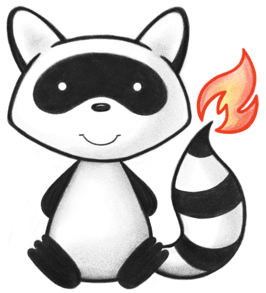
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum AuditEventObjectRoleEnum { 009 010 /** 011 * Display: <b>Patient</b><br> 012 * Code Value: <b>1</b> 013 * 014 * This object is the patient that is the subject of care related to this event. It is identifiable by patient ID or equivalent. The patient may be either human or animal. 015 */ 016 PATIENT("1", "http://hl7.org/fhir/object-role"), 017 018 /** 019 * Display: <b>Location</b><br> 020 * Code Value: <b>2</b> 021 * 022 * This is a location identified as related to the event. This is usually the location where the event took place. Note that for shipping, the usual events are arrival at a location or departure from a location. 023 */ 024 LOCATION("2", "http://hl7.org/fhir/object-role"), 025 026 /** 027 * Display: <b>Report</b><br> 028 * Code Value: <b>3</b> 029 * 030 * This object is any kind of persistent document created as a result of the event. This could be a paper report, film, electronic report, DICOM Study, etc. Issues related to medical records life cycle management are conveyed elsewhere. 031 */ 032 REPORT("3", "http://hl7.org/fhir/object-role"), 033 034 /** 035 * Display: <b>Domain Resource</b><br> 036 * Code Value: <b>4</b> 037 * 038 * A logical object related to a health record event. This is any healthcare specific resource (object) not restricted to FHIR defined Resources. 039 */ 040 DOMAIN_RESOURCE("4", "http://hl7.org/fhir/object-role"), 041 042 /** 043 * Display: <b>Master file</b><br> 044 * Code Value: <b>5</b> 045 * 046 * This is any configurable file used to control creation of documents. Examples include the objects maintained by the HL7 Master File transactions, Value Sets, etc. 047 */ 048 MASTER_FILE("5", "http://hl7.org/fhir/object-role"), 049 050 /** 051 * Display: <b>User</b><br> 052 * Code Value: <b>6</b> 053 * 054 * A human participant not otherwise identified by some other category. 055 */ 056 USER("6", "http://hl7.org/fhir/object-role"), 057 058 /** 059 * Display: <b>List</b><br> 060 * Code Value: <b>7</b> 061 * 062 * (deprecated) 063 */ 064 LIST("7", "http://hl7.org/fhir/object-role"), 065 066 /** 067 * Display: <b>Doctor</b><br> 068 * Code Value: <b>8</b> 069 * 070 * Typically a licensed person who is providing or performing care related to the event, generally a physician. The key distinction between doctor and practitioner is with regards to their role, not the licensing. The doctor is the human who actually performed the work. The practitioner is the human or organization that is responsible for the work. 071 */ 072 DOCTOR("8", "http://hl7.org/fhir/object-role"), 073 074 /** 075 * Display: <b>Subscriber</b><br> 076 * Code Value: <b>9</b> 077 * 078 * A person or system that is being notified as part of the event. This is relevant in situations where automated systems provide notifications to other parties when an event took place. 079 */ 080 SUBSCRIBER("9", "http://hl7.org/fhir/object-role"), 081 082 /** 083 * Display: <b>Guarantor</b><br> 084 * Code Value: <b>10</b> 085 * 086 * Insurance company, or any other organization who accepts responsibility for paying for the healthcare event. 087 */ 088 GUARANTOR("10", "http://hl7.org/fhir/object-role"), 089 090 /** 091 * Display: <b>Security User Entity</b><br> 092 * Code Value: <b>11</b> 093 * 094 * A person or active system object involved in the event with a security role. 095 */ 096 SECURITY_USER_ENTITY("11", "http://hl7.org/fhir/object-role"), 097 098 /** 099 * Display: <b>Security User Group</b><br> 100 * Code Value: <b>12</b> 101 * 102 * A person or system object involved in the event with the authority to modify security roles of other objects. 103 */ 104 SECURITY_USER_GROUP("12", "http://hl7.org/fhir/object-role"), 105 106 /** 107 * Display: <b>Security Resource</b><br> 108 * Code Value: <b>13</b> 109 * 110 * A passive object, such as a role table, that is relevant to the event. 111 */ 112 SECURITY_RESOURCE("13", "http://hl7.org/fhir/object-role"), 113 114 /** 115 * Display: <b>Security Granularity Definition</b><br> 116 * Code Value: <b>14</b> 117 * 118 * (deprecated) Relevant to certain RBAC security methodologies. 119 */ 120 SECURITY_GRANULARITY_DEFINITION("14", "http://hl7.org/fhir/object-role"), 121 122 /** 123 * Display: <b>Practitioner</b><br> 124 * Code Value: <b>15</b> 125 * 126 * Any person or organization responsible for providing care. This encompasses all forms of care, licensed or otherwise, and all sorts of teams and care groups. Note, the distinction between practitioners and the doctor that actually provided the care to the patient. 127 */ 128 PRACTITIONER("15", "http://hl7.org/fhir/object-role"), 129 130 /** 131 * Display: <b>Data Destination</b><br> 132 * Code Value: <b>16</b> 133 * 134 * The source or destination for data transfer, when it does not match some other role. 135 */ 136 DATA_DESTINATION("16", "http://hl7.org/fhir/object-role"), 137 138 /** 139 * Display: <b>Data Repository</b><br> 140 * Code Value: <b>17</b> 141 * 142 * A source or destination for data transfer that acts as an archive, database, or similar role. 143 */ 144 DATA_REPOSITORY("17", "http://hl7.org/fhir/object-role"), 145 146 /** 147 * Display: <b>Schedule</b><br> 148 * Code Value: <b>18</b> 149 * 150 * An object that holds schedule information. This could be an appointment book, availability information, etc. 151 */ 152 SCHEDULE("18", "http://hl7.org/fhir/object-role"), 153 154 /** 155 * Display: <b>Customer</b><br> 156 * Code Value: <b>19</b> 157 * 158 * An organization or person that is the recipient of services. This could be an organization that is buying services for a patient, or a person that is buying services for an animal. 159 */ 160 CUSTOMER("19", "http://hl7.org/fhir/object-role"), 161 162 /** 163 * Display: <b>Job</b><br> 164 * Code Value: <b>20</b> 165 * 166 * An order, task, work item, procedure step, or other description of work to be performed; e.g. a particular instance of an MPPS. 167 */ 168 JOB("20", "http://hl7.org/fhir/object-role"), 169 170 /** 171 * Display: <b>Job Stream</b><br> 172 * Code Value: <b>21</b> 173 * 174 * A list of jobs or a system that provides lists of jobs; e.g. an MWL SCP. 175 */ 176 JOB_STREAM("21", "http://hl7.org/fhir/object-role"), 177 178 /** 179 * Display: <b>Table</b><br> 180 * Code Value: <b>22</b> 181 * 182 * (Deprecated) 183 */ 184 TABLE("22", "http://hl7.org/fhir/object-role"), 185 186 /** 187 * Display: <b>Routing Criteria</b><br> 188 * Code Value: <b>23</b> 189 * 190 * An object that specifies or controls the routing or delivery of items. For example, a distribution list is the routing criteria for mail. The items delivered may be documents, jobs, or other objects. 191 */ 192 ROUTING_CRITERIA("23", "http://hl7.org/fhir/object-role"), 193 194 /** 195 * Display: <b>Query</b><br> 196 * Code Value: <b>24</b> 197 * 198 * The contents of a query. This is used to capture the contents of any kind of query. For security surveillance purposes knowing the queries being made is very important. 199 */ 200 QUERY("24", "http://hl7.org/fhir/object-role"), 201 202 ; 203 204 /** 205 * Identifier for this Value Set: 206 * 207 */ 208 public static final String VALUESET_IDENTIFIER = ""; 209 210 /** 211 * Name for this Value Set: 212 * AuditEventObjectRole 213 */ 214 public static final String VALUESET_NAME = "AuditEventObjectRole"; 215 216 private static Map<String, AuditEventObjectRoleEnum> CODE_TO_ENUM = new HashMap<String, AuditEventObjectRoleEnum>(); 217 private static Map<String, Map<String, AuditEventObjectRoleEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, AuditEventObjectRoleEnum>>(); 218 219 private final String myCode; 220 private final String mySystem; 221 222 static { 223 for (AuditEventObjectRoleEnum next : AuditEventObjectRoleEnum.values()) { 224 CODE_TO_ENUM.put(next.getCode(), next); 225 226 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 227 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, AuditEventObjectRoleEnum>()); 228 } 229 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 230 } 231 } 232 233 /** 234 * Returns the code associated with this enumerated value 235 */ 236 public String getCode() { 237 return myCode; 238 } 239 240 /** 241 * Returns the code system associated with this enumerated value 242 */ 243 public String getSystem() { 244 return mySystem; 245 } 246 247 /** 248 * Returns the enumerated value associated with this code 249 */ 250 public static AuditEventObjectRoleEnum forCode(String theCode) { 251 AuditEventObjectRoleEnum retVal = CODE_TO_ENUM.get(theCode); 252 return retVal; 253 } 254 255 /** 256 * Converts codes to their respective enumerated values 257 */ 258 public static final IValueSetEnumBinder<AuditEventObjectRoleEnum> VALUESET_BINDER = new IValueSetEnumBinder<AuditEventObjectRoleEnum>() { 259 @Override 260 public String toCodeString(AuditEventObjectRoleEnum theEnum) { 261 return theEnum.getCode(); 262 } 263 264 @Override 265 public String toSystemString(AuditEventObjectRoleEnum theEnum) { 266 return theEnum.getSystem(); 267 } 268 269 @Override 270 public AuditEventObjectRoleEnum fromCodeString(String theCodeString) { 271 return CODE_TO_ENUM.get(theCodeString); 272 } 273 274 @Override 275 public AuditEventObjectRoleEnum fromCodeString(String theCodeString, String theSystemString) { 276 Map<String, AuditEventObjectRoleEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 277 if (map == null) { 278 return null; 279 } 280 return map.get(theCodeString); 281 } 282 283 }; 284 285 /** 286 * Constructor 287 */ 288 AuditEventObjectRoleEnum(String theCode, String theSystem) { 289 myCode = theCode; 290 mySystem = theSystem; 291 } 292 293 294}