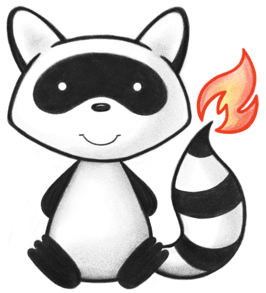
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum AuditEventObjectLifecycleEnum { 009 010 /** 011 * Display: <b>Origination / Creation</b><br> 012 * Code Value: <b>1</b> 013 * 014 * Origination / Creation 015 */ 016 ORIGINATION___CREATION("1", "http://hl7.org/fhir/object-lifecycle"), 017 018 /** 019 * Display: <b>Import / Copy from original</b><br> 020 * Code Value: <b>2</b> 021 * 022 * Import / Copy from original 023 */ 024 IMPORT___COPY_FROM_ORIGINAL("2", "http://hl7.org/fhir/object-lifecycle"), 025 026 /** 027 * Display: <b>Amendment</b><br> 028 * Code Value: <b>3</b> 029 * 030 * Amendment 031 */ 032 AMENDMENT("3", "http://hl7.org/fhir/object-lifecycle"), 033 034 /** 035 * Display: <b>Verification</b><br> 036 * Code Value: <b>4</b> 037 * 038 * Verification 039 */ 040 VERIFICATION("4", "http://hl7.org/fhir/object-lifecycle"), 041 042 /** 043 * Display: <b>Translation</b><br> 044 * Code Value: <b>5</b> 045 * 046 * Translation 047 */ 048 TRANSLATION("5", "http://hl7.org/fhir/object-lifecycle"), 049 050 /** 051 * Display: <b>Access / Use</b><br> 052 * Code Value: <b>6</b> 053 * 054 * Access / Use 055 */ 056 ACCESS___USE("6", "http://hl7.org/fhir/object-lifecycle"), 057 058 /** 059 * Display: <b>De-identification</b><br> 060 * Code Value: <b>7</b> 061 * 062 * De-identification 063 */ 064 DE_IDENTIFICATION("7", "http://hl7.org/fhir/object-lifecycle"), 065 066 /** 067 * Display: <b>Aggregation, summarization, derivation</b><br> 068 * Code Value: <b>8</b> 069 * 070 * Aggregation, summarization, derivation 071 */ 072 AGGREGATION__SUMMARIZATION__DERIVATION("8", "http://hl7.org/fhir/object-lifecycle"), 073 074 /** 075 * Display: <b>Report</b><br> 076 * Code Value: <b>9</b> 077 * 078 * Report 079 */ 080 REPORT("9", "http://hl7.org/fhir/object-lifecycle"), 081 082 /** 083 * Display: <b>Export / Copy to target</b><br> 084 * Code Value: <b>10</b> 085 * 086 * Export / Copy to target 087 */ 088 EXPORT___COPY_TO_TARGET("10", "http://hl7.org/fhir/object-lifecycle"), 089 090 /** 091 * Display: <b>Disclosure</b><br> 092 * Code Value: <b>11</b> 093 * 094 * Disclosure 095 */ 096 DISCLOSURE("11", "http://hl7.org/fhir/object-lifecycle"), 097 098 /** 099 * Display: <b>Receipt of disclosure</b><br> 100 * Code Value: <b>12</b> 101 * 102 * Receipt of disclosure 103 */ 104 RECEIPT_OF_DISCLOSURE("12", "http://hl7.org/fhir/object-lifecycle"), 105 106 /** 107 * Display: <b>Archiving</b><br> 108 * Code Value: <b>13</b> 109 * 110 * Archiving 111 */ 112 ARCHIVING("13", "http://hl7.org/fhir/object-lifecycle"), 113 114 /** 115 * Display: <b>Logical deletion</b><br> 116 * Code Value: <b>14</b> 117 * 118 * Logical deletion 119 */ 120 LOGICAL_DELETION("14", "http://hl7.org/fhir/object-lifecycle"), 121 122 /** 123 * Display: <b>Permanent erasure / Physical destruction</b><br> 124 * Code Value: <b>15</b> 125 * 126 * Permanent erasure / Physical destruction 127 */ 128 PERMANENT_ERASURE___PHYSICAL_DESTRUCTION("15", "http://hl7.org/fhir/object-lifecycle"), 129 130 ; 131 132 /** 133 * Identifier for this Value Set: 134 * 135 */ 136 public static final String VALUESET_IDENTIFIER = ""; 137 138 /** 139 * Name for this Value Set: 140 * AuditEventObjectLifecycle 141 */ 142 public static final String VALUESET_NAME = "AuditEventObjectLifecycle"; 143 144 private static Map<String, AuditEventObjectLifecycleEnum> CODE_TO_ENUM = new HashMap<String, AuditEventObjectLifecycleEnum>(); 145 private static Map<String, Map<String, AuditEventObjectLifecycleEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, AuditEventObjectLifecycleEnum>>(); 146 147 private final String myCode; 148 private final String mySystem; 149 150 static { 151 for (AuditEventObjectLifecycleEnum next : AuditEventObjectLifecycleEnum.values()) { 152 CODE_TO_ENUM.put(next.getCode(), next); 153 154 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 155 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, AuditEventObjectLifecycleEnum>()); 156 } 157 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 158 } 159 } 160 161 /** 162 * Returns the code associated with this enumerated value 163 */ 164 public String getCode() { 165 return myCode; 166 } 167 168 /** 169 * Returns the code system associated with this enumerated value 170 */ 171 public String getSystem() { 172 return mySystem; 173 } 174 175 /** 176 * Returns the enumerated value associated with this code 177 */ 178 public static AuditEventObjectLifecycleEnum forCode(String theCode) { 179 AuditEventObjectLifecycleEnum retVal = CODE_TO_ENUM.get(theCode); 180 return retVal; 181 } 182 183 /** 184 * Converts codes to their respective enumerated values 185 */ 186 public static final IValueSetEnumBinder<AuditEventObjectLifecycleEnum> VALUESET_BINDER = new IValueSetEnumBinder<AuditEventObjectLifecycleEnum>() { 187 @Override 188 public String toCodeString(AuditEventObjectLifecycleEnum theEnum) { 189 return theEnum.getCode(); 190 } 191 192 @Override 193 public String toSystemString(AuditEventObjectLifecycleEnum theEnum) { 194 return theEnum.getSystem(); 195 } 196 197 @Override 198 public AuditEventObjectLifecycleEnum fromCodeString(String theCodeString) { 199 return CODE_TO_ENUM.get(theCodeString); 200 } 201 202 @Override 203 public AuditEventObjectLifecycleEnum fromCodeString(String theCodeString, String theSystemString) { 204 Map<String, AuditEventObjectLifecycleEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 205 if (map == null) { 206 return null; 207 } 208 return map.get(theCodeString); 209 } 210 211 }; 212 213 /** 214 * Constructor 215 */ 216 AuditEventObjectLifecycleEnum(String theCode, String theSystem) { 217 myCode = theCode; 218 mySystem = theSystem; 219 } 220 221 222}