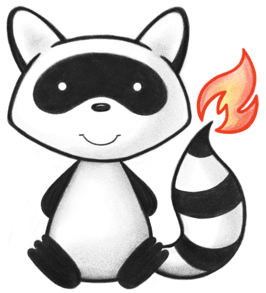
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum AnswerFormatEnum { 009 010 /** 011 * Display: <b>Boolean</b><br> 012 * Code Value: <b>boolean</b> 013 * 014 * Answer is a yes/no answer. 015 */ 016 BOOLEAN("boolean", "http://hl7.org/fhir/answer-format"), 017 018 /** 019 * Display: <b>Decimal</b><br> 020 * Code Value: <b>decimal</b> 021 * 022 * Answer is a floating point number. 023 */ 024 DECIMAL("decimal", "http://hl7.org/fhir/answer-format"), 025 026 /** 027 * Display: <b>Integer</b><br> 028 * Code Value: <b>integer</b> 029 * 030 * Answer is an integer. 031 */ 032 INTEGER("integer", "http://hl7.org/fhir/answer-format"), 033 034 /** 035 * Display: <b>Date</b><br> 036 * Code Value: <b>date</b> 037 * 038 * Answer is a date. 039 */ 040 DATE("date", "http://hl7.org/fhir/answer-format"), 041 042 /** 043 * Display: <b>Date Time</b><br> 044 * Code Value: <b>dateTime</b> 045 * 046 * Answer is a date and time. 047 */ 048 DATE_TIME("dateTime", "http://hl7.org/fhir/answer-format"), 049 050 /** 051 * Display: <b>Instant</b><br> 052 * Code Value: <b>instant</b> 053 * 054 * Answer is a system timestamp. 055 */ 056 INSTANT("instant", "http://hl7.org/fhir/answer-format"), 057 058 /** 059 * Display: <b>Time</b><br> 060 * Code Value: <b>time</b> 061 * 062 * Answer is a time (hour/minute/second) independent of date. 063 */ 064 TIME("time", "http://hl7.org/fhir/answer-format"), 065 066 /** 067 * Display: <b>String</b><br> 068 * Code Value: <b>string</b> 069 * 070 * Answer is a short (few words to short sentence) free-text entry. 071 */ 072 STRING("string", "http://hl7.org/fhir/answer-format"), 073 074 /** 075 * Display: <b>Text</b><br> 076 * Code Value: <b>text</b> 077 * 078 * Answer is a long (potentially multi-paragraph) free-text entry (still captured as a string). 079 */ 080 TEXT("text", "http://hl7.org/fhir/answer-format"), 081 082 /** 083 * Display: <b>Url</b><br> 084 * Code Value: <b>url</b> 085 * 086 * Answer is a url (website, FTP site, etc.). 087 */ 088 URL("url", "http://hl7.org/fhir/answer-format"), 089 090 /** 091 * Display: <b>Choice</b><br> 092 * Code Value: <b>choice</b> 093 * 094 * Answer is a Coding drawn from a list of options. 095 */ 096 CHOICE("choice", "http://hl7.org/fhir/answer-format"), 097 098 /** 099 * Display: <b>Open Choice</b><br> 100 * Code Value: <b>open-choice</b> 101 * 102 * Answer is a Coding drawn from a list of options or a free-text entry. 103 */ 104 OPEN_CHOICE("open-choice", "http://hl7.org/fhir/answer-format"), 105 106 /** 107 * Display: <b>Attachment</b><br> 108 * Code Value: <b>attachment</b> 109 * 110 * Answer is binary content such as a image, PDF, etc. 111 */ 112 ATTACHMENT("attachment", "http://hl7.org/fhir/answer-format"), 113 114 /** 115 * Display: <b>Reference</b><br> 116 * Code Value: <b>reference</b> 117 * 118 * Answer is a reference to another resource (practitioner, organization, etc.). 119 */ 120 REFERENCE("reference", "http://hl7.org/fhir/answer-format"), 121 122 /** 123 * Display: <b>Quantity</b><br> 124 * Code Value: <b>quantity</b> 125 * 126 * Answer is a combination of a numeric value and unit, potentially with a comparator (<, >, etc.). 127 */ 128 QUANTITY("quantity", "http://hl7.org/fhir/answer-format"), 129 130 ; 131 132 /** 133 * Identifier for this Value Set: 134 * 135 */ 136 public static final String VALUESET_IDENTIFIER = ""; 137 138 /** 139 * Name for this Value Set: 140 * AnswerFormat 141 */ 142 public static final String VALUESET_NAME = "AnswerFormat"; 143 144 private static Map<String, AnswerFormatEnum> CODE_TO_ENUM = new HashMap<String, AnswerFormatEnum>(); 145 private static Map<String, Map<String, AnswerFormatEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, AnswerFormatEnum>>(); 146 147 private final String myCode; 148 private final String mySystem; 149 150 static { 151 for (AnswerFormatEnum next : AnswerFormatEnum.values()) { 152 CODE_TO_ENUM.put(next.getCode(), next); 153 154 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 155 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, AnswerFormatEnum>()); 156 } 157 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 158 } 159 } 160 161 /** 162 * Returns the code associated with this enumerated value 163 */ 164 public String getCode() { 165 return myCode; 166 } 167 168 /** 169 * Returns the code system associated with this enumerated value 170 */ 171 public String getSystem() { 172 return mySystem; 173 } 174 175 /** 176 * Returns the enumerated value associated with this code 177 */ 178 public static AnswerFormatEnum forCode(String theCode) { 179 AnswerFormatEnum retVal = CODE_TO_ENUM.get(theCode); 180 return retVal; 181 } 182 183 /** 184 * Converts codes to their respective enumerated values 185 */ 186 public static final IValueSetEnumBinder<AnswerFormatEnum> VALUESET_BINDER = new IValueSetEnumBinder<AnswerFormatEnum>() { 187 @Override 188 public String toCodeString(AnswerFormatEnum theEnum) { 189 return theEnum.getCode(); 190 } 191 192 @Override 193 public String toSystemString(AnswerFormatEnum theEnum) { 194 return theEnum.getSystem(); 195 } 196 197 @Override 198 public AnswerFormatEnum fromCodeString(String theCodeString) { 199 return CODE_TO_ENUM.get(theCodeString); 200 } 201 202 @Override 203 public AnswerFormatEnum fromCodeString(String theCodeString, String theSystemString) { 204 Map<String, AnswerFormatEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 205 if (map == null) { 206 return null; 207 } 208 return map.get(theCodeString); 209 } 210 211 }; 212 213 /** 214 * Constructor 215 */ 216 AnswerFormatEnum(String theCode, String theSystem) { 217 myCode = theCode; 218 mySystem = theSystem; 219 } 220 221 222}