_IDENTIFIER = ""; 077 078 /** 079 * Name for this Value Set: 080 * AdmitSource 081 */ 082 public static final String VALUESET_NAME = "AdmitSource"; 083 084 private static Map<String, AdmitSourceEnum> CODE_TO_ENUM = new HashMap<String, AdmitSourceEnum>(); 085 private static Map<String, Map<String, AdmitSourceEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, AdmitSourceEnum>>(); 086 087 private final String myCode; 088 private final String mySystem; 089 090 static { 091 for (AdmitSourceEnum next : AdmitSourceEnum.values()) { 092 CODE_TO_ENUM.put(next.getCode(), next); 093 094 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 095 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, AdmitSourceEnum>()); 096 } 097 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 098 } 099 } 100 101 /** 102 * Returns the code associated with this enumerated value 103 */ 104 public String getCode() { 105 return myCode; 106 } 107 108 /** 109 * Returns the code system associated with this enumerated value 110 */ 111 public String getSystem() { 112 return mySystem; 113 } 114 115 /** 116 * Returns the enumerated value associated with this code 117 */ 118 public static AdmitSourceEnum forCode(String theCode) { 119 AdmitSourceEnum retVal = CODE_TO_ENUM.get(theCode); 120 return retVal; 121 } 122 123 /** 124 * Converts codes to their respective enumerated values 125 */ 126 public static final IValueSetEnumBinder<AdmitSourceEnum> VALUESET_BINDER = new IValueSetEnumBinder<AdmitSourceEnum>() { 127 @Override 128 public String toCodeString(AdmitSourceEnum theEnum) { 129 return theEnum.getCode(); 130 } 131 132 @Override 133 public String toSystemString(AdmitSourceEnum theEnum) { 134 return theEnum.getSystem(); 135 } 136 137 @Override 138 public AdmitSourceEnum fromCodeString(String theCodeString) { 139 return CODE_TO_ENUM.get(theCodeString); 140 } 141 142 @Override 143 public AdmitSourceEnum fromCodeString(String theCodeString, String theSystemString) { 144 Map<String, AdmitSourceEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 145 if (map == null) { 146 return null; 147 } 148 return map.get(theCodeString); 149 } 150 151 }; 152 153 /** 154 * Constructor 155 */ 156 AdmitSourceEnum(String theCode, String theSystem) { 157 myCode = theCode; 158 mySystem = theSystem; 159 } 160 161 162}