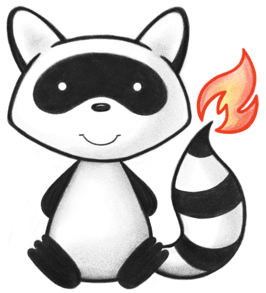
001 002package ca.uhn.fhir.model.dstu2.valueset; 003 004import ca.uhn.fhir.model.api.*; 005import java.util.HashMap; 006import java.util.Map; 007 008public enum AuditEventSourceTypeEnum { 009 010 /** 011 * Display: <b>User Device</b><br> 012 * Code Value: <b>1</b> 013 * 014 * End-user display device, diagnostic device. 015 */ 016 USER_DEVICE("1", "http://hl7.org/fhir/security-source-type"), 017 018 /** 019 * Display: <b>Data Interface</b><br> 020 * Code Value: <b>2</b> 021 * 022 * Data acquisition device or instrument. 023 */ 024 DATA_INTERFACE("2", "http://hl7.org/fhir/security-source-type"), 025 026 /** 027 * Display: <b>Web Server</b><br> 028 * Code Value: <b>3</b> 029 * 030 * Web Server process or thread. 031 */ 032 WEB_SERVER("3", "http://hl7.org/fhir/security-source-type"), 033 034 /** 035 * Display: <b>Application Server</b><br> 036 * Code Value: <b>4</b> 037 * 038 * Application Server process or thread. 039 */ 040 APPLICATION_SERVER("4", "http://hl7.org/fhir/security-source-type"), 041 042 /** 043 * Display: <b>Database Server</b><br> 044 * Code Value: <b>5</b> 045 * 046 * Database Server process or thread. 047 */ 048 DATABASE_SERVER("5", "http://hl7.org/fhir/security-source-type"), 049 050 /** 051 * Display: <b>Security Server</b><br> 052 * Code Value: <b>6</b> 053 * 054 * Security server, e.g. a domain controller. 055 */ 056 SECURITY_SERVER("6", "http://hl7.org/fhir/security-source-type"), 057 058 /** 059 * Display: <b>Network Device</b><br> 060 * Code Value: <b>7</b> 061 * 062 * ISO level 1-3 network component. 063 */ 064 NETWORK_DEVICE("7", "http://hl7.org/fhir/security-source-type"), 065 066 /** 067 * Display: <b>Network Router</b><br> 068 * Code Value: <b>8</b> 069 * 070 * ISO level 4-6 operating software. 071 */ 072 NETWORK_ROUTER("8", "http://hl7.org/fhir/security-source-type"), 073 074 /** 075 * Display: <b>Other</b><br> 076 * Code Value: <b>9</b> 077 * 078 * other kind of device (defined by DICOM, but some other code/system can be used). 079 */ 080 OTHER("9", "http://hl7.org/fhir/security-source-type"), 081 082 ; 083 084 /** 085 * Identifier for this Value Set: 086 * 087 */ 088 public static final String VALUESET_IDENTIFIER = ""; 089 090 /** 091 * Name for this Value Set: 092 * Audit Event Source Type 093 */ 094 public static final String VALUESET_NAME = "Audit Event Source Type"; 095 096 private static Map<String, AuditEventSourceTypeEnum> CODE_TO_ENUM = new HashMap<String, AuditEventSourceTypeEnum>(); 097 private static Map<String, Map<String, AuditEventSourceTypeEnum>> SYSTEM_TO_CODE_TO_ENUM = new HashMap<String, Map<String, AuditEventSourceTypeEnum>>(); 098 099 private final String myCode; 100 private final String mySystem; 101 102 static { 103 for (AuditEventSourceTypeEnum next : AuditEventSourceTypeEnum.values()) { 104 CODE_TO_ENUM.put(next.getCode(), next); 105 106 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 107 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, AuditEventSourceTypeEnum>()); 108 } 109 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 110 } 111 } 112 113 /** 114 * Returns the code associated with this enumerated value 115 */ 116 public String getCode() { 117 return myCode; 118 } 119 120 /** 121 * Returns the code system associated with this enumerated value 122 */ 123 public String getSystem() { 124 return mySystem; 125 } 126 127 /** 128 * Returns the enumerated value associated with this code 129 */ 130 public static AuditEventSourceTypeEnum forCode(String theCode) { 131 AuditEventSourceTypeEnum retVal = CODE_TO_ENUM.get(theCode); 132 return retVal; 133 } 134 135 /** 136 * Converts codes to their respective enumerated values 137 */ 138 public static final IValueSetEnumBinder<AuditEventSourceTypeEnum> VALUESET_BINDER = new IValueSetEnumBinder<AuditEventSourceTypeEnum>() { 139 @Override 140 public String toCodeString(AuditEventSourceTypeEnum theEnum) { 141 return theEnum.getCode(); 142 } 143 144 @Override 145 public String toSystemString(AuditEventSourceTypeEnum theEnum) { 146 return theEnum.getSystem(); 147 } 148 149 @Override 150 public AuditEventSourceTypeEnum fromCodeString(String theCodeString) { 151 return CODE_TO_ENUM.get(theCodeString); 152 } 153 154 @Override 155 public AuditEventSourceTypeEnum fromCodeString(String theCodeString, String theSystemString) { 156 Map<String, AuditEventSourceTypeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 157 if (map == null) { 158 return null; 159 } 160 return map.get(theCodeString); 161 } 162 163 }; 164 165 /** 166 * Constructor 167 */ 168 AuditEventSourceTypeEnum(String theCode, String theSystem) { 169 myCode = theCode; 170 mySystem = theSystem; 171 } 172 173 174}